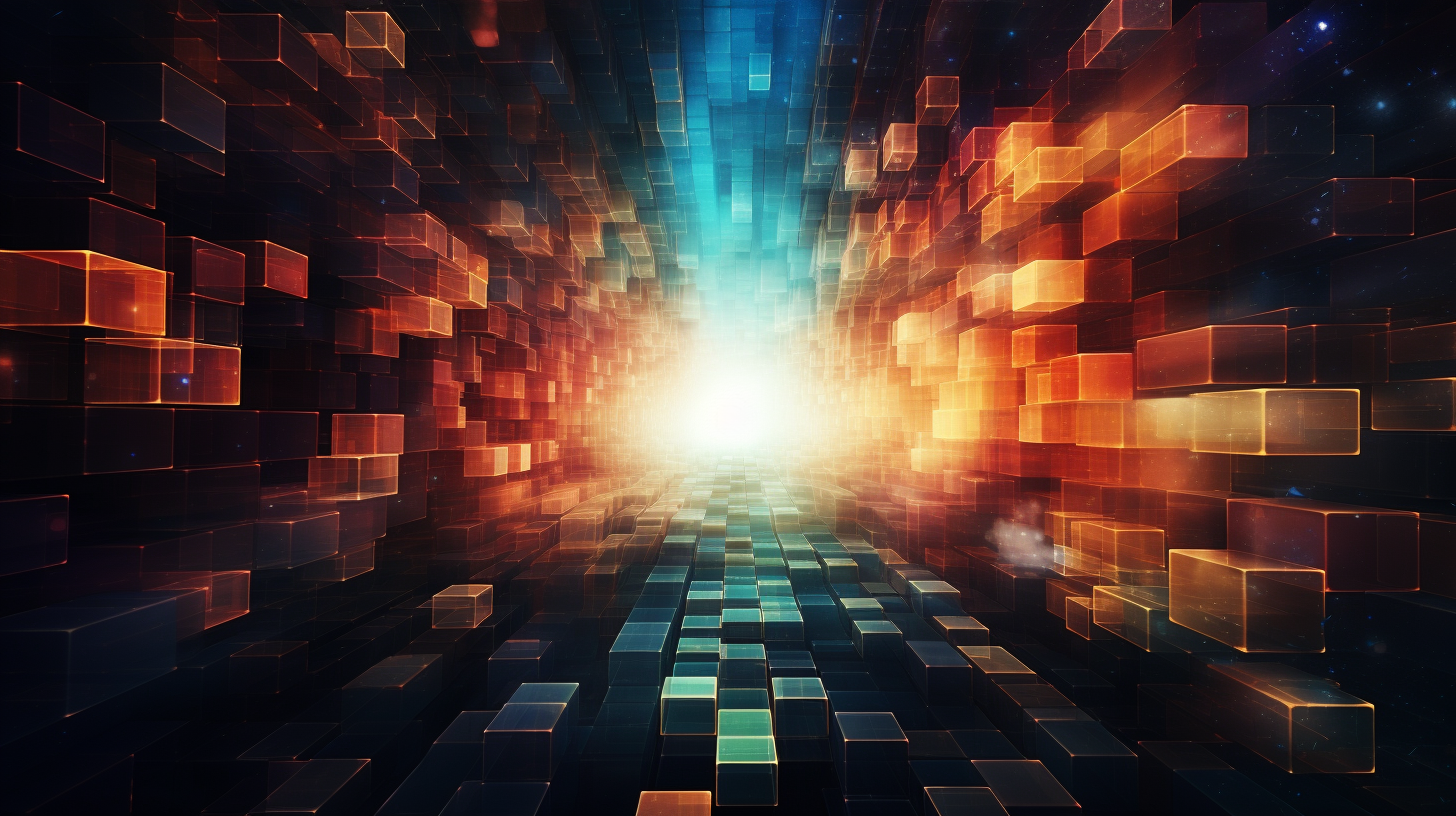
Introduction to Composer
Composer is a tool for dependency management in PHP. It allows you to declare the libraries your project depends on and it will manage (install/update) them for you. Composer is not a package manager in the same sense as Yum or Apt are. Yes, it deals with “packages” or libraries, but it manages them on a per-project basis, installing them in a directory (e.g. vendor
) inside your project. By default, it does not install anything globally. Thus, it’s a dependency manager.
Composer works by checking a file called composer.json
which is located in the root of your project. This file contains a JSON object that describes the dependencies of your project and may contain other metadata as well. The composer.json
file can also contain other configuration settings such as minimum-stability, autoload, and scripts.
{ "require": { "monolog/monolog": "1.0.*" } }
This is an example of what a simple composer.json
file might look like. In this example, we are telling Composer that our project requires the Monolog library, and we want to use any version in the 1.0.* range.
To use Composer, you first need to download and install it. Composer is available for download on their official website at getcomposer.org/download. Once installed, you can run Composer commands from your project’s root directory where the composer.json
file is located.
To install the dependencies defined in your composer.json
file, you would run the following command:
composer install
This command reads the composer.json
file from the current directory, resolves the dependencies, and installs them into the vendor
directory.
If you want to add a new dependency, you can run the require command like this:
composer require monolog/monolog
This command will automatically find the latest version of Monolog that matches your project’s requirements and update your composer.json
and composer.lock
files accordingly.
Composer also handles autoloading for you. After installing your dependencies, Composer generates a file called vendor/autoload.php
. By including this file in your project, all of the classes in your dependencies will be automatically loaded when needed.
require __DIR__ . '/vendor/autoload.php';
Composer is a powerful tool that simplifies dependency management for PHP projects. It makes it easy to manage third-party libraries and ensures that all team members are working with the same set of dependencies.
Managing Dependencies with Composer
Managing dependencies with Composer is a straightforward process that can save you a lot of time and hassle in the long run. Once you have a composer.json
file in your project directory, you can start adding, updating, and removing dependencies as needed.
To add a new dependency to your project, you use the require
command. This command updates your composer.json
file and installs the package. For example, if you want to add the Guzzle HTTP client to your project, you would run:
composer require guzzlehttp/guzzle
This command will find the latest version of Guzzle this is compatible with your project’s requirements and add it to your composer.json
file. It will also update your composer.lock
file, which locks the installed versions of your packages, ensuring consistency across installations.
To update a single dependency to its latest version, you can run the update
command with the package name:
composer update guzzlehttp/guzzle
If you want to update all of your dependencies at the same time, simply run:
composer update
This will check for newer versions of all the packages listed in your composer.json
file and update them if available. It’s a good practice to regularly update your dependencies to benefit from bug fixes and new features.
If at any point you need to remove a dependency from your project, Composer makes it easy. Just run the remove
command like so:
composer remove guzzlehttp/guzzle
This will remove Guzzle from your composer.json
and composer.lock
files and uninstall it from the vendor directory.
In addition to these basic commands, Composer also allows you to manage package versions with great flexibility. You can specify exact versions, version ranges, or even use wildcards. For example:
- Exact Version: “monolog/monolog”: “1.25.3”
- Version Range: “symfony/symfony”: “4.4.*”
- Wildcard Version: “laravel/framework”: “5.8.*”
- Minimum Stability: “minimum-stability”: “dev”
This level of control ensures that you can manage the stability and compatibility of your project with precision.
Once you’ve managed your dependencies, Composer’s autoload feature simplifies including them in your project. You just need to require the vendor/autoload.php
file, and Composer takes care of the rest.
require_once __DIR__ . '/vendor/autoload.php';
Managing dependencies with Composer is an essential skill for any PHP developer. With its easy-to-use commands for adding, updating, and removing packages, along with version control and autoloading features, Composer streamlines the process of maintaining a healthy codebase for your PHP projects.
Best Practices for Dependency Management in PHP
When it comes to managing dependencies in PHP, there are several best practices that can help ensure your project remains maintainable and scalable. Here are some key practices to follow:
- Keep your composer.json and composer.lock files up-to-date: It’s important to regularly update your dependencies to incorporate any security patches or bug fixes. Use
composer update
to update all dependencies, orcomposer update vendor/package
to update a specific package. - Commit your composer.lock file to version control: This file locks your dependencies to specific versions, ensuring that every member of your team is using the exact same versions. This minimizes the “it works on my machine” syndrome.
- Use version constraints wisely: It’s tempting to use wildcards or very loose version constraints to ensure you’re getting the latest versions of packages, but this can lead to unexpected issues when a package is updated. Instead, use more specific version constraints like
^1.2.3
which allows for updates that don’t break backward compatibility. - Avoid committing vendor directory: The
vendor/
directory can become quite large, and there’s no need to commit it to version control as it can be easily generated by runningcomposer install
. Instead, add/vendor/
to your.gitignore
file. - Use autoloading: Composer provides a powerful autoloading mechanism that eliminates the need for manual
require
statements. Simply include thevendor/autoload.php
file, and all of your dependencies will be loaded automatically. - Handle conflicts carefully: Sometimes, different packages may require different versions of the same dependency. Use the
conflict
key in yourcomposer.json
to prevent installation of incompatible versions. - Use scripts for automation: Composer allows you to define scripts that can automate tasks like clearing cache, running tests, or deploying your project. This helps in maintaining consistency across different environments.
- Think using private repositories: If you are working with proprietary code, you can use Composer’s repository configuration to manage private packages just as easily as public ones.
Here is an example of using a version constraint in your composer.json
:
{ "require": { "symfony/symfony": "^4.4" } }
And here is an example of using Composer scripts:
{ "scripts": { "test": "phpunit --configuration phpunit.xml", "deploy": "php deploy.php" } }
By following these best practices for dependency management in PHP, you can ensure that your project is robust, consistent, and easy to maintain for all developers involved.
Source: https://www.plcourses.com/php-and-dependency-management-composer/