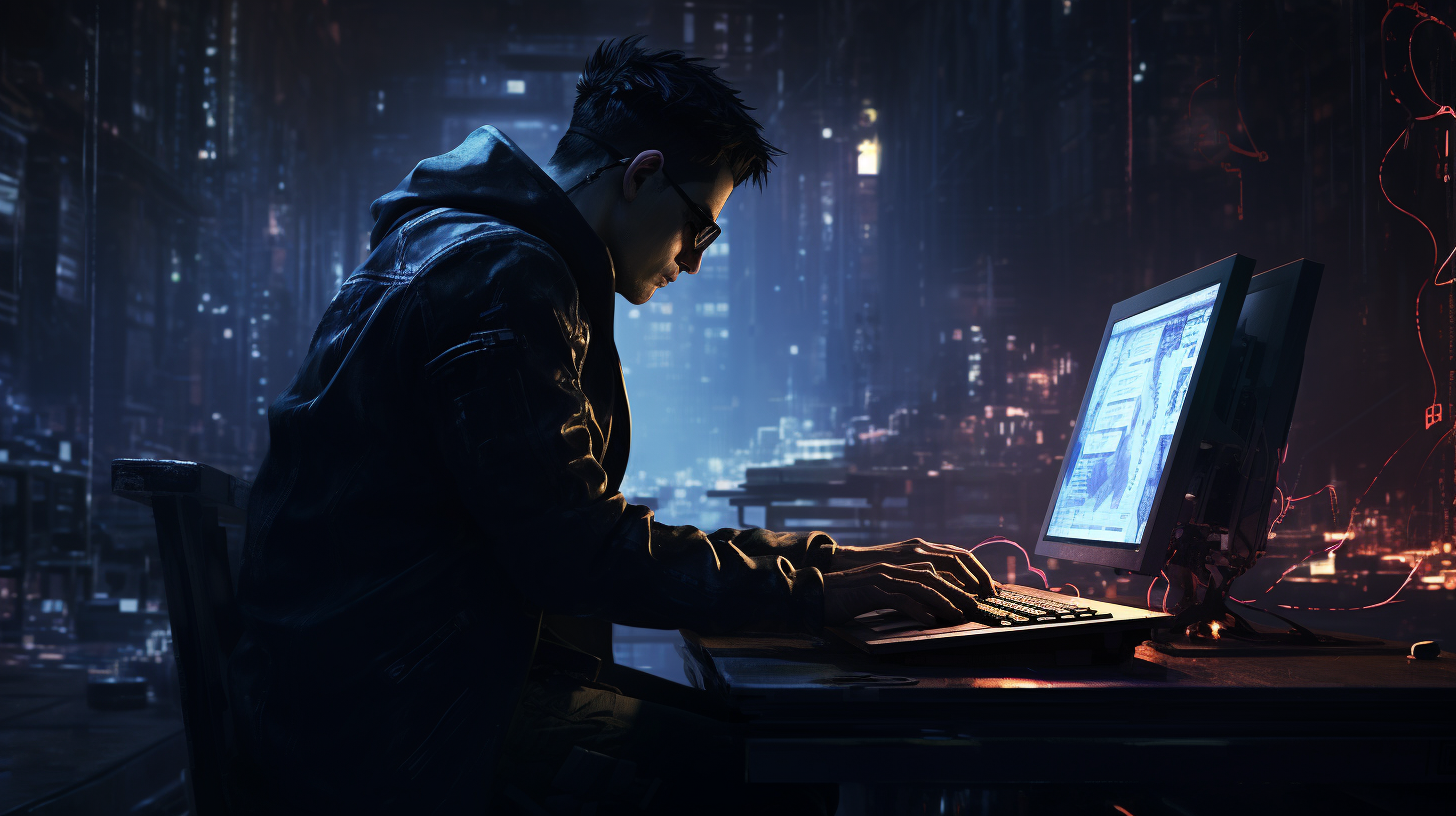
Overview of Control Structures in PHP
Control structures are fundamental elements in any programming language, including PHP. They allow developers to control the flow of execution of the script based on certain conditions. In PHP, there are several types of control structures, including conditional statements and loops. Conditional statements, such as if, else, and switch, are used to execute different blocks of code based on whether a condition is true or false. Loops, such as for, while, and foreach, are used to execute a block of code repeatedly until a certain condition is met.
In this section, we will focus on the conditional control structures: if, else, and switch.
- If – This is the most basic control structure. It executes a block of code only if a specified condition is true.
- Else – This is used in conjunction with if. It executes a block of code if the condition in the if statement is false.
- Switch – That is similar to a series of if statements but is cleaner and more manageable when you have a large number of conditions to check.
A simple example of an if statement in PHP is:
if ($a > $b) { echo "a is greater than b"; }
If the value of variable $a
is greater than $b
, the message “a is greater than b” will be printed.
Adding an else statement to the above example would look like this:
if ($a > $b) { echo "a is greater than b"; } else { echo "a is NOT greater than b"; }
Now, if $a
is not greater than $b
, the message “a is NOT greater than b” will be printed.
For multiple conditions, a switch statement is more suitable:
switch ($i) { case 0: echo "i equals 0"; break; case 1: echo "i equals 1"; break; case 2: echo "i equals 2"; break; default: echo "i is not equal to 0, 1 or 2"; }
This will check the value of $i
and execute the corresponding block of code based on its value.
Understanding and using these control structures effectively especially important for creating logical and efficient PHP scripts.
Using the If Statement
The if statement is the most fundamental control structure in PHP. It allows you to execute a block of code only if a specified condition is true. The syntax of an if statement is simple: you use the keyword if
, followed by a condition enclosed in parentheses, and then a block of code enclosed in curly braces.
if (condition) { // code to be executed if condition is true }
The condition can be any expression that evaluates to a boolean value of true
or false
. If the condition is true, the code within the block will be executed. If the condition is false, the code block will be skipped.
Here’s a practical example:
$age = 20; if ($age >= 18) { echo "You are eligible to vote."; }
In this example, the condition checks if the variable $age
is greater than or equal to 18. If this condition is true, the message “You are eligible to vote.” will be printed to the screen.
It’s also possible to use multiple conditions within an if statement by using logical operators such as and
(&&
), or
(||
), and not
(!
). For example:
$age = 20; $registered_voter = true; if ($age >= 18 && $registered_voter) { echo "You are eligible to vote."; } else { echo "You are not eligible to vote."; }
In the above code, the if statement checks if both conditions are true: the person is at least 18 years old and is a registered voter. If both conditions are met, the message “You are eligible to vote.” will be printed. Otherwise, the alternative message “You are not eligible to vote.” will be displayed.
The if statement is versatile and can be used in a variety of situations to control the flow of a PHP script. By understanding how to use the if statement effectively, you can create more dynamic and responsive applications.
Implementing the Else Statement
When the condition in the if statement is false, the else statement comes into play. The else statement is used to execute an alternative block of code. It is written immediately after the closing curly brace of the if block. It is important to note that the else statement does not have a condition; it simply serves as the default option when the if condition fails.
if ($temperature > 30) { echo "It's a hot day!"; } else { echo "It's not a hot day."; }
In the above example, if the value of $temperature
is greater than 30, the script will print “It’s a hot day!”. If the value of $temperature
is 30 or below, the else block will execute and print “It’s not a hot day.”.
It’s also possible to chain multiple conditions together using elseif. The elseif statement allows for additional condition checks if the initial if condition is false. Here’s an example:
if ($score >= 90) { echo "You got an A!"; } elseif ($score >= 80) { echo "You got a B!"; } elseif ($score >= 70) { echo "You got a C!"; } elseif ($score >= 60) { echo "You got a D!"; } else { echo "You failed the exam."; }
This example checks the value of $score
and prints a message based on the range it falls into. If none of the if or elseif conditions are true, the else block is executed, indicating that the student failed the exam.
The else and elseif statements provide additional flexibility to the conditional control structures in PHP, allowing developers to handle multiple scenarios efficiently in their code.
Understanding the Switch Statement
The switch statement is a control structure that enables you to execute different blocks of code based on the value of a single expression. It’s similar to using multiple if-elseif-else statements, but it is cleaner and more manageable, especially when dealing with multiple conditions. The basic syntax of a switch statement is as follows:
switch (expression) { case value1: // code to be executed if expression equals value1 break; case value2: // code to be executed if expression equals value2 break; // ... default: // code to be executed if expression doesn't match any case }
The expression is evaluated once, and its value is compared with the values specified in each case. If a match is found, the corresponding block of code is executed. The break statement at the end of each case block is crucial; it prevents the script from running into the next case block. If no matching case is found, the default block is executed. The default block is optional, but it is good practice to include it to handle unexpected values.
Here’s an example of a switch statement in action:
$day = "Monday"; switch ($day) { case "Monday": echo "Start of the work week."; break; case "Friday": echo "Last day of the work week!"; break; case "Saturday": case "Sunday": echo "It's the weekend!"; break; default: echo "It's a regular work day."; }
In this example, the value of $day
is “Monday”, so the first case block is executed, and “Start of the work week.” is printed. Note that the cases for “Saturday” and “Sunday” are combined—this is because the same block of code should be executed for both cases, which is a common use of switch statements to group multiple cases that share the same code.
The switch statement is a powerful tool in PHP that can greatly simplify the code and improve its readability when working with multiple conditions that rely on the same variable or expression.
Best Practices for Using Control Structures in PHP
When working with control structures in PHP, it’s important to follow best practices to ensure your code is efficient, readable, and easy to maintain. Here are some key best practices to keep in mind:
- Always use descriptive variable names that make it clear what the variable represents. This makes your code easier to understand and maintain.
$temperature = 28; if ($temperature > 30) { echo "It's a hot day!"; } else { echo "It's not a hot day."; }
if ($age >= 18 && $registered_voter) { echo "You are eligible to vote."; }
===
and !==
to avoid unexpected type conversions.if ($a === $b) { echo "a is equal to b"; }
if ($condition) { // code block }
switch ($userRole) { case 'admin': // admin-specific code break; case 'editor': // editor-specific code break; case 'subscriber': // subscriber-specific code break; default: // default code }
By adhering to these best practices, you’ll write control structures in PHP that are not only functional but also clean and maintainable. Remember, writing code is often a collaborative effort, and following these guidelines will make it easier for others to work with your code.
Source: https://www.plcourses.com/control-structures-in-php-if-else-switch/