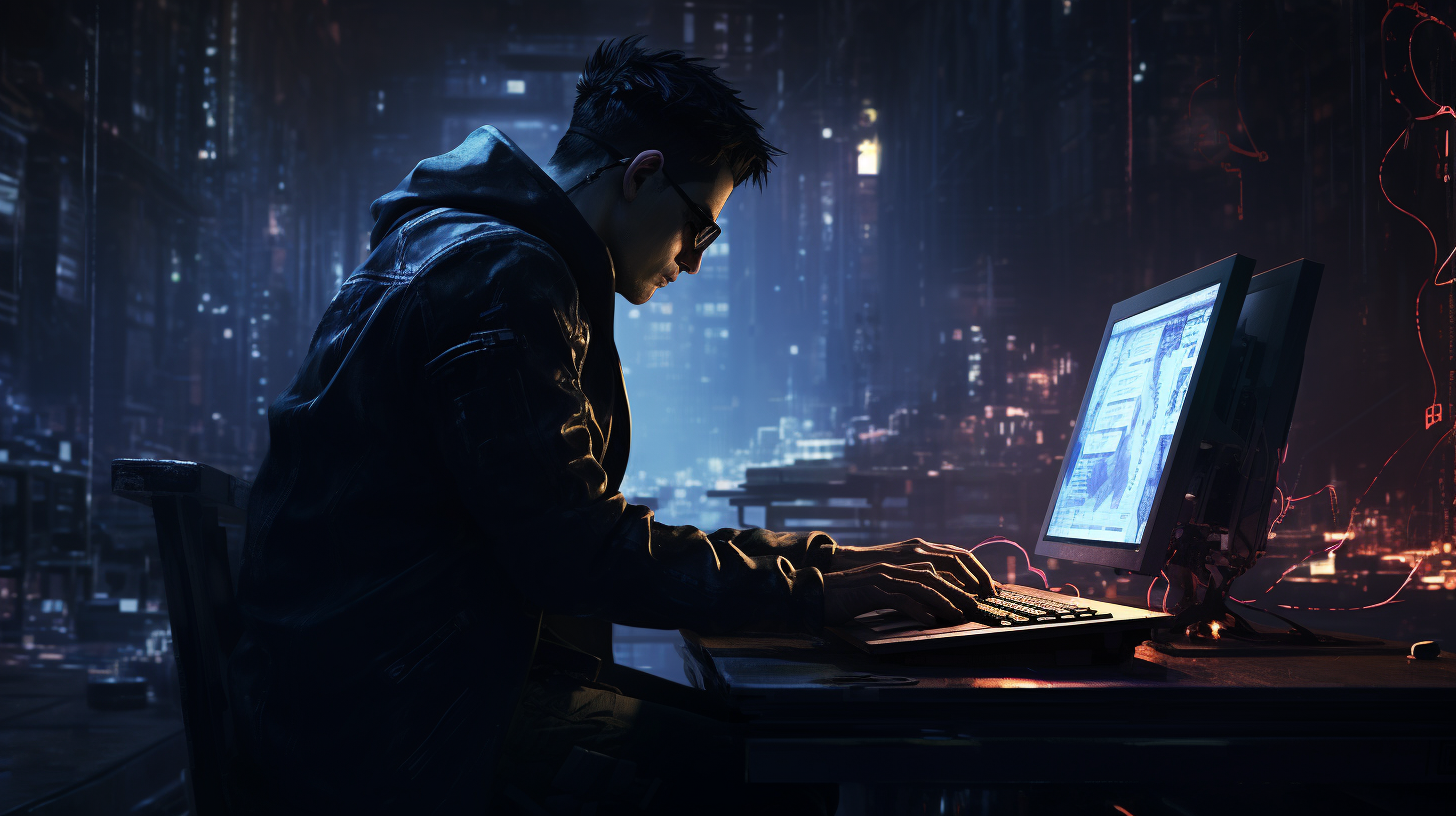
Understanding PHP Basics
When you’re just starting out with PHP, it is essential to grasp the fundamental concepts that form the foundation of this versatile scripting language. PHP is a server-side language, which means that it runs on a server rather than on the user’s browser. This allows PHP to interact with databases, manage files, and handle user input in a secure and efficient manner.
One of the first things you need to understand is the basic syntax of PHP. A PHP script starts with the <?php
tag and ends with the ?>
tag. Anything outside these tags is not processed as PHP code. Here’s a simple example:
<?php echo "Hello, world!"; ?>
Another key concept is that PHP is embedded within HTML. This means you can switch between HTML and PHP as needed within a single file, which typically has a .php
extension. Here’s an example of PHP and HTML mixed together:
<!DOCTYPE html> <html> <head> <title>My PHP Website</title> </head> <body> <?php echo "<h1>Welcome to my Website</h1>"; ?> <p>This is a regular HTML paragraph.</p> </body> </html>
It’s also important to understand how to comment your code. Comments are ignored by the PHP interpreter but are invaluable for explaining what your code does to anyone who reads it, including your future self. In PHP, you can create single-line comments using //
or #
, and multi-line comments using /* ... */
.
<?php // That's a single-line comment echo "Hello, world!"; // That is another single-line comment # This is also a single-line comment /* That's a multi-line comment block that spans over multiple lines. */ ?>
Lastly, it is crucial to understand the difference between echo and print in PHP. Both are used to output data to the screen. echo is slightly faster and can take multiple parameters, while print can only take one argument and always returns 1, making it useful in expressions. Here’s an example of both:
<?php echo "Hello, ", "world!"; print "Hello, world!"; ?>
Grasping these basics will set you up for success as you continue to learn and explore PHP. Remember, practice makes perfect, so keep writing and testing your code!
Common Syntax Errors
As a beginner in PHP, you’re bound to encounter syntax errors. These errors occur when the code is not written according to the rules of the PHP language. Syntax errors can be frustrating, but they’re also an essential part of the learning process. Let’s take a look at some of the most common syntax errors and how to fix them.
Missing Semicolons
One of the most common mistakes in PHP is forgetting to end a statement with a semicolon. In PHP, each statement must end with a semicolon, like this:
<?php echo "Hello, world!"; ?>
Forgetting the semicolon will result in a parse error, which will halt the execution of the script. The error message will usually tell you where the parser expected a semicolon, which can help you locate the mistake.
Unclosed Quotes
Another common error is forgetting to close quotes around strings. If you start a string with a single or double quote, you must also end it with the same type of quote:
<?php echo "This is a string"; echo 'This is also a string'; ?>
If you forget to close a quote, PHP will continue reading the rest of your code as if it were part of the string until it encounters another quote, which can lead to unexpected results or errors.
Missing or Extra Brackets
Brackets, or curly braces, are used to group statements together, such as in if statements or loops. It is important to make sure that you have an equal number of opening and closing brackets:
<?php if ($condition) { echo "Condition is true"; } ?>
If you have an extra or missing bracket, PHP will throw a syntax error. Pay close attention to the structure of your code to ensure that all brackets are properly paired.
Using Reserved Words
PHP has a list of keywords that have special meaning within the language. These keywords cannot be used as names for variables, functions, classes, or any other identifiers. For example, you cannot name a variable $echo
because echo
is a reserved word used to output strings:
<?php // Incorrect $echo = "Hello, world!"; // Correct $greeting = "Hello, world!"; echo $greeting; ?>
Using reserved words as identifiers will result in a syntax error. Refer to the PHP documentation for a complete list of reserved words to avoid this mistake.
Syntax errors are a natural part of the learning process. By understanding common mistakes and learning to read error messages carefully, you can quickly identify and correct these errors. Remember to always double-check your semicolons, quotes, brackets, and variable names. With practice, you’ll become more proficient in writing error-free PHP code.
Handling Variables and Data Types
Handling variables and data types in PHP is a fundamental skill that every developer should master. Variables are used to store data that can be manipulated and retrieved throughout your code. PHP is a loosely typed language, which means that you do not need to declare the data type of a variable. However, this flexibility can also lead to unexpected behaviors if not handled properly.
Let’s look at some common issues related to variables and data types and how to solve them.
- Implicit Type Conversion
PHP performs type juggling when it encounters a variable with a data type this is not expected. While this can be convenient, it can also lead to bugs if not accounted for. For example:
$a = "5" + 2; // PHP will treat the string "5" as the integer 5 echo $a; // Outputs 7
Developers should be aware of the type of data they are working with and perform explicit type casting when necessary to avoid confusion:
$a = (int)"5" + 2; // Explicitly casting string to integer echo $a; // Outputs 7
- Using Undefined Variables
PHP will issue a notice if you attempt to use a variable that has not been defined. This can happen if you misspell a variable name or forget to set it before use. To avoid this, always initialize your variables:
// Correct way to initialize a variable $name = ""; echo $name; // No notice since $name is defined
- Variable Variable Names
PHP allows the use of variable variable names, which can be confusing and lead to hard-to-debug code. A variable variable takes the value of a variable and treats it as the name of another variable:
$varName = 'foo'; $$varName = 'bar'; echo $foo; // Outputs 'bar'
While this feature can be useful in certain scenarios, it is generally best to avoid it unless absolutely necessary for readability and maintainability of your code.
- Checking Variable Types
When you need to ensure that a variable is of a certain type, use the is_type
functions provided by PHP, such as is_int()
, is_string()
, or is_array()
. This can prevent errors when working with functions that require specific types:
$number = 5; if (is_int($number)) { // Do something with the integer }
Handling variables and data types correctly very important for writing robust PHP code. By being aware of implicit type conversion, initializing variables, avoiding variable variable names, and checking variable types, you can prevent many common mistakes. Remember to always write clear and predictable code to make your development process smoother and your applications more reliable.
Troubleshooting Errors with Functions
When working with functions in PHP, it is common to encounter errors that can be difficult to troubleshoot. Functions are blocks of code that perform a specific task and can be reused throughout your script. Understanding how to debug and fix errors related to functions very important for any PHP developer. Here are some common issues with functions and their solutions:
- This error occurs when you try to call a function that has not been defined. Make sure you have included the file where the function is defined or check for any typos in the function name.
// Example of undefined function error myFunction(); // Error: Call to undefined function myFunction()
// Example of function name collision function myFunction() { // Some code } function myFunction() { // Error: Cannot redeclare myFunction() // Some code }
// Example of incorrect number of arguments function add($a, $b) { return $a + $b; } echo add(2); // Warning: Missing argument 2 for add() echo add(2, 3, 4); // Warning: Too many arguments to function add()
// Example of variable scope issue $var = "Hello, world!"; function myFunction() { echo $var; // Notice: Undefined variable: var } myFunction();
return
statement. Without it, the function will return NULL
by default.// Example of function not returning expected value function add($a, $b) { $sum = $a + $b; // Missing return statement } $result = add(2, 3); echo $result; // Outputs nothing since add() returns NULL
Debugging function errors often involves carefully checking the function definition, the way it is called, and the scope of the variables involved. Always test your functions thoroughly and ponder using unit tests to catch errors early in the development process. With practice and attention to detail, you’ll become adept at troubleshooting and resolving function-related issues in PHP.
Best Practices for PHP Development
As a PHP developer, following best practices very important for writing clean, maintainable, and efficient code. Here are some essential best practices to keep in mind:
- Choose names that clearly indicate the purpose of the variable or function. This makes your code easier to understand and maintain.
// Good naming $customerName = "Neil Hamilton"; function calculateTotal($prices) { // Code to calculate total } // Bad naming $cn = "Alex Stein"; function ct($p) { // Code to calculate total }
// Calculates the total with a 10% discount // Used for promotional sales function calculateDiscountedTotal($total) { return $total * 0.9; }
$username = filter_input(INPUT_POST, 'username', FILTER_SANITIZE_STRING); if (empty($username)) { // Handle error }
ini_set('display_errors', 1); error_reporting(E_ALL);
By following these best practices, you’ll write PHP code that’s not only functional but also clean and professional. This will benefit you, your team, and any future developers who may work with your code.
Source: https://www.plcourses.com/php-for-beginners-common-mistakes-and-solutions/