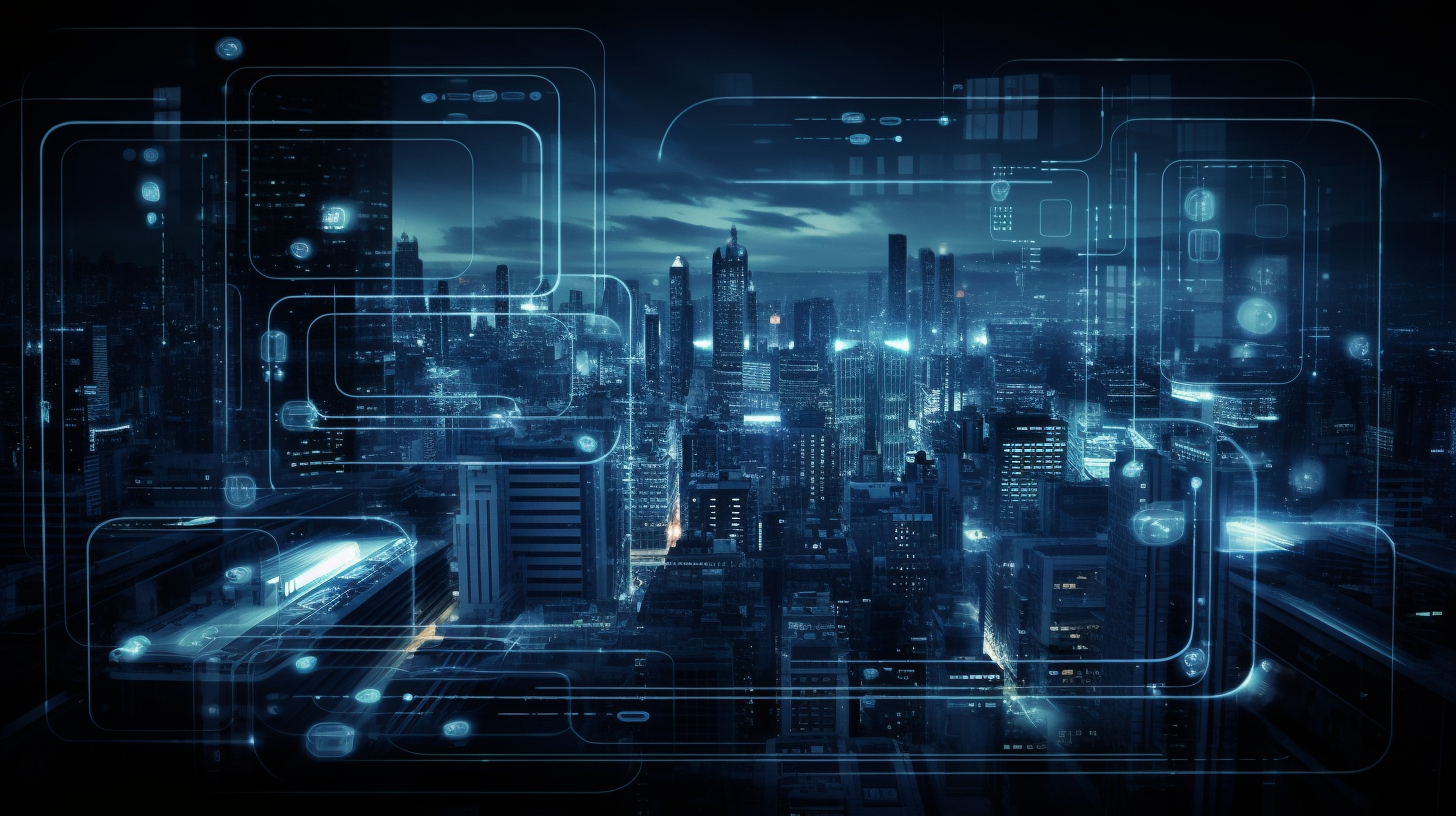
Understanding API Security
API security is a critical aspect of web development that ensures the safe exchange of data between different systems. APIs, or Application Programming Interfaces, are the tools that allow different software applications to communicate with each other. As such, they are a prime target for attackers looking to exploit vulnerabilities and gain unauthorized access to sensitive data.
At its core, API security revolves around three main principles:
- Confidentiality: Ensuring that the data being transmitted is only accessible to those with the correct authorization.
- Integrity: Guaranteeing that the data being sent is not tampered with during transmission.
- Availability: Making sure the API is accessible to users when they need it, and not disrupted by malicious attacks.
To achieve these principles, developers must implement various security measures, such as authentication and authorization protocols, data encryption, and input validation. In PHP, this can be done using built-in functions and libraries that help to protect against common threats.
For example, to ensure data integrity, PHP developers can use hash functions to create a unique fingerprint of the data being sent. If the data is altered in transit, the hash will not match, and the receiver will know that the integrity of the data has been compromised.
// Example of creating a hash in PHP $data = 'Sensitive API Data'; $hash = hash('sha256', $data); echo $hash;
Another important aspect of API security is input validation. This involves checking any data that is sent to the API to ensure it meets certain criteria and does not contain malicious code. PHP provides several functions for input validation, such as filter_var()
and preg_match()
.
// Example of input validation in PHP $input = '[email protected]'; if (filter_var($input, FILTER_VALIDATE_EMAIL)) { echo "Valid email address"; } else { echo "Invalid email address"; }
By understanding the principles of API security and utilizing PHP’s built-in functions and libraries, developers can create secure APIs that protect against common threats and ensure the safe exchange of data between systems.
Common Vulnerabilities in PHP APIs
When it comes to PHP APIs, there are several common vulnerabilities that developers need to be aware of. These vulnerabilities can lead to a range of issues, from data leaks to complete system takeovers. Below are some of the most prevalent security risks associated with PHP APIs.
- SQL Injection: This occurs when an attacker is able to insert a malicious SQL query through the API input, which can then be executed by the database. To prevent this, developers should use prepared statements and parameterized queries.
$stmt = $pdo->prepare('SELECT * FROM users WHERE email = :email'); $stmt->execute(['email' => $input]);
echo htmlspecialchars($userInput, ENT_QUOTES, 'UTF-8');
It is essential for developers to regularly review and update their API security measures to protect against these and other vulnerabilities. By being proactive and vigilant, developers can significantly reduce the risk of security breaches in their PHP APIs.
Best Practices for Securing PHP APIs
Securing PHP APIs requires a comprehensive approach that encompasses various best practices. These practices not only help to mitigate potential vulnerabilities but also ensure that the API is robust and can withstand attempted breaches. Here are some of the key best practices to follow:
- Use HTTPS: Always use HTTPS to encrypt data in transit. This prevents attackers from intercepting sensitive information. Ensure that your server is configured with a valid SSL/TLS certificate.
- Validate and Sanitize Input: Never trust the data received by your API. Use PHP’s filtering functions to validate and sanitize all inputs. This helps prevent SQL injection, XSS, and other injection attacks.
- Implement Rate Limiting: Protect your API from brute force and DDoS attacks by limiting the number of requests a user can make within a certain timeframe.
- Use Authentication and Authorization: Implement strong authentication mechanisms and ensure that users are authorized to access certain endpoints or resources.
- Keep Dependencies Updated: Regularly update all libraries and dependencies to patch known vulnerabilities.
- Handle Errors Gracefully: Customize error messages to avoid exposing stack traces or sensitive information. Log errors for monitoring but don’t disclose them to the end-user.
Below are examples of how you can implement some of these best practices in PHP:
// Example of using HTTPS if (empty($_SERVER['HTTPS']) || $_SERVER['HTTPS'] === "off") { header('HTTP/1.1 301 Moved Permanently'); header('Location: https://' . $_SERVER['HTTP_HOST'] . $_SERVER['REQUEST_URI']); exit; } // Example of input validation using filter_var() $input = $_GET['email']; if (!filter_var($input, FILTER_VALIDATE_EMAIL)) { // Handle invalid email echo "Invalid email address"; exit; } // Example of rate limiting $rateLimit = 100; // requests per hour $cacheKey = 'api-rate-limit-' . $_SERVER['REMOTE_ADDR']; $attempts = apcu_fetch($cacheKey) ?: 0; if ($attempts >= $rateLimit) { header('HTTP/1.1 429 Too Many Requests'); exit('Rate limit exceeded'); } apcu_store($cacheKey, ++$attempts, 3600);
Remember, security is an ongoing process. Regularly audit your code, stay informed about new vulnerabilities, and always follow security best practices when developing and maintaining your PHP APIs.
Authentication and Authorization Techniques
Authentication and authorization are critical components of API security. They ensure that only legitimate users can access your API, and that they can only perform actions they’re permitted to do. In PHP, there are several techniques we can use to implement these security measures.
API Keys
One common method is to require an API key with each request. This key is a unique identifier for each user and is checked on the server to confirm it’s valid.
// Example of checking an API key in PHP $providedApiKey = $_GET['api_key']; $validApiKeys = ['key1', 'key2', 'key3']; // List of valid API keys if (!in_array($providedApiKey, $validApiKeys)) { header('HTTP/1.1 401 Unauthorized'); exit('Invalid API Key'); }
Token-Based Authentication
Another approach is token-based authentication, such as OAuth 2.0 or JSON Web Tokens (JWT). After the initial login, the server issues a token which the client must send with each subsequent request.
// Example of validating a JWT in PHP use FirebaseJWTJWT; $jwt = getBearerToken(); // function to extract token from Authorization header $secretKey = 'your-secret-key'; try { $decoded = JWT::decode($jwt, $secretKey, array('HS256')); // Proceed if token is valid } catch(Exception $e) { header('HTTP/1.1 401 Unauthorized'); exit('Invalid token'); }
OAuth 2.0
OAuth 2.0 is a protocol that allows for secure authorization in a simple and standardized way from web, mobile, and desktop applications. Using libraries like league/oauth2-server
, you can set up a robust authorization server.
// Example of setting up an OAuth 2.0 server in PHP $server = new LeagueOAuth2ServerAuthorizationServer( $clientRepository, $accessTokenRepository, $scopeRepository, $privateKeyPath, $encryptionKey ); // ... setup grant types and response types ...
Role-Based Access Control (RBAC)
In addition to authentication, authorization defines what authenticated users are allowed to do. RBAC is a popular method where users are assigned roles, and those roles determine the permissions.
// Example of checking user role in PHP $userRole = getUserRole(); // function to get user role if ($userRole !== 'admin') { header('HTTP/1.1 403 Forbidden'); exit('Access denied'); }
Implementing these techniques properly requires careful planning and understanding of security principles. Always ensure that keys and tokens are stored securely, and consider using additional measures like two-factor authentication for even greater security.
Implementing Secure Communication with APIs
When communicating with APIs, it’s important to ensure that the data being exchanged is done so securely to prevent interception or tampering by malicious parties. There are several methods that PHP developers can use to implement secure communication with APIs, which help to maintain the confidentiality and integrity of the data.
SSL/TLS Encryption
One of the most fundamental steps in securing API communication is to use SSL/TLS encryption. This encrypts the data transmitted between the client and the server, making it unreadable to anyone who might intercept it. In PHP, you can ensure that your API requests are sent over HTTPS by using cURL with the appropriate options:
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, "https://api.example.com/data"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, true); // Verify the peer's SSL certificate $response = curl_exec($ch); curl_close($ch);
HTTP Headers
Setting HTTP headers can also enhance the security of your API communications. Headers such as Content-Security-Policy
, X-Content-Type-Options
, and X-Frame-Options
can prevent various types of attacks such as clickjacking and content sniffing.
header('Content-Security-Policy: default-src 'none';'); header('X-Content-Type-Options: nosniff'); header('X-Frame-Options: DENY');
API Tokens and HMAC
Using API tokens is a common method for authenticating requests. Tokens can be passed in HTTP headers to authenticate the request. Additionally, using Hash-based Message Authentication Code (HMAC) with a secret key can ensure that the message has not been altered in transit.
$apiToken = 'your-api-token'; $secretKey = 'your-secret-key'; $data = 'data-to-send'; $hash = hash_hmac('sha256', $data, $secretKey); $headers = [ 'Authorization: Bearer ' . $apiToken, 'X-Signature: ' . $hash, ]; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, "https://api.example.com/secure-data"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); $response = curl_exec($ch); curl_close($ch);
Public Key Infrastructure (PKI)
For even more robust security, you can implement a Public Key Infrastructure (PKI) where you encrypt the data with a public key and the server decrypts it with a private key. This ensures that only the server can read the data even if it’s intercepted.
// Encrypting data with public key $publicKey = openssl_pkey_get_public('file://path/to/public.pem'); $rawData = 'Sensitive information'; openssl_public_encrypt($rawData, $encryptedData, $publicKey); // Send $encryptedData via HTTPS
Securing API communication is a multi-faceted process that involves encryption, proper handling of authentication tokens, and setting secure HTTP headers. By implementing these methods in your PHP applications, you can greatly enhance the security of your API interactions and protect sensitive data from falling into the wrong hands.
Source: https://www.plcourses.com/php-and-api-security/