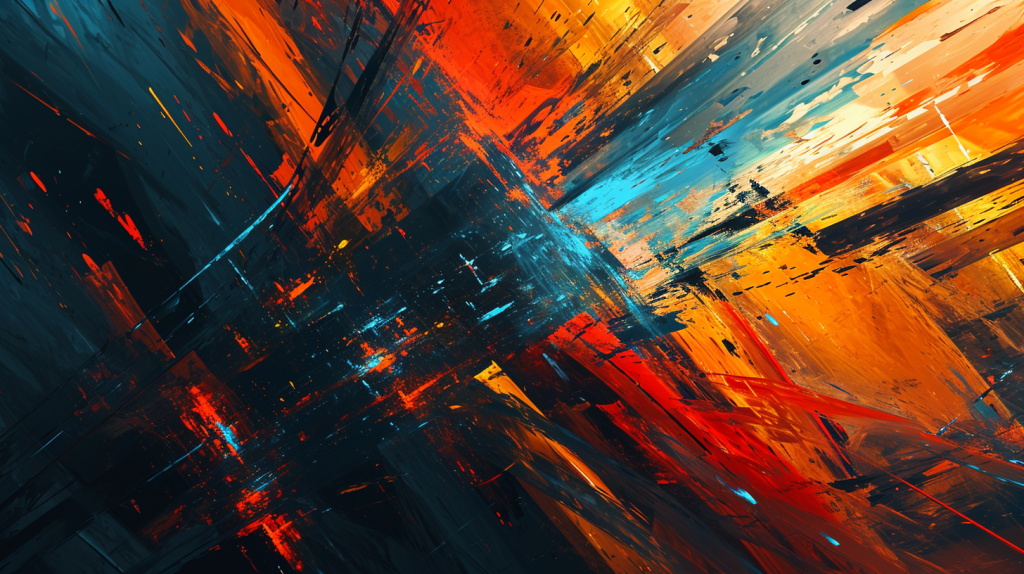
Flask provides a simple way to redirect users to a different URL using the redirect()
function. This function is useful when you want to navigate users from one endpoint to another – for example, after a form submission or when a resource has been moved to a new location. To use redirect()
, you must first import it from the Flask module:
from flask import Flask, redirect, url_for
Once imported, you can use redirect()
in your route functions by passing the desired destination URL or endpoint name to the function. For example:
@app.route('/old-page') def old_page(): return redirect(url_for('new_page')) @app.route('/new-page') def new_page(): return 'This is the new page location.'
In the above code, when users access /old-page
, they will be redirected to /new-page
by using the url_for()
function which generates URLs by the function’s name. It is important to use url_for()
instead of hardcoding URLs to make your application more maintainable and to avoid issues when the URL structure changes.
Redirects can also include HTTP response status codes. By default, Flask uses a 302 Found status code which indicates a temporary redirect. If a permanent redirect is necessary, for instance, if a page has been moved permanently, you should use a 301 Moved Permanently status code instead:
@app.route('/old-url') def old_url(): return redirect(url_for('new_url'), code=301) @app.route('/new-url') def new_url(): return 'The URL has been permanently moved here.'
A 301 redirect is helpful for SEO purposes because it transfers the SEO history from the old URL to the new URL. It’s also cached by browsers, reducing the load on the server over time.
It’s important to note that redirect()
only performs an HTTP redirect. If you need to pass additional data during the redirect process, you can use the session
object in Flask which allows you to store information between requests.
Here is an example of using session
with redirect()
:
from flask import session @app.route('/login', methods=['POST']) def login(): # ... authentication logic here ... session['user_id'] = user.id return redirect(url_for('dashboard')) @app.route('/dashboard') def dashboard(): user_id = session.get('user_id') # ... use the user_id as needed ... return 'Welcome to your dashboard.'
With the above code, after a user successfully logs in, their user ID is stored in the session and then they’re redirected to their dashboard where the user ID is retrieved and used as needed.
Setting up redirects in Flask is a simpler process and is essential for creating a seamless user experience and maintaining the integrity of your web application’s URL structure.
Handling Errors in Flask
When it comes to handling errors in Flask, the framework offers a way to define custom error handlers for different types of errors. This is important because it allows you to control what the user sees when something goes wrong, rather than displaying a generic error page.
Flask uses the @app.errorhandler decorator to register a function to handle a specific error. For example, to handle a 404 Not Found error, you can create a function like this:
@app.errorhandler(404) def page_not_found(error): return 'This page does not exist.', 404
This function will be called whenever a 404 error occurs, and it will return the text ‘This page does not exist.’ along with the 404 status code.
Similarly, you can handle other HTTP errors such as 500 Internal Server Error:
@app.errorhandler(500) def internal_server_error(error): return 'An internal server error occurred.', 500
You can also create custom error handlers for your own exceptions. Let’s say you have a custom exception called AuthenticationError. You can handle it as follows:
class AuthenticationError(Exception): pass @app.errorhandler(AuthenticationError) def handle_authentication_error(error): return 'Authentication failed.', 401
With this handler, whenever an AuthenticationError is raised, the function handle_authentication_error()
will be called, and a 401 Unauthorized status code will be returned along with the message.
It is also a good practice to log errors, especially for 500 Internal Server Error, so that you can review server logs for debugging purposes. Here’s an example of how you might log an error:
import logging from flask import Flask app = Flask(__name__) @app.errorhandler(500) def internal_server_error(error): app.logger.error(f'Internal server error: {error}') return 'An unexpected error has occurred.', 500
Error handling is an essential part of Flask application development. Properly handled errors improve the user experience and make it easier for developers to debug issues. By using Flask’s error handling capabilities, you can ensure that your application behaves predictably and reliably, even when things go wrong.
Customizing Error Pages
While Flask’s default error pages are functional, they can be quite bland and don’t offer much in the way of user experience. Thankfully, Flask allows you to customize error pages to match the look and feel of your application. This not only improves the user experience but also gives you a chance to provide helpful information to the user, such as suggestions on what to do next or links to other parts of your site.
To customize error pages, you still use the @app.errorhandler
decorator, but instead of returning a string, you can return a rendered template. Let’s say you have a custom 404 error page template called 404.html. You can serve this template as follows:
@app.errorhandler(404) def page_not_found(error): return render_template('404.html'), 404
Here, render_template
is a Flask function that renders a template file. The '404.html'
is the template file name this is stored in the templates directory of your Flask application. This function will render the custom 404 page whenever a 404 error occurs.
Similarly, for a 500 Internal Server Error, you can have a custom template called 500.html. The error handler would look like this:
@app.errorhandler(500) def internal_server_error(error): return render_template('500.html'), 500
It is also a good idea to pass additional information to the templates. For example, for a 404 error page, you might want to tell the user the URL they tried to access does not exist. You can pass this information to the template using the request
object from Flask:
from flask import request @app.errorhandler(404) def page_not_found(error): url = request.url return render_template('404.html', url=url), 404
In your 404.html template, you can use the passed url
variable to display a message to the user:
<p>Sorry, the page you're looking for <code>{{ url }}</code> does not exist.</p>
Customizing error pages allows you to maintain a consistent brand image across your application, even when errors occur. It is a small detail that can greatly enhance the overall user experience and make your application feel more professional and polished.
Best Practices for Redirects and Error Handling in Flask
When implementing redirects and error handling in Flask, there are several best practices you should follow to ensure your web application remains uncomplicated to manage and maintainable. Here are some key tips:
- When redirecting or handling errors, use the appropriate HTTP status codes to accurately reflect the nature of the response. That is important for SEO and for users who may be interacting with your application programmatically.
- Always use the
url_for()
function to generate URLs for redirects and links in error pages. This makes your code more maintainable and helps prevent broken links if your URL structure changes. - Customize error pages to give users helpful information about what went wrong and what they can do next. A friendly and informative error page can turn a frustrating experience into a positive one.
- Make sure to log errors, especially server errors (HTTP 500), so that you have a record of what went wrong and can debug issues more effectively.
- Regularly test your error handlers to ensure they’re working as expected. Automated tests can help catch issues that might otherwise be overlooked.
- Avoid creating long chains of redirects, as this can slow down user experience and complicate your application’s logic. Instead, aim to redirect users directly to their final destination whenever possible.
- Only use 301 redirects when you are certain a resource has moved permanently. Overuse of 301 redirects can lead to caching issues and confusion if the resource moves again.
Following these best practices will help ensure that your Flask application’s redirects and error handling are both easy to use and robust. Remember, the goal is to provide a seamless experience for your users, even when they encounter errors or when resources move.
Here’s an example of logging an error with a meaningful message and status code:
@app.errorhandler(404) def page_not_found(error): current_app.logger.error(f'Page not found: {request.url}') return render_template('404.html'), 404
By adhering to these best practices, you can create a Flask application that not only handles redirects and errors gracefully but also maintains a high standard of quality and user experience.
Source: https://www.pythonlore.com/implementing-flask-redirect-and-errors/