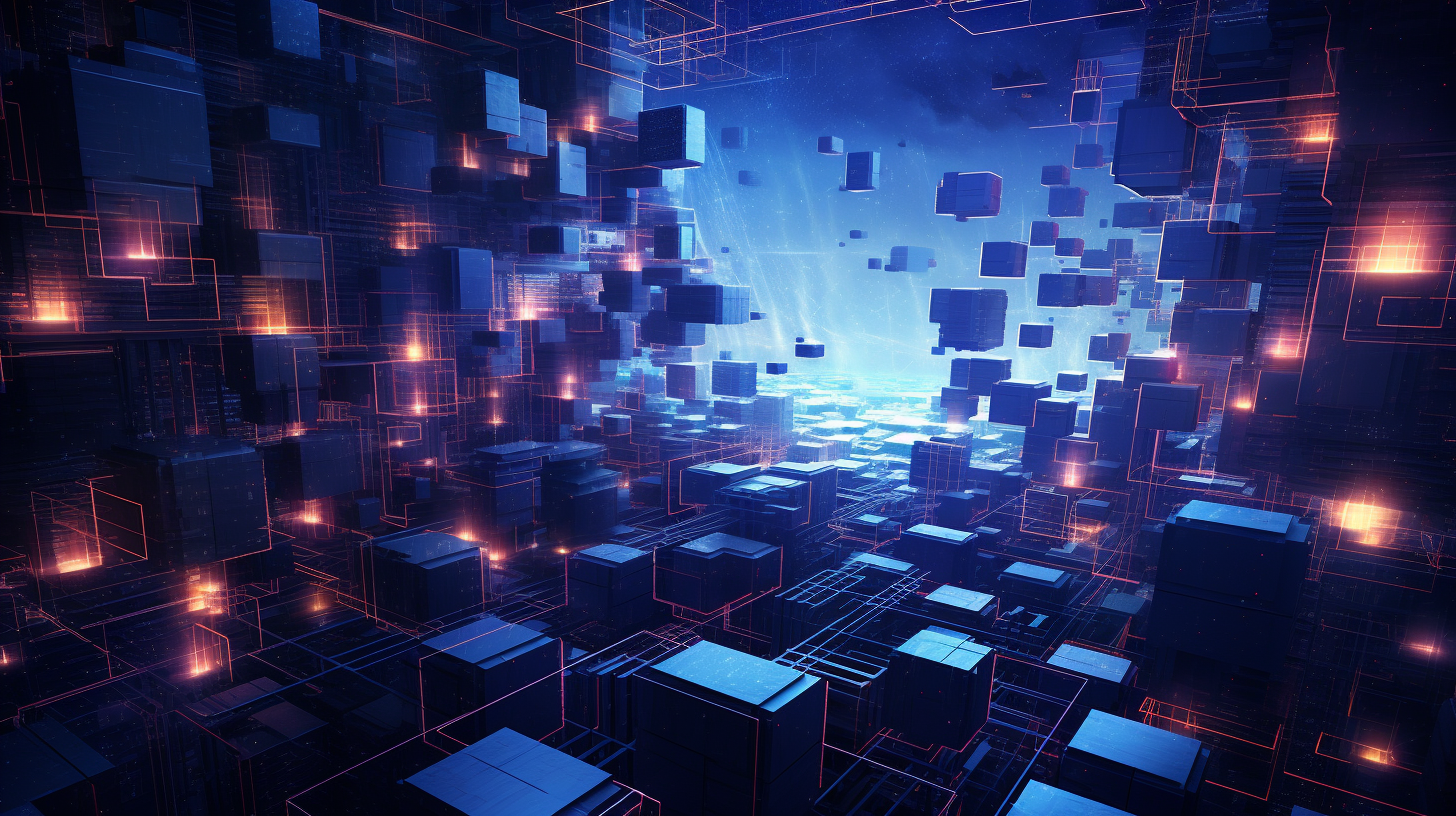
MongoDB’s change streams are a feature that allows applications to access real-time data changes without the complexity and risk of tailing the oplog. Developers can use change streams to subscribe to all data changes on a MongoDB cluster and immediately react to them. This capability is invaluable for applications that require real-time analytics, auditing, replication, and much more.
Change streams provide a simple and efficient way to listen to changes at the collection, database, or cluster level. When a change occurs, MongoDB provides a change event document that contains all the relevant information about the change. This includes the operation type (such as insert, update, delete), the document’s _id field, and, for updates, the updated fields along with their new values.
One of the key benefits of using change streams is that they use MongoDB’s replication capabilities to provide a consistent and ordered stream of changes. This ensures that subscribers receive changes in the order they were applied to the database. Furthermore, change streams are resilient and continue to work seamlessly even in the event of network errors or primary failovers.
Change streams are available in MongoDB versions 3.6 and later, and they leverage the aggregation framework. This allows developers to filter and transform change event streams using the powerful aggregation pipeline stages. As a result, applications can focus on specific changes that are relevant to their use case, thereby reducing the amount of processing and network I/O required.
It’s essential to note that change streams require a replica set or sharded cluster to function. They are not available for standalone MongoDB instances. Using change streams in production environments typically involves careful consideration of read and write concerns, as well as the capacity planning to handle the volume of change events effectively.
With the introduction of change streams, MongoDB continues to enhance its capabilities as a contemporary, general-purpose database that supports the diverse needs of applications in the 21st century.
Setting up Change Streams with Pymongo
Now that we understand the concept of change streams in MongoDB, let’s dive into how we can set them up using Pymongo, the popular Python MongoDB driver. The process is simpler and involves just a few steps. Here’s a step-by-step guide to setting up change streams with Pymongo:
Step 1: Establish a connection to MongoDB
First, you need to establish a connection to your MongoDB cluster. Ensure that you are connecting to a replica set or sharded cluster, as change streams are not supported on standalone instances.
from pymongo import MongoClient # Replace the URI below with your MongoDB deployment's connection string. conn_str = "mongodb://localhost:27017/?replicaSet=myReplicaSet" client = MongoClient(conn_str)
Step 2: Access the Database and Collection
After establishing a connection, access the database and collection you want to watch for changes.
db = client.myDatabase collection = db.myCollection
Step 3: Create a Change Stream
You can create a change stream on a collection, a database, or an entire cluster. Here, we’ll create a change stream that listens to all changes in a specific collection.
change_stream = collection.watch()
Step 4: Iterate Over the Change Stream
Once you have set up the change stream, you can iterate over it to process the change events in real-time.
for change in change_stream: print(change)
Step 5: Filtering Change Events
If you are only interested in specific types of changes, you can use the aggregation pipeline to filter the change events. For example, if you want to listen to only ‘insert’ and ‘update’ operations, you can do so by passing a pipeline to the watch
method.
pipeline = [ {'$match': {'operationType': {'$in': ['insert', 'update']}}} ] change_stream = collection.watch(pipeline)
With these steps, you have successfully set up a change stream using Pymongo. You can now start processing the change events and integrate them into your application logic.
Remember, it is essential to handle exceptions and errors when working with change streams. We’ll cover error handling in more detail in a later subsection.
Handling Change Events in Real-Time
As you begin to handle change events in real-time, it’s important to understand the structure of the change event document that MongoDB provides. This document includes fields such as operationType, fullDocument, and documentKey, which give you the necessary information to understand and react to the change.
for change in change_stream: if change['operationType'] == 'insert': document = change['fullDocument'] handle_insert(document) elif change['operationType'] == 'update': document_key = change['documentKey'] updated_fields = change['updateDescription']['updatedFields'] handle_update(document_key, updated_fields) elif change['operationType'] == 'delete': document_key = change['documentKey'] handle_delete(document_key)
When processing change events, it’s crucial to implement the logic that will be triggered by each type of operation. For example, when handling an insert operation, you may want to perform additional computations or update other systems with the new data. The following Python function demonstrates how you might handle an insert event:
def handle_insert(document): print(f"New document inserted: {document}") # Additional processing logic here
Similarly, when an update occurs, you’ll receive information about which fields were modified. This allows you to react only to the relevant changes, as seen in this example:
def handle_update(document_key, updated_fields): print(f"Document with key {document_key} updated with fields: {updated_fields}") # Additional processing logic here
For delete operations, you may want to perform cleanup tasks or log the deletion. See this simple example:
def handle_delete(document_key): print(f"Document with key {document_key} was deleted") # Additional processing logic here
When handling change events in real-time, it is also important to consider the performance implications. You may want to batch process events or use asynchronous processing to ensure that your application can handle the volume of change events efficiently.
Another important aspect is handling potential duplicates. Change streams provide at-least-once delivery, which means that in certain scenarios, such as a network error or a primary failover, you might receive the same change event more than once. Therefore, your application logic should be idempotent to handle these cases gracefully.
Handling change events in real-time involves setting up a change stream, understanding the structure of the change event document, and implementing the logic to react to different types of operations. By doing so, you can leverage the power of MongoDB’s change streams to build responsive and dynamic applications.
Implementing Change Stream Error Handling
Implementing robust error handling is an essential part of working with change streams in MongoDB using Pymongo. This ensures that your application can recover gracefully from potential issues and continue processing change events without interruption. The following are some key considerations and strategies for implementing effective change stream error handling:
Network Errors and Disconnections
Network issues are inevitable in distributed systems, and your application should be prepared to handle them. If a network error occurs while listening to a change stream, the Pymongo driver will attempt to automatically resume the stream. However, it is crucial to catch any exceptions that may be raised during this process:
try: for change in change_stream: print(change) except pymongo.errors.PyMongoError as e: print(f"An error occurred: {e}") # Logic to handle the error or reconnect to the change stream
Change Stream Resumability
MongoDB change streams support resumability, which allows them to pick up where they left off in the event of a transient failure. The change stream provides a resume token that can be used to restart the stream from a specific point. It is essential to store this token and use it when restarting the stream:
resume_token = None try: for change in change_stream: print(change) resume_token = change['_id'] except pymongo.errors.PyMongoError: if resume_token: change_stream = collection.watch(resume_after=resume_token)
Handling Primary Failovers
In a replica set, primary failovers can occur, resulting in temporary unavailability of the change stream. Your application should be resilient enough to handle these events. The Pymongo driver provides built-in mechanisms to automatically recover from primary failovers, but you should still account for potential exceptions:
try: for change in change_stream: # Process change events except pymongo.errors.ConnectionFailure as e: print(f"Connection failed: {e}") # Reconnect logic here
Ensuring Idempotent Operations
Since change streams guarantee at-least-once delivery, your application may receive duplicate change events. It’s crucial to design your event handling logic to be idempotent, meaning that applying the same event multiple times will not result in inconsistencies. This can be achieved by checking if the change has already been processed before taking action:
processed_changes = set() for change in change_stream: change_id = change['_id'] if change_id not in processed_changes: # Process the change event processed_changes.add(change_id) else: print(f"Skipping duplicate change event with ID: {change_id}")
Monitoring and Alerting
It is also important to implement monitoring and alerting mechanisms to detect and respond to errors in real-time. This can include logging errors, sending notifications, or triggering automated recovery processes.
By considering these error handling strategies, you can build a resilient application that effectively utilizes change streams in MongoDB with Pymongo. This will help ensure continuous real-time data processing, even in the face of transient failures or disruptions.
Best Practices for Using Change Streams in MongoDB
When using change streams in MongoDB with Pymongo, there are several best practices that can help you ensure efficient and reliable real-time data processing. Here are some key considerations:
- Filtering change events with an aggregation pipeline can significantly reduce the volume of events your application has to process. However, be cautious about using complex filters that might impact performance. Opt for simple filters that target specific operation types or fields.
- Change streams can generate a large number of events, especially in busy databases. It’s important to monitor the resource usage of your application and the MongoDB server to prevent bottlenecks. Ponder using batch processing or scaling your application to handle high volumes of change events.
- If the schema of your documents changes, it can affect how you process change events. Make sure to update your change event handling logic to accommodate any schema changes and ensure that your application continues to function correctly.
- When shutting down your application, ensure that you close the change stream cursor properly. This helps prevent resource leaks and ensures that the application can be restarted without issues.
- Change streams can expose sensitive data through the change events. Always implement appropriate security measures, such as encryption in transit and at rest, and restrict access to the change streams to authorized users only.
- Regularly monitor the health of your change streams. This includes checking for errors, lag, or interruptions in the event stream. Implement alerting mechanisms to notify you of any issues that may arise.
By following these best practices, you can make the most out of change streams in MongoDB and build robust applications that react to data changes in real-time. Additionally, always stay informed about updates to MongoDB and Pymongo, as new features and improvements can further enhance your use of change streams.
For example, it’s good practice to implement a mechanism to restart the change stream in case of an unexpected shutdown. Here’s a simple example of how to resume a change stream using the last received resume token:
resume_token = None try: for change in change_stream: # Process change events here resume_token = change['_id'] except Exception as e: # Handle any exceptions that occurred print(f"An error occurred: {e}") finally: # On shutdown, resume the change stream using the last resume token if resume_token: change_stream = collection.watch(resume_after=resume_token)
Adhering to these best practices will help ensure that your application effectively leverages the power of change streams in MongoDB, leading to more responsive and resilient data processing flows.
Source: https://www.pythonlore.com/using-change-streams-in-mongodb-with-pymongo/