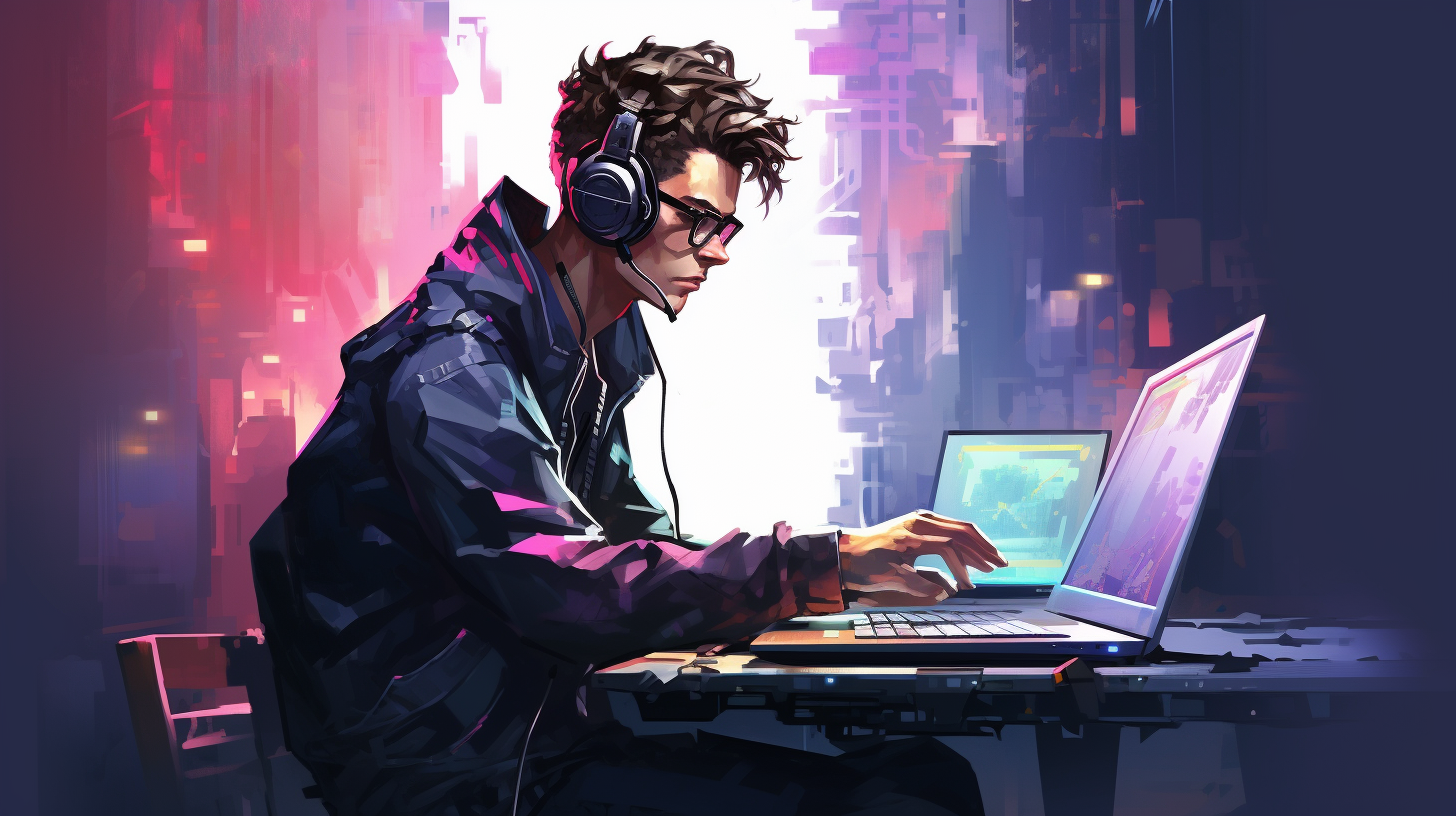
HTTP verbs are an important component of HTTP requests that define the action to be performed on the specified resource. They are sometimes referred to as “methods”. Each verb has a specific meaning and use case, and it is essential to understand them to utilize the Python Requests library effectively.
The most commonly used HTTP verbs are GET, POST, PUT, PATCH, and DELETE.
- Used to retrieve data from a specified resource. GET requests should only retrieve data and have no other effect.
- Used to send data to a server to create/update a resource. The data sent to the server is stored in the request body of the HTTP request.
- Used to send data to a server to create or replace a resource. It’s similar to POST, but PUT requests are idempotent, meaning that multiple identical PUT requests should have the same effect as a single request.
- Used to apply partial modifications to a resource. It is similar to PUT but only applies a partial update rather than a complete replacement.
- Used to delete a specified resource.
Each of these verbs corresponds to a typical CRUD (Create, Read, Update, Delete) operation in database management. It is important to note that not all servers support all verbs, and sometimes additional configuration is required to handle them properly.
When working with the Python Requests library, these HTTP verbs are represented by methods such as requests.get()
for GET requests, requests.post()
for POST requests, and so on. Understanding these verbs and their characteristics allows you to make the appropriate request based on the action you want to perform.
It’s always important to consider the idempotent nature of the HTTP verbs. For example, making multiple GET requests should not change the state of the resource, whereas making multiple POST requests might create multiple resources or change the state multiple times.
Here’s an example of using the GET method with Python Requests:
import requests response = requests.get('https://api.example.com/data') print(response.text)
This simple code snippet sends a GET request to the specified URL and prints out the response data. As you delve into more advanced usage of Python Requests with HTTP verbs, you’ll see how to add headers, parameters, and body data to your requests, as well as handle different types of responses and status codes.
Making GET Requests
When making GET requests with Python Requests, you can pass in additional parameters to customize your request. These parameters can be used to send data to the server, such as filtering information or pagination data. To add parameters to your GET request, you can use the params argument in the requests.get()
function. Here’s an example:
params = {'key1': 'value1', 'key2': 'value2'} response = requests.get('https://api.example.com/data', params=params) print(response.url) # https://api.example.com/data?key1=value1&key2=value2 print(response.text)
This will append the parameters to the URL as a query string. The Requests library takes care of encoding the parameters, so you don’t have to worry about URL encoding.
Headers can also be important when making GET requests. Some APIs require authentication tokens or other information to be passed in the header of the request. You can add custom headers to your request using the headers argument. Here’s an example:
headers = {'Authorization': 'Bearer YOUR_ACCESS_TOKEN'} response = requests.get('https://api.example.com/protected_data', headers=headers) print(response.text)
When working with APIs that return JSON data, Python Requests can automatically decode the JSON response content for you. Instead of using response.text
, you can use response.json()
to get a parsed JSON object:
response = requests.get('https://api.example.com/data') data = response.json() print(data) # Prints the JSON object
Handling response status codes is also an important aspect of making GET requests. The status code indicates the result of the HTTP request. For example, a 200 OK status code means the request was successful, while a 404 Not Found means the resource you are trying to access does not exist. You can check the status code of the response like so:
response = requests.get('https://api.example.com/data') if response.status_code == 200: print('Success!') elif response.status_code == 404: print('Not Found.')
Using the advanced features of the Python Requests library with GET requests allows you to craft precise and efficient HTTP requests to interact with APIs and other web services.
Making POST Requests
When it comes to making POST requests using the Python Requests library, the process is similar to making GET requests, but with a few key differences. The most notable difference is that POST requests often include data that needs to be sent to the server in the request body. This data can be in various formats, such as form data, JSON, or XML.
Here’s an example of how to send form data with a POST request:
import requests form_data = {'username': 'user', 'password': 'pass'} response = requests.post('https://api.example.com/login', data=form_data) print(response.text)
In this example, the data
parameter is used to send the form data to the server. The Requests library automatically encodes the data as form-encoded before sending it.
If you need to send JSON data, you can use the json
parameter instead:
import requests json_data = {'key': 'value'} response = requests.post('https://api.example.com/resource', json=json_data) print(response.text)
By using the json
parameter, the Requests library will automatically serialize your dictionary to a JSON formatted string and also set the appropriate content-type header for you.
Just like with GET requests, you can also include custom headers in your POST request:
import requests headers = {'Authorization': 'Bearer YOUR_ACCESS_TOKEN'} json_data = {'key': 'value'} response = requests.post('https://api.example.com/protected_resource', headers=headers, json=json_data) print(response.text)
Handling response status codes is also crucial when making POST requests. A successful POST request typically returns a 201 Created status code, indicating that the resource was successfully created or updated. However, it is always a good idea to check the status code to handle different scenarios:
response = requests.post('https://api.example.com/resource', json=json_data) if response.status_code == 201: print('Resource created successfully!') else: print(f'Error: {response.status_code}')
Using the advanced features of the Python Requests library with POST requests allows you to send complex data to the server and handle various types of responses. That’s particularly useful when working with web APIs that rely on POST requests for creating or updating resources.
Making PUT and PATCH Requests
When it comes to making PUT and PATCH requests with the Python Requests library, the concepts are similar to those used in POST requests, with PUT being used to update or create an entire resource and PATCH for making partial updates. Both of these HTTP verbs require data to be sent in the request body, just like with POST requests.
Here’s an example of how to make a PUT request to update an existing resource:
import requests update_data = {'name': 'New Name', 'description': 'Updated description'} response = requests.put('https://api.example.com/resource/1', data=update_data) print(response.text)
In the above example, we’re sending a dictionary with the updated fields for the resource with ID 1. The Requests library will encode this data as form-encoded before sending it. If you want to send JSON data, you can use the json
parameter, just like in the POST request example:
import requests update_data = {'name': 'New Name', 'description': 'Updated description'} response = requests.put('https://api.example.com/resource/1', json=update_data) print(response.text)
For PATCH requests, which are used for partial updates, you only need to include the fields you want to update:
import requests partial_update = {'description': 'Partially updated description'} response = requests.patch('https://api.example.com/resource/1', json=partial_update) print(response.text)
As with the other HTTP verbs, it’s important to handle the response status codes to determine if the request was successful:
response = requests.put('https://api.example.com/resource/1', json=update_data) if response.status_code == 200: print('Resource updated successfully!') elif response.status_code == 404: print('Resource not found.') else: print(f'Error: {response.status_code}')
Using PUT and PATCH requests with the Python Requests library allows you to update resources efficiently. Whether you’re updating a whole resource or just a part of it, these HTTP verbs are essential for maintaining up-to-date and accurate data on the server.
Making DELETE Requests
When it comes to using the DELETE HTTP verb with the Python Requests library, it’s relatively straightforward. A DELETE request is used when you want to remove a resource from the server. It’s a powerful action that should be used cautiously, as it can lead to permanent deletion of data.
Here is a simple example of how to perform a DELETE request:
import requests response = requests.delete('https://api.example.com/resource/1') print(response.text)
In the above example, we’re sending a DELETE request to the URL of the resource we want to remove, in this case, the resource with ID 1. The Python Requests library doesn’t require any additional parameters for a DELETE request, rendering it effortless to use.
As always, it’s important to handle the response status codes to determine if the DELETE request was successful:
response = requests.delete('https://api.example.com/resource/1') if response.status_code == 200: print('Resource deleted successfully!') elif response.status_code == 404: print('Resource not found.') else: print(f'Error: {response.status_code}')
A 200 OK status code indicates that the deletion was successful, while a 404 Not Found means that the resource you’re trying to delete does not exist. Other status codes may indicate different issues that need to be handled accordingly.
Using the DELETE method with the Python Requests library is an effective way to remove resources from a server. However, due to the permanent nature of deletion, it’s advised to implement confirmation mechanisms in your application to prevent accidental deletion of important data.
Source: https://www.pythonlore.com/advanced-usage-of-python-requests-with-http-verbs/