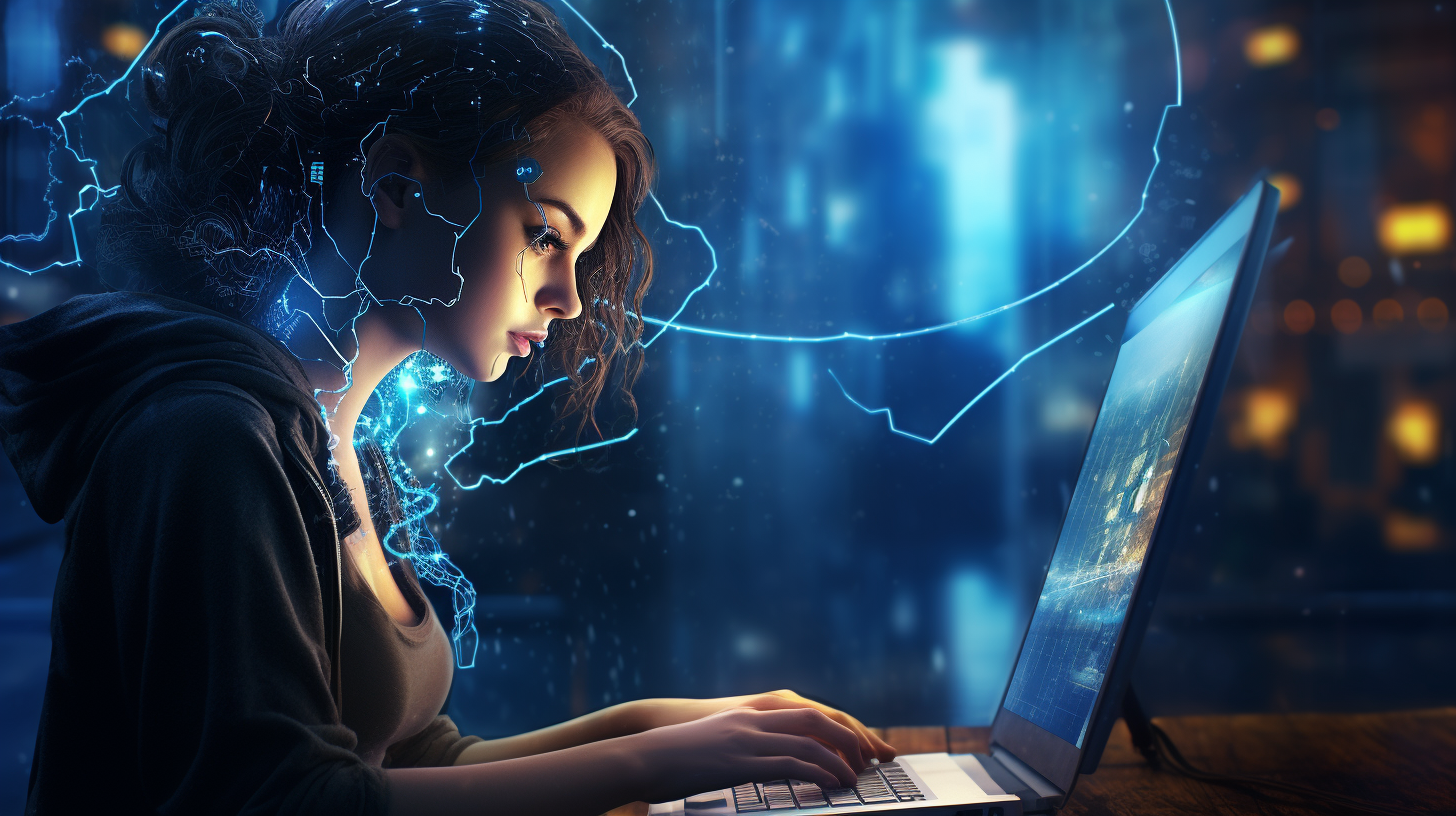
Overview of Swift Package Manager
The Swift Package Manager is a tool for managing the distribution of Swift code. It automates the process of downloading, compiling, and linking dependencies for Swift projects. Introduced in Swift 3.0, the Swift Package Manager is integrated with the Swift build system to automate the process of downloading, compiling, and linking dependencies.
Key Features of Swift Package Manager:
- Support for both executable and library Swift packages
- Dependency resolution based on semantic versioning
- Support for declaring package dependencies from a variety of sources, including Git repositories
- Support for compiling Swift, C, C++, and Objective-C code
- Automatic generation of Xcode project files
Using the Swift Package Manager is straightforward. To initialize a new package, you can use the swift package init
command. Here’s an example:
swift package init --type executable
This will create a new directory with the necessary files to start a Swift package, including a Package.swift
manifest file which is used to define the package and its dependencies.
The
Package.swift
manifest file is at the heart of the Swift Package Manager. It defines the package’s name, its contents, and its dependencies on other packages.
Adding dependencies to a Swift package is done by specifying them in the dependencies
array of the Package.swift
file. For example:
dependencies: [ .package(url: "https://github.com/apple/example-package-playingcard.git", from: "3.0.0") ]
This tells the Swift Package Manager to download and integrate the specified package into your project, starting from version 3.0.0.
The Swift Package Manager also includes a command line tool, swift build
, which can be used to build a Swift package. It compiles the source files, links the resulting binaries, and produces an executable or a library.
swift build
The Swift Package Manager provides a standardized method for managing Swift code dependencies, making it easier for developers to share and reuse code across different projects.
Creating a Package
Creating a package with Swift Package Manager is an essential skill for Swift developers. It begins with setting up the package structure, which can be done with a simple command. Here’s how you can create a new Swift package:
swift package init --type library
This command creates a new directory containing the structure for a library package. If you’re creating an executable package, you would replace --type library
with --type executable
. The directory will include a Package.swift
file, which especially important for defining the package, as well as other directories like Sources
and Tests
.
The Package.swift
file uses the Swift Package Description API and defines the name of the package, its products, and any dependencies it might have. Here is an example of what the contents of a Package.swift
file might look like:
// swift-tools-version:5.3 import PackageDescription let package = Package( name: "MyLibrary", products: [ // Products define the executables and libraries a package produces, and make them visible to other packages. .library( name: "MyLibrary", targets: ["MyLibrary"]), ], dependencies: [ // Dependencies declare other packages that this package depends on. // .package(url: /* package url */, from: "1.0.0"), ], targets: [ // Targets are the basic building blocks of a package. A target can define a module or a test suite. // Targets can depend on other targets in this package, and on products in packages this package depends on. .target( name: "MyLibrary", dependencies: []), .testTarget( name: "MyLibraryTests", dependencies: ["MyLibrary"]), ] )
Within the targets
section, you define the modules of your package. A target can be either a library or an executable, and it contains the source files and resources for that module. If your package has dependencies, you can declare them in the dependencies
array, specifying the URL to the Git repository and the version of the package you want to depend on.
Once you have set up your package, you can begin adding source files to the Sources
directory. Each target you define in your Package.swift
should correspond to a directory inside Sources
with the same name. For example, if you have a target named “MyLibrary”, you should have a directory called Sources/MyLibrary
with your Swift files inside it.
After adding your source files, you can build your package using:
swift build
This will compile the source files and produce a binary if your package is an executable or a library that can be imported by other Swift packages or applications.
Creating a Swift package is just the beginning. Once your package is set up, you can start adding dependencies, managing versions, and using advanced features to make the most of the Swift Package Manager.
Managing Dependencies
When it comes to managing dependencies in Swift Package Manager, the process is centralized through the use of the Package.swift
manifest file. Each dependency is represented as a package which can be added to the list of dependencies for your project. Managing dependencies involves adding, updating, and removing them as needed.
To add a dependency, you need to specify the location of the package and the version constraints. The Swift Package Manager uses semantic versioning, which helps with compatibility and dependency resolution. Here’s an example of adding a dependency in your Package.swift
file:
dependencies: [ .package(url: "https://github.com/apple/example-package-deckofplayingcards.git", from: "1.0.0") ]
This line of code tells the Swift Package Manager to fetch the package from the given URL and to use versions starting from 1.0.0.
Updating a dependency is just as simple. If you want to update to a newer version, you can change the version constraint in the Package.swift
file and run swift package update
in the terminal. This will prompt Swift Package Manager to resolve the new set of dependencies.
Removing a dependency is done by simply removing the corresponding line from the dependencies array in the Package.swift
file and then running swift package resolve
to update the dependency graph.
It’s also possible to specify a range of versions for a dependency:
dependencies: [ .package(url: "https://github.com/apple/example-package-deckofplayingcards.git", "1.0.0"..<"2.0.0") ]
This indicates that any version greater than or equal to 1.0.0 and less than 2.0.0 is acceptable.
For dependencies that are still in active development, you can specify a branch or a specific commit instead of a version number:
dependencies: [ .package(url: "https://github.com/apple/example-package-deckofplayingcards.git", .branch("main")), .package(url: "https://github.com/apple/example-package-deckofplayingcards.git", .revision("1a2b3c4d")) ]
Using the .branch("main")
specifier will pull the latest commit on the main branch, while .revision("1a2b3c4d")
will pull a specific commit, ensuring that everyone working on the project has the exact same code.
Dependency management is a critical part of any project, and Swift Package Manager provides a robust set of tools to handle it efficiently. By specifying dependencies in the Package.swift
file, you can ensure that your project has everything it needs to build successfully and that your dependencies are kept up-to-date.
Resolving Versioning Issues
When working with Swift Package Manager, one of the challenges you may encounter is resolving versioning issues with dependencies. Versioning issues can occur when two or more dependencies require different versions of the same package, or when there are incompatible versions specified in your Package.swift
manifest file. To resolve these issues, Swift Package Manager provides several strategies.
Pin Dependencies
You can pin a dependency to a specific version by updating the Package.swift
file with the exact version you need. This ensures that Swift Package Manager always uses that version, avoiding conflicts with other dependencies.
dependencies: [ .package(url: "https://github.com/apple/example-package-deckofplayingcards.git", .exact("2.0.0")) ]
Branch and Commit-Based Dependencies
If a specific branch or commit of a dependency is needed, perhaps to access a bug fix or new feature not yet released, you can specify it directly in the dependencies array:
dependencies: [ .package(url: "https://github.com/apple/example-package-deckofplayingcards.git", .branch("bugfix-branch")), .package(url: "https://github.com/apple/example-package-deckofplayingcards.git", .revision("1a2b3c4d")) ]
Resolving Dependency Conflicts
When there is a conflict between dependency versions, Swift Package Manager will try to find a compatible version that satisfies all requirements. If it can’t, you may need to adjust the version constraints in your Package.swift
file to find a compatible version. This may involve using a more permissive version range or updating other dependencies to compatible versions.
For example, if two dependencies require different major versions of the same package, you may need to update one of your dependencies to a version that supports both major versions, or choose an alternative package that fits all requirements.
Using the resolve
Command
The swift package resolve
command can be used to automatically resolve version conflicts. This command updates the Package.resolved
file, which locks the resolved dependency graph. If you encounter a versioning issue, running this command may help Swift Package Manager find a compatible set of dependencies.
swift package resolve
By carefully managing your dependencies and using the tools provided by Swift Package Manager, you can resolve versioning issues and maintain a stable and compatible dependency graph for your Swift projects.
Advanced Features and Best Practices
When working with Swift Package Manager, there are several advanced features and best practices that can help streamline the development process and ensure your packages are robust and maintainable.
Conditional Dependencies
Sometimes, you may want to include dependencies only for certain platforms or configurations. Swift Package Manager allows you to define platform-specific dependencies using conditional statements within your Package.swift
file. For example:
targets: [ .target( name: "MyLibrary", dependencies: [ .product(name: "DependencyA", package: "DependencyAPackage"), .target(name: "MyLibraryHelpers"), ], condition: .when(platforms: [.iOS]) ) ]
This will ensure that DependencyA
is only included when the package is built for iOS platforms.
Package Products
Defining products in your package is essential for making libraries or executables available to other packages or applications. When you define a product, you specify whether it is a library or an executable, and which targets it includes. Good practice is to provide a descriptive name for your products to make it clear what functionality they offer. For example:
products: [ .library( name: "Networking", targets: ["Networking"]), .executable( name: "NetworkTool", targets: ["NetworkToolExecutable"]) ]
Versioning and Tagging
Proper versioning especially important for dependency management. Always use semantic versioning (major.minor.patch) and tag your releases accordingly in your version control system. This makes it easier for Swift Package Manager to resolve dependencies and ensures that consumers of your package can rely on versioned releases. To tag a release in Git, you can use:
git tag 1.0.0 git push --tags
Package Documentation
Documentation is an essential part of any package. Swift Package Manager supports generating documentation from comments using jazzy
or similar tools. Documenting your public interfaces will help other developers understand how to use your package and what to expect from it.
Testing Best Practices
Always include tests with your package to ensure its functionality is working as expected. Swift Package Manager makes it easy to define test targets:
targets: [ .testTarget( name: "MyLibraryTests", dependencies: ["MyLibrary"]), ]
Run your test suite with the swift test
command regularly to catch any regressions or issues early on.
By using these advanced features and following best practices, you can create high-quality Swift packages that are easy to maintain and integrate into other projects.
Source: https://www.plcourses.com/swift-package-manager/