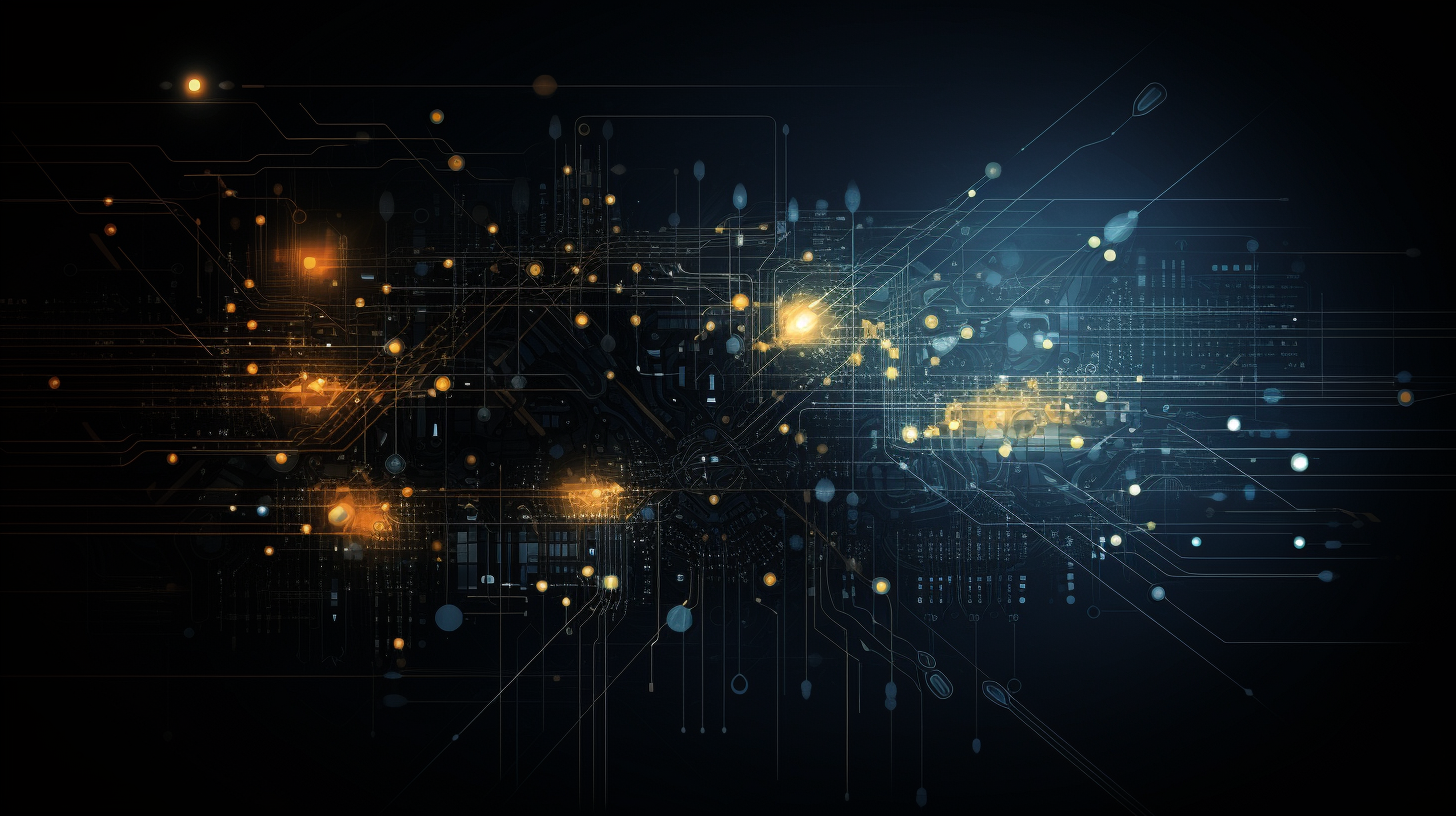
Introduction to Unit Testing
Unit testing is a method of testing individual units or components of a software application to ensure they’re functioning correctly. In the context of PHP, a unit could be a single function, method, or class. The primary goal of unit testing is to validate that each unit of the software performs as expected.
Unit tests are typically automated and written by developers as they write their code. These tests are then executed frequently throughout the development process to ensure that new changes do not break existing functionality. This approach to testing is known as test-driven development (TDD), where tests are written before the actual code is implemented.
Unit testing offers several benefits, including:
- By testing units in isolation, issues can be identified and resolved early in the development process.
- Writing tests encourages developers to write cleaner, more modular code.
- With a suite of passing tests, developers can refactor code with the assurance that they haven’t introduced new bugs.
- Well-written unit tests can serve as documentation for how a particular unit of code is intended to behave.
To create a unit test in PHP, developers use assertions to check the output of a unit against an expected result. For example:
function add($a, $b) { return $a + $b; } // Test case public function testAddFunction() { $result = add(1, 2); $this->assertEquals(3, $result); }
In the example above, the testAddFunction
is a unit test for the add
function. The test uses an assertion method, assertEquals
, provided by a unit testing framework to compare the expected result, 3
, with the actual result returned by the add
function.
It’s important to note that unit tests should be isolated, meaning they should not rely on external systems such as databases or APIs. This isolation ensures that tests run quickly and consistently, making them more reliable and easier to maintain.
In the next section, we will discuss how to set up PHPUnit, a popular unit testing framework for PHP, to start writing and running your own unit tests.
Setting Up PHPUnit in PHP
PHPUnit is a widely-used unit testing framework for PHP that provides a rich set of assertions and testing tools to help you write and execute your tests. Setting up PHPUnit is a straightforward process that can be done using Composer, PHP’s dependency manager.
To begin, you’ll need to have Composer installed on your system. If you do not have Composer installed, you can download it from getcomposer.org. Once Composer is ready, setting up PHPUnit is as simple as requiring it in your project.
Open your terminal or command line interface and navigate to the root directory of your PHP project. Run the following command to install PHPUnit:
composer require --dev phpunit/phpunit ^9
This command tells Composer to add PHPUnit as a development dependency in your project, specifically the version 9 series. Once the installation is complete, you will have a vendor
directory in your project, which includes the PHPUnit library and an autoload file to include in your test scripts.
Next, you’ll want to set up a basic configuration file for PHPUnit, named phpunit.xml
. This file will define some default settings for your tests, such as bootstrap files, test directories, and more. Here’s an example of a simple phpunit.xml
configuration:
<?xml version="1.0" encoding="UTF-8"?> <phpunit bootstrap="vendor/autoload.php" colors="true" verbose="true"> <testsuites> <testsuite name="My Test Suite"> <directory>./tests</directory> </testsuite> </testsuites> </phpunit>
In this configuration, we’re specifying the bootstrap
file, which is the Composer autoload file. We’re also defining a testsuite
with the name “My Test Suite” that will include all the test files located in the ./tests
directory of our project.
With PHPUnit installed and configured, you’re now ready to write your first unit test, which we will cover in the next section of this article.
Writing Unit Tests with PHPUnit
Writing unit tests with PHPUnit involves creating test cases that will verify the behavior of your PHP code. Each test case should focus on a single aspect of the code to ensure that it works as expected. In PHPUnit, test cases are represented as classes that extend the PHPUnitFrameworkTestCase
class.
To create a unit test, start by creating a new PHP file in your tests directory. The name of the file should reflect the class or feature you are testing, and it should end with Test.php. For example, if you’re testing a Calculator
class, you might create a file named CalculatorTest.php.
Here’s an example of a simple test case for our Calculator
class:
use PHPUnitFrameworkTestCase; class CalculatorTest extends TestCase { public function testAdd() { $calculator = new Calculator(); $result = $calculator->add(2, 3); $this->assertEquals(5, $result); } }
In the example above, we have a test method testAdd()
which creates an instance of the Calculator
class and calls its add
method. The assertEquals
assertion checks if the result is equal to the expected value of 5.
It’s important to follow some naming conventions when writing PHPUnit tests. Test methods should start with the word test followed by a description of what the test is checking. If you prefer, you can also use the @test
annotation in the method’s docblock to indicate that it’s a test method.
PHPUnit also provides a variety of assertion methods to test different conditions. Some of the most commonly used assertions include:
- assertTrue – Checks that a condition is true.
- assertFalse – Checks that a condition is false.
- assertNull – Checks that a variable is null.
- assertNotNull – Checks that a variable is not null.
- assertCount – Checks the number of elements in an array or countable object.
- assertSame – Checks if two variables refer to the same object or value.
- assertNotSame – Checks if two variables do not refer to the same object or value.
Here’s an example of a test case using different assertion methods:
public function testIsEmpty() { $stack = []; $this->assertEmpty($stack); array_push($stack, 'foo'); $this->assertNotEmpty($stack); $this->assertSame('foo', $stack[0]); }
In this example, we’re testing if an array is empty, then not empty after adding an element, and finally, if the first element is the string ‘foo’.
Remember to keep your tests isolated and avoid dependencies on external systems. If your code interacts with a database or external service, consider using mock objects or stubs to simulate those interactions.
With these guidelines in mind, you can start writing your own unit tests with PHPUnit to ensure your PHP code is robust and reliable.
Running and Analyzing Unit Tests
Once you have written your unit tests, it’s time to run them and analyze the results. Running your tests is simple with PHPUnit. Open your terminal or command line interface and navigate to the root directory of your PHP project. To run all the tests in your test suite, execute the following command:
vendor/bin/phpunit
PHPUnit will search for the phpunit.xml configuration file and execute all the tests defined in the specified test suite. If you want to run a specific test file, you can provide the path to the test file as an argument:
vendor/bin/phpunit tests/CalculatorTest.php
As the tests run, PHPUnit will provide real-time feedback on the console, indicating which tests have passed or failed. A test is considered passed if all assertions within the test method return true. A test fails if any assertion fails or an uncaught exception is thrown.
After all tests have been executed, PHPUnit will display a summary of the test results, including the number of tests run, assertions made, and any failures or errors encountered. An example output might look like this:
PHPUnit 9.5.0 by Sebastian Bergmann and contributors. ..... 5 / 5 (100%) Time: 00:00.012, Memory: 6.00 MB OK (5 tests, 10 assertions)
In the example above, 5 tests were run with 10 assertions, and all tests passed successfully. If there are any failures, PHPUnit will provide detailed information about the failed test, including the file name, line number, and a message describing the failure. This information very important for diagnosing and fixing the problem.
For example, if a test fails due to an assertion error, you may see output like this:
There was 1 failure: 1) CalculatorTest::testAdd Failed asserting that 4 matches expected 5. /tests/CalculatorTest.php:10
This output indicates that the testAdd method in CalculatorTest failed because the expected result was 5, but the actual result was 4. With this information, you can quickly locate the issue in your code and make the necessary corrections.
It’s also possible to generate code coverage reports with PHPUnit, which provide insights into which parts of your codebase are being tested and which are not. To generate a code coverage report, you need to have Xdebug or pcov installed and enabled in your PHP configuration. Then, you can run the following command:
vendor/bin/phpunit --coverage-text
The coverage report will show the percentage of code covered by your tests, broken down by file and method. This information is valuable for identifying untested areas of your code and prioritizing additional tests to write.
Running and analyzing unit tests with PHPUnit is a straightforward process that provides immediate feedback on the health of your code. By paying attention to the test results and code coverage reports, you can maintain a high-quality codebase and catch issues early in the development cycle.
Best Practices for Unit Testing in PHP
Adopting best practices for unit testing in PHP can significantly improve the effectiveness and maintainability of your test suite. Here are some key practices to consider when writing unit tests:
- The name of your test should clearly describe what the test is trying to verify. This makes it easier for other developers to understand the purpose of the test and what aspect of the code it covers.
- Each test should be independent of others. Tests should not share state or rely on the order in which they are run. This isolation helps ensure that tests are reliable and can be run in any order.
- A unit test should focus on a single behavior or aspect of the code. This makes it easier to diagnose issues when a test fails, as there’s only one potential cause.
- When testing code that depends on external services or systems, use mock objects or stubs to simulate those dependencies. This helps keep your tests fast and focused on the code you’re actually testing.
- Start your test by stating the expected outcome using assertions, then perform the actions that lead to that outcome. This pattern, known as Arrange-Act-Assert, helps keep your tests organized and clear.
- In addition to testing the common usage of your code, be sure to test edge cases and error conditions. This helps ensure your code is robust and handles unexpected inputs gracefully.
- Slow tests can become a bottleneck in the development process. Aim to keep your tests as fast as possible by avoiding unnecessary I/O operations and keeping test data minimal.
Here’s an example of a well-structured unit test in PHP that follows these best practices:
use PHPUnitFrameworkTestCase; class StringFormatterTest extends TestCase { public function testConcatenateWithSpaceShouldJoinStrings() { $formatter = new StringFormatter(); $result = $formatter->concatenateWithSpace('Hello', 'World'); $this->assertEquals('Hello World', $result); } public function testConcatenateWithSpaceShouldHandleEmptyStrings() { $formatter = new StringFormatter(); $result = $formatter->concatenateWithSpace('', 'World'); $this->assertEquals(' World', $result); } }
In the example above, we have two test methods with descriptive names that explain what they’re testing. The tests are isolated, testing one behavior each, and follow the Arrange-Act-Assert pattern. They also handle an edge case by testing how the method behaves with an empty string as input.
By adhering to these best practices, your unit tests will be more effective, easier to understand, and simpler to maintain as your codebase evolves.
Source: https://www.plcourses.com/php-and-unit-testing-phpunit/