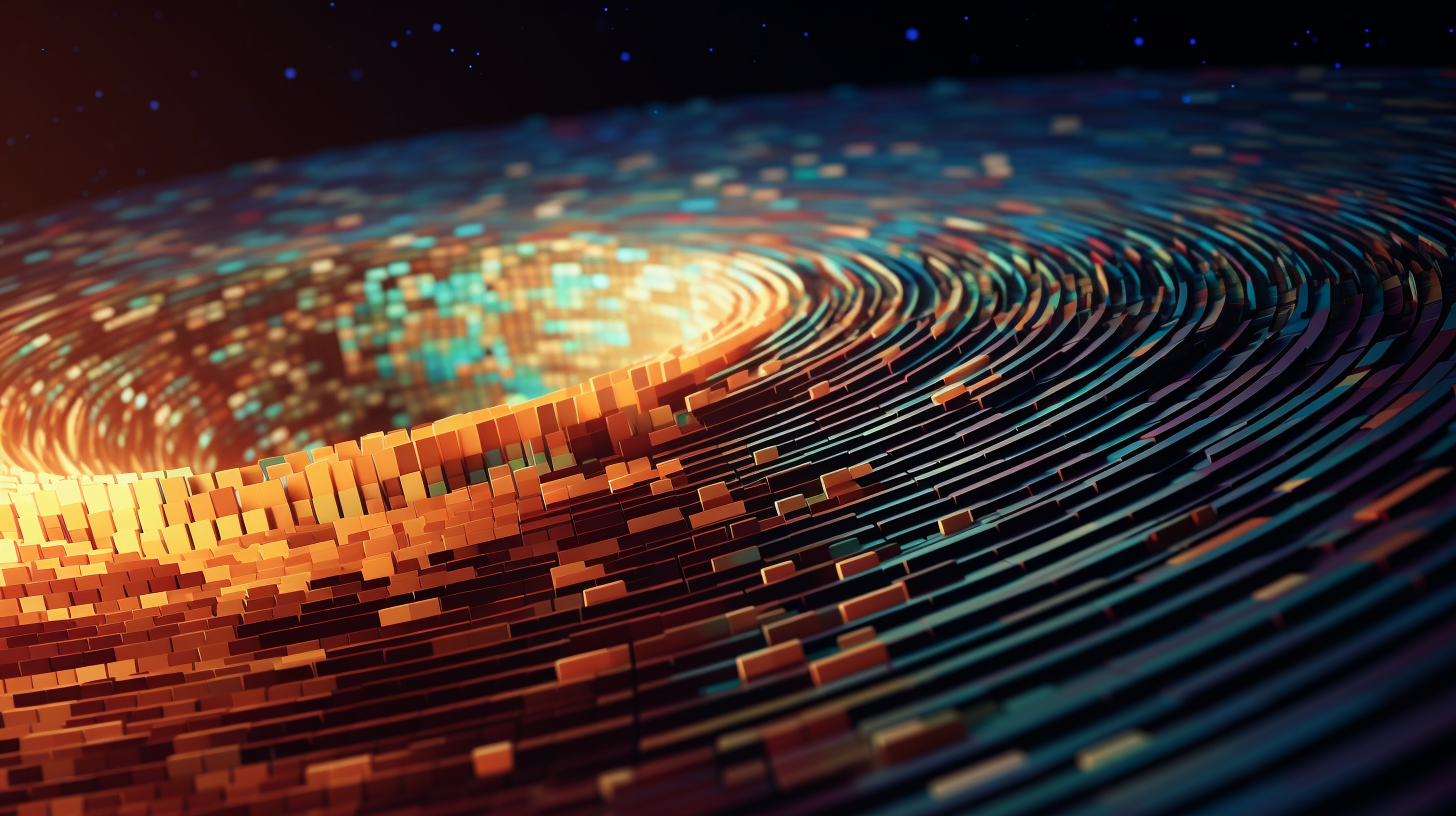
Understanding Error Handling in Swift
Error handling in Swift is an important part of writing robust code. It allows developers to gracefully handle errors when they occur, ensuring that the program can continue to run or terminate in a controlled manner. Swift provides a variety of ways to handle errors, including using do-catch blocks, throwing errors, and handling them as optional values.
In Swift, an error is represented by a value of a type that conforms to the Error protocol. That’s a simple protocol that doesn’t require any additional properties or methods:
protocol Error {}
You can represent an error using any type that conforms to the Error protocol, including enumerations. Enumerations are particularly well suited to error handling because they can group related error conditions together:
enum FileError: Error { case fileNotFound case fileNotReadable case fileCorrupted }
Once you have defined your errors, you can use the throw statement to throw an error. This will immediately transfer the control flow of the program to the nearest enclosing error-handling context:
func readFile(atPath path: String) throws -> String { guard let file = FileHandle(forReadingAtPath: path) else { throw FileError.fileNotFound } let fileData = file.readDataToEndOfFile() guard let fileContents = String(data: fileData, encoding: .utf8) else { throw FileError.fileNotReadable } return fileContents }
It’s important to note that in Swift, error handling is not the same as exception handling found in other languages like Java or C++. Swift’s error handling is more predictable and doesn’t involve unwinding the call stack, making it more efficient and safer to use.
By understanding how to properly handle errors in Swift, you can write more reliable and resilient code that can handle unexpected events gracefully.
Handling Errors Using Do-Catch
When you are working with functions that can throw errors, you’ll need a way to handle these errors at runtime. That’s where the do-catch statement comes into play. The do-catch statement allows you to run a block of code and handle any errors that are thrown by the code within the do block.
To use do-catch, you begin by writing a do statement followed by a block of code that can potentially throw an error. You then write one or more catch clauses to handle the error. Here’s the general structure:
do { // Code that can throw an error } catch { // Error handling }
Within the catch block, you have access to a local constant called error
which represents the error that was thrown. You can use this constant to inspect the error and respond appropriately.
Here’s an example of using do-catch to handle errors when reading a file:
do { let fileContents = try readFile(atPath: "path/to/file.txt") print(fileContents) } catch FileError.fileNotFound { print("The file could not be found.") } catch FileError.fileNotReadable { print("The file is not readable.") } catch { print("An unknown error occurred: (error)") }
In this example, if the readFile(atPath:)
function throws an error, control is immediately transferred to the catch clauses. The first two catch clauses match specific errors, while the last catch clause is a generic catch-all for any other errors that might be thrown.
You can also use multiple catch clauses to handle specific errors, like so:
do { let fileContents = try readFile(atPath: "path/to/file.txt") // Use fileContents } catch FileError.fileNotFound { // Handle file not found error } catch FileError.fileNotReadable, FileError.fileCorrupted { // Handle file not readable or file corrupted error } catch { // Handle any other errors }
This approach allows you to handle different errors in different ways, providing more granular control over your error handling logic.
It is important to note that the try
keyword is used before a function call that can throw an error. This keyword is a signal that an error can be thrown, and the do-catch statement is prepared to handle it.
Using do-catch is a powerful way to handle errors in Swift, so that you can write code that’s both safe and easy to understand.
Propagating Errors Using Throws
When you want to pass an error from a function to the code that calls it, you use the throws
keyword in the function’s declaration. This indicates that the function can throw an error, and it’s the caller’s responsibility to handle it. Propagating errors is a way to delegate the responsibility of error handling to the caller, rather than handling them within the function itself.
func canThrowErrors() throws -> String { // ... function body }
To call a function that can throw an error, you use the try
keyword before the function call. If an error is thrown, it will propagate to the calling code. The calling code can then handle the error using a do-catch statement, or continue to propagate it.
do { let result = try canThrowErrors() // Use result } catch { // Handle the error }
If the calling function is not in a position to handle the error, you can further propagate the error by marking the calling function with throws
as well. This creates a chain of function calls that can throw errors, with the error being handled at the appropriate level in the call stack.
func anotherFunction() throws { let result = try canThrowErrors() // Use result }
When you mark a function with throws
, you are making a promise that the function will handle all possible errors, either by catching them with a do-catch block or by propagating them. If a function calls another function that throws an error but does not handle or propagate the error, Swift will not compile the code.
It is also possible to use the try?
and try!
variations to handle errors in different ways. try?
converts the result to an optional, which will be nil
if an error is thrown, while try!
will cause a runtime error if an error is thrown. These options give you additional flexibility in how you handle errors in Swift.
let result = try? canThrowErrors() // result is an optional let forcedResult = try! canThrowErrors() // runtime error if an error is thrown
Understanding how to propagate errors using throws
is critical for writing functions that interact with potentially erroneous states or external systems. It provides a structured way to handle errors and ensures that your code remains clean and maintainable.
Handling Errors with Optional Values
Handling errors with optional values is another strategy in Swift that can be used to deal with operations that may fail. Optional values either contain a value or they’re nil
to indicate the absence of a value. By using optionals, you can check for the presence of a value before proceeding with any operations that depend on it.
Ponder a function that tries to extract a number from a string:
func extractNumber(from string: String) -> Int? { return Int(string) }
In this function, Int(string)
is a failable initializer for the Int
type, which means it returns an optional Int
. If the string can be converted to an integer, it will return an optional containing the integer value. If not, it will return nil
.
To handle this optional value, you can use optional binding to safely unwrap the Int
:
if let number = extractNumber(from: "123") { print("The number is (number).") } else { print("The string does not contain a valid number.") }
Optional binding is a way to check if an optional contains a value, and if so, to make that value available as a temporary constant or variable. In the example above, if extractNumber
returns a value, it’s unwrapped and assigned to the constant number
, which can then be used within the if
statement’s braces.
Swift also provides the guard
statement, which is used to transfer program control out of a scope if an optional doesn’t contain a value:
func printNumber(from string: String) { guard let number = extractNumber(from: string) else { print("The string does not contain a valid number.") return } print("The number is (number).") }
With guard
, if the extractNumber
function returns nil
, the else
block is executed, printing an error message and exiting the function. Otherwise, number
is available for use in the rest of the function’s scope.
Using optionals and optional binding is a clear and concise way to handle errors in Swift without the need for throwing and catching errors. It allows you to write safe code that is easy to read and maintain.
It is important to note that optionals are not a replacement for error handling, but rather a complementary tool. In some cases, you might want to use optionals for simple checks, and reserve error handling for more complex operations where you need to understand what went wrong, not just that something went wrong.
Source: https://www.plcourses.com/handling-errors-in-swift/