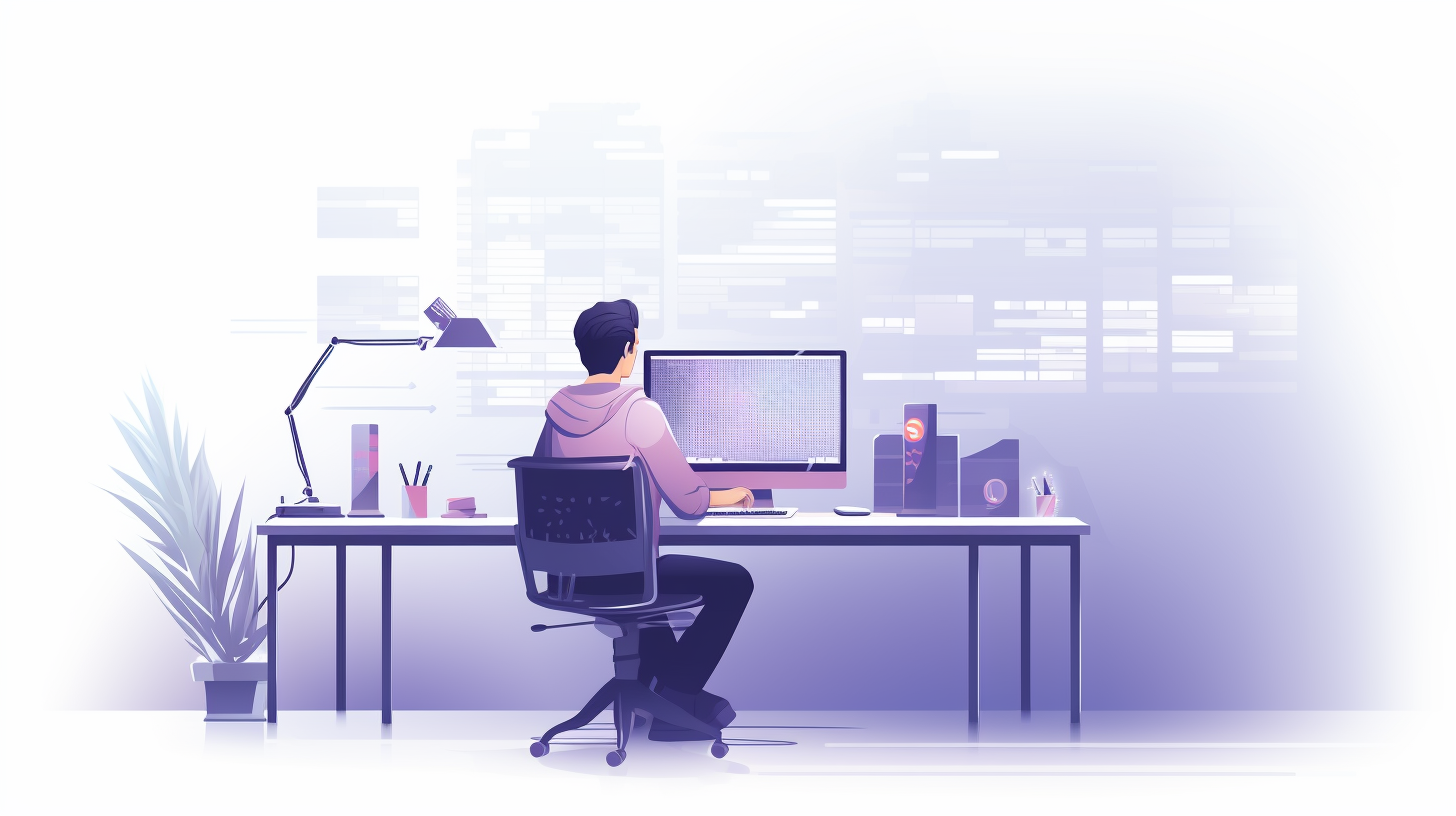
Arithmetic Operators
Arithmetic operators in Java are used to perform basic mathematical operations such as addition, subtraction, multiplication, and division. They can be applied to any primitive data types such as int, float, double, long, short, byte, and char.
The most commonly used arithmetic operators in Java are:
- Addition (+): Adds two values together.
int sum = 10 + 5; // sum is 15
- Subtraction (-): Subtracts one value from another.
int difference = 10 - 5; // difference is 5
- Multiplication (*): Multiplies two values.
int product = 10 * 5; // product is 50
- Division (/): Divides one value by another.
int quotient = 10 / 5; // quotient is 2
- Modulus (%): Returns the remainder of a division.
int remainder = 10 % 3; // remainder is 1
It’s important to note that when performing arithmetic operations, the result will have the same data type as the largest operand. For example, if you add an integer and a double, the result will be a double:
double result = 5 + 3.14; // result is 8.14
In addition to the basic operators, Java also provides compound assignment operators that combine an arithmetic operation with an assignment. These are useful for updating a variable’s value based on its current value:
- Addition assignment (+=): Adds and assigns the value.
int number = 5; number += 3; // number is now 8
- Subtraction assignment (-=): Subtracts and assigns the value.
int number = 5; number -= 3; // number is now 2
- Multiplication assignment (*=): Multiplies and assigns the value.
int number = 5; number *= 3; // number is now 15
- Division assignment (/=): Divides and assigns the value.
int number = 15; number /= 3; // number is now 5
- Modulus assignment (%=): Computes the remainder and assigns the value.
int difference = 10 - 5; // difference is 5
0
Using these operators can make your code more concise and easier to read. For instance:
Instead of writing
number = number + 1;
, you can simply writenumber += 1;
to increment the value of number by one.
Arithmetic operators are fundamental in any programming language and are widely used in various calculations and algorithms. Understanding how to use them effectively is essential for any Java developer.
Comparison Operators
Comparison operators in Java are used to compare two values and return a boolean result, either true
or false
. These operators are essential in controlling the flow of a program through conditional statements such as if
, else
, and loops like while
and for
.
The most commonly used comparison operators in Java are:
- Equal to (==): Checks if two values are equal.
int a = 5; int b = 5; boolean isEqual = (a == b); // isEqual is true
- Not equal to (!=): Checks if two values are not equal.
int a = 5; int b = 6; boolean isNotEqual = (a != b); // isNotEqual is true
- Greater than (>): Checks if the left value is greater than the right value.
int a = 6; int b = 5; boolean isGreater = (a > b); // isGreater is true
- Less than (<): Checks if the left value is less than the right value.
int a = 4; int b = 5; boolean isLess = (a < b); // isLess is true
- Greater than or equal to (>=): Checks if the left value is greater than or equal to the right value.
int a = 5; int b = 5; boolean isGreaterOrEqual = (a >= b); // isGreaterOrEqual is true
- Less than or equal to (<=): Checks if the left value is less than or equal to the right value.
int a = 4; int b = 5; boolean isLessOrEqual = (a <= b); // isLessOrEqual is true
It’s important to use the correct operator for the comparison you intend to perform. For example, using ==
when you meant to use =
can lead to unexpected results, as one is a comparison operator and the other is an assignment operator.
Note: When comparing objects in Java, use the
equals()
method instead of the==
operator. The==
operator compares object references, not the content of the objects.
Comparison operators are often used in conjunction with logical operators to form more complex conditions. For instance:
int score = 75; boolean hasPassed = score >= 50; boolean hasDistinction = score >= 85; if (hasPassed && !hasDistinction) { System.out.println("You passed without distinction."); } else if (hasDistinction) { System.out.println("You passed with distinction!"); } else { System.out.println("Sorry, you did not pass."); }
In this example, we check if a student has passed a test and if they have achieved a distinction. We use comparison operators to evaluate the student’s score and logical operators to combine the conditions. Understanding how to use comparison operators effectively is important for making decisions in your Java programs.
Logical Operators
Logical operators in Java are used to combine multiple boolean expressions and return a single boolean result. These operators are crucial for constructing complex conditional statements and controlling the flow of a program.
The most commonly used logical operators in Java are:
- Logical AND (&&): Returns true if both boolean expressions are true. Otherwise, it returns false.
boolean a = true; boolean b = false; boolean result = a && b; // result is false
- Logical OR (||): Returns true if at least one of the boolean expressions is true. Otherwise, it returns false.
boolean a = true; boolean b = false; boolean result = a || b; // result is true
- Logical NOT (!): Reverses the value of a boolean expression. If the expression is true, it returns false, and vice versa.
boolean a = true; boolean result = !a; // result is false
Logical operators are often used in combination with comparison operators to create more complex conditions:
int x = 10; int y = 20; boolean isWithinRange = (x > 5) && (y < 25); // isWithinRange is true
This code checks if the variable x
is greater than 5 and the variable y
is less than 25. Both conditions must be true for the entire expression to return true.
Note that the logical AND operator (&&) uses short-circuit evaluation. If the first operand evaluates to false, the second operand is not evaluated because the result will be false regardless.
Similarly, the logical OR operator (||) also uses short-circuit evaluation. If the first operand evaluates to true, the second operand is not evaluated because the result will be true regardless.
Understanding how to use logical operators effectively allows you to construct powerful conditional logic in your Java programs.
Source: https://www.plcourses.com/java-operators-types-and-usage/