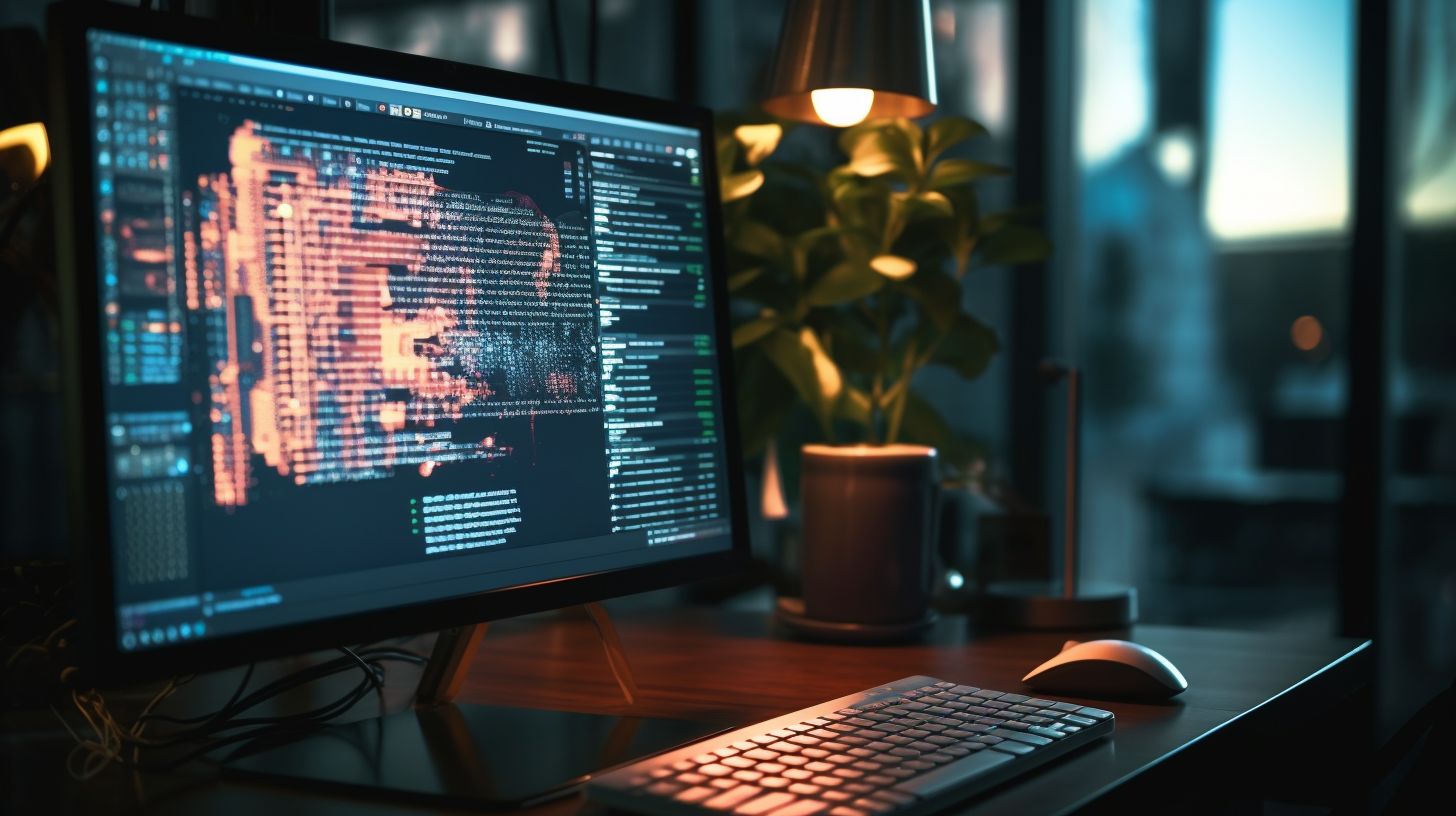
Scikit-learn is a powerful machine learning library for Python that provides a wide range of algorithms for classification, regression, clustering, and dimensionality reduction. It is built upon the foundations of NumPy, SciPy, and matplotlib, making it a robust tool for data analysis and predictive modeling. Scikit-learn is designed to be accessible and efficient, allowing developers to implement complex machine learning models with ease.
One of the key features of Scikit-learn is its consistent API, which allows users to easily switch between different algorithms and compare their results. Whether you’re a beginner or an experienced machine learning practitioner, Scikit-learn offers something for everyone.
The library includes a variety of preprocessing methods, such as feature scaling and encoding categorical variables, which are essential steps in preparing data for machine learning models. Scikit-learn also provides tools for model evaluation and selection, including cross-validation and hyperparameter tuning.
Here’s an example of how to use Scikit-learn to train a simple linear regression model:
from sklearn.linear_model import LinearRegression from sklearn.model_selection import train_test_split from sklearn.metrics import mean_squared_error # Sample data X = [[1], [2], [3], [4]] y = [1, 2, 3, 4] # Splitting data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Initialize the model model = LinearRegression() # Fit the model on the training data model.fit(X_train, y_train) # Make predictions on the test data y_pred = model.predict(X_test) # Evaluate the model mse = mean_squared_error(y_test, y_pred) print(f'Mean Squared Error: {mse}')
Scikit-learn’s extensive documentation and community support make it an indispensable tool in the field of machine learning. Whether you’re working on a small project or a large-scale data science operation, Scikit-learn’s versatility and ease of use can help you achieve your goals.
Overview of Pandas and NumPy
Pandas and NumPy are two of the most popular libraries in Python for data manipulation and numerical computing, respectively. Understanding these libraries is essential when working with Scikit-learn, as they provide the data structures and operations needed for preprocessing and handling data before feeding it into machine learning models.
Pandas is a library that offers data structures and operations for manipulating numerical tables and time series. It is particularly well-suited for handling structured data, where you can use its DataFrame
object to store and manipulate data in a table format. Pandas provides a wide range of functionalities, including the ability to handle missing data, perform group operations, and support for time-series data analysis.
import pandas as pd # Create a simple DataFrame data = {'Name': ['John', 'Anna', 'Peter', 'Linda'], 'Age': [28, 22, 34, 42], 'City': ['New York', 'Paris', 'Berlin', 'London']} df = pd.DataFrame(data) # Accessing data print(df.loc[2, 'Age']) # Output: 34
NumPy, on the other hand, is a library for numerical computing in Python. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. NumPy is extremely fast and efficient because it is implemented in C and provides vectorized operations.
import numpy as np # Create a simple NumPy array arr = np.array([1, 2, 3, 4]) # Perform vectorized operations arr_squared = arr ** 2 print(arr_squared) # Output: [1 4 9 16]
When working with Scikit-learn, you’ll often find yourself converting between Pandas DataFrames
and NumPy arrays. This is because Scikit-learn’s algorithms typically expect data in the form of NumPy arrays. Fortunately, converting between these two formats is straightforward, thanks to the seamless integration provided by both libraries.
# Convert a Pandas DataFrame to a NumPy array numpy_array_from_df = df.values # Convert a NumPy array to a Pandas DataFrame df_from_numpy_array = pd.DataFrame(numpy_array_from_df, columns=df.columns) print(numpy_array_from_df) print(df_from_numpy_array)
The integration of Scikit-learn with Pandas and NumPy simplifies the workflow for machine learning tasks by providing a consistent interface to handle all stages of data processing, from cleaning and preparation to modeling and evaluation.
Data Preparation with Pandas and NumPy
Before we can apply machine learning algorithms to our data, it’s important to prepare it properly. Data preparation involves cleaning the data, dealing with missing values, feature engineering, and many other tasks that are necessary to make the data ready for modeling. Pandas and NumPy offer a wide array of functions and methods to facilitate these processes.
Handling Missing Data:
Missing data can be a significant issue when training machine learning models. Pandas provides several methods to handle missing values, such as dropping rows or columns with missing values or filling them with a specific value or a computed value like the mean or median.
import numpy as np import pandas as pd # Create a DataFrame with missing values df_missing = pd.DataFrame({'A': [1, 2, np.nan, 4], 'B': [np.nan, 2, 3, 4], 'C': [1, 2, 3, np.nan]}) # Drop rows with any missing values df_no_missing_rows = df_missing.dropna() # Fill missing values with the mean of the column df_filled_with_mean = df_missing.fillna(df_missing.mean())
Feature Engineering:
Pandas also excels at feature engineering, which is the process of creating new features from existing ones to improve model performance. This can be done by applying transformations, creating interaction terms, or one-hot encoding categorical variables.
# Create interaction term between two columns df['Interaction'] = df['Age'] * df['B'] # One-hot encode categorical variables df_encoded = pd.get_dummies(df, columns=['City'])
Scaling and Normalization:
Scaling and normalization are critical preprocessing steps, especially for algorithms sensitive to the scale of the data like SVM or k-nearest neighbors. While Scikit-learn offers tools for this, it’s also possible to perform these tasks using Pandas and NumPy.
# Scaling a column to have zero mean and unit variance df['Age_Scaled'] = (df['Age'] - df['Age'].mean()) / df['Age'].std() # Normalizing a column to be between 0 and 1 df['Age_Normalized'] = (df['Age'] - df['Age'].min()) / (df['Age'].max() - df['Age'].min())
Once the data is preprocessed and ready, we can convert it into a format that Scikit-learn’s algorithms can work with, which is typically a NumPy array.
# Convert the preprocessed DataFrame to a NumPy array X = df_encoded.drop('Name', axis=1).values
With these steps completed, our dataset is now properly prepared for integration with Scikit-learn models. Proper data preparation not only facilitates a smoother modeling process but can also lead to more accurate and reliable results.
Integrating Scikit-learn with Pandas and NumPy
Integrating Scikit-learn with Pandas and NumPy is a straightforward process once you have your data prepared. The key to this integration is understanding how to convert your Pandas DataFrames to NumPy arrays, which can then be used directly with Scikit-learn’s machine learning algorithms.
Let’s ponder an example where we have a dataset containing housing prices, and we want to build a regression model to predict these prices based on various features. Our dataset is initially in a Pandas DataFrame format, and we’ve already completed the necessary data preparation steps.
import pandas as pd from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression from sklearn.metrics import mean_squared_error # Assume df is our prepared DataFrame with the last column 'Price' as the target variable X = df.drop('Price', axis=1).values y = df['Price'].values # Splitting the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Initialize the Linear Regression model regressor = LinearRegression() # Fit the model on the training data regressor.fit(X_train, y_train) # Make predictions on the test data predictions = regressor.predict(X_test) # Evaluate the model mse = mean_squared_error(y_test, predictions) print(f'Mean Squared Error: {mse}')
In this example, we first convert our DataFrame into NumPy arrays by using the values
attribute. We then split our data into training and testing sets using Scikit-learn’s train_test_split
function. After initializing and fitting a Linear Regression model, we make predictions and evaluate our model using the mean squared error metric.
Another important aspect of integration is using Scikit-learn’s preprocessing tools alongside Pandas. For instance, we might want to scale our features using Scikit-learn’s StandardScaler:
from sklearn.preprocessing import StandardScaler # Initialize the StandardScaler scaler = StandardScaler() # Fit the scaler on the training data and transform both training and testing sets X_train_scaled = scaler.fit_transform(X_train) X_test_scaled = scaler.transform(X_test) # Re-train our model on the scaled data regressor.fit(X_train_scaled, y_train) # Make predictions on the scaled test data scaled_predictions = regressor.predict(X_test_scaled) # Evaluate the scaled model scaled_mse = mean_squared_error(y_test, scaled_predictions) print(f'Scaled Mean Squared Error: {scaled_mse}')
In this case, we use the StandardScaler
to standardize our features. Note that we fit the scaler only on the training data and then transform both the training and testing sets. That’s to avoid any information leak from the test set into our model during training.
The integration of Scikit-learn with Pandas and NumPy is not limited to linear regression; it extends to all types of models offered by Scikit-learn. The process remains consistent: prepare your data with Pandas and NumPy, convert it to NumPy arrays, and feed it into Scikit-learn’s machine learning algorithms. This seamless integration makes Python a powerful tool for machine learning applications.
Case Study: Applying Scikit-learn with Pandas and NumPy
In this case study, we’ll demonstrate how to apply Scikit-learn with Pandas and NumPy for a classification task using the Iris dataset. The Iris dataset is a well-known dataset in the machine learning community, which includes measurements for iris flowers of three different species.
First, we will load the data using Pandas, perform some basic preprocessing, and then use Scikit-learn to build a classifier. We will also evaluate the performance of our model using various metrics provided by Scikit-learn.
import pandas as pd from sklearn.datasets import load_iris from sklearn.model_selection import train_test_split from sklearn.preprocessing import StandardScaler from sklearn.neighbors import KNeighborsClassifier from sklearn.metrics import classification_report, confusion_matrix # Load Iris dataset iris = load_iris() df_iris = pd.DataFrame(data= np.c_[iris['data'], iris['target']], columns= iris['feature_names'] + ['target']) # Split the dataset into features and target variable X = df_iris.iloc[:, :-1].values y = df_iris.iloc[:, -1].values # Split the data into training and test sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42) # Initialize StandardScaler scaler = StandardScaler() # Fit on training set only scaler.fit(X_train) # Apply transform to both the training set and the test set X_train = scaler.transform(X_train) X_test = scaler.transform(X_test) # Initialize KNN classifier classifier = KNeighborsClassifier(n_neighbors=5) # Train the model using the training sets classifier.fit(X_train, y_train) # Predictions on the test set y_pred = classifier.predict(X_test) # Evaluating the Algorithm print(confusion_matrix(y_test, y_pred)) print(classification_report(y_test, y_pred))
In this example, we load the Iris dataset and convert it into a Pandas DataFrame. We then split our data into feature variables X
and the target variable y
. We follow this by splitting our data into a training set and a test set using Scikit-learn’s train_test_split
function.
We use Scikit-learn’s StandardScaler
to standardize our features. Standardization involves rescaling the features so that they have a mean of 0 and a standard deviation of 1. That is important because features with larger scales can disproportionately influence the model.
Next, we initialize the KNeighborsClassifier from Scikit-learn with n_neighbors=5
. After training our classifier on the training data, we make predictions for the test set and print out a confusion matrix and a classification report to evaluate our model. The confusion matrix gives us an insight into the number of correct and incorrect predictions for each class, while the classification report provides key metrics such as precision, recall, and f1-score.
As we can see, integrating Scikit-learn with Pandas and NumPy is not only efficient but also allows us to take advantage of the powerful data handling capabilities of Pandas along with the high-performance computing power of NumPy. This integration simplifies the process of building and evaluating machine learning models in Python.
Source: https://www.pythonlore.com/scikit-learn-integration-with-pandas-and-numpy/