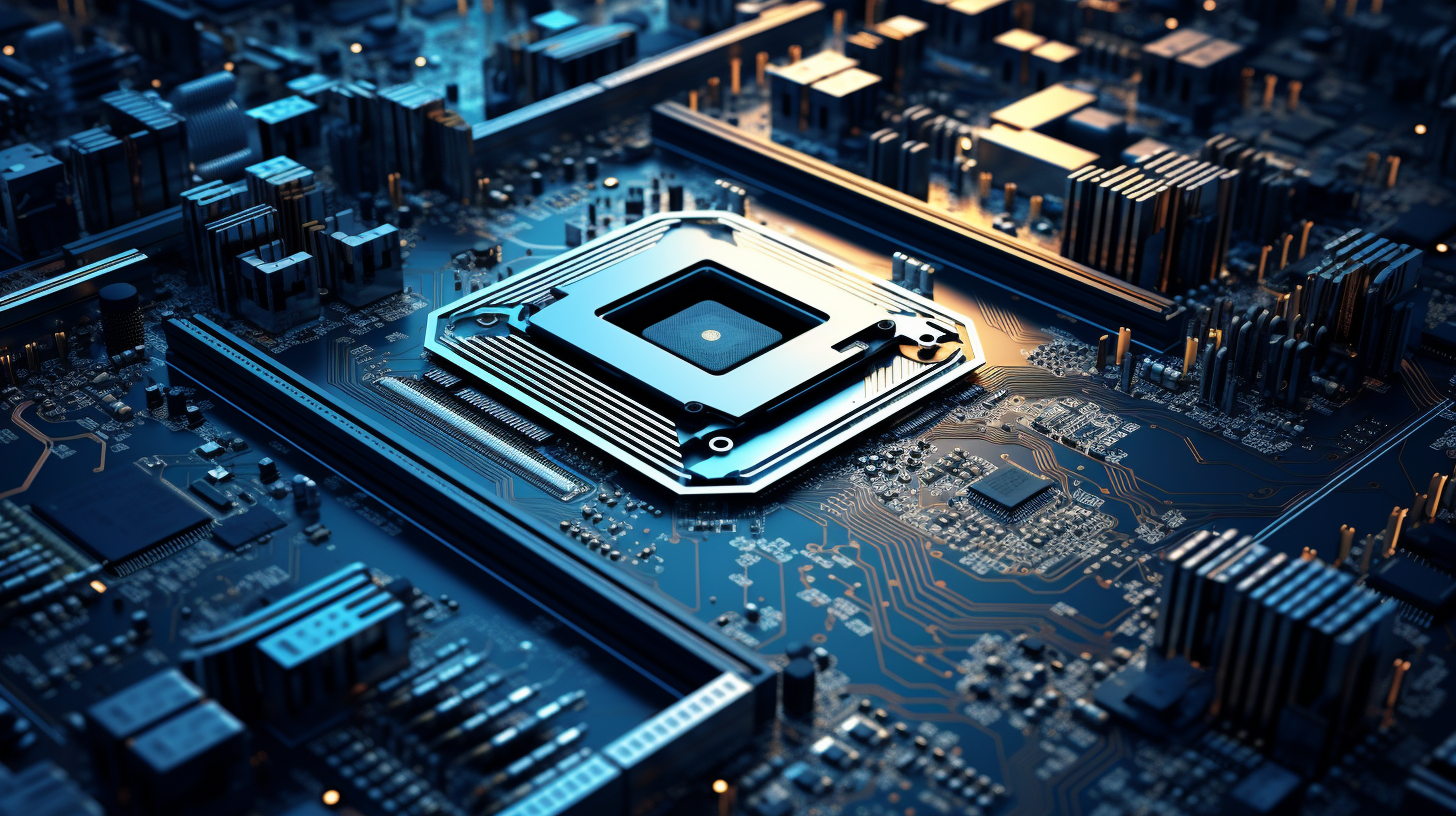
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write, and also easy for machines to parse and generate. Python provides built-in support for working with JSON through its json module. This tutorial will explore how to use Python to store and retrieve data in JSON format.
What is JSON?
JSON is a format for storing and transporting data. It is often used in web applications to transmit data between a server and a web page. JSON data is represented as key-value pairs, where the keys are strings and the values can be strings, numbers, booleans, arrays, or other JSON objects. Here is an example of JSON data:
{ "name": "Mitch Carter", "age": 30, "is_active": true, "hobbies": ["reading", "coding", "gaming"], "address": { "street": "123 Main St", "city": "New York", "state": "NY" } }
Storing Data in JSON Format
To store data in JSON format, we need to convert our Python objects into JSON. The json module provides the dump()
function to write JSON data to a file-like object. Here’s an example:
import json data = { "name": "Mitch Carter", "age": 30, "is_active": True, "hobbies": ["reading", "coding", "gaming"], "address": { "street": "123 Main St", "city": "New York", "state": "NY" } } # Open a file to write JSON data with open('data.json', 'w') as file: json.dump(data, file)
This code snippet creates a Python dictionary called data
and stores some key-value pairs in it. Then, it uses the json.dump()
function to write the contents of data
to a file named data.json
.
Retrieving Data from JSON Format
To retrieve data from a JSON file, we need to parse the JSON data and convert it back into Python objects. The json module provides the load()
function to read JSON data from a file-like object. Here’s an example:
import json # Open a file to read JSON data with open('data.json', 'r') as file: data = json.load(file) print(data)
This code snippet opens the data.json
file in read mode and uses the json.load()
function to parse its contents and convert them back into Python objects. The resulting data is stored in the data
variable, which we then print out to see the retrieved JSON data.
Parsing JSON Data
In addition to reading JSON data from a file, we can also parse JSON data directly from a string using the loads()
function. Here’s an example:
import json json_data = '{"name": "Mitch Carter", "age": 30, "is_active": true}' data = json.loads(json_data) print(data)
In this code snippet, we have a JSON string stored in the json_data
variable. We use the json.loads()
function to parse the JSON string and convert it into a Python object, which we then print out.
Python provides a convenient and easy-to-use module, called json, for storing and retrieving data in JSON format. You can store Python objects in JSON format using the json.dump()
function, and retrieve JSON data using the json.load()
function. Additionally, you can parse JSON data directly from a string using the json.loads()
function. By leveraging the power of JSON and Python, you can easily handle data storage and retrieval in your applications.
Source: https://www.plcourses.com/python-and-json-data-storage-and-retrieval/