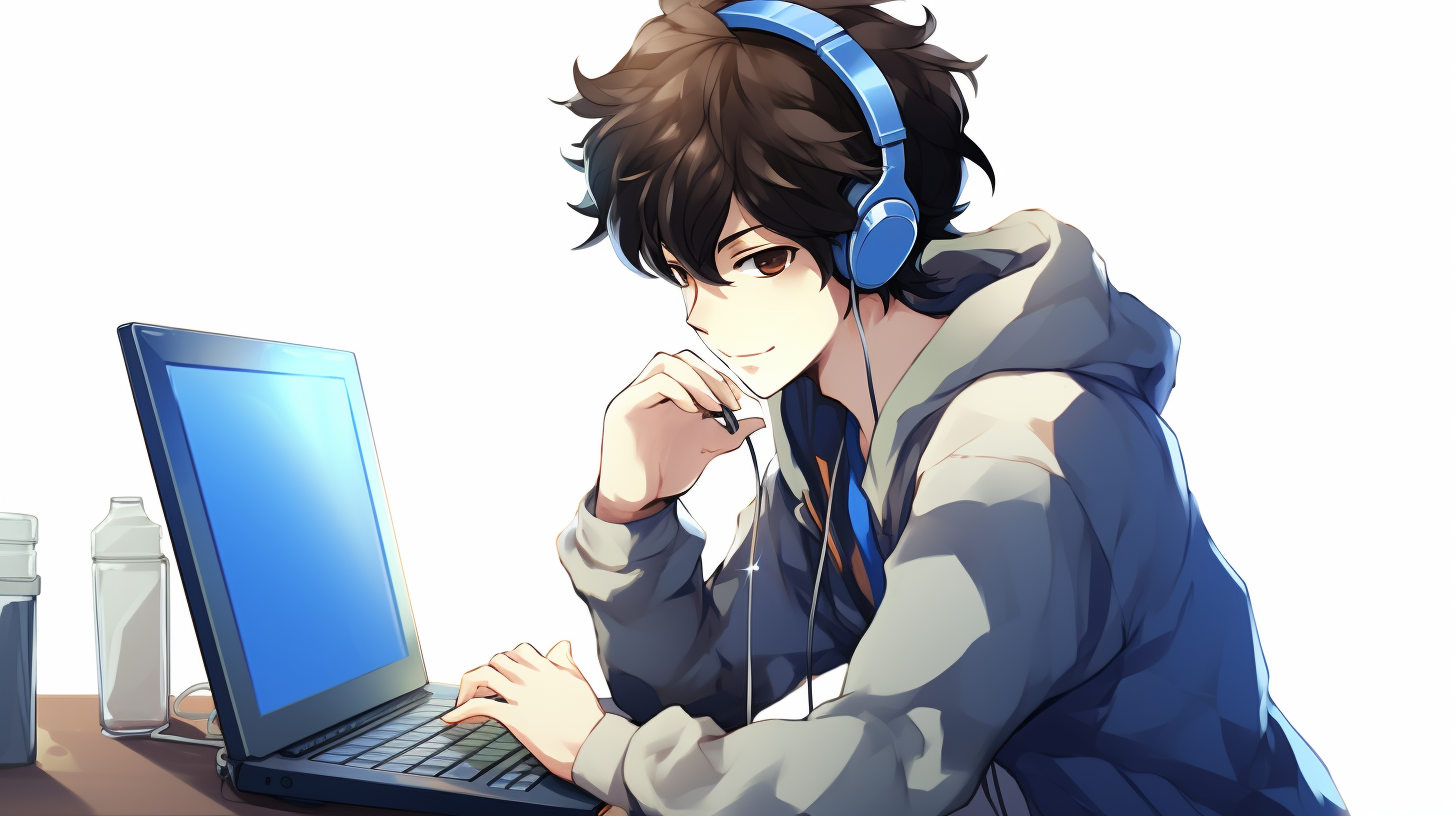
JSON, which stands for JavaScript Object Notation, is a lightweight data-interchange format that’s easy for humans to read and write and easy for machines to parse and generate. It is based on a subset of the JavaScript Programming Language Standard ECMA-262 3rd Edition – December 1999. JSON is a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others. These properties make JSON an perfect data-interchange language.
JSON is built on two structures:
- A collection of name/value pairs. In various languages, this is realized as an object, record, struct, dictionary, hash table, keyed list, or associative array.
- An ordered list of values. In most languages, this is realized as an array, vector, list, or sequence.
These are universal data structures. Virtually all state-of-the-art programming languages support them in one form or another. It makes sense that a data format that’s interchangeable with programming languages also be based on these structures.
In JSON, they take on these forms:
{ "firstName": "John", "lastName": "Smith", "isAlive": true, "age": 25, "address": { "streetAddress": "21 2nd Street", "city": "New York", "state": "NY", "postalCode": "10021-3100" }, "phoneNumbers": [ { "type": "home", "number": "212 555-1234" }, { "type": "office", "number": "646 555-4567" } ], "children": [], "spouse": null }
This makes JSON an perfect format for data interchange when building web applications or APIs that communicate with client-side JavaScript or mobile applications, as well as for storing configuration data or communicating between different parts of a distributed system.
Exploring the json.dumps Function in Python
Python’s json module provides a method called dumps
which stands for “dump string”. This method is used to convert a Python object into a JSON string. The dumps
function takes several optional parameters that allow for customization of the serialization process.
Let’s look at a simple example of converting a Python dictionary to a JSON string using json.dumps
:
import json data = { "firstName": "John", "lastName": "Doe", "isAlive": True, "age": 27 } json_string = json.dumps(data) print(json_string)
In the code above, we define a Python dictionary named data
with some key-value pairs. We then use the dumps
function to convert this dictionary into a JSON string. The output of the print statement would be:
{"firstName": "John", "lastName": "Doe", "isAlive": true, "age": 27}
Note that the boolean value True
in Python is converted to true
in JSON, following JSON’s syntax rules.
The dumps
function also allows us to control aspects of the JSON output, such as indentation, sorting keys, and more. For example, if we want to pretty-print the JSON with an indentation of 4 spaces, we can do the following:
json_string = json.dumps(data, indent=4) print(json_string)
This would result in the following more readable output:
{ "firstName": "John", "lastName": "Doe", "isAlive": true, "age": 27 }
Other useful options available in dumps
include:
- sort_keys: When set to
True
, the keys in the output will be sorted alphabetically. - separators: A tuple specifying how to separate items in the JSON output. By default, it’s set to
(', ', ': ')
, but it can be customized. - skipkeys: When set to
True
, keys that are not of a basic type (string, number, boolean, None) will be skipped instead of raising aTypeError
.
An example using these optional parameters could look like this:
json_string = json.dumps(data, indent=4, sort_keys=True, separators=(',', ': ')) print(json_string)
This would generate a nicely formatted and sorted JSON string with custom separators.
Understanding the capabilities of the dumps
function is important for effectively converting Python objects to JSON strings, especially when dealing with complex data structures or when you need to ensure that the output conforms to specific formatting requirements.
Converting Python Objects to JSON Strings: Key Concepts and Considerations
When converting Python objects to JSON strings using json.dumps
, it’s important to understand how different Python data types are mapped to JSON. For instance, Python dictionaries are converted to JSON objects, lists and tuples become JSON arrays, strings remain strings, booleans are converted to their corresponding true or false values in JSON, and None becomes null. However, not all Python data types can be directly serialized to JSON. Types like datetime, bytes, or custom objects require special handling.
For example, if we try to serialize a Python object that includes a datetime, the json.dumps
function will raise a TypeError:
import json from datetime import datetime data = { "timestamp": datetime.now(), "message": "Hello, world!" } # This will raise a TypeError json_string = json.dumps(data)
To handle this situation, we can use the default parameter of the dumps
function to specify a function that will be called for objects that can’t be serialized natively. This function should return a serializable version of the object or raise a TypeError if it cannot handle the object:
def default_converter(o): if isinstance(o, datetime): return o.__str__() json_string = json.dumps(data, default=default_converter) print(json_string)
In the code above, we define a default_converter
function that checks if the object is an instance of datetime and returns its string representation. The dumps
function then uses this converter to serialize the datetime object.
It’s also worth noting that while json.dumps
can handle most of the basic Python data types, it does not support serializing custom objects by default. If you need to serialize a custom object, you’ll have to provide a serialization method yourself. One common approach is to define a to_json
method in your custom class that returns a dictionary representation of the object:
class User: def __init__(self, name, age): self.name = name self.age = age def to_json(self): return { "name": self.name, "age": self.age } user = User("Alice", 30) json_string = json.dumps(user.to_json()) print(json_string)
This method ensures that your custom objects can be serialized in a way that’s compatible with JSON while still retaining control over how the object is represented.
In summary, when using json.dumps
for converting Python objects to JSON strings, keep in mind the mapping between Python and JSON data types, handle non-serializable types with the default parameter, and provide serialization methods for custom objects. These considerations will help you create JSON strings that accurately represent your data and can be easily consumed by other systems or applications.
Advanced Techniques and Best Practices for json.dumps Usage
When working with json.dumps
, there are several advanced techniques and best practices that can help streamline the process and produce more efficient JSON strings. Here are some tips to consider:
- Use the
ensure_ascii
parameter: By default,json.dumps
will escape any non-ASCII characters in the output with Unicode escape sequences. This can make the output harder to read and can increase the size of the JSON string. If you’re sure that the consumer of your JSON can handle non-ASCII characters, you can setensure_ascii=False
to prevent this behavior. - Handle large data sets with
json.JSONEncoder
: When dealing with very large data sets, usingjson.dumps
can be memory-intensive since it generates the entire JSON string in memory. Instead, you can subclassjson.JSONEncoder
and override itsiterencode()
method to encode the data incrementally. - Customize serialization with
cls
parameter: If you have special serialization needs or want to serialize custom objects without defining a separate method for each class, you can subclassjson.JSONEncoder
and pass it as thecls
parameter tojson.dumps
. Your custom encoder can then define how different objects are serialized.
Let’s take a look at how these techniques can be applied in practice:
import json # Non-ASCII characters example data = { "name": "José", "age": 27 } # Without ensure_ascii=False, the non-ASCII character 'é' will be escaped json_string = json.dumps(data) print(json_string) # Output: {"name": "Josu00e9", "age": 27} # With ensure_ascii=False, 'é' will be preserved json_string = json.dumps(data, ensure_ascii=False) print(json_string) # Output: {"name": "José", "age": 27}
To handle large data sets incrementally, you could use a custom JSON encoder like this:
class LargeDataSetEncoder(json.JSONEncoder): def iterencode(self, o, _one_shot=False): # Custom encoding logic for large data sets # Yield each string chunk instead of building the entire JSON string in memory pass # Use the custom encoder json_string_chunks = LargeDataSetEncoder().iterencode(large_data_set) for chunk in json_string_chunks: # Process each chunk (e.g., write it to a file or send it over a network) pass
If you need to serialize custom objects, create a custom JSON encoder:
class CustomEncoder(json.JSONEncoder): def default(self, o): if isinstance(o, CustomObject): return o.to_json() # Assume CustomObject has a to_json method return super().default(o) # Use the custom encoder custom_object = CustomObject() json_string = json.dumps(custom_object, cls=CustomEncoder) print(json_string)
To wrap it up, using these advanced techniques and best practices when using json.dumps
can lead to more readable, efficient, and flexible JSON serialization. Keep these tips in mind as you work with JSON in Python to improve your data interchange capabilities.
Source: https://www.pythonlore.com/understanding-json-dumps-for-converting-python-objects-to-json-strings/