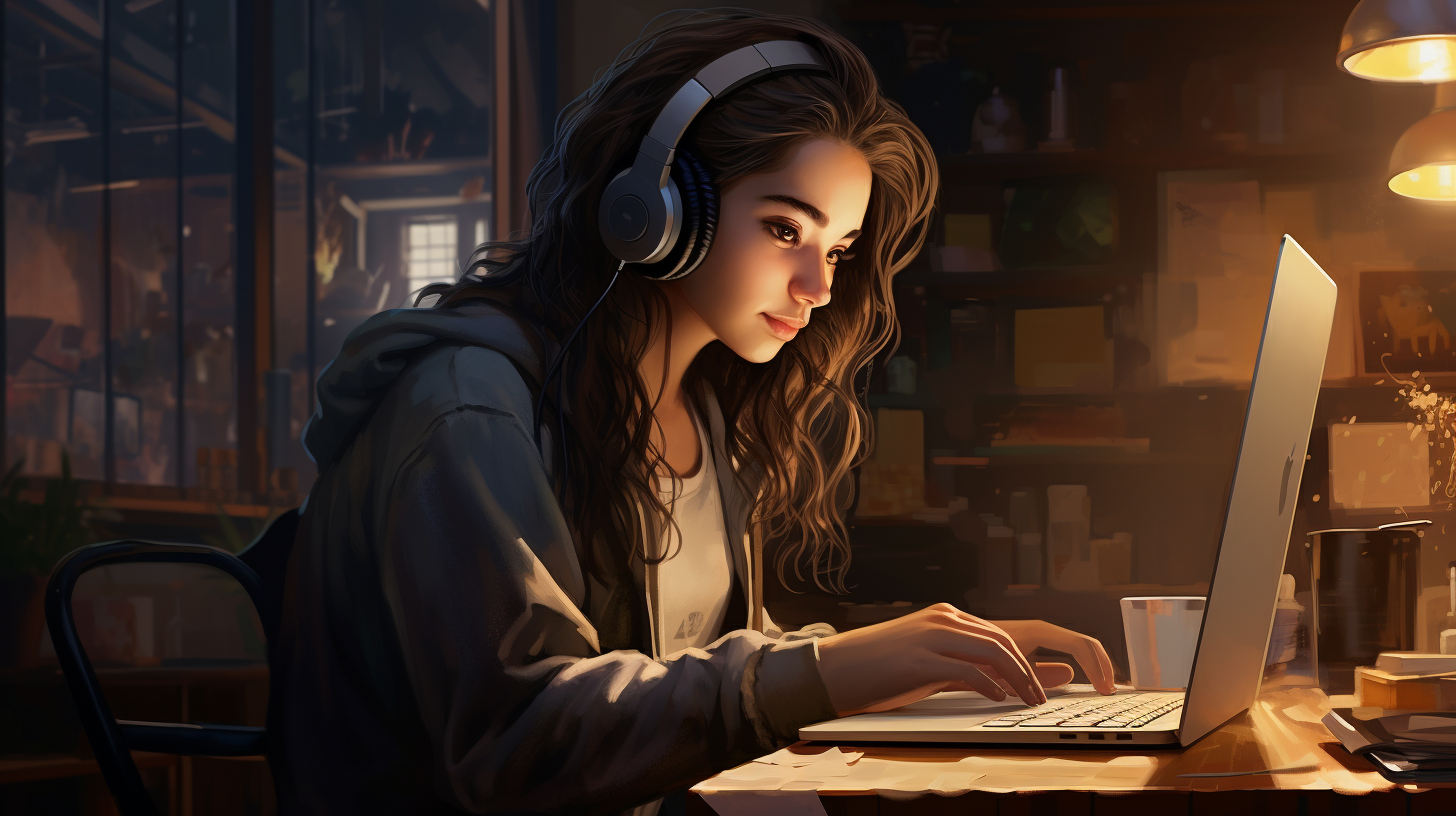
Introduction to Java Servlets
Java Servlets are server-side Java programs that handle client requests and generate dynamic web content. They run in a servlet container, which is a part of a web server that interacts with the servlets. Servlets are an essential part of any Java-based web application and are used to extend the capabilities of servers that host applications accessed by means of a request-response programming model.
Servlets can respond to any type of request but are commonly used to extend the applications hosted by web servers. They work by receiving a request from a client, processing it, and then returning a response. This request-response model is what makes servlets so powerful in creating dynamic web content.
One of the key advantages of using Java Servlets is that they are platform-independent, which means they can run on any operating system that has a Java Virtual Machine (JVM). They also provide a way to generate HTML pages dynamically, which means that the content of the page can change based on user input or other factors.
//Example of a simple Hello World Servlet import java.io.*; import javax.servlet.*; import javax.servlet.http.*; public class HelloWorldServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { PrintWriter out = response.getWriter(); out.println("<html>"); out.println("<body>"); out.println("<h1>Hello, World</h1>"); out.println("</body>"); out.println("</html>"); } }
The above code is an example of a simple servlet that generates an HTML page with a “Hello, World” message. The doGet method is called when a GET request is made to the servlet. This method generates the HTML content and writes it to the response object, which is then sent back to the client.
Servlets are also capable of handling more complex tasks, such as interacting with databases, performing calculations, and managing user sessions. They can be used in combination with other Java technologies, such as JavaServer Pages (JSP) and JavaBeans, to create powerful and scalable web applications.
Java Servlets provide a robust and scalable way to build web applications. They offer a high number of benefits, including platform independence, dynamic content generation, and the ability to handle complex tasks. In the next sections, we will delve deeper into the key components and functionality of Java Servlets as well as best practices for building web applications using this technology.
Key Components and Functionality of Java Servlets
Java Servlets consist of several key components that work together to process client requests and generate dynamic web content. Understanding these components and their functionality is important for any Java developer working with servlets. Let’s take a closer look at some of the most important ones.
- Servlet Interface: All servlets must implement the Servlet interface, which defines lifecycle methods such as
init()
,service()
, anddestroy()
. These methods are called by the servlet container to manage the lifecycle of a servlet.
public interface Servlet { public void init(ServletConfig config) throws ServletException; public void service(ServletRequest req, ServletResponse res) throws ServletException, IOException; public void destroy(); }
doGet()
, doPost()
, etc. Most servlets will extend this class instead of directly implementing the Servlet interface.public abstract class HttpServlet extends GenericServlet { protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { // Handle GET request } protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { // Handle POST request } // Other HTTP-specific methods... }
public interface ServletConfig { public String getServletName(); public ServletContext getServletContext(); public String getInitParameter(String name); }
public interface ServletRequest { public Object getAttribute(String name); public String getParameter(String name); // Other methods... } public interface ServletResponse { public void setContentType(String type); public PrintWriter getWriter() throws IOException; // Other methods... }
These components work together to allow a servlet to handle client requests and generate responses. For example, when a client sends an HTTP GET request to a web server, the servlet container will call the doGet()
method of the corresponding servlet. The servlet can then use the HttpServletRequest object to read any request parameters and the HttpServletResponse object to generate an HTML page or other content to return to the client.
The combination of these key components allows Java Servlets to effectively process client requests, access web application resources, and produce dynamic content, making them an integral part of Java-based web applications.
Best Practices for Building Web Applications with Java Servlets
When building web applications with Java Servlets, it’s important to adhere to best practices to ensure your application is secure, maintainable, and efficient. Below are some essential best practices to consider:
- Use MVC Architecture: Implement the Model-View-Controller (MVC) pattern to separate concerns and make your application easier to manage. This involves creating servlets that act as controllers, JSPs or other templating systems for views, and JavaBeans or other business objects for the model.
- Implement Robust Exception Handling: Always catch and handle exceptions properly in your servlets. Provide meaningful error messages to users and log exceptions for debugging purposes.
- Minimize Servlet Concurrency Issues: Use thread-safe practices since servlets are inherently multi-threaded. Avoid using instance variables in servlets unless they are read-only or you have implemented proper synchronization.
- Use Connection Pooling: If your application interacts with a database, use connection pooling to manage database connections efficiently and improve performance.
- Optimize Servlet Initialization: Perform expensive operations such as opening database connections or reading configuration files in the init() method of your servlet rather than in the service methods (doGet, doPost).
- Ensure Proper Session Management: Use HTTP sessions judiciously, as they can consume a lot of memory. Also, protect sensitive data by implementing session timeouts and handling session fixation attacks.
- Utilize Servlet Filters: Use filters to preprocess requests and post-process responses for tasks such as logging, authentication, and input validation.
- Follow Secure Coding Practices: Protect against common security vulnerabilities such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
Here’s an example of how you might implement connection pooling in a servlet:
import javax.servlet.ServletException; import javax.sql.DataSource; import java.sql.Connection; import java.sql.SQLException; import javax.annotation.Resource; public class DatabaseAccessServlet extends HttpServlet { @Resource(name = "jdbc/MyDataSource") private DataSource dataSource; protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { try (Connection connection = dataSource.getConnection()) { // Use the connection to interact with the database } catch (SQLException e) { throw new ServletException("Unable to connect to database", e); } } }
This example uses the @Resource annotation to inject a DataSource object, which is configured for connection pooling. The servlet obtains a database connection from the pool in the doGet method, uses it, and then automatically returns it to the pool when it’s done.
By following these best practices, you can build robust, secure, and high-performing web applications with Java Servlets.
Source: https://www.plcourses.com/java-servlets-building-web-applications/