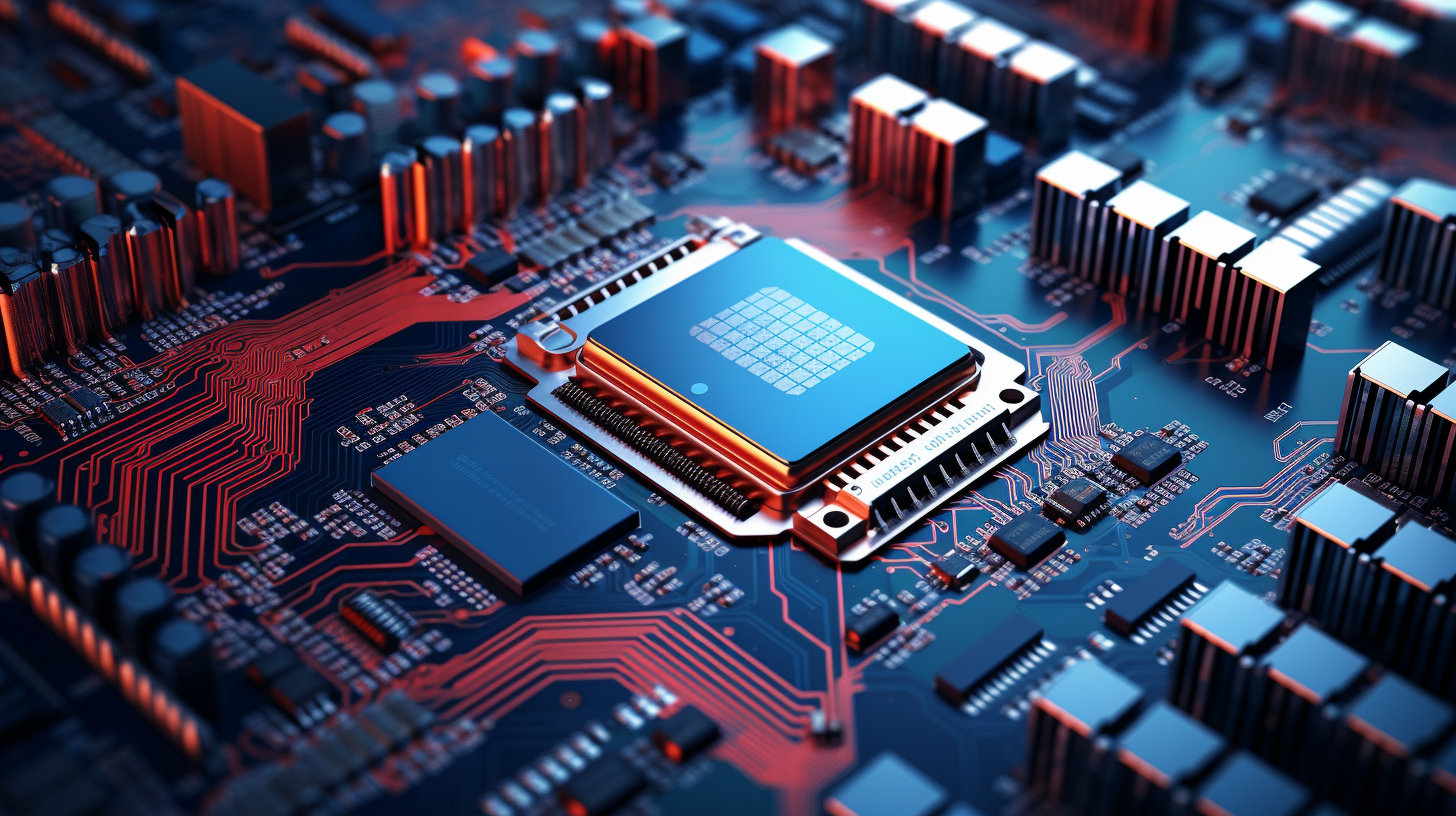
Understanding JavaScript Performance Bottlenecks
JavaScript performance bottlenecks can arise from various areas within your code. To optimize your application effectively, it’s important to identify what is causing the slowdown. One common bottleneck is poorly written algorithms that can lead to inefficient code execution. For example, a misused for-loop that unnecessarily iterates through an array multiple times can drastically impact performance.
for (let i = 0; i < array.length; i++) { // Inefficient code that runs multiple times }
Another bottleneck can be found in the way you manipulate the DOM (Document Object Model). Excessive or unnecessary DOM updates can cause a significant reduction in speed, as they require the browser to re-render parts of the page.
// Updating the DOM in a loop - a common performance bottleneck for (let i = 0; i < items.length; i++) { document.getElementById('list').innerHTML += '
Memory leaks are another type of bottleneck where unused memory is not properly released, leading to increased memory usage and eventually slowing down the application. This can happen when event listeners are not removed after they are no longer needed or when global variables are overused.
// Potential memory leak from an event listener document.getElementById('button').addEventListener('click', function() { // Event listener logic });
- Garbage Collection: JavaScript engines use garbage collection to manage memory, but this process can also be a source of performance issues. If the garbage collector is triggered too often, it can lead to pauses in code execution, known as “garbage collection jank.”
- Forced Synchronous Layout: Also known as “layout thrashing,” this occurs when JavaScript forces the browser to perform layout operations repeatedly within a short span of time, which can severely degrade performance.
By understanding these common bottlenecks, developers can start to write more optimized JavaScript code. It’s essential to profile and measure your code’s performance to identify specific issues within your application.
Remember: Always measure before and after making changes to ensure that your optimizations are having the desired effect on performance.
Techniques for Optimizing JavaScript Code
Optimizing JavaScript code is important for improving the performance of your web applications. Here are some techniques that can help you write more efficient code:
- Minimize DOM Access: Accessing the DOM can be expensive. Minimize interactions with the DOM by caching references to elements and only updating the DOM when necessary.
// Cache DOM reference var list = document.getElementById('list'); // Use cached reference to update DOM list.innerHTML += '' + newItem + '';
// Inefficient for (let i = 0; i < items.length; i++) { let result = calculateValue(i) * CONSTANT_VALUE; } // Optimized let constantCalculation = CONSTANT_VALUE; for (let i = 0; i < items.length; i++) { let result = calculateValue(i) * constantCalculation; }
// Debounce example function debounce(func, wait) { var timeout; return function() { clearTimeout(timeout); timeout = setTimeout(func, wait); }; } window.addEventListener('resize', debounce(function() { // Event handler logic }, 100));
Remember, optimization is about making smart choices, not just about following best practices blindly. Always use profiling tools to identify which parts of your code are the bottlenecks and focus your optimization efforts there.
“Premature optimization is the root of all evil.” – Donald Knuth
While it’s important to be mindful of performance, it’s also important not to sacrifice readability and maintainability of your code for the sake of optimization. Striking a balance is key.
Tools and Best Practices for Measuring and Improving Performance
When it comes to measuring and improving JavaScript performance, there are a high number of tools and best practices that can help developers pinpoint issues and optimize their code. Here’s a rundown of some of the most useful approaches:
- Profiling Tools: State-of-the-art browsers come equipped with powerful profiling tools within their developer consoles. Tools like Chrome’s DevTools allow you to record and analyze your website’s run-time performance, giving you insights into where bottlenecks occur. These tools can show you how much time is spent on script execution, rendering, painting, and other tasks.
// Example of using console.time() and console.timeEnd() for simple performance measurement console.time('Array initialize'); var arr = new Array(1000000); for (var i = 0; i < arr.length; i++) { arr[i] = new Object(); } console.timeEnd('Array initialize'); // Outputs: Array initialize: 1234.567ms
// Example of using Performance API performance.mark('startTask'); // Task code here performance.mark('endTask'); performance.measure('measureTask', 'startTask', 'endTask'); var duration = performance.getEntriesByName('measureTask')[0].duration; console.log('Task duration: ' + duration + 'ms');
- Reducing the number of HTTP requests by bundling files and using sprites.
- Optimizing images and other assets.
- Using content delivery networks (CDNs) to reduce latency.
- Implementing caching strategies.
Always validate the impact of your optimizations by measuring performance before and after changes. Sometimes, what seems like an optimization may not have a significant effect or could even make performance worse.
By combining these tools and practices with the techniques discussed earlier in this article, developers can effectively measure and improve the performance of their JavaScript applications, leading to faster load times, smoother interactions, and an overall better user experience.
Source: https://www.plcourses.com/javascript-performance-optimization/