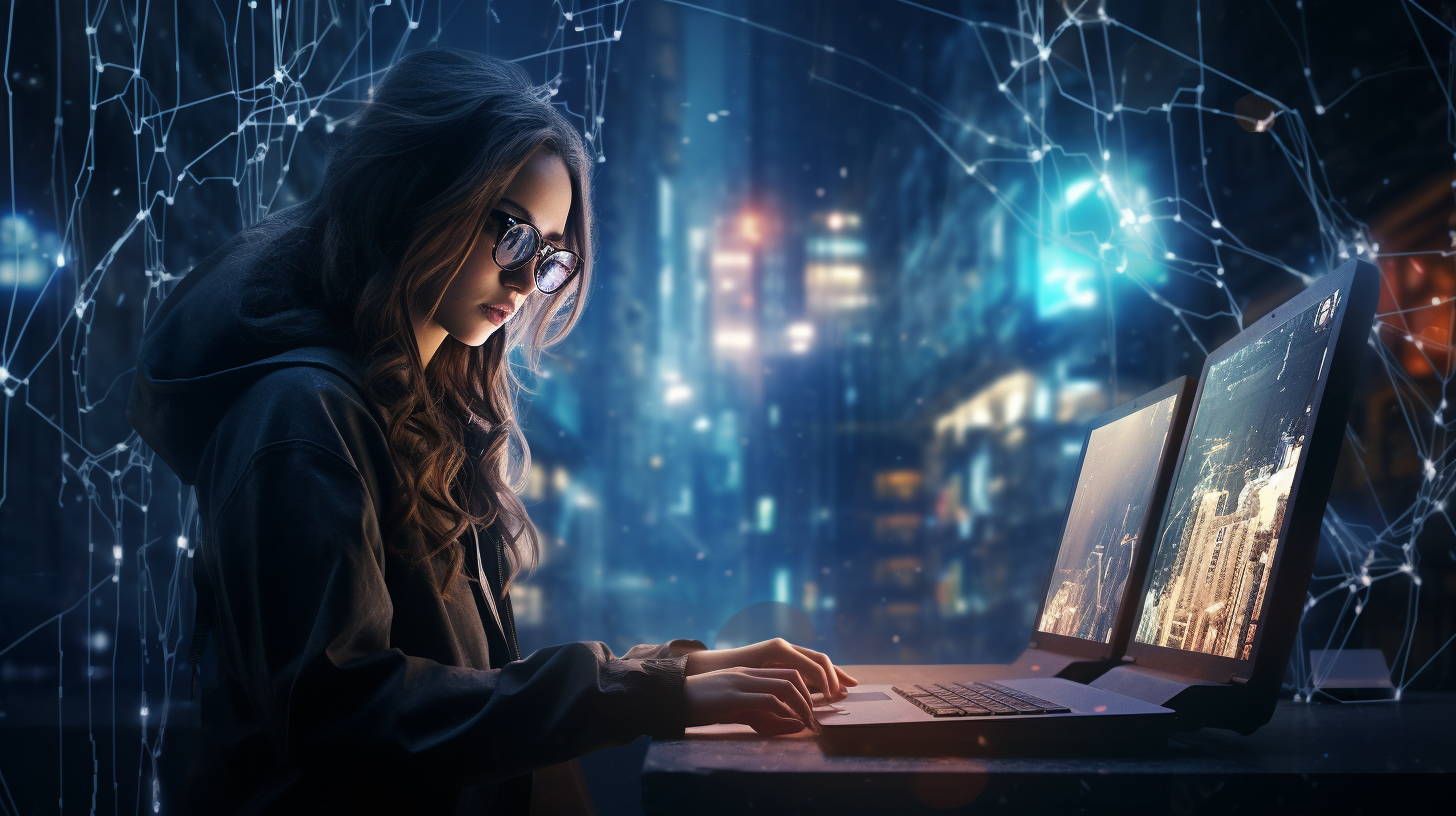
Introduction to Swift Programming Language
Swift is a powerful and intuitive programming language created by Apple for building apps for iOS, Mac, Apple TV, and Apple Watch. It’s designed to give developers more freedom than ever before. Swift is uncomplicated to manage and open source, so anyone with an idea can create something incredible.
Developers often favor Swift for its speed and efficiency, as well as its clean syntax which makes the code easy to read and write. Swift incorporates safe programming patterns and adds contemporary features to make programming easier, more flexible, and more fun.
Swift’s strong typing and error handling prevent errors and crashes in production, making it a reliable choice for app development. Here is an example of Swift’s syntax:
// Define a constant using 'let' let maximumNumberOfLoginAttempts = 10 // Define a variable using 'var' var currentLoginAttempt = 0 // Define a simple function func greet(person: String) -> String { let greeting = "Hello, " + person + "!" return greeting }
Swift also includes features like optionals, which handle the absence of a value, and closures, which are self-contained blocks of functionality that can be passed around in your code. Here’s an example using both:
// Optional variable var optionalString: String? = "Hello" // Using optional binding to unwrap the optional if let unwrappedString = optionalString { print(unwrappedString) } else { print("The optional was nil") } // Closure example let names = ["Chris", "Alex", "Ewa", "Barry", "Daniella"] var reversedNames = names.sorted(by: { (s1: String, s2: String) -> Bool in return s1 > s2 })
As Swift continues to evolve, it remains focused on performance, design, and safety. This makes it an excellent choice for developers looking to create fast, reliable, and maintainable applications.
Overview of ResearchKit Framework
ResearchKit is an open-source framework introduced by Apple that allows developers and researchers to create powerful apps for medical research. The framework provides a rich set of tools that make it easier to gather, analyze, and visualize health data. It is designed to work seamlessly with Swift, making it a robust choice for building research applications.
At its core, ResearchKit is designed to handle three main tasks:
- Data Collection: Through a variety of customizable modules, ResearchKit enables the collection of different types of data, including surveys, consent forms, and active tasks that require user interaction.
- Data Management: ResearchKit simplifies the process of managing the collected data, ensuring privacy and compliance with regulatory standards.
- Participant Recruitment: ResearchKit can help researchers reach a wider audience by allowing them to recruit study participants directly through their app.
One of the key components of ResearchKit is the Consent Module. This module provides a standardized way to obtain informed consent from study participants. Developers can customize the consent document to include all necessary information and then use a visual consent flow to guide users through the process. Here’s an example of how you might set up a consent document in Swift:
let consentDocument = ORKConsentDocument() consentDocument.title = "Example Consent" let sectionTypes: [ORKConsentSectionType] = [ .overview, .dataGathering, .privacy, .dataUse, .timeCommitment, .studySurvey, .studyTasks, .withdrawal ] let consentSections: [ORKConsentSection] = sectionTypes.map { contentSectionType in let section = ORKConsentSection(type: contentSectionType) section.summary = "If you wish to complete this study..." section.content = "In this study you will be asked..." return section } consentDocument.sections = consentSections
Another important module is the Survey Module, which allows developers to create detailed surveys using a variety of question types. Surveys can include multiple-choice questions, sliders, and even more complex types like image capture or audio recording. Here’s an example of creating a simple survey question:
let questionStep = ORKQuestionStep(identifier: "MoodStep", title: "Mood Survey", question: "How are you feeling today?", answer: ORKAnswerFormat.choiceAnswerFormatWithStyle(.singleChoice, textChoices: [ ORKTextChoice(text: "Happy", value: 0 as NSCoding & NSCopying & NSObjectProtocol), ORKTextChoice(text: "Sad", value: 1 as NSCoding & NSCopying & NSObjectProtocol), ORKTextChoice(text: "Tired", value: 2 as NSCoding & NSCopying & NSObjectProtocol) ]))
ResearchKit also includes modules for active tasks, where users are asked to perform activities under semi-controlled conditions while iPhone sensors collect data. For example, a task could ask users to say “Ahh” into the microphone to test their vocal cord function, or walk a short distance to measure their gait and balance.
ResearchKit’s flexibility and integration with Swift make it a powerful tool for medical researchers and developers alike. By leveraging the capabilities of ResearchKit in Swift applications, complex research tasks can be simplified and made accessible to a broader audience.
Whether you’re looking to conduct a medical study or simply gather health-related data from users, ResearchKit combined with Swift offers a robust framework that can help you achieve your goals efficiently and effectively.
Benefits of Using Swift with ResearchKit
Swift’s compatibility with ResearchKit brings a high number of advantages to developers and researchers alike. The synergy between the two provides a streamlined process for creating apps that can have a real impact on medical research and patient care. Here are some of the benefits of using Swift with ResearchKit:
- Seamless Integration: Swift and ResearchKit are designed to work together seamlessly. This integration allows for easier implementation of ResearchKit’s features, such as surveys and consent forms, directly into Swift applications.
- Increased Productivity: Swift’s clean syntax and modern features like optionals and closures make coding more efficient, which in turn speeds up the development process. This is particularly beneficial when dealing with the complex logic often required in research apps.
- Better Performance: Swift’s focus on performance means that apps built with ResearchKit will run smoothly, even when handling large amounts of data or complex tasks.
- Enhanced User Experience: Swift’s capabilities allow developers to create intuitive and visually appealing interfaces for research apps. A good user experience is important for encouraging participation in medical studies.
- Stronger Data Security: With Swift’s strong typing and error handling, apps built with ResearchKit are less likely to encounter crashes or security issues, ensuring that sensitive medical data is protected.
Here’s an example of how Swift’s contemporary syntax can simplify the creation of a ResearchKit survey task:
// Create a simple instruction step let instructionStep = ORKInstructionStep(identifier: "IntroStep") instructionStep.title = "Welcome to the Study" instructionStep.text = "This study will help us understand how people feel." // Create a question step let ageQuestionStep = ORKQuestionStep(identifier: "AgeStep", title: "Age", question: "How old are you?", answer: ORKAnswerFormat.integerAnswerFormat(withUnit: "years")) // Create an ordered task with both steps let surveyTask = ORKOrderedTask(identifier: "SurveyTask", steps: [instructionStep, ageQuestionStep])
The above code demonstrates how a simple survey can be constructed using Swift’s concise syntax, making the code easy to read and maintain. Additionally, the integration between Swift and ResearchKit allows developers to build powerful research tools without having to deal with the complexities of data collection and user management.
Overall, the combination of Swift’s contemporary language features and ResearchKit’s specialized modules provides a potent toolkit for creating apps that can facilitate medical research and ultimately improve patient outcomes.
Case Studies: Successful Applications of Swift and ResearchKit
One of the most successful applications of Swift and ResearchKit is the mPower app, which was developed to study Parkinson’s disease. The app uses a variety of active tasks to measure symptoms such as tremor, balance, and gait. Here is how an active task for measuring tremor can be set up in Swift:
let tremorTask = ORKOrderedTask.tremorTest(withIdentifier: "TremorTask", intendedUseDescription: nil, activeStepDuration: 10, activeTaskOptions: .excludeAll, handOptions: .both, options: ORKPredefinedTaskOption())
Another example is the MyHeart Counts app, which aims to collect cardiovascular data from participants. The app includes surveys and tasks that require users to perform exercises while their heart rate is monitored. Here’s how you can create a heart rate measurement task:
let heartRateTask = ORKOrderedTask.heartRateMeasurement(withIdentifier: "HeartRateTask", intendedUseDescription: "Measure your heart rate", heartRateSensorLocation: .chest, duration: 60, recordingSettings: nil, options: [])
These case studies demonstrate the potential of combining Swift with ResearchKit to create apps that not only advance medical research but also engage users in their health management. By leveraging the power of both technologies, developers have been able to create apps that are both powerful and simple to operate.
- mPower App: A Parkinson’s disease study app that utilizes active tasks designed to measure symptoms.
- MyHeart Counts App: A cardiovascular health app that collects data through user exercises and surveys.
These applications showcase the successful integration of Swift and ResearchKit in real-world scenarios, providing valuable insights into how technology can be used to support medical research and patient care.
Future Developments and Possibilities for Swift and ResearchKit
The future of Swift and ResearchKit holds immense potential for the medical research community and app developers. With continuous updates to Swift and the expansion of ResearchKit’s capabilities, there are several exciting possibilities on the horizon.
Integration with Machine Learning: Swift’s compatibility with machine learning frameworks like CoreML can enhance ResearchKit apps by providing advanced data analysis and pattern recognition. This could lead to more accurate study results and personalized healthcare recommendations.
Improved Data Visualization: Future developments in Swift’s graphical capabilities could allow for more sophisticated data visualization within ResearchKit apps. Interactive charts and real-time data updates could provide researchers and participants with better insights into study findings.
Broader Participant Reach: As Swift and ResearchKit apps become more prevalent, there’s potential to reach a wider demographic of participants. This could lead to more diverse and comprehensive study results.
Enhanced Security Features: Protecting sensitive health data is paramount. Future versions of Swift may introduce new security features that can be leveraged in ResearchKit to ensure participant privacy and data integrity.
Here’s an example of how machine learning could be integrated into a ResearchKit app using Swift:
import CoreML // Load the machine learning model let model = try? VNCoreMLModel(for: MyHealthClassifier().model) // Create a CoreML request let request = VNCoreMLRequest(model: model!) { request, error in guard let results = request.results as? [VNClassificationObservation] else { return } // Handle the results of the machine learning model } // Perform the machine learning request on some data let handler = VNImageRequestHandler(ciImage: ciImage, options: [:]) try? handler.perform([request])
Wearable Integration: With the growing popularity of wearable technology, ResearchKit apps could be enhanced to collect health data from devices like the Apple Watch. This would provide researchers with a more comprehensive view of participants’ health.
As we look ahead, the fusion of Swift’s evolution and ResearchKit’s growth promises to unlock new frontiers in medical research and healthcare app development. The possibilities are vast, and the impact on society could be profound.
- Machine Learning for predictive analytics in health studies
- Advanced data visualization tools for researchers and participants
- Expanded reach for participant recruitment through app visibility
- New security measures to safeguard sensitive health information
- Seamless integration with wearable technology for continuous health monitoring
The future is bright for Swift and ResearchKit, and we can expect to see groundbreaking apps that push the boundaries of what’s possible in medical research and patient care.
Source: https://www.plcourses.com/swift-and-researchkit/