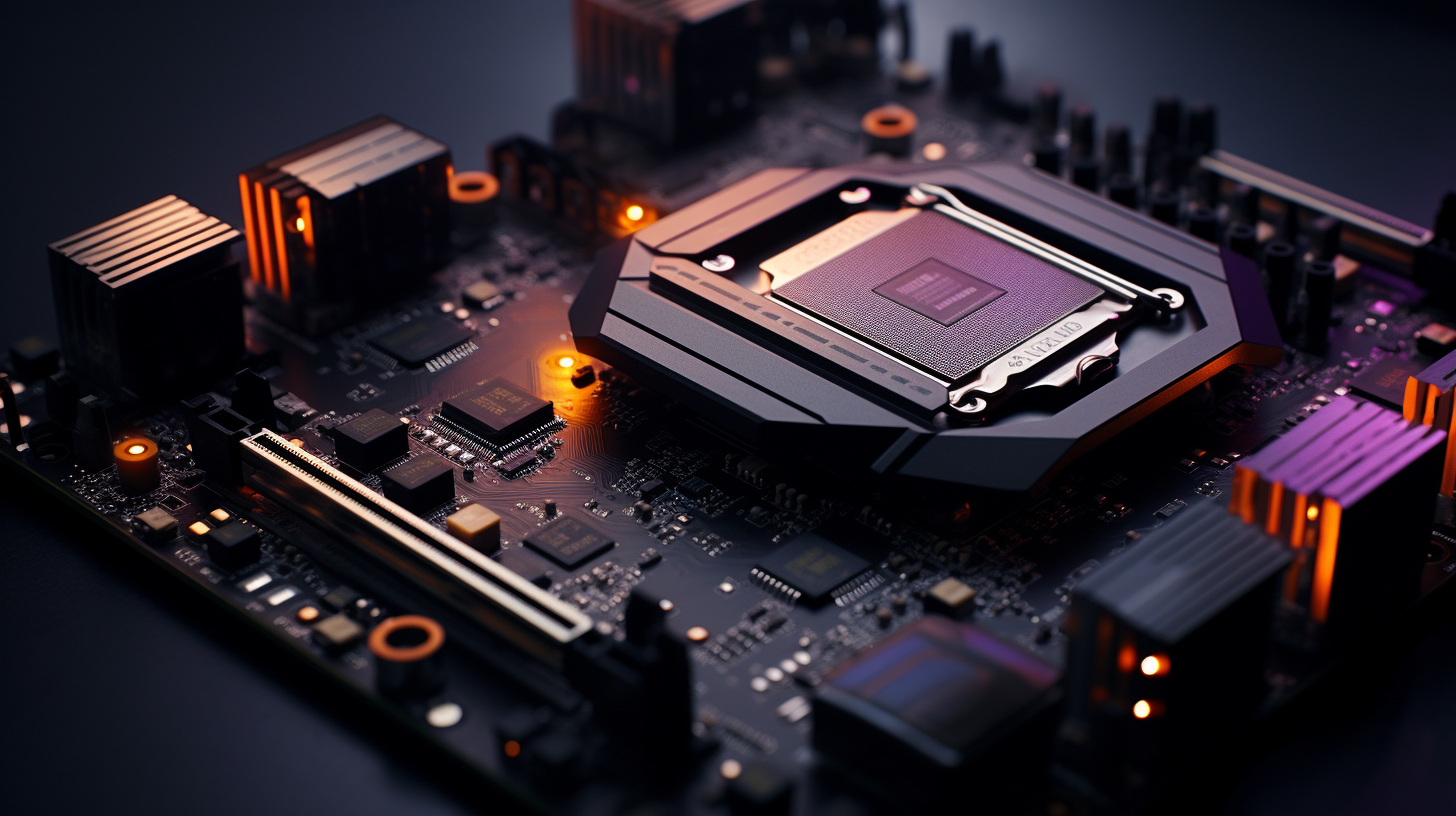
Understanding GraphQL and its Benefits
GraphQL is a query language for APIs that was developed by Facebook in 2012 and open-sourced in 2015. It provides a more efficient, powerful, and flexible alternative to the traditional REST API. GraphQL allows clients to request only the data they need, reducing the amount of data transferred over the network and improving performance.
One of the key benefits of GraphQL is its ability to fetch multiple resources in a single request. With traditional REST APIs, you would need to make multiple requests to different endpoints to retrieve related data. With GraphQL, you can retrieve all the related data you need in a single query.
{ user(id: "1") { name email posts { title content } } }
This query would return the name and email of the user with the ID of 1, as well as the titles and contents of all their posts.
Another benefit of GraphQL is its strong type system. GraphQL APIs are defined by a schema that specifies the types of data that can be queried. This means that you can validate queries against the schema at build time, catching errors before they happen at runtime.
type User { id: ID! name: String! email: String! posts: [Post!]! } type Post { id: ID! title: String! content: String! }
This schema defines a User type with fields for id, name, email, and posts, as well as a Post type with fields for id, title, and content. The exclamation marks indicate that these fields are non-nullable.
GraphQL also provides a powerful introspection system, which allows clients to discover the schema and capabilities of the API. This makes it easier to build tools and integrations for GraphQL APIs.
Using GraphQL can greatly simplify the process of building and consuming APIs, providing benefits in terms of performance, flexibility, and developer experience.
Setting Up a PHP Development Environment
Before we dive into implementing GraphQL in PHP, it’s important to set up a proper PHP development environment. This involves installing PHP, a web server like Apache or Nginx, and optionally a database such as MySQL or PostgreSQL. Additionally, you might need Composer, the dependency manager for PHP, to manage libraries and dependencies.
First, if you haven’t already installed PHP on your system, you can download it from the official PHP website or use a package manager like apt for Ubuntu or Homebrew for macOS. Ensure that you install PHP 7 or higher to take advantage of the latest features and improvements.
sudo apt-get install php
Next, set up a web server. For simplicity, we’ll use Apache in this example. You can install Apache using your system’s package manager:
sudo apt-get install apache2
After installing Apache, you can start the server and ensure it’s running by checking the status:
sudo systemctl start apache2 sudo systemctl status apache2
If you plan to interact with a database, install MySQL or PostgreSQL. For MySQL:
sudo apt-get install mysql-server
Composer is essential for managing PHP packages. Install it by following the instructions on the Composer website or using the command below:
php -r "copy('https://getcomposer.org/installer', 'composer-setup.php');" php -r "if (hash_file('sha384', 'composer-setup.php') === 'a5c698ffe4b8e382eeb5f17a7c9c4d3b53b4ac8e3b5899fcb1a4cbe8e1dde78e') { echo 'Installer verified'; } else { echo 'Installer corrupt'; unlink('composer-setup.php'); } echo PHP_EOL;" php composer-setup.php php -r "unlink('composer-setup.php');"
With PHP, a web server, and Composer installed, you’re now ready to begin developing with PHP and GraphQL. In the next section, we’ll go through how to implement GraphQL in your PHP applications.
Implementing GraphQL in PHP
Implementing GraphQL in a PHP application involves several steps, including setting up a GraphQL server, defining a schema, and creating resolver functions. Let’s get started.
First, you need to install a GraphQL PHP library. We’ll use webonyx/graphql-php in this example. Install it using Composer:
composer require webonyx/graphql-php
Next, create a schema. As mentioned earlier, a schema defines the types and relationships in your API. Here’s a basic example:
use GraphQLTypeDefinitionObjectType; use GraphQLTypeDefinitionType; use GraphQLTypeSchema; $queryType = new ObjectType([ 'name' => 'Query', 'fields' => [ 'echo' => [ 'type' => Type::string(), 'args' => [ 'message' => ['type' => Type::string()], ], 'resolve' => function ($root, $args) { return $root['prefix'] . $args['message']; } ], ], ]); $schema = new Schema([ 'query' => $queryType ]);
This defines a simple schema with a single query type that echoes back a message. The resolve
function is where you define how to fetch the data.
Now, set up the GraphQL server to handle requests. Create an endpoint, e.g., /graphql
, in your application where you will process GraphQL requests:
use GraphQLGraphQL; use GraphQLTypeSchema; use GraphQLErrorFormattedError; use GraphQLUtilsBuildSchema; try { $rawInput = file_get_contents('php://input'); $input = json_decode($rawInput, true); $query = $input['query']; $variableValues = isset($input['variables']) ? $input['variables'] : null; $rootValue = ['prefix' => 'You said: ']; $result = GraphQL::executeQuery($schema, $query, $rootValue, null, $variableValues); $output = $result->toArray(); } catch (Exception $e) { $output = [ 'errors' => [FormattedError::createFromException($e)] ]; } header('Content-Type: application/json'); echo json_encode($output);
Here we’re handling a POST request containing a JSON body with the GraphQL query. The executeQuery
function processes the query against the defined schema and returns the result.
With these steps, you have implemented a basic GraphQL server in PHP. You can now extend the schema with more types and queries, and implement more complex resolvers to handle database interactions or other business logic.
Remember to secure your endpoint appropriately and handle errors and exceptions as needed. With this setup, you’re ready to build more complex APIs using PHP and GraphQL.
Building APIs with PHP and GraphQL
Building APIs with PHP and GraphQL is a straightforward process once you have your development environment set up and a basic understanding of GraphQL concepts. In this section, we will delve into creating a more complex API that includes multiple types, queries, and mutations.
First, let’s expand our schema to include more types. For example, we might want to add a User type and a Post type:
use GraphQLTypeDefinitionObjectType; use GraphQLTypeDefinitionType; use GraphQLTypeSchema; $postType = new ObjectType([ 'name' => 'Post', 'fields' => [ 'id' => ['type' => Type::nonNull(Type::id())], 'title' => ['type' => Type::string()], 'content' => ['type' => Type::string()], ], ]); $userType = new ObjectType([ 'name' => 'User', 'fields' => [ 'id' => ['type' => Type::nonNull(Type::id())], 'name' => ['type' => Type::string()], 'email' => ['type' => Type::string()], 'posts' => [ 'type' => Type::listOf($postType), 'resolve' => function ($user) { // Fetch posts from a data source using the user ID return getPostsByUserId($user['id']); } ], ], ]); $queryType = new ObjectType([ 'name' => 'Query', 'fields' => [ 'user' => [ 'type' => $userType, 'args' => [ 'id' => ['type' => Type::nonNull(Type::id())], ], 'resolve' => function ($root, $args) { // Fetch user from a data source using the ID return getUserById($args['id']); } ], ], ]); $schema = new Schema([ 'query' => $queryType, ]);
In this example, we’ve defined two new types: Post and User. Each user has a list of posts, which we can fetch using a resolver function. The Query type now includes a user field that takes an ID argument and returns a user object.
Next, let’s add mutations to our API to allow clients to create new users and posts:
$mutationType = new ObjectType([ 'name' => 'Mutation', 'fields' => [ 'createUser' => [ 'type' => $userType, 'args' => [ 'name' => ['type' => Type::nonNull(Type::string())], 'email' => ['type' => Type::nonNull(Type::string())], ], 'resolve' => function ($root, $args) { // Create a new user and return it return createUser($args['name'], $args['email']); } ], 'createPost' => [ 'type' => $postType, 'args' => [ 'userId' => ['type' => Type::nonNull(Type::id())], 'title' => ['type' => Type::string()], 'content' => ['type' => Type::string()], ], 'resolve' => function ($root, $args) { // Create a new post and return it return createPost($args['userId'], $args['title'], $args['content']); } ], ], ]); $schema = new Schema([ 'query' => $queryType, 'mutation' => $mutationType, ]);
Here we’ve added a Mutation type with two fields: createUser and createPost. Each mutation takes arguments needed to create the respective entities and returns the newly created object.
To handle these mutations, we would need to implement the createUser
and createPost
functions, which would interact with our data source to persist the new entities.
With these additional types and mutations, our PHP GraphQL API is becoming more powerful and capable of handling real-world scenarios. Clients can now query for users and their posts, as well as create new users and posts through mutations.
Remember to test your API thoroughly and handle any edge cases or errors that may occur. This will ensure a robust and reliable API for your clients to use.
In the next section, we’ll discuss how to test and debug your PHP GraphQL APIs to ensure they are functioning correctly.
Testing and Debugging PHP GraphQL APIs
Testing and debugging are critical steps in the development of any API, including those built with PHP and GraphQL. It’s important to ensure that your API is returning the correct data and handling errors gracefully. In this section, we’ll explore some strategies and tools for testing and debugging your PHP GraphQL APIs.
Unit Testing
- Create unit tests for your resolver functions to ensure they return the expected data.
- Mock the database or other external services to test your resolvers in isolation.
- Use testing frameworks like PHPUnit to automate your tests and check for regressions.
// Example of a unit test for a resolver function public function testUserResolverReturnsCorrectData() { $userResolver = new UserResolver(); $expectedUser = ['id' => '1', 'name' => 'Mitch Carter', 'email' => '[email protected]']; $actualUser = $userResolver->resolve(null, ['id' => '1']); $this->assertEquals($expectedUser, $actualUser); }
Integration Testing
- Test the entire request-response cycle, from receiving a GraphQL query to returning the response.
- Ensure that your API handles queries and mutations correctly with all their associated arguments.
// Example of an integration test for a GraphQL query public function testUserQueryReturnsCorrectResponse() { $query = '{ user(id: "1") { name email } }'; $expectedResponse = ['data' => ['user' => ['name' => 'Mitch Carter', 'email' => '[email protected]']]]; $actualResponse = $this->graphqlServer->executeQuery($query, null); $this->assertEquals($expectedResponse, $actualResponse); }
Debugging Tools
- Use tools like GraphiQL or GraphQL Playground to manually test and debug queries and mutations.
- Inspect the schema and try out different queries interactively.
- Check the error messages and stack traces for clues when something goes wrong.
Error Handling
- Implement error handling in your API to return meaningful error messages to clients.
- Log errors on the server side for further investigation.
// Example of error handling in a GraphQL endpoint try { // ... execute query ... } catch (Exception $e) { $error = FormattedError::createFromException($e); $output = ['errors' => [$error]]; // Optionally log the error here } echo json_encode($output);
Effective testing and debugging practices are essential to building robust PHP GraphQL APIs. By writing thorough tests and using the right tools, you can catch issues early and ensure that your API is reliable and uncomplicated to manage. Always strive to provide clear error messages and document your API well to help clients understand how to use it properly.
Source: https://www.plcourses.com/php-and-graphql-building-apis/