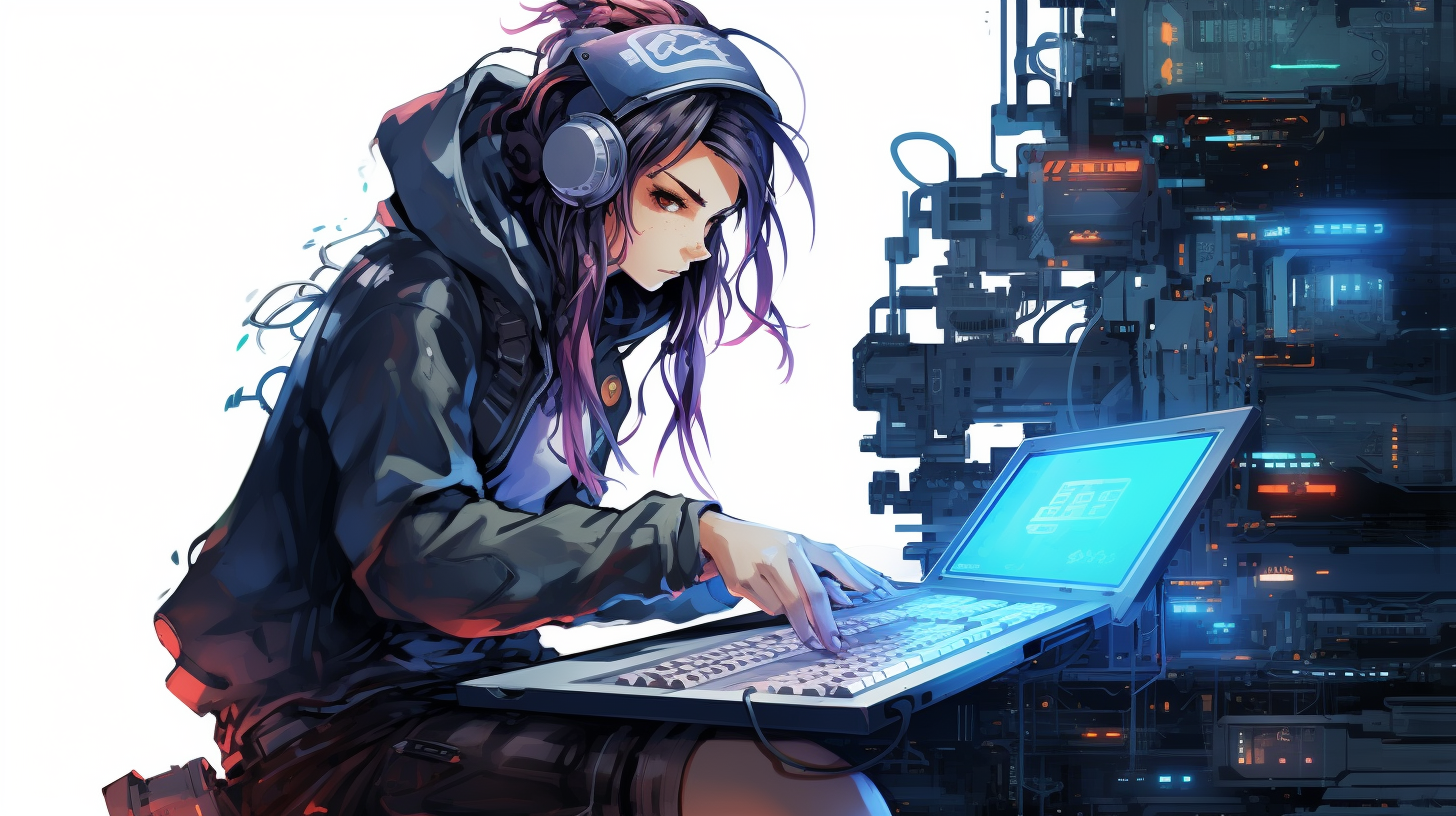
Introduction to Event-Driven Architecture
Event-Driven Architecture (EDA) is a design paradigm orchestrated around the production, detection, consumption, and reaction to events. An event can be defined as a significant change in state or an occurrence that’s identified by software and may trigger further processing.
In EDA, components, known as event producers, generate events and publish them to a channel or a mediator, such as an event bus or a message queue. Event consumers, on the other hand, subscribe to these channels and react to the events by executing specific logic or triggering additional processes. The decoupling of producers and consumers is one of the key characteristics of this architecture, providing flexibility and scalability.
Events in an EDA are typically lightweight and contain the necessary information for consumers to process them. They can be categorized into different types, such as:
- Domain events: Reflect a change within the business domain, for example, an order being placed.
- System events: Indicate a change in the system’s state, like a server starting up.
- Time-based events: Triggered by the passage of time or scheduled occurrences.
One common approach to handling events in Java is using the Observer pattern. Here’s a simple Java code example:
public interface EventListener { void onEvent(Event e); } public class EventProducer { private List listeners = new ArrayList<>(); public void addListener(EventListener listener) { listeners.add(listener); } public void produceEvent(Event e) { listeners.forEach(listener -> listener.onEvent(e)); } } public class EventConsumer implements EventListener { @Override public void onEvent(Event e) { // Handle event } }
This code illustrates a basic producer-consumer setup where EventProducer
notifies all registered EventConsumer
s about an event occurrence. The consumers implement an EventListener
interface, allowing them to react accordingly when an event is produced.
Note: The actual event processing logic would depend on the application’s requirements and the type of event being handled.
EDA enables systems to be more responsive and capable of handling asynchronous operations effectively. It’s particularly well-suited for distributed systems and microservices where different components operate independently but need to communicate and synchronize their state changes efficiently.
Key Concepts and Principles of Event-Driven Architecture
In an Event-Driven Architecture, understanding the key concepts and principles is fundamental to designing and implementing a system that’s both responsive and scalable. Here are some of the core principles:
- Decoupling: Producers and consumers operate independently of each other. This separation allows for the system components to be evolved, scaled, and maintained without affecting other parts of the system.
- Asynchrony: Events are produced and consumed asynchronously, meaning the producer does not wait for the consumer to process an event. This non-blocking behavior leads to more efficient use of resources.
- Scalability: Since components are decoupled, they can be scaled independently to meet demand without impacting the entire system.
- Reusability: Components can be reused across different parts of the system or in different applications altogether, as they do not have hard dependencies on other components.
- Resilience: The system is more resilient to failures as the components are isolated, making it easier to identify and handle failures in a localized manner.
Apart from these principles, there are also some key concepts that need to be understood:
- Events: These are the core of an EDA. An event represents a state change or an occurrence in the system. It’s important that events are granular and contain all necessary information for consumers to act upon them.
- Event Channels: These are the mediums through which events are communicated between producers and consumers. Examples include message queues or event streams.
- Event Processing: This refers to how events are handled once received. This could involve simple actions like logging, complex operations like data transformations, or triggering other events.
Let’s look at a Java code example that demonstrates these concepts:
import java.util.concurrent.BlockingQueue; import java.util.concurrent.LinkedBlockingQueue; public class EventChannel { private final BlockingQueue queue = new LinkedBlockingQueue<>(); public void publish(Event event) { queue.add(event); } public Event take() throws InterruptedException { return queue.take(); } } public class EventProcessor implements Runnable { private final EventChannel channel; public EventProcessor(EventChannel channel) { this.channel = channel; } @Override public void run() { while (true) { try { Event event = channel.take(); // Process event } catch (InterruptedException e) { Thread.currentThread().interrupt(); break; } } } }
In this example, EventChannel
acts as an event channel using a BlockingQueue
to hold events. The EventProcessor
is a consumer that processes events by taking them off the queue. This setup allows for asynchronous communication between event producers and consumers, aligning with the principles of EDA.
Note: The code examples provided are intended to illustrate the concepts and are simplified for readability. In a real-world scenario, additional considerations such as error handling and concurrency control would be necessary.
Implementing Event-Driven Architecture in Java
Implementing an Event-Driven Architecture in Java involves several steps and considerations to ensure that the system is designed for effective event handling and processing. In this section, we’ll go through the steps necessary to set up an EDA in a Java application.
Firstly, you need to define the events that your system will handle. Events should be immutable objects that encapsulate all the necessary information for the event’s processing. Here’s an example of a simple event class:
public class OrderEvent { private final String orderId; private final String orderStatus; public OrderEvent(String orderId, String orderStatus) { this.orderId = orderId; this.orderStatus = orderStatus; } // Getters }
Next, you need to create event producers. These are the components responsible for publishing events to the event channel. In Java, this can be done by creating a method that constructs the event and then sends it to the event channel:
public class OrderService { private final EventChannel eventChannel; public OrderService(EventChannel eventChannel) { this.eventChannel = eventChannel; } public void createOrder(String orderId) { // Order creation logic OrderEvent orderCreatedEvent = new OrderEvent(orderId, "CREATED"); eventChannel.publish(orderCreatedEvent); } }
For the event consumers, you will need to implement logic that subscribes to the event channel and processes events. In Java, this could be done by creating a class that implements Runnable
and continuously listens for new events:
public class OrderEventListener implements Runnable { private final EventChannel eventChannel; public OrderEventListener(EventChannel eventChannel) { this.eventChannel = eventChannel; } @Override public void run() { while (!Thread.currentThread().isInterrupted()) { try { OrderEvent event = (OrderEvent) eventChannel.take(); // Event processing logic } catch (InterruptedException e) { Thread.currentThread().interrupt(); } } } }
You will also need to manage the lifecycle of these consumer threads, ensuring they start and stop appropriately with your application.
Source: https://www.plcourses.com/java-and-event-driven-architecture/