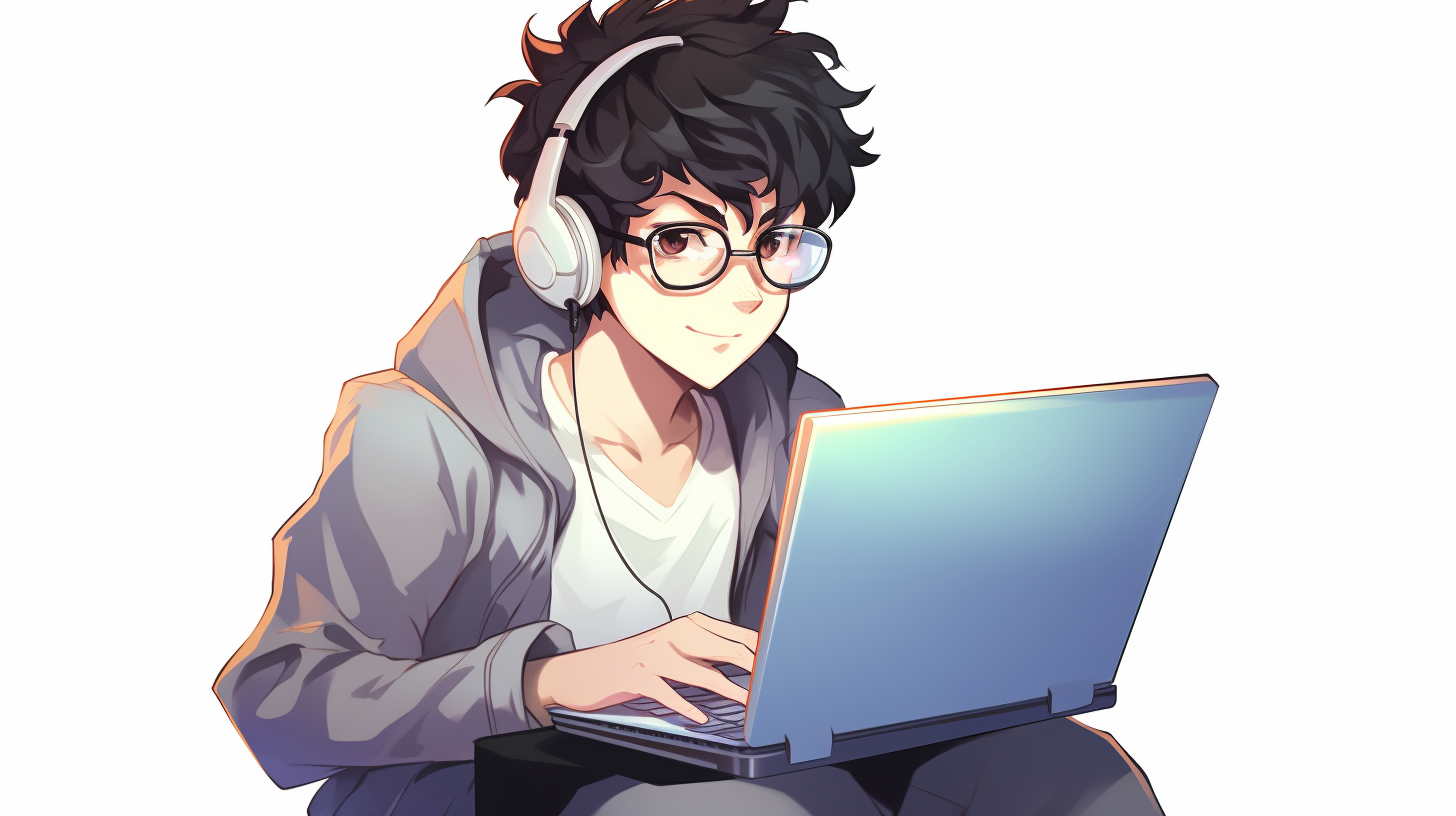
When working with JSON in Python, you may sometimes encounter objects that are not natively serializable into JSON format. That is where the JSONEncoder subclass comes into play. The json
module in Python provides a class called JSONEncoder
that can be subclassed to support the encoding of complex objects into JSON.
The JSONEncoder
class has a method default
which can be overridden to implement custom serialization behavior. When the json.dumps()
or json.dump()
functions encounter an object that is not natively serializable, they call the default
method of the encoder.
import json class MyEncoder(json.JSONEncoder): def default(self, obj): # Implement custom serialization logic here pass
This approach allows developers to extend the default JSON encoding to support a wide variety of complex objects by providing specific serialization logic for those objects. For example, if you want to serialize a Python datetime object, which is not natively supported by the json
module, you can do so by customizing the default
method:
from datetime import datetime class DateTimeEncoder(json.JSONEncoder): def default(self, obj): if isinstance(obj, datetime): return obj.isoformat() return super().default(obj) now = datetime.now() json_string = json.dumps(now, cls=DateTimeEncoder) print(json_string) # Output will be the ISO formatted datetime string
The above code snippet demonstrates how a custom JSONEncoder
subclass can be created to handle the serialization of datetime objects into a JSON-friendly format. By using this technique, developers can effectively manage the conversion of complex objects to JSON, ensuring smooth data interchange between systems and applications.
Customizing JSON encoding for complex objects
Another example of customizing JSON encoding for complex objects is when dealing with custom Python objects. Imagine you have a class Person
with attributes name
and age
, and you want to convert instances of this class to JSON. You can create a subclass of JSONEncoder
that knows how to handle Person
objects:
class Person: def __init__(self, name, age): self.name = name self.age = age class PersonEncoder(json.JSONEncoder): def default(self, obj): if isinstance(obj, Person): return {'name': obj.name, 'age': obj.age} return super().default(obj) person = Person("Alice", 30) json_string = json.dumps(person, cls=PersonEncoder) print(json_string) # Output will be a JSON string representing the person
This custom encoder converts Person
instances into dictionaries before serialization, in a format that can be easily represented in JSON.
It’s also possible to handle more complex data structures such as lists or dictionaries containing instances of custom objects. For example, let’s say you have a list of Person
objects that you want to serialize:
people = [Person("Alice", 30), Person("Bob", 25), Person("Charlie", 35)] json_string = json.dumps(people, cls=PersonEncoder) print(json_string) # Output will be a JSON array of person objects
In this case, the default
method of PersonEncoder
will be called for each object in the list, converting them into serializable form before the list itself is serialized as a JSON array.
The ability to customize JSON encoding by subclassing JSONEncoder
provides a powerful tool for developers to handle serialization of complex objects in Python. Whether it’s a single object, a nested data structure, or a combination of different data types, the default
method can be tailored to meet the specific needs of the application.
Handling nested objects and data structures
Handling nested objects and data structures can be a bit more intricate. When dealing with nested objects, each level of the object needs to be able to be serialized into JSON format. This requires recursive serialization logic in our custom JSONEncoder subclass. Think the following example where we have a class Family that contains a list of Person objects:
class Family: def __init__(self, members): self.members = members class FamilyEncoder(json.JSONEncoder): def default(self, obj): if isinstance(obj, Family): return {'members': json.dumps(obj.members, cls=PersonEncoder)} elif isinstance(obj, Person): return {'name': obj.name, 'age': obj.age} return super().default(obj) family = Family([Person("Alice", 30), Person("Bob", 25), Person("Charlie", 35)]) json_string = json.dumps(family, cls=FamilyEncoder) print(json_string) # Output will be a JSON string with nested person objects
In the above code, FamilyEncoder
handles instances of Family by recursively calling json.dumps
on its members with the appropriate PersonEncoder. This ensures that the nested Person objects are also serialized correctly.
Complex nested data structures such as dictionaries containing lists of custom objects or vice versa can also be managed with a similar approach. For instance:
data_structure = { 'family1': Family([Person("Alice", 30), Person("Bob", 25)]), 'family2': Family([Person("Charlie", 35), Person("Dave", 40)]) } json_string = json.dumps(data_structure, cls=FamilyEncoder) print(json_string) # Output will be a JSON string with nested family and person objects
This demonstrates the versatility and power of subclassing JSONEncoder
. By carefully implementing the default
method, we can serialize even the most complex of objects and data structures into JSON format. As always, it is important to ensure that each custom object type is checked and handled accordingly to prevent any serialization errors.
Advanced techniques for complex object serialization
Advanced techniques for complex object serialization go beyond simple custom objects and nested data structures. They involve additional strategies to manage serialization of objects that have more intricate relationships or metadata.
One such technique is to use a custom marker or identifier to tag complex objects during serialization. This can be useful for objects that have a reference to themselves (recursive data structures) or when you have objects that reference each other (graph-like structures).
class Node: def __init__(self, value, children=None): self.value = value self.children = children if children is not None else [] class NodeEncoder(json.JSONEncoder): def default(self, obj): if isinstance(obj, Node): return {'__type__':'Node', 'value': obj.value, 'children': obj.children} return super().default(obj)
In the example above, we’ve added a special __type__
key to the serialized dictionary to indicate that the object is of type Node. This can be useful when deserializing, as we can check for this marker and reconstruct the original object structure accordingly.
Another advanced technique involves handling serialization of objects that contain non-serializable attributes, like file handles or database connections. For such cases, we might choose to only serialize a subset of the object’s state.
class DataSource: def __init__(self, name, connection): self.name = name self.connection = connection # A database connection that's not serializable def __getstate__(self): state = self.__dict__.copy() del state['connection'] # Remove the non-serializable entry return state class DataSourceEncoder(json.JSONEncoder): def default(self, obj): if isinstance(obj, DataSource): return obj.__getstate__() return super().default(obj)
The __getstate__
method is used here to return a dictionary of the object’s state without the non-serializable attributes. The JSONEncoder subclass then uses this method to obtain a serializable representation of the DataSource object.
Finally, it’s also possible to handle serialization of objects that require special initialization parameters that are not part of their state. For example, an object representing a connection pool may need a URL and credentials to instantiate, but these are not part of the pool’s state.
class ConnectionPool: def __init__(self, url, credentials): self.pool = initialize_pool(url, credentials) def __getstate__(self): # Only serialize the state necessary to recreate the pool return {'url': self.url, 'credentials': self.credentials} def __setstate__(self, state): # Use the state to recreate the pool upon deserialization self.__init__(state['url'], state['credentials'])
In this case, we implement both __getstate__
and __setstate__
methods to control what gets serialized and how the object is reconstructed during deserialization. Note that __setstate__
is not directly used by JSONEncoder but would be used by the corresponding deserialization logic.
These advanced techniques showcase the flexibility of JSONEncoder subclassing. By implementing custom serialization logic in the default
method and using additional methods like __getstate__
, we can effectively serialize complex objects with unique requirements or relationships. This allows for more comprehensive data interchange capabilities in Python applications.
Source: https://www.pythonlore.com/handling-complex-objects-with-jsonencoder-subclass/