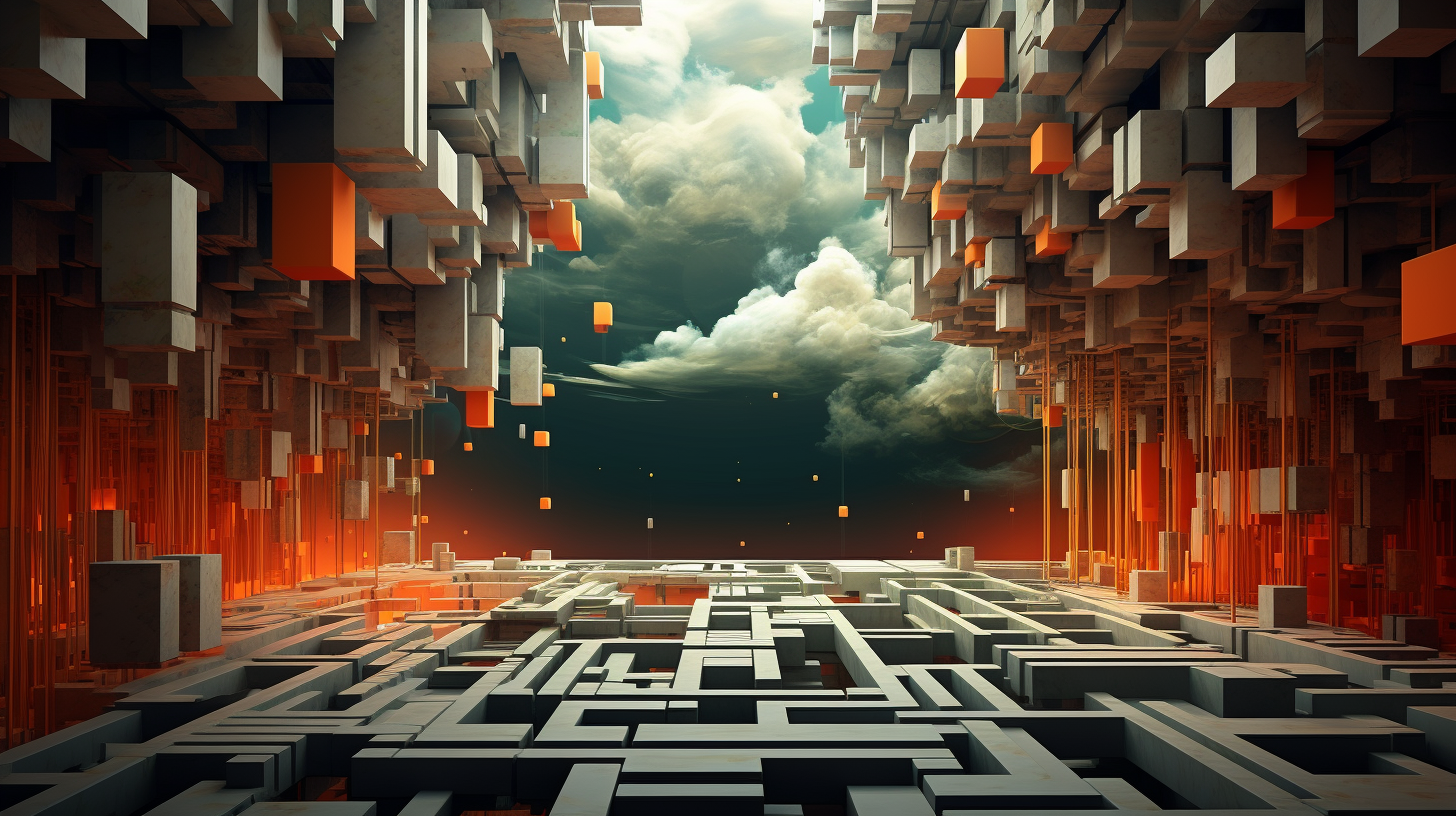
Introduction to Swift Programming Language
Swift is a powerful and intuitive programming language created by Apple for building apps for iOS, Mac, Apple TV, and Apple Watch. It’s designed to give developers more freedom than ever before. Swift is user-friendly and open source, so anyone with an concept can create something incredible.
Developers often praise Swift for its simplicity and performance. The language was built with the goal of being both easy to learn and capable of handling the most complex programming tasks. Its syntax encourages developers to write clean and consistent code which may lead to fewer mistakes.
Swift is a type-safe language which means the code helps you to be clear about the types of values your code can work with. If part of your code requires a String, type safety prevents you from passing it an Int by mistake. Likewise, type safety prevents you from accidentally passing an optional String to a piece of code that requires a non-optional String.
var myVariable = 42 myVariable = "Hello, World!" // Error: Type 'String' does not conform to expected type 'Int'
Swift also provides modern features developers love like closures, generics, and optionals. Closures are self-contained blocks of functionality that can be passed around and used in your code. Generics are one of the most powerful features of Swift, and much of the Swift standard library is built with generic code. Optionals signify that a value could be present or absent.
func printIfPossible(_ maybeString: Optional) { if let definitelyString = maybeString { print(definitelyString) } else { print("Nothing to see here") } }
Swift’s automatic reference counting (ARC) manages the memory usage in your app, so that you don’t have to, freeing you to concentrate on your app’s functionality rather than memory management.
Swift is a robust and intuitive programming language that empowers developers to create incredible apps with ease and confidence. Its safety features, combined with its contemporary syntax and rich set of capabilities, make it an essential tool for any developer working on Apple’s platforms.
Overview of CoreGraphics Framework
The CoreGraphics framework is a powerful, low-level API for rendering 2D graphics in apps on Apple’s platforms. It provides developers with the tools needed to draw shapes, images, and text directly to the screen or to off-screen surfaces before displaying them. This framework is an essential part of the broader Quartz 2D drawing engine and is used across iOS, macOS, watchOS, and tvOS.
At its core, CoreGraphics operates on a context-based drawing model. Developers define a CGContext to specify where the drawing operations should take place. This could be anything from the screen of the device to an off-screen bitmap. Once a context is established, you can draw lines, rectangles, circles, and other shapes using a variety of functions provided by the framework.
- Paths: Create complex shapes by combining lines and curves.
- Colors and Gradients: Fill shapes with solid colors or gradients.
- Images: Draw images within a graphics context.
- Text: Render text with custom fonts and styles.
- Transformations: Apply transformations such as translation, rotation, and scaling to alter the coordinate space of the drawing context.
One of the key benefits of CoreGraphics is its hardware-accelerated performance. When you use CoreGraphics, much of the heavy lifting is done by the GPU, which ensures smooth rendering even for complex scenes. Additionally, because CoreGraphics is a C-based API, it can be easily integrated with Swift code using bridging headers.
CoreGraphics is not just about drawing; it also provides functions for image data management, color management, and PDF document creation and display, making it an incredibly versatile tool for developers.
Here’s a simple example of how you might set up a custom view in Swift and use CoreGraphics to draw a red rectangle:
import UIKit class MyCustomView: UIView { override func draw(_ rect: CGRect) { guard let context = UIGraphicsGetCurrentContext() else { return } // Set the fill color to red context.setFillColor(UIColor.red.cgColor) // Create a rectangle with the same size as the view let rectangle = CGRect(x: 0, y: 0, width: self.frame.width, height: self.frame.height) // Fill the rectangle with the current fill color context.fill(rectangle) } }
The power of CoreGraphics lies in its flexibility and control. While higher-level frameworks like UIKit provide convenient ways to handle common graphics tasks, CoreGraphics gives you complete control over the rendering process. Whether you’re developing a game, a data visualization tool, or any app that requires custom graphics, CoreGraphics provides the building blocks you need to create stunning visual effects.
Utilizing CoreGraphics with Swift
Utilizing CoreGraphics with Swift can unlock the potential for creating custom and efficient graphics in your applications. The integration between Swift and CoreGraphics is seamless, allowing you to draw and manipulate graphics directly within your Swift code. Let’s dive into how you can leverage CoreGraphics in your Swift projects.
Drawing Paths: One of the most common tasks in CoreGraphics is drawing paths. A path can be a line, a circle, or even a complex shape made up of curves and lines. Here is an example of how to draw a simple line:
import UIKit class LineView: UIView { override func draw(_ rect: CGRect) { guard let context = UIGraphicsGetCurrentContext() else { return } // Start a new path context.beginPath() // Move to the starting point of the line context.move(to: CGPoint(x: 10, y: 10)) // Add a line segment to the path context.addLine(to: CGPoint(x: 100, y: 100)) // Set the stroke color to blue context.setStrokeColor(UIColor.blue.cgColor) // Set the line width context.setLineWidth(5) // Stroke the path context.strokePath() } }
Working with Colors and Gradients: CoreGraphics allows you to fill shapes with colors or gradients. Here’s how you can fill a rectangle with a gradient:
import UIKit class GradientView: UIView { override func draw(_ rect: CGRect) { guard let context = UIGraphicsGetCurrentContext() else { return } let colors = [UIColor.red.cgColor, UIColor.yellow.cgColor] as CFArray let colorSpace = CGColorSpaceCreateDeviceRGB() let gradient = CGGradient(colorsSpace: colorSpace, colors: colors, locations: [0.0, 1.0])! let startPoint = CGPoint(x: rect.midX, y: rect.minY) let endPoint = CGPoint(x: rect.midX, y: rect.maxY) context.drawLinearGradient(gradient, start: startPoint, end: endPoint, options: []) } }
Rendering Images: CoreGraphics also allows you to render images within a context. You can draw an image onto a view as follows:
import UIKit class ImageView: UIView { override func draw(_ rect: CGRect) { guard let context = UIGraphicsGetCurrentContext() else { return } guard let image = UIImage(named: "example.png")?.cgImage else { return } // Draw the image in the view's frame context.draw(image, in: rect) } }
Applying Transformations: Transformations such as translation, rotation, and scaling can be applied to alter the coordinate space of the drawing context. Here’s how to rotate a square:
import UIKit class RotatedSquareView: UIView { override func draw(_ rect: CGRect) { guard let context = UIGraphicsGetCurrentContext() else { return } // Move the origin of the context to the center of the view context.translateBy(x: rect.midX, y: rect.midY) // Rotate the context by 45 degrees context.rotate(by: CGFloat.pi / 4) // Move the origin back context.translateBy(x: -rect.midX, y: -rect.midY) // Draw a square with the transformed context let square = CGRect(x: rect.midX - 50, y: rect.midY - 50, width: 100, height: 100) context.setFillColor(UIColor.green.cgColor) context.fill(square) } }
By mastering these CoreGraphics techniques in Swift, you can create intricate and performant graphics that will enhance the visual appeal of your applications. Whether you need to draw custom shapes, work with images, or apply complex transformations, CoreGraphics offers the tools necessary for detailed and creative graphics work.
Source: https://www.plcourses.com/swift-and-coregraphics/