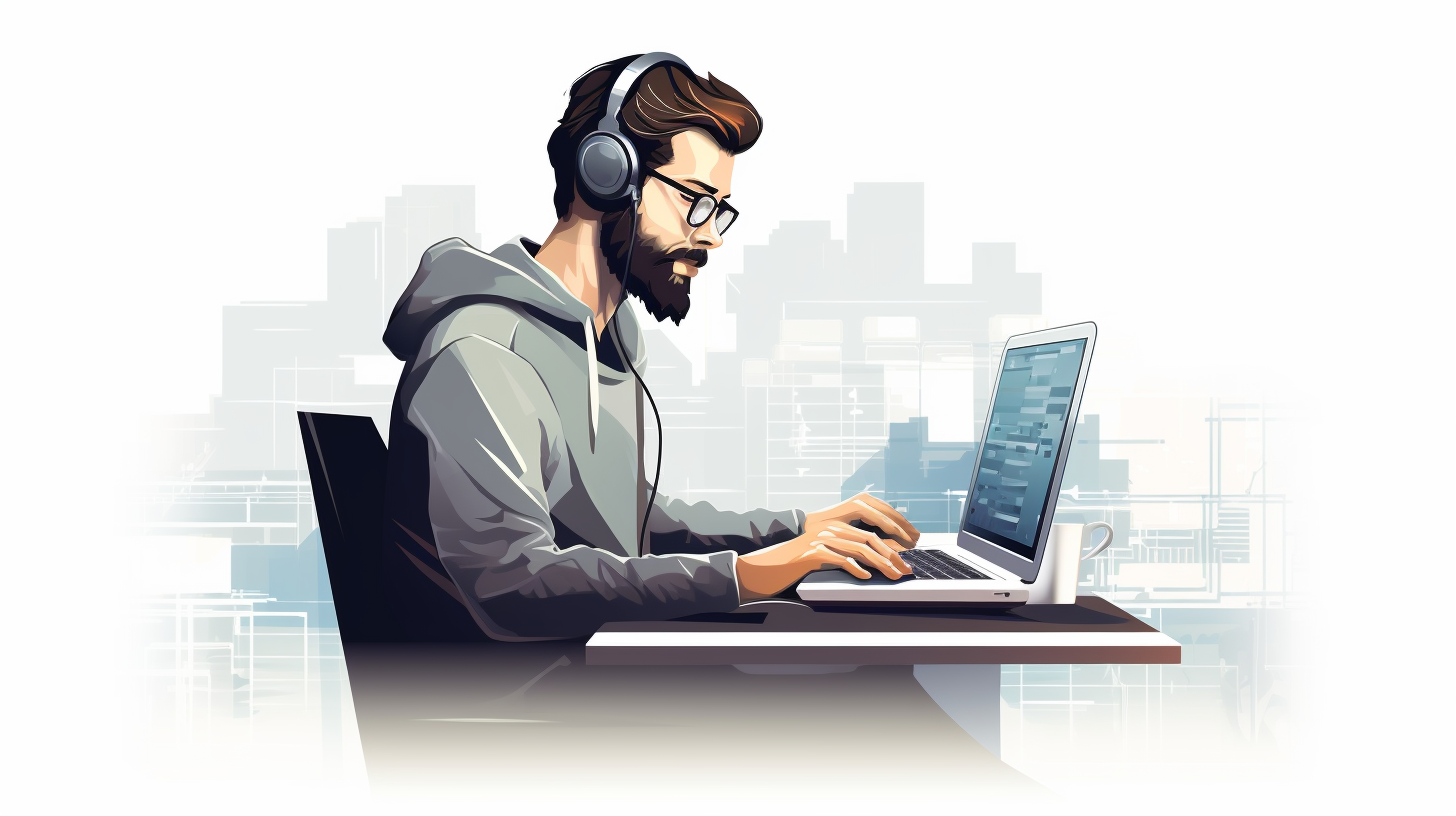
Introduction to Text Mining
Text mining, also known as text data mining or text analytics, is the process of deriving high-quality information from text. It involves the discovery by computer of new, previously unknown information, by automatically extracting information from different written resources. The goal is to turn text into data for analysis, via the application of natural language processing (NLP) and analytical methods.
A typical text mining process involves several steps such as information retrieval, natural language processing, information extraction, and data mining. These steps help in transforming unstructured text into structured data suitable for analysis. Text mining is widely used in various fields such as marketing, finance, healthcare, and social media to extract insights from large volumes of text data.
One of the key challenges in text mining is dealing with the vast amount of unstructured data. Unlike structured data, which is easy to analyze, unstructured text is complex and requires specialized tools and techniques for processing. Java, with its robust libraries and frameworks, provides an excellent platform for building text mining applications.
For example, ponder the following Java code snippet that uses the OpenNLP library for sentence detection:
InputStream inputStream = new FileInputStream("en-sent.bin"); SentenceModel model = new SentenceModel(inputStream); SentenceDetectorME detector = new SentenceDetectorME(model); String paragraph = "Text mining is an exciting field. It has many applications."; String sentences[] = detector.sentDetect(paragraph); for(String sentence : sentences) { System.out.println(sentence); }
This code loads a pre-trained sentence detection model and uses it to split a paragraph into individual sentences. Such a technique is a fundamental step in preprocessing text for further mining tasks.
Text mining can also involve more complex tasks such as sentiment analysis, topic modeling, and entity recognition. These tasks require sophisticated algorithms and machine learning techniques to accurately interpret the meaning and context of the text.
Text mining is a powerful tool for extracting valuable information from text data. With the aid of Java and its rich set of libraries, developers can build effective text mining solutions that can uncover hidden insights and support decision-making processes across various industries.
Overview of Java for Text Mining
Java is a versatile and powerful programming language that is widely used for building enterprise-level applications. Its strength lies in its robustness, platform independence, and a vast ecosystem of libraries and frameworks. When it comes to text mining, Java offers several advantages that make it a preferred choice for developers.
Firstly, Java provides a rich set of libraries for natural language processing and text analysis. Libraries such as Apache OpenNLP, Stanford NLP, and LingPipe offer pre-built models and algorithms for tasks like sentence detection, tokenization, part-of-speech tagging, and named entity recognition. These libraries simplify the process of text mining by providing developers with the tools they need to preprocess and analyze text data efficiently.
For instance, the following Java code demonstrates how to use the Stanford NLP library for tokenization:
import edu.stanford.nlp.simple.*; public class TokenizationExample { public static void main(String[] args) { Document doc = new Document("Text mining helps organizations make sense of unstructured data."); for (Sentence sent : doc.sentences()) { System.out.println(sent.words()); } } }
This code creates a new Document
object from a string of text and then iterates over each Sentence
to print out the list of words. It demonstrates the ease with which developers can tokenize text using Java.
Secondly, Java’s strong typing and object-oriented features make it easy to manage complex text mining projects. Developers can create modular, reusable code this is easier to maintain and scale. The ability to create custom classes and objects also allows for better organization of text mining workflows and data structures.
Moreover, Java’s performance is another key advantage. With Just-In-Time (JIT) compilation and optimization, Java applications can achieve high performance, which is important when processing large volumes of text data. The language’s multithreading capabilities also allow for concurrent processing, further enhancing performance.
Finally, Java’s active community and wealth of resources mean that developers have access to extensive documentation, forums, and support. This is invaluable when building complex text mining applications that may require troubleshooting and optimization.
Java provides a comprehensive environment for text mining with its powerful NLP libraries, strong typing and object-oriented features, performance optimization capabilities, and supportive community. All these factors contribute to Java being a top choice for developers looking to analyze and extract insights from text data.
Preprocessing Text Data in Java
Preprocessing is a critical step in text mining that involves preparing and cleaning text data before it can be analyzed. In Java, preprocessing can be achieved through various libraries and frameworks, each offering different functionalities to handle text data effectively.
One of the key preprocessing tasks is tokenization, which is the process of breaking down a stream of text into words, phrases, symbols, or other meaningful elements called tokens. The following Java code uses the Apache OpenNLP library to perform tokenization:
InputStream inputStream = new FileInputStream("en-token.bin"); TokenizerModel model = new TokenizerModel(inputStream); Tokenizer tokenizer = new TokenizerME(model); String[] tokens = tokenizer.tokenize("Text mining is an exciting field with many applications."); for (String token : tokens) { System.out.println(token); }
Another important preprocessing step is stemming, which involves reducing words to their root form. This helps in consolidating different forms of a word into a single representation. The following code snippet demonstrates stemming using the Snowball Stemmer library:
SnowballStemmer stemmer = new englishStemmer(); String[] words = {"mining", "mined", "miner"}; for (String word : words) { stemmer.setCurrent(word); stemmer.stem(); System.out.println(stemmer.getCurrent()); }
Stop word removal is another preprocessing technique where commonly used words that do not contribute much meaning to a sentence are removed. Here’s how you can remove stop words using the Lucene library:
CharArraySet stopWords = StopAnalyzer.ENGLISH_STOP_WORDS_SET; TokenStream tokenStream = new StandardTokenizer(); tokenStream = new StopFilter(tokenStream, stopWords); CharTermAttribute charTermAttribute = tokenStream.addAttribute(CharTermAttribute.class); tokenStream.reset(); while (tokenStream.incrementToken()) { String term = charTermAttribute.toString(); System.out.println(term); }
Finally, normalization is used to convert text into a consistent format. This may include converting all characters to lowercase, removing punctuation, or converting numbers to their word equivalents. Here is an example of normalization in Java:
String text = "Text Mining is an EXCITING field, with many applications!"; // Convert to lowercase text = text.toLowerCase(); // Remove punctuation text = text.replaceAll("[^a-zA-Z0-9s]", ""); System.out.println(text);
These preprocessing steps are essential for ensuring that the text data is in the right format for further analysis. By leveraging Java’s robust libraries, developers can preprocess text efficiently and prepare it for more complex text mining tasks.
Text Mining Techniques in Java
Text mining techniques in Java are diverse and cater to a wide range of analysis needs. One such technique is sentiment analysis, which aims to determine the emotional tone behind a body of text. That is particularly useful in understanding customer opinions and social media analysis. Here’s an example of how sentiment analysis can be implemented using the Stanford NLP library:
import edu.stanford.nlp.pipeline.*; Properties props = new Properties(); props.setProperty("annotators", "tokenize, ssplit, parse, sentiment"); StanfordCoreNLP pipeline = new StanfordCoreNLP(props); String text = "Java text mining is incredibly useful and exciting!"; Annotation annotation = pipeline.process(text); for (CoreMap sentence : annotation.get(CoreAnnotations.SentencesAnnotation.class)) { String sentiment = sentence.get(SentimentCoreAnnotations.SentimentClass.class); System.out.println(sentiment); }
Another technique is topic modeling, which identifies the topics that a set of documents covers. The Latent Dirichlet Allocation (LDA) is a popular algorithm used for topic modeling. Java developers can utilize the Mallet library to perform LDA:
import cc.mallet.topics.*; import cc.mallet.types.*; // Load and convert data to a form suitable for the model InstanceList instances = LDAUtils.loadInstances("text_data_directory", "stoplists/en.txt"); // Create an LDA model with 10 topics ParallelTopicModel model = new ParallelTopicModel(10); model.addInstances(instances); // Run the model model.setNumThreads(2); model.setNumIterations(1000); model.estimate(); // Get the top words for each topic Object[][] topWords = model.getTopWords(10); for (int i = 0; i topWords.length; i++) { System.out.println("Topic " + i + ": "); for (int j = 0; j topWords[i].length; j++) { System.out.print(topWords[i][j] + " "); } System.out.println("n"); }
Entity recognition is another powerful text mining technique that identifies and classifies named entities within text into predefined categories such as the names of persons, organizations, locations, expressions of times, quantities, monetary values, percentages, etc. The Apache OpenNLP library provides a named entity recognition (NER) feature that can be utilized as follows:
InputStream inputStreamNameFinder = new FileInputStream("en-ner-person.bin"); TokenNameFinderModel model = new TokenNameFinderModel(inputStreamNameFinder); NameFinderME nameFinder = new NameFinderME(model); String[] sentence = new String[]{ "John", "Smith", "is", "a", "software", "engineer", "at", "Oracle" }; Span nameSpans[] = nameFinder.find(sentence); for(Span s: nameSpans) System.out.println("Name: " + s.toString());
These techniques demonstrate just a few ways Java can be used for text mining. By leveraging Java’s extensive libraries and frameworks, developers can apply these techniques to extract meaningful patterns and insights from large volumes of text data.
Applications and Challenges of Text Mining in Java
Text mining in Java has a wide array of applications, ranging from sentiment analysis for customer feedback to topic modeling for document classification. However, with these applications come various challenges that developers need to address.
Applications of Text Mining in Java:
- Sentiment Analysis: Businesses use sentiment analysis to gauge public opinion on products, services, or brands. For example, analyzing tweets mentioning a new product launch can provide insights into consumer reactions.
- Topic Modeling: This technique is used to automatically identify topics present in a collection of documents. It can be used for organizing large sets of unstructured text data, such as news articles or academic papers, into coherent groups.
- Named Entity Recognition: NER can be applied to extract specific information from text, such as names of people, organizations, or locations. This is particularly useful in fields like journalism or intelligence gathering.
- Text Classification: Java can be used to build models that categorize text into predefined classes, which is beneficial for spam filtering, language detection, or categorizing support tickets.
Challenges of Text Mining in Java:
- Handling Large Datasets: Text mining often involves processing large volumes of data, which can be resource-intensive. Optimizing code and using efficient data structures becomes important.
- Data Preprocessing: The quality of text mining results heavily depends on the preprocessing steps. Developers must implement robust preprocessing pipelines to handle noise in the data.
- Natural Language Understanding: Accurately interpreting the context and nuances of natural language is a complex challenge. Advanced NLP techniques and machine learning models are often required.
- Scalability: Text mining applications need to be scalable to handle growing data sizes and complexity. This may involve using distributed computing frameworks like Apache Hadoop or Apache Spark.
Despite these challenges, Java continues to be a popular choice for text mining due to its robust ecosystem and performance capabilities. Let’s look at a code example that demonstrates how Java can be used for sentiment analysis:
import opennlp.tools.sentiment.SentimentME; import opennlp.tools.sentiment.SentimentModel; import opennlp.tools.sentiment.SentimentFactory; import opennlp.tools.util.ObjectStream; import opennlp.tools.util.MarkableFileInputStreamFactory; // Load the sentiment model InputStream modelIn = new FileInputStream("en-sentiment.bin"); SentimentModel model = new SentimentModel(modelIn); // Create a sentiment analyzer SentimentME sentimentAnalyzer = new SentimentME(model); // Analyze the sentiment of a sentence String sentence = "I love using Java for text mining!"; String sentiment = sentimentAnalyzer.getBestSentiment(sentence); System.out.println("The sentiment of the sentence is: " + sentiment);
This code snippet showcases how Java can be used to perform sentiment analysis using the OpenNLP library. Developers can build upon such examples to create more sophisticated text mining applications that can overcome the challenges and deliver valuable insights from text data.
Source: https://www.plcourses.com/java-and-text-mining-analyzing-text-data/