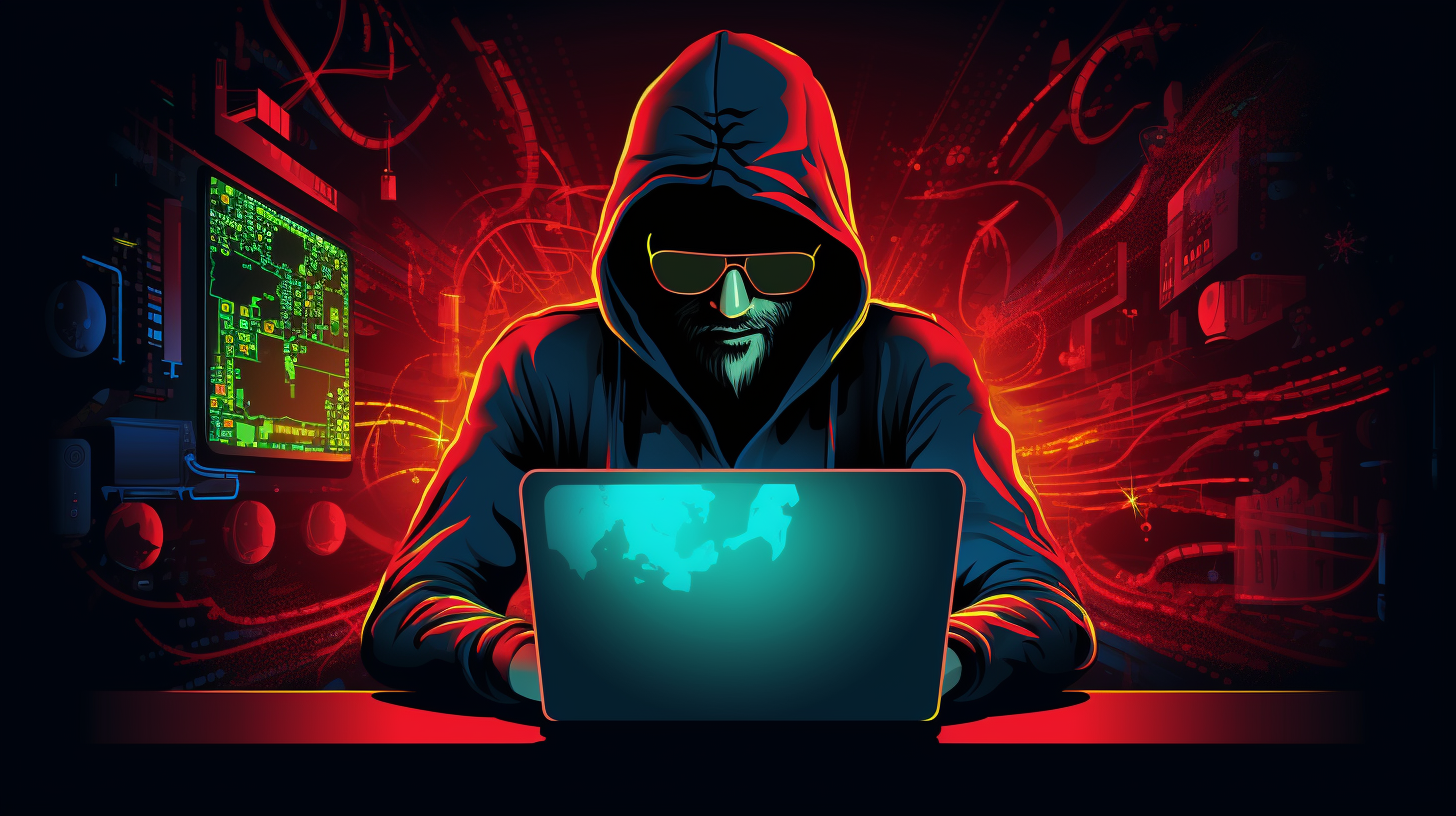
Understanding Java Performance Bottlenecks
Java performance bottlenecks can occur at various levels within an application. It is important to understand the common areas where performance issues may arise to effectively tune and optimize Java applications. Here are some of the key areas to consider when identifying performance bottlenecks:
- Garbage Collection (GC): Inefficient garbage collection can lead to pauses and slow down the application. It’s essential to monitor GC behavior and tune the garbage collector for optimal performance.
- Memory Leaks: Memory leaks occur when objects that are no longer needed are still referenced, preventing them from being garbage collected. This can lead to increased memory usage and eventually OutOfMemoryError.
- CPU Usage: High CPU usage can indicate inefficient algorithms, excessive looping, or lack of proper threading. Profiling CPU usage can help identify hotspots in the code.
- I/O Operations: Slow I/O operations, such as file access or network communication, can significantly impact performance. Optimizing these operations or using asynchronous I/O can improve throughput.
- Synchronization: Overuse of synchronization or contention for shared resources can lead to thread blocking and reduced concurrency, impacting performance negatively.
To diagnose and pinpoint the exact bottleneck, developers can use various profiling tools and techniques. For example, using Java’s built-in profiler, JVisualVM, or third-party tools like YourKit or JProfiler can provide insights into memory usage, CPU cycles, and thread states.
It’s important to measure and profile an application’s performance before making any changes. Premature optimization without proper analysis can lead to unnecessary complexity without significant performance gains.
Let’s look at an example of identifying a memory leak using a code snippet:
public class MemoryLeakExample { private static final List
In the above code, we have a static list that keeps getting filled with new Object
instances without ever being cleared. This will eventually lead to a memory leak as the list grows indefinitely. Profiling tools would help identify the growing size of the list as a potential memory leak source.
Understanding performance bottlenecks is the first step in Java performance tuning. Once identified, developers can apply specific optimization techniques and strategies to mitigate these issues and enhance the application’s speed and efficiency.
Techniques for Optimizing Java Code
Optimizing Java code is a important step to improve the overall performance of an application. Here are some techniques that can be used to write more efficient Java code:
- Use Efficient Data Structures: Choosing the right data structure can have a significant impact on performance. For example, using an
ArrayList
for frequent insertions or deletions may not be as efficient as using aLinkedList
. - Avoid Unnecessary Object Creation: Creating new objects can be expensive. Reusing existing objects or using primitive types instead of wrapper classes can reduce memory overhead and improve performance.
- Minimize Synchronization: Synchronization is essential for thread safety, but overusing it can lead to contention and slow down the application. Use synchronization blocks sparingly and think alternative concurrency mechanisms like
java.util.concurrent
package. - Use Lazy Initialization: Delaying the creation of an object until it’s needed can save resources. However, ensure that this does not introduce thread safety issues.
- Optimize Loops: Loops can be a major source of inefficiency, especially if they contain complex logic or perform expensive operations. Consider breaking down complex loops, caching results, and using enhanced for-loops for collections.
Let’s take a look at a code example that demonstrates loop optimization:
public class LoopOptimizationExample { public void optimizedLoop() { Listlist = new ArrayList>(Arrays.asList("a", "b", "c", "d", "e")); String result = ""; // Instead of using get(i) in each iteration, we use an enhanced for-loop for (String s : list) { result += s; } } }
In the above example, we used an enhanced for-loop to iterate over the list, which is more efficient than using get(i)
in each iteration. This kind of optimization can lead to better performance, especially with large collections.
Note: Always profile and measure the performance impact before and after applying these optimizations. Not all techniques will have the same effect on every application, and some may even degrade performance if not used appropriately.
By applying these techniques, developers can write more performant Java code that runs faster and uses resources more efficiently. However, it’s important to strike a balance between code readability, maintainability, and performance optimization.
Advanced Java Performance Tuning Strategies
As we delve into advanced Java performance tuning strategies, it’s essential to consider techniques that go beyond basic optimizations. These strategies often involve a deeper understanding of the Java Virtual Machine (JVM) and require careful analysis and testing. Here are some advanced strategies that can significantly improve the performance of your Java applications:
- JVM Tuning: The JVM has several parameters that can be fine-tuned to optimize performance. These include heap size, garbage collector settings, and Just-In-Time (JIT) compiler options. For example, adjusting the maximum heap size with the
-Xmx
parameter can help prevent frequent garbage collections in memory-intensive applications. - Native Code Integration: In some cases, integrating native code using the Java Native Interface (JNI) can yield performance improvements. This should be done judiciously, as native code can introduce complexity and potential security risks.
- Bytecode Optimization: Tools like ProGuard can optimize and obfuscate Java bytecode, resulting in smaller and potentially faster code. However, be aware that this may make debugging more challenging.
- Concurrent Data Structures: The
java.util.concurrent
package provides several data structures optimized for concurrent access. Using these can improve scalability in multi-threaded applications. - Application-Specific Tuning: Sometimes, the best optimizations are those tailored to the application’s specific needs. This might involve algorithmic changes, caching strategies, or custom data structures.
Let’s consider an example where JVM tuning can be applied to improve performance:
// Example of setting maximum heap size // Run the application with the following JVM argument java -Xmx1024m com.example.MyApplication
In this example, we set the maximum heap size to 1024 megabytes, which can help accommodate larger data sets without frequent garbage collections.
Another example is using concurrent data structures to improve multi-threaded performance:
import java.util.concurrent.ConcurrentHashMap; public class ConcurrentDataStructureExample { private ConcurrentHashMapconcurrentMap = new ConcurrentHashMap>(); public void performConcurrentOperations() { // Operations on the concurrentMap will benefit from improved scalability concurrentMap.put(1, "value1"); String value = concurrentMap.get(1); // Other concurrent operations... } }
In this code snippet, we use a ConcurrentHashMap
, which provides better thread-safety and performance under high concurrency compared to a synchronized HashMap
.
Note: Advanced performance tuning strategies can lead to significant improvements, but they also come with trade-offs. It is important to thoroughly test and validate the impact of any changes on your specific application and workload. Additionally, always ensure that maintainability and code clarity are not sacrificed in pursuit of performance.
To wrap it up, advanced Java performance tuning requires a combination of JVM knowledge, careful analysis, and sometimes creative solutions tailored to the application’s needs. By applying these strategies judiciously and measuring their impact, developers can achieve substantial performance gains.
Source: https://www.plcourses.com/java-performance-tuning-tips-and-techniques/