Hi, If you are looking for the PHP script to learn how to send email with attachment in PHP without going to spam then you are in the right place. I know you searched a lot on Google to find source code but every code sends that to spam, not in the inbox. Check our demo to confirm.
Here I am going to give a PHP script by which your email with an attachment will always go to inbox. Sending an email with attachment is the most common task in web development.
When you are applying for a job or submitting any application, you often might be noticed that they accept a file to upload. It may be anything like your CV or resume, profile picture or any other document.
Sending details to email is sometimes good in terms of saving space on our server. Since we are not storing the details on our database or attachment to our server folders.
How to do that?
Here we will use a simple HTML form accepting basic details like name, email, subject, message, and an attachment. The attachment has a validation that only PDF, DOC, JPG, JPEG, & PNG files are allowed to upload. In the previous tutorial, we learned about how to send email in PHP step by step but here we will do the same with attachment.
So what are we waiting for? Let’s start. Check the files and folder we are going to create.
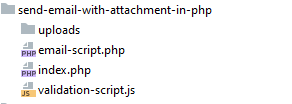
- index.php is the main file which is a simple HTML form as I told above.
- email-script.php is the script file that sends an email with an attachment.
- validation-script.js is a javaScript validation file.
- uploads folder saves the attachment to send emails and deletes later.
Now we should create these files. I am giving you just code block here. Make sure you put these CDN in to head off your index.php to work your code.
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
And include this javaScript validation file also before closing to the body tag.
<script src="validation-script.js"></script>
index.php
<div class="container"> <div class="row"> <div class="col-lg-8 posts-list"> <div class="card card-signin my-5"> <div class="card-body"> <center><img src="../website/img/coding-birds-online/coding-birds-online-favicon.png" width="70"></center> <h5 class="card-title text-center">Send email with attachment in PHP</h5> <form method="post" action="email-script.php" enctype="multipart/form-data" id="emailForm"> <div class="form-group"> <input type="text" name="name" id="name" class="form-control" placeholder="Name" > <div id="nameError" style="color: red;font-size: 14px;display: none">nameError</div> </div> <div class="form-group"> <input type="email" name="email" id="email" class="form-control" placeholder="Email address" > <div id="emailError" style="color: red;font-size: 14px;display: none">emailError</div> </div> <div class="form-group"> <input type="text" name="subject" id="subject" class="form-control" placeholder="Subject" > <div id="subjectError" style="color: red;font-size: 14px;display: none">subjectError</div> </div> <div class="form-group"> <textarea name="message" id="message" class="form-control" placeholder="Write your message here"></textarea> <div id="messageError" style="color: red;font-size: 14px;display: none">messageError</div> </div> <div class="form-group"> <input type="file" name="attachment" id="attachment" class="form-control"> <div id="attachmentError" style="color: red;font-size: 14px;display: none">attachmentError</div> </div> <div class="submit"> <input type="submit" name="submit" onclick="return validateEmailSendForm();" class="btn btn-success" value="SUBMIT"> </div> </form> </div> </div> </div> </div> </div>
If you notice this form uses an action to email-script.php this file. Create this file also.
email-script.php
<?php if(isset($_POST['submit'])){ // Get the submitted form data $email = $_POST['email']; $name = $_POST['name']; $subject = $_POST['subject']; $message = $_POST['message']; $uploadStatus = 1; // Upload attachment file if(!empty($_FILES["attachment"]["name"])){ // File path config $targetDir = "uploads/"; $fileName = basename($_FILES["attachment"]["name"]); $targetFilePath = $targetDir . $fileName; $fileType = pathinfo($targetFilePath,PATHINFO_EXTENSION); // Allow certain file formats $allowTypes = array('pdf', 'doc', 'docx', 'jpg', 'png', 'jpeg'); if(in_array($fileType, $allowTypes)){ // Upload file to the server if(move_uploaded_file($_FILES["attachment"]["tmp_name"], $targetFilePath)){ $uploadedFile = $targetFilePath; }else{ $uploadStatus = 0; $statusMsg = "Sorry, there was an error uploading your file."; } }else{ $uploadStatus = 0; $statusMsg = 'Sorry, only PDF, DOC, JPG, JPEG, & PNG files are allowed to upload.'; } } if($uploadStatus == 1){ // Recipient $toEmail = $email; // Sender $from = '[email protected]'; $fromName = 'Coding Birds Online'; // Subject $emailSubject = 'Email attachment request Submitted by '.$name; // Message $htmlContent = '<h2>Contact Request Submitted</h2> <p><b>Name:</b> '.$name.'</p> <p><b>Email:</b> '.$email.'</p> <p><b>Subject:</b> '.$subject.'</p> <p><b>Message:</b><br/>'.$message.'</p>'; // Header for sender info $headers = "From: $fromName"." <".$from.">"; if(!empty($uploadedFile) && file_exists($uploadedFile)){ // Boundary $semi_rand = md5(time()); $mime_boundary = "==Multipart_Boundary_x{$semi_rand}x"; // Headers for attachment $headers .= "nMIME-Version: 1.0n" . "Content-Type: multipart/mixed;n" . " boundary="{$mime_boundary}""; // Multipart boundary $message = "--{$mime_boundary}n" . "Content-Type: text/html; charset="UTF-8"n" . "Content-Transfer-Encoding: 7bitnn" . $htmlContent . "nn"; // Preparing attachment if(is_file($uploadedFile)){ $message .= "--{$mime_boundary}n"; $fp = @fopen($uploadedFile,"rb"); $data = @fread($fp,filesize($uploadedFile)); @fclose($fp); $data = chunk_split(base64_encode($data)); $message .= "Content-Type: application/octet-stream; name="".basename($uploadedFile).""n" . "Content-Description: ".basename($uploadedFile)."n" . "Content-Disposition: attachment;n" . " filename="".basename($uploadedFile).""; size=".filesize($uploadedFile).";n" . "Content-Transfer-Encoding: base64nn" . $data . "nn"; } $message .= "--{$mime_boundary}--"; $returnpath = "-f" . $email; // Send email $mail = mail($toEmail, $emailSubject, $message, $headers, $returnpath); // Delete attachment file from the server @unlink($uploadedFile); }else{ // Set content-type header for sending HTML email $headers .= "rn". "MIME-Version: 1.0"; $headers .= "rn". "Content-type:text/html;charset=UTF-8"; // Send email $mail = mail($toEmail, $emailSubject, $htmlContent, $headers); } // If mail sent if($mail){ $statusMsg = 'Your email attachment request has been submitted successfully !'; }else{ $statusMsg = 'Your request submission failed, please try again.'; } } echo '<script>alert("'.$statusMsg.'");window.location.href="./";</script>'; } ?>
validation-script.js
function validateEmailSendForm() { var name = $("#name").val(); var email = $("#email").val(); var subject = $("#subject").val(); var message = $("#message").val(); var attachment = $("#attachment").val(); if (name == ""){ $("#nameError").show(); $("#nameError").html("Please enter your name"); $("#nameError").fadeOut(4000); $("#name").focus(); return false; }else if (email == ""){ $("#emailError").show(); $("#emailError").html("Please enter your email"); $("#emailError").fadeOut(4000); $("#email").focus(); return false; }else if (!validateEmail(email)){ $("#emailError").show(); $("#emailError").html("Please enter valid email"); $("#emailError").fadeOut(4000); $("#email").focus(); return false; }else if (subject == ""){ $("#subjectError").show(); $("#subjectError").html("Please enter subject"); $("#subjectError").fadeOut(4000); $("#subject").focus(); return false; }else if (message == ""){ $("#messageError").show(); $("#messageError").html("Please enter some message"); $("#messageError").fadeOut(4000); $("#message").focus(); return false; }else if (attachment == ""){ $("#attachmentError").show(); $("#attachmentError").html("Please select a attachment"); $("#attachmentError").fadeOut(4000); $("#attachment").focus(); return false; }else{ return true; } function validateEmail(inputText) { var mailformat = /^w+([.-]?w+)*@w+([.-]?w+)*(.w{2,3})+$/; if(inputText.match(mailformat)) { return true; } else{ return false; } } }
Run the code!
Now it’s time to run and test our code. If you did everything step by step then you will not face any errors and you will get output something like this.
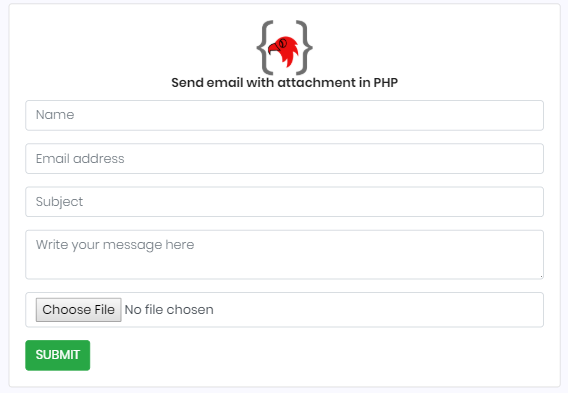
This form is validated properly like empty fields, valid email and attachment file accepted. When you fill that form and hit the submit button then you will get the alert message of success or errors.
Check this also How to make a dynamic pie chart in PHP in 2 steps.
Attention !!! It may take 1 or 2 minutes to receive email with attachment so please have patience. But I am sure you will receive in primary inbox not to spam.

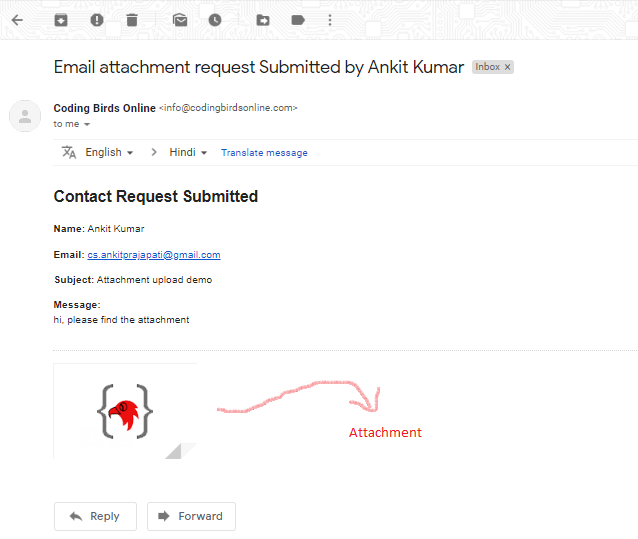
Source Code & Demo
You can download the full 100% working source code from here. You check this demo also.
Conclusion
I hope you learned explained above, If you have any suggestions, are appreciated. And if you have any errors comment here. You can download the full 100% working source code from here.
Ok, Thanks for reading this article, see you in the next post.
Happy Coding 🙂
Source: https://codingbirdsonline.com/how-to-send-email-with-attachment-in-php/