To hide email addresses or contact numbers partially is the most common thing in web application development. If you might have noticed on so many websites when you register by using your email id or contact number, then they shoot a verification code on your email or phone.
And you can’t see the full email address or contact. They make it partially hidden by using some stars *. You can check this demo also.
So If you are looking to do the same thing on PHP and looking for how to partially hide email address in PHP using AJAX then you are in the right place.
Check this post also on how to clone a specific folder from a git repository.
How to do?
Here I will use a simple form accepting email id. When you press the submit button you will get a partially hidden email without page refresh.
To do this I will use AJAX functionality so that we can achieve this without page refresh. So without wasting time lets make out hands dirty with code.
- Create index.php file is the main file
- Now create script.php file is the logic file to hide email address partially.
index.php
<!doctype html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <link rel="icon" href="https://codingbirdsonline.com/wp-content/uploads/2019/12/cropped-coding-birds-favicon-2-1-192x192.png" type="image/x-icon"> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> <title>How to partially hide email address in PHP using AJAX - Coding Birds Online</title> <style>.required{color: #FF0000;}</style> </head> <body> <div class="container"> <div class="row"> <div class="col-sm-9 col-md-7 col-lg-5 mx-auto"> <div class="card card-signin my-5"> <div class="card-body"> <center><img width="50" src="https://codingbirdsonline.com/wp-content/uploads/2019/12/cropped-coding-birds-favicon-2-1-192x192.png"></center> <h5 class="card-title text-center">Partially hide email address in php </h5> <form id="myForm"> <div class="form-label-group"> <label for="inputEmail">Email <span class="required">*</span></label> <input type="text" name="email" id="email" class="form-control" placeholder="Enter email "> </div><br/> <div id="partiallyHiddenEmail"></div><br/> <button type="submit" name="submitBtn" id="submitBtn" class="btn btn-md btn-primary btn-block text-uppercase" >Hide</button> </form> </div> </div> </div> </div> </div> <script type="text/javascript"> $("form#myForm").on("submit",function (e) { e.preventDefault(); var email = $("#email").val(); if (email == ""){ alert("Please enter email"); $("#email").focus(); }else if (!validateEmail(email)){ alert("Please enter valid email"); $("#email").focus(); } else { $.post("script.php",{key:"hideEmailAddress",email:email},function (response) { $("#partiallyHiddenEmail").html(response); }); } }); function validateEmail(inputText) { var mailformat = /^w+([.-]?w+)*@w+([.-]?w+)*(.w{2,3})+$/; if(inputText.match(mailformat)) { return true; } else{ return false; } } </script> </body> </html>
This file uses the AJAX call, which in turn calls the script.php file.
script.php
<?php if ($_POST['key'] == "hideEmailAddress"){ $email = $_POST['email']; $partiallyHiddenEmail = mask_email($email); echo $partiallyHiddenEmail; } /* Here's the logic: We want to show X numbers. If length of STR is less than X, hide all. Else replace the rest with *. */ function mask_email($email) { $mail_parts = explode("@", $email); $domain_parts = explode('.', $mail_parts[1]); $mail_parts[0] = mask($mail_parts[0], 2, 1); // show first 2 letters and last 1 letter $domain_parts[0] = mask($domain_parts[0], 2, 1); // same here $mail_parts[1] = implode('.', $domain_parts); return implode("@", $mail_parts); } function mask($str, $first, $last) { $len = strlen($str); $toShow = $first + $last; return substr($str, 0, $len <= $toShow ? 0 : $first).str_repeat("*", $len - ($len <= $toShow ? 0 : $toShow)).substr($str, $len - $last, $len <= $toShow ? 0 : $last); }
Run the Code!
When you run the code you will get something like this. if you get any errors, don’t worry I will provide the full 100% working source code.
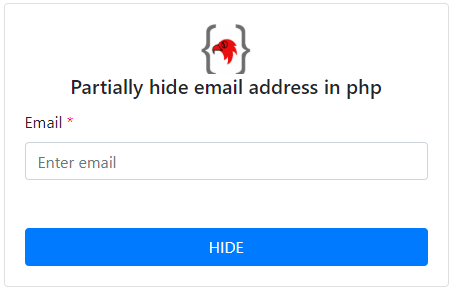
If you enter the email address [email protected], Of course, you can enter your own. You will get the following output.

Source Code & Demo
You can download the full 100% working source code from here. You check this demo also.
Conclusion
I hope you learned explained above, If you have any suggestions, are appreciated. And if you have any errors comment here. You can download the full 100% working source code from here.
Ok, Thanks for reading this article, see you in the next post.
Happy Coding 🙂
Source: https://codingbirdsonline.com/how-to-partially-hide-email-address-in-php-ajax/