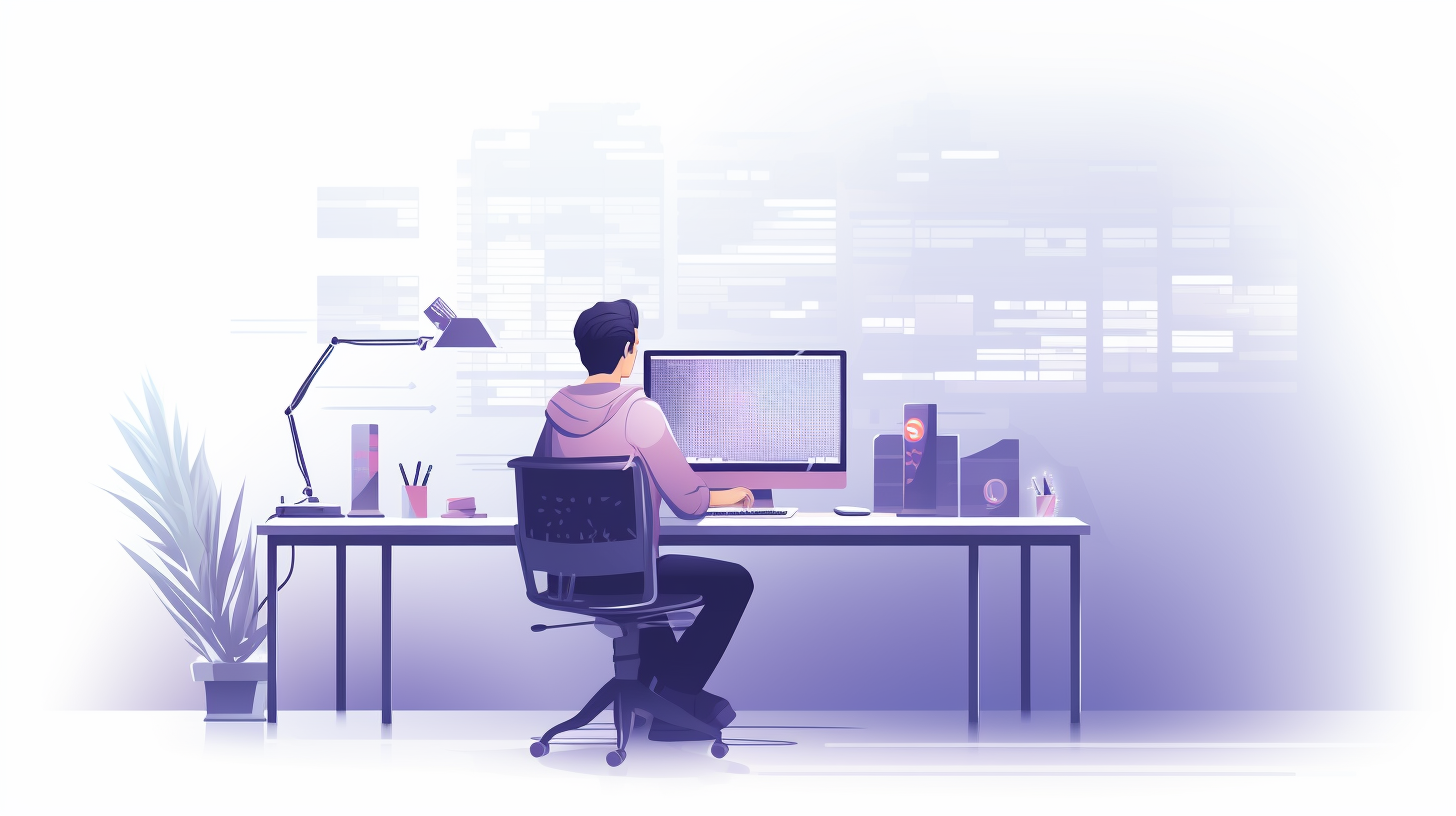
User-Defined Functions (UDFs) in SQL serve as powerful tools that allow developers to encapsulate reusable logic within the database itself. These functions enable the creation of customized operations that can be invoked as part of SQL statements, significantly enhancing modularity and clarity in database queries.
A UDF can return a single value or a table, depending on its configuration. The primary advantage of using UDFs lies in their reusability across multiple queries and applications, fostering a more organized and efficient codebase.
UDFs are typically categorized into three types: scalar functions, table-valued functions, and inline table-valued functions. Scalar functions return a single value, while table-valued functions return a set of rows. Inline table-valued functions, a subtype of table-valued functions, offer a streamlined syntax that can improve performance.
To create a user-defined function, you must define its structure, including parameters and return types. Below is an example of a simple scalar function that takes a numeric input and returns its square:
CREATE FUNCTION dbo.SquareNumber ( @InputNumber INT ) RETURNS INT AS BEGIN RETURN @InputNumber * @InputNumber; END;
After defining this function, you can call it within your SQL queries. For example, to calculate the square of the number 5, you would use:
SELECT dbo.SquareNumber(5) AS SquareValue;
This query would yield a result of 25, demonstrating the function’s utility. By encapsulating logic in a UDF, you not only promote code reuse but also enhance the maintainability of your SQL scripts, as changes to the logic need to be made in only one place.
Moreover, UDFs can be particularly valuable when dealing with complex calculations or transformations that are otherwise cumbersome to repeat across various queries. For instance, think a scenario where you frequently need to convert temperatures from Fahrenheit to Celsius. Instead of repeating the conversion formula in every query, you can encapsulate the logic in a UDF:
CREATE FUNCTION dbo.FahrenheitToCelsius ( @Fahrenheit FLOAT ) RETURNS FLOAT AS BEGIN RETURN (@Fahrenheit - 32) * 5.0 / 9.0; END;
Once created, this function can be invoked seamlessly within SELECT statements:
SELECT dbo.FahrenheitToCelsius(98.6) AS CelsiusValue;
This approach not only simplifies your queries but also minimizes the risk of errors associated with manual calculations. However, it’s essential to use UDFs judiciously, as poorly optimized functions can lead to performance bottlenecks, particularly when executed on large datasets.
Creating and Implementing UDFs for Data Transformation
To implement UDFs effectively for data transformation, you must ponder both the structure of the function and the context in which it will be used. The process begins with defining the function’s parameters and specifying its return type, ensuring that it aligns with the intended transformation logic. A well-designed function not only encapsulates the transformation logic but also optimizes it for performance.
For more complex transformations, you might want to create a table-valued function. This type of UDF is particularly useful when you need to return a dataset rather than a single scalar value. For example, let’s say you need a function that returns employee details filtered by their department. You can define the table-valued function as follows:
CREATE FUNCTION dbo.GetEmployeesByDepartment ( @DepartmentID INT ) RETURNS TABLE AS RETURN ( SELECT EmployeeID, FirstName, LastName, JobTitle FROM Employees WHERE DepartmentID = @DepartmentID );
After creating this function, you can easily retrieve a list of employees from a specific department using a simple SELECT statement:
SELECT * FROM dbo.GetEmployeesByDepartment(3);
This query will return all employees belonging to the department with ID 3, showcasing how UDFs can streamline data retrieval and transformation processes.
When creating UDFs, it’s crucial to ensure that the logic remains efficient, particularly in cases where large datasets are involved. Using inline table-valued functions can help mitigate performance issues since they allow for optimization by the SQL Server query engine. For instance, here is an example of an inline table-valued function that performs a similar role as the previous example:
CREATE FUNCTION dbo.InlineGetEmployeesByDepartment ( @DepartmentID INT ) RETURNS TABLE AS RETURN ( SELECT EmployeeID, FirstName, LastName, JobTitle FROM Employees WHERE DepartmentID = @DepartmentID );
The inline function’s syntax is more concise, and because it’s treated like a view, it can be optimized during query execution. Calling this function remains the same:
SELECT * FROM dbo.InlineGetEmployeesByDepartment(2);
Within the scope of data transformation, UDFs allow for a high degree of customization and modularity. However, while they can simplify the reuse of complex logic, it’s imperative to monitor their performance. Avoid using UDFs in a way that would lead to row-by-row operations when set-based operations would suffice. The difference in performance can be staggering, especially with large volumes of data.
Optimizing Performance with UDFs in SQL Queries
When integrating User-Defined Functions (UDFs) into your SQL queries, performance optimization becomes paramount, especially as the scale and complexity of your data processing needs grow. While UDFs offer the allure of encapsulation and reusability, they can also inadvertently introduce inefficiencies if not designed with an eye towards execution performance.
One of the first considerations in optimizing UDFs is understanding how they’re executed within the SQL Server environment. Scalar UDFs, for instance, are typically executed in a row-by-row manner when they are called in a SELECT statement. This can lead to severe performance degradation, particularly when processing large datasets. Instead of relying solely on scalar functions, think rewriting expressions as inline table-valued functions or using common table expressions (CTEs) whenever possible.
For example, if you have a scalar UDF that calculates a discount based on an input value, using it in a query like this:
SELECT ProductID, dbo.CalculateDiscount(Price) AS DiscountedPrice FROM Products;
This could lead to inefficiencies due to the function being executed for each row. A better approach would be to incorporate the discount logic directly into your SQL statement or use an inline table-valued function, allowing SQL Server’s optimizer to better leverage set-based processing:
CREATE FUNCTION dbo.InlineCalculateDiscount ( @Price DECIMAL(10, 2) ) RETURNS TABLE AS RETURN ( SELECT @Price * 0.9 AS DiscountedPrice -- Assuming a fixed 10% discount ); SELECT ProductID, d.DiscountedPrice FROM Products CROSS APPLY dbo.InlineCalculateDiscount(Price) AS d;
By using CROSS APPLY
, the discount calculation is performed in a set-based manner, improving performance significantly.
Moreover, ponder caching results when working with UDFs that operate on static or less frequently changing data. If a function pulls from a relatively static dataset, caching the output for repeated calls can eliminate unnecessary computations. SQL Server’s result caching through indexed views or temporary tables can be advantageous here.
It’s also beneficial to analyze execution plans to identify bottlenecks caused by UDFs. Use SQL Server Management Studio’s execution plan feature to visualize how your queries are executed and where optimizations can be made. For instance, if you notice that your UDF calls lead to scans instead of seeks, it may be time to reevaluate the indexing strategy for the underlying tables.
In addition, you should leverage built-in SQL functions wherever feasible. SQL Server has a rich set of built-in functions that are optimized for performance and can often provide the same results as a custom UDF without the associated overhead. For example, instead of creating a UDF to calculate the number of days between two dates, you can use the built-in DATEDIFF
function directly:
SELECT ProductID, DATEDIFF(DAY, StartDate, EndDate) AS DaysBetween FROM ProductSchedule;
Lastly, testing and profiling should be integral parts of your UDF development lifecycle. Use SQL Server’s built-in tools to measure the performance impact of your functions under various workloads. Using SQL Profiler
or Extended Events
can provide insights into how often your UDFs are invoked and their execution times, which will allow you to fine-tune the logic for optimal performance.
Real-World Applications of User-Defined Functions in Data Processing
In the context of data processing, User-Defined Functions (UDFs) offer significant advantages by allowing developers to encapsulate complex logic and reuse it across multiple queries and applications. Their versatility makes them ideal candidates for a variety of real-world applications, ranging from simple calculations to sophisticated data transformations.
One common use case for UDFs is in the sphere of financial applications, where precise calculations are crucial. For instance, let’s say you need to calculate the compound interest for various investments based on different rates and time periods. Instead of repeating the calculation logic in multiple queries, you can create a scalar UDF to handle this:
CREATE FUNCTION dbo.CalculateCompoundInterest ( @Principal DECIMAL(10, 2), @Rate DECIMAL(5, 2), @Time INT ) RETURNS DECIMAL(10, 2) AS BEGIN RETURN @Principal * POWER((1 + @Rate / 100), @Time); END;
With this function in place, invoking it becomes simpler, allowing for easily readable queries:
SELECT InvestmentID, dbo.CalculateCompoundInterest(InvestmentAmount, InterestRate, InvestmentDuration) AS FutureValue FROM Investments;
Another practical use of UDFs is in data cleansing processes. Consider a scenario where you frequently need to standardize phone numbers stored in various formats. A UDF can become your go-to solution for transforming these values into a consistent format. Here’s an example of a scalar UDF that formats phone numbers:
CREATE FUNCTION dbo.FormatPhoneNumber ( @PhoneNumber VARCHAR(15) ) RETURNS VARCHAR(15) AS BEGIN RETURN '(' + SUBSTRING(@PhoneNumber, 1, 3) + ') ' + SUBSTRING(@PhoneNumber, 4, 3) + '-' + SUBSTRING(@PhoneNumber, 7, 4); END;
By using this function in your updates, you can easily ensure that all phone numbers conform to a specific format:
UPDATE Contacts SET PhoneNumber = dbo.FormatPhoneNumber(PhoneNumber);
Furthermore, data analytics often involves aggregating or summarizing information from large datasets. UDFs can play an essential role in these scenarios, particularly when you need to compute metrics that are not simpler. For example, you might want to compute the average sales per month but only for the top-selling products. A table-valued function can encapsulate this logic:
CREATE FUNCTION dbo.GetTopProductsAverageSales ( @TopN INT ) RETURNS TABLE AS RETURN ( SELECT ProductID, AVG(SalesAmount) AS AverageSales FROM Sales WHERE ProductID IN (SELECT TOP(@TopN) ProductID FROM Sales GROUP BY ProductID ORDER BY SUM(SalesAmount) DESC) GROUP BY ProductID );
Invoking this function allows you to quickly analyze top-selling products and their average sales:
SELECT * FROM dbo.GetTopProductsAverageSales(10);
In addition to these examples, UDFs can be invaluable in implementing business logic that needs to be consistent throughout various applications. By encapsulating this logic within a UDF, you can ensure that any changes to the logic only require a single point of modification, enhancing maintainability and reducing the risk of errors in your database operations.
However, when using UDFs in real-world applications, it’s vital to monitor performance. As previously discussed, poorly optimized UDFs can lead to performance degradation, especially when working with large datasets. Therefore, understanding the specific use case and potential data volumes will allow you to design UDFs that are not only functional but also efficient.
Source: https://www.plcourses.com/sql-and-user-defined-functions-for-data-processing/