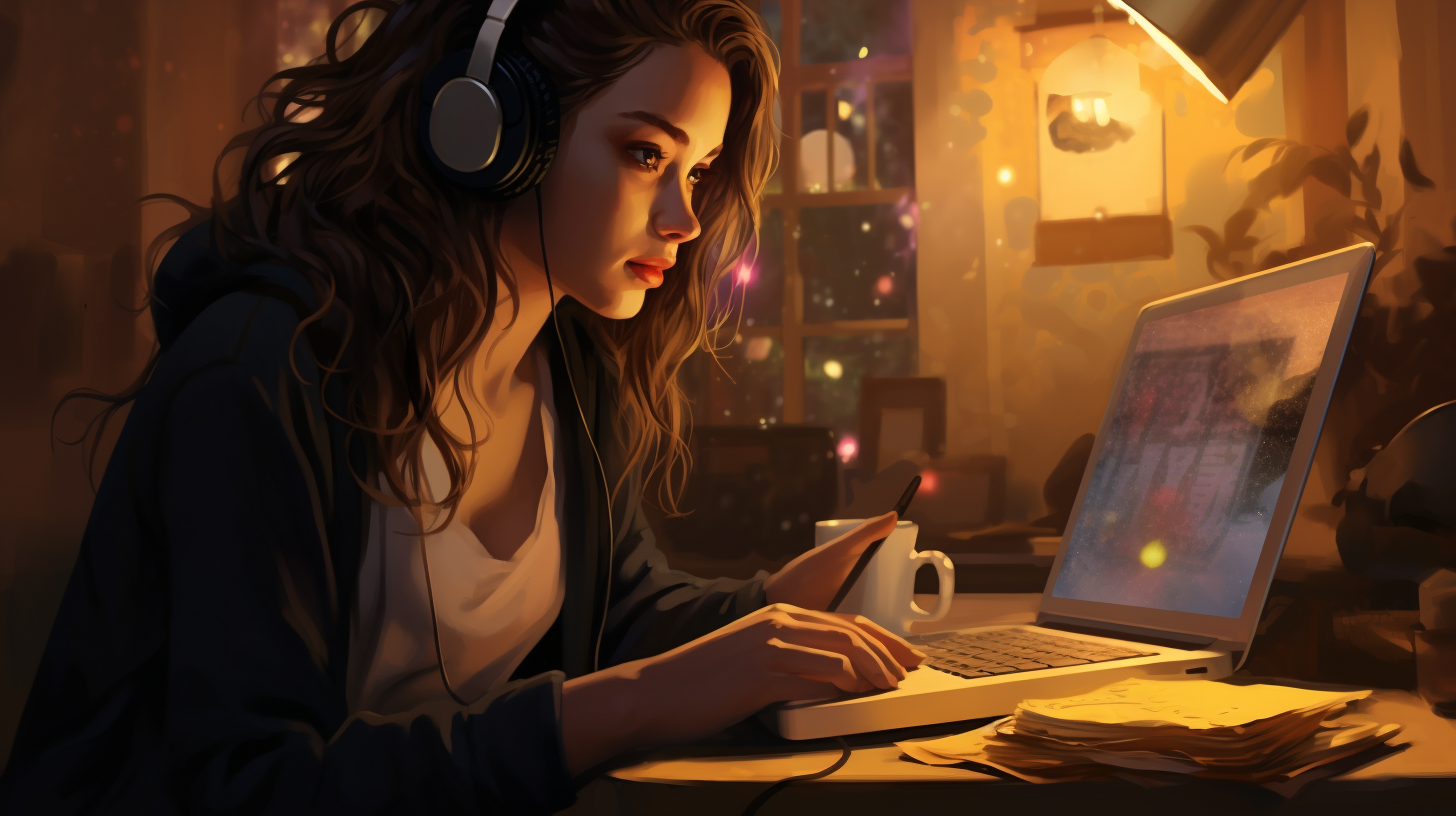
Overview of Swift Programming Language
Swift is a powerful and intuitive programming language created by Apple for building apps for iOS, Mac, Apple TV, and Apple Watch. It’s designed to give developers more freedom than ever before. Swift is easy to use and open source, so anyone with an idea can create something incredible.
Swift is a type-safe language which means the language helps you to be clear about the types of values your code can work with. If part of your code requires a String
, type safety prevents you from passing it an Int
by mistake. Likewise, type safety prevents you from accidentally passing an optional String
to a piece of code that requires a non-optional String
.
var optionalString: String? = "Hello" var requiredString: String = optionalString! // Forced unwrapping of the optional
Swift also makes extensive use of variables and constants. Variables are declared using the var
keyword and can be changed after they’re created. Constants are declared with the let
keyword and cannot be changed once their value is set.
var variable = "I can change" let constant = "I cannot change" variable = "See, I changed!" // constant = "If you uncomment this line, it will result in a compile-time error"
Swift has many other features that make it unique, such as:
- Closures that are first-class objects, which means they can be nested and passed around in your code.
- Tuples that allow you to create and pass around groupings of values.
- Generics that enable you to write flexible, reusable functions and types.
- Structs and classes that both support methods, properties, and initializers.
Swift also provides powerful features that are not available in Objective-C, such as:
- Optionals that indicate the absence of a value by wrapping around any type.
- Enumerations that can have methods associated with them.
- Pattern matching that allows you to easily match values in a
switch
statement.
enum Beverage: CaseIterable { case coffee, tea, juice } let favoriteBeverage = Beverage.coffee switch favoriteBeverage { case .coffee: print("I love coffee") case .tea: print("Tea is relaxing") case .juice: print("Juice is refreshing") }
Swift’s syntax encourages you to write clean and consistent code which may even feel strict at times. However, the benefits of a clean and consistent codebase, with fewer bugs, are significant. Swift’s compiler, debugger, and other tools are constantly improving to help you write better code, faster.
Swift is not just a language, it is a complete system for developing apps that are fast, safe, and expressive.
Introduction to QuickLook Framework
The QuickLook framework is a powerful tool provided by Apple that allows developers to provide rich previews of their content within their applications. It is available on iOS, macOS, and other Apple platforms. With QuickLook, users can preview documents, images, and other types of files directly in the app without having to open them in a separate application.
QuickLook is especially useful for file browsing apps, email clients, or any application that deals with a variety of file types. It is designed to be easy to implement and provides a consistent user experience across all Apple devices.
To use the QuickLook framework, you need to import it into your Swift file:
import QuickLook
Once imported, you can create a QLPreviewController
which is the main class used for previewing items. The controller requires a data source that conforms to the QLPreviewControllerDataSource
protocol. This data source provides the items to be previewed.
class MyPreviewController: QLPreviewControllerDataSource { var items: [QLPreviewItem] = [] func numberOfPreviewItems(in controller: QLPreviewController) -> Int { return items.count } func previewController(_ controller: QLPreviewController, previewItemAt index: Int) -> QLPreviewItem { return items[index] } }
With the data source in place, you can present the QLPreviewController
just like any other view controller:
let previewController = QLPreviewController() previewController.dataSource = MyPreviewController() present(previewController, animated: true)
QuickLook handles the rest, providing a effortless to handle interface to browse through the items. It supports a wide range of file formats, including PDFs, images, text files, and Microsoft Office documents, among others.
One of the key benefits of QuickLook is that it provides a seamless experience for users. They don’t need to leave your app to view a file, and they get a consistent preview interface no matter what type of file they’re viewing.
Overall, the QuickLook framework is a powerful addition to any app that handles files. It is simple to implement, provides a great user experience, and supports a wide range of file types.
Integrating QuickLook in Swift Applications
Integrating QuickLook into your Swift applications can significantly enhance the user experience by providing instant access to file previews. To begin the integration process, you must first ensure that your Swift application is set up to handle the file types you wish to preview. This involves adopting the QLPreviewControllerDataSource
protocol in one of your classes, typically a view controller.
import QuickLook class PreviewController: UIViewController, QLPreviewControllerDataSource { var files: [URL] = [] func numberOfPreviewItems(in controller: QLPreviewController) -> Int { return files.count } func previewController(_ controller: QLPreviewController, previewItemAt index: Int) -> QLPreviewItem { return files[index] as QLPreviewItem } }
After implementing the data source protocol, instantiate a QLPreviewController
and set its dataSource
to an instance of your class that conforms to QLPreviewControllerDataSource
. Present the preview controller when you want to show the previews.
let quickLookController = QLPreviewController() quickLookController.dataSource = previewController present(quickLookController, animated: true)
To further customize the preview experience, you can implement the QLPreviewControllerDelegate
protocol. This allows you to respond to user actions, such as when they select an item for preview or when they dismiss the preview controller.
extension PreviewController: QLPreviewControllerDelegate { func previewControllerWillDismiss(_ controller: QLPreviewController) { // Handle the preview controller dismissal if needed } func previewController(_ controller: QLPreviewController, shouldOpen url: URL, for item: QLPreviewItem) -> Bool { // Return true if you want the URL to be opened return true } }
By integrating QuickLook into your Swift applications, you provide users with a familiar and consistent way to preview files, which can be instrumental in building simple to operate and professional apps.
Customizing QuickLook Previews
Customizing QuickLook previews in your Swift applications can greatly enhance the user experience by providing a tailored preview for your specific content. To begin customizing, you can create your own objects that conform to the QLPreviewItem
protocol. This allows you to provide additional information about the item, such as its title or URL.
class CustomPreviewItem: NSObject, QLPreviewItem { var previewItemURL: URL? var previewItemTitle: String? init(url: URL, title: String) { self.previewItemURL = url self.previewItemTitle = title } }
Once you have your custom preview item, you can return it from the previewController(_:previewItemAt:)
method of your data source:
func previewController(_ controller: QLPreviewController, previewItemAt index: Int) -> QLPreviewItem { let customItem = CustomPreviewItem(url: files[index], title: "Custom Title") return customItem }
Additionally, you can customize the QuickLook preview controller’s appearance and behavior by modifying its properties. For instance, you can set the background color or hide the navigation bar:
let previewController = QLPreviewController() previewController.view.backgroundColor = UIColor.lightGray previewController.navigationController?.isNavigationBarHidden = true
For a more advanced customization, you can implement your own view controller that conforms to the QLPreviewingController
protocol. This protocol allows you to provide a custom preview interface for a specific item type. Here’s a basic example of a custom previewing controller:
class MyCustomPreviewController: UIViewController, QLPreviewingController { func preparePreviewOfFile(at url: URL, completionHandler handler: @escaping (Error?) -> Void) { // Set up your custom view using the URL // Call the completion handler when the preview is ready to be displayed handler(nil) } }
To use your custom previewing controller, you must register it with the QuickLook preview controller:
QLPreviewController.register(MyCustomPreviewController.self, forPreviewingAt: supportedURL)
By customizing QuickLook previews, you can provide a unique and engaging preview experience that aligns with the look and feel of your application, making it stand out from the rest.
Best Practices for Using QuickLook in Swift Applications
When using QuickLook in your Swift applications, it’s important to follow best practices to ensure a smooth and efficient user experience. Here are some tips for making the most out of QuickLook:
- Always load files asynchronously to prevent blocking the main thread. This ensures that your application remains responsive while QuickLook prepares the preview.
- Be mindful of the file formats you support. QuickLook supports a wide range of file types, but it is best to check for compatibility and provide alternatives if necessary.
- Implement proper error handling to gracefully handle situations where a file cannot be previewed.
- If your app deals with sensitive content, make sure to handle it securely and think implementing additional security measures when using QuickLook.
- Take advantage of customization options to align the QuickLook preview with your app’s design. However, avoid over-customizing to maintain the familiar interface users expect.
- Thoroughly test the QuickLook integration on different devices and with various file types to ensure consistency and reliability.
Here’s an example of how you can load files asynchronously for QuickLook:
DispatchQueue.global(qos: .userInitiated).async { let previewItemURL = self.prepareFileForPreview() DispatchQueue.main.async { self.presentPreview(previewItemURL) } } func presentPreview(_ url: URL) { guard let previewController = QLPreviewController(dataSource: self) else { return } // Set up previewController if needed present(previewController, animated: true) }
And here’s an example of error handling:
func previewController(_ controller: QLPreviewController, previewItemAt index: Int) -> QLPreviewItem { guard let previewItem = items[safe: index] else { // Handle the error appropriately print("Error: Requested item index is out of bounds") return DummyPreviewItem() // Return a dummy item or handle as needed } return previewItem }
By following these best practices, you can leverage the full potential of QuickLook in your Swift applications, providing a seamless and efficient preview experience for your users.
Source: https://www.plcourses.com/swift-and-quicklook/