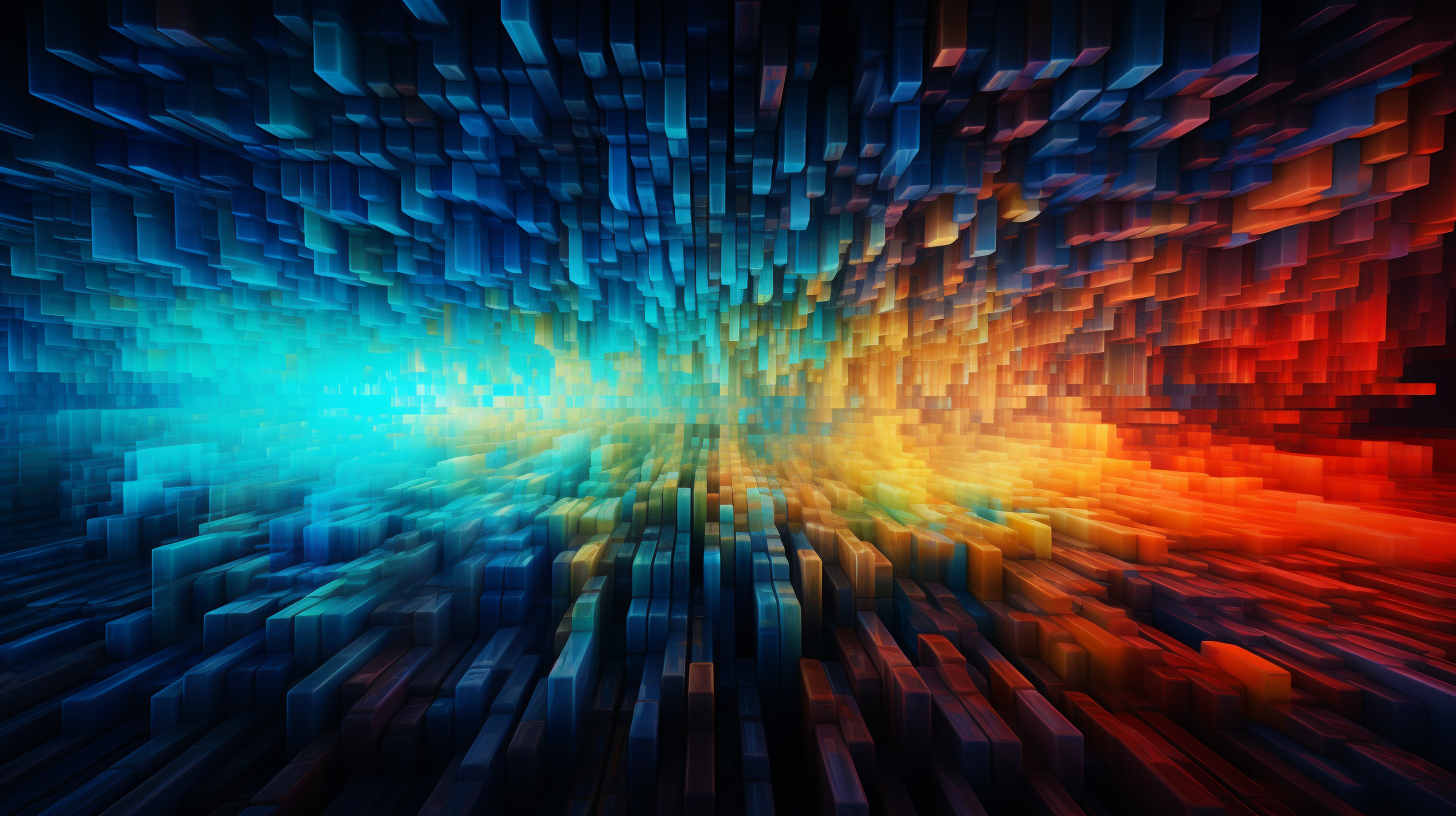
In Python, regular expressions (often shortened to regex) are sequences of characters used to match character combinations in strings. They’re incredibly useful for extracting information from text such as code, files, logs, or even documents. One of the functions provided by Python’s re
module is re.fullmatch()
, which checks if the entire string matches the regular expression pattern provided.
The re.fullmatch()
function is used when you want to ensure that the entire string conforms to a particular pattern, rather than just containing a substring that fits the pattern. This means that if the string has additional characters before or after the pattern, re.fullmatch()
will not consider it a match.
This function is particularly useful when validating inputs, such as checking if a user’s input is exactly what’s expected. For example, it is commonly used in validating emails, phone numbers, or user IDs.
Let’s consider a scenario where you want to validate a string to ensure it’s exactly an alphanumeric word. With re.fullmatch()
, you can use the pattern 'w+'
which means “one or more word characters”. If you try to match this pattern against the string “Hello123”, re.fullmatch()
will return a match object since the entire string is an uninterrupted sequence of word characters.
import re pattern = r'w+' string = 'Hello123' match = re.fullmatch(pattern, string) if match: print("The string is an alphanumeric word.") else: print("The string is not an alphanumeric word.")
In contrast, if the string has additional characters that are not word characters, like spaces or punctuation, re.fullmatch()
will not find a match.
Understanding how re.fullmatch()
works is important for any Python developer, especially when dealing with data validation and processing. In this article, we’ll delve deeper into the syntax, parameters, and provide examples of how to use re.fullmatch()
in real-world scenarios.
Syntax and Parameters
The syntax for re.fullmatch()
is simpler. It takes two mandatory arguments: the pattern and the string, and an optional flags argument. The pattern is the regular expression to be matched, and the string is the text in which you are searching for a match. The flags argument is used to modify the behavior of the pattern matching.
Here’s the basic syntax:
import re match = re.fullmatch(pattern, string, flags=0)
The pattern argument is a string that contains the regular expression. In Python, regular expressions use the backslash character (”) to indicate special forms or to allow special characters to be used without invoking their special meaning. This can lead to confusion with Python’s usage of the same character for the same purpose in string literals. To avoid this, raw strings are often used by prefixing the string with ‘r’ or ‘R’. This tells Python not to handle backslashes in any special way.
The string argument is the text you want to search for the pattern. If the pattern is found in the string, re.fullmatch()
returns a match object. Otherwise, it returns None
.
The optional flags argument can be used to modify the behavior of the regular expression. This argument can be a combination of the following values:
re.IGNORECASE
(orre.I
) – Makes the pattern case-insensitive.re.MULTILINE
(orre.M
) – Allows the start and end metacharacters (^ and $) to match at the beginning and end of each line instead of the beginning and end of the string.re.DOTALL
(orre.S
) – Allows the dot (.) to match any character, including a newline.re.ASCII
(orre.A
) – Makes the w, W, b, B, d, D, s and S sequences only match ASCII characters, rather than all unicode characters.re.LOCALE
(orre.L
) – No effect in Python 3, as locale-aware matching is deprecated.re.VERBOSE
(orre.X
) – Allows you to write regular expressions that are more readable by granting you more whitespace and comments.
Here’s an example that uses the re.IGNORECASE
flag:
import re pattern = r'hello' string = 'Hello World' match = re.fullmatch(pattern, string, flags=re.IGNORECASE) if match: print("The string matches the pattern, ignoring case.") else: print("The string does not match the pattern.")
It’s important to note that re.fullmatch()
requires the pattern to match the whole string and not just a subset of it. That’s the main difference between re.fullmatch()
and re.match()
, which only requires the beginning of the string to match the pattern.
Examples of Using re.fullmatch
Now let’s look at some practical examples where re.fullmatch()
can be applied in real-world scenarios.
Suppose you are creating a sign-up form and you need to ensure that the user’s password meets certain criteria. For instance, the password must be at least 8 characters long, include one uppercase letter, one lowercase letter, and one digit. You can use re.fullmatch()
to validate the password against a regex pattern that represents these rules.
import re pattern = r'^(?=.*[a-z])(?=.*[A-Z])(?=.*d)[A-Za-zd]{8,}$' password = 'Password123' match = re.fullmatch(pattern, password) if match: print("The password is valid.") else: print("The password is invalid.")
In this example, the pattern uses a combination of positive lookaheads to ensure that at least one lowercase letter, one uppercase letter, and one digit are present. It then specifies that the total length of the password should be at least 8 characters.
Another common use case is verifying the format of date strings. For example, if you expect the date to be in the format ‘YYYY-MM-DD’, you can use re.fullmatch()
to check if a given date string matches this pattern.
import re pattern = r'd{4}-d{2}-d{2}' date_string = '2021-03-15' match = re.fullmatch(pattern, date_string) if match: print("The date format is correct.") else: print("The date format is incorrect.")
This pattern ensures that the year is four digits, followed by a hyphen, two digits for the month, another hyphen, and two digits for the day.
Lastly, if you are working with URL validation, re.fullmatch()
can be a powerful tool to match against a complex pattern that describes a valid URL format.
import re pattern = r'(https?://)?(www.)?([A-Za-z0-9-]+).([a-z]{2,6})(/[A-Za-z0-9-]*)*/?$' url = 'https://www.example.com/path-to-page' match = re.fullmatch(pattern, url) if match: print("The URL is valid.") else: print("The URL is invalid.")
Here, the pattern checks for the optional ‘http://’ or ‘https://’, an optional ‘www.’, followed by the domain name and extension. The pattern also considers the path and trailing slash as optional.
These examples demonstrate the versatility of re.fullmatch()
in ensuring that entire strings adhere to specific formats, which is a vital aspect of data validation in programming.
Limitations and Considerations
While re.fullmatch()
is a powerful tool for full string matching, there are certain limitations and considerations that developers should be aware of when using this function.
Limitation of Pattern Complexity: The more complex the regex pattern, the harder it is to read and maintain. Overly complex patterns can lead to decreased code readability and potential errors. It’s often best to break down complex patterns into simpler, more manageable ones.
Performance Considerations: Regex operations can be slow, especially with large strings or complex patterns. When working with large datasets or in performance-critical applications, it is important to ponder the impact of using re.fullmatch()
and optimize the regex pattern as much as possible.
Unicode Handling: By default, re.fullmatch()
will match any Unicode characters. However, if you are working with ASCII data, you can use the re.ASCII
flag to limit matches to ASCII characters only. This can be important when working with data that is expected to be in a specific character set.
import re pattern = r'w+' string = 'Café' # Without the re.ASCII flag, this will match because 'é' is a Unicode word character match = re.fullmatch(pattern, string) if match: print("The string is an alphanumeric word (Unicode).") else: print("The string is not an alphanumeric word (Unicode).") # With the re.ASCII flag, this will not match because 'é' is not an ASCII word character match = re.fullmatch(pattern, string, flags=re.ASCII) if match: print("The string is an alphanumeric word (ASCII).") else: print("The string is not an alphanumeric word (ASCII).")
Matching Line Breaks: If you need to match patterns that may include line breaks, think using the re.DOTALL
flag, which allows the dot (.) metacharacter to match any character including a newline.
Lookahead and Lookbehind Assertions: While re.fullmatch()
is great for validating full strings, it does not support variable-length lookbehind assertions, which can limit its use in certain scenarios where you need to check for preceding patterns of variable length.
In conclusion, while re.fullmatch()
is a valuable function for validating that an entire string matches a pattern, it’s important to be mindful of the limitations and think the performance and readability of your regex patterns. By understanding these considerations, you can use re.fullmatch()
effectively in your Python projects.
Conclusion and Best Practices
As with all tools in a developer’s toolbox, using re.fullmatch()
effectively requires understanding when and how to apply it. Here are some best practices to keep in mind:
- While it may be tempting to use a single complex regex to match a pattern, it is often more readable and maintainable to split your pattern into more manageable parts or to use multiple simpler regex checks.
- Prefix your pattern strings with
r
to avoid confusion with Python’s string escape sequences. This can save you from hard-to-find bugs in your pattern matching. - Use the
re.VERBOSE
flag to write multi-line regex patterns with comments. This can greatly enhance the readability of complex patterns and make your code easier to understand for other developers. - Regex patterns can be tricky to get right. Always test your patterns against a variety of strings to ensure they match exactly what you expect and nothing more.
- If you’re using the same pattern multiple times, think precompiling it with
re.compile()
for better performance. - User-provided regex patterns can lead to security vulnerabilities such as ReDoS (Regular Expression Denial of Service). Validate and sanitize any user input used as part of a regex pattern.
Here’s an example of how you might use re.fullmatch()
with some of these best practices in mind:
import re # Precompiled pattern for email validation email_pattern = re.compile( r""" ^ # start of string [a-zA-Z0-9._%+-]+ # username part @ # symbol [a-zA-Z0-9.-]+ # domain part .[a-zA-Z]{2,4} # top-level domain $ # end of string """, re.VERBOSE ) email = '[email protected]' match = email_pattern.fullmatch(email) if match: print("The email is valid.") else: print("The email is invalid.")
By following these best practices, you can make the most of re.fullmatch()
and ensure that your Python code remains clean, efficient, and secure.
Source: https://www.pythonlore.com/exploring-re-fullmatch-for-full-string-matching/