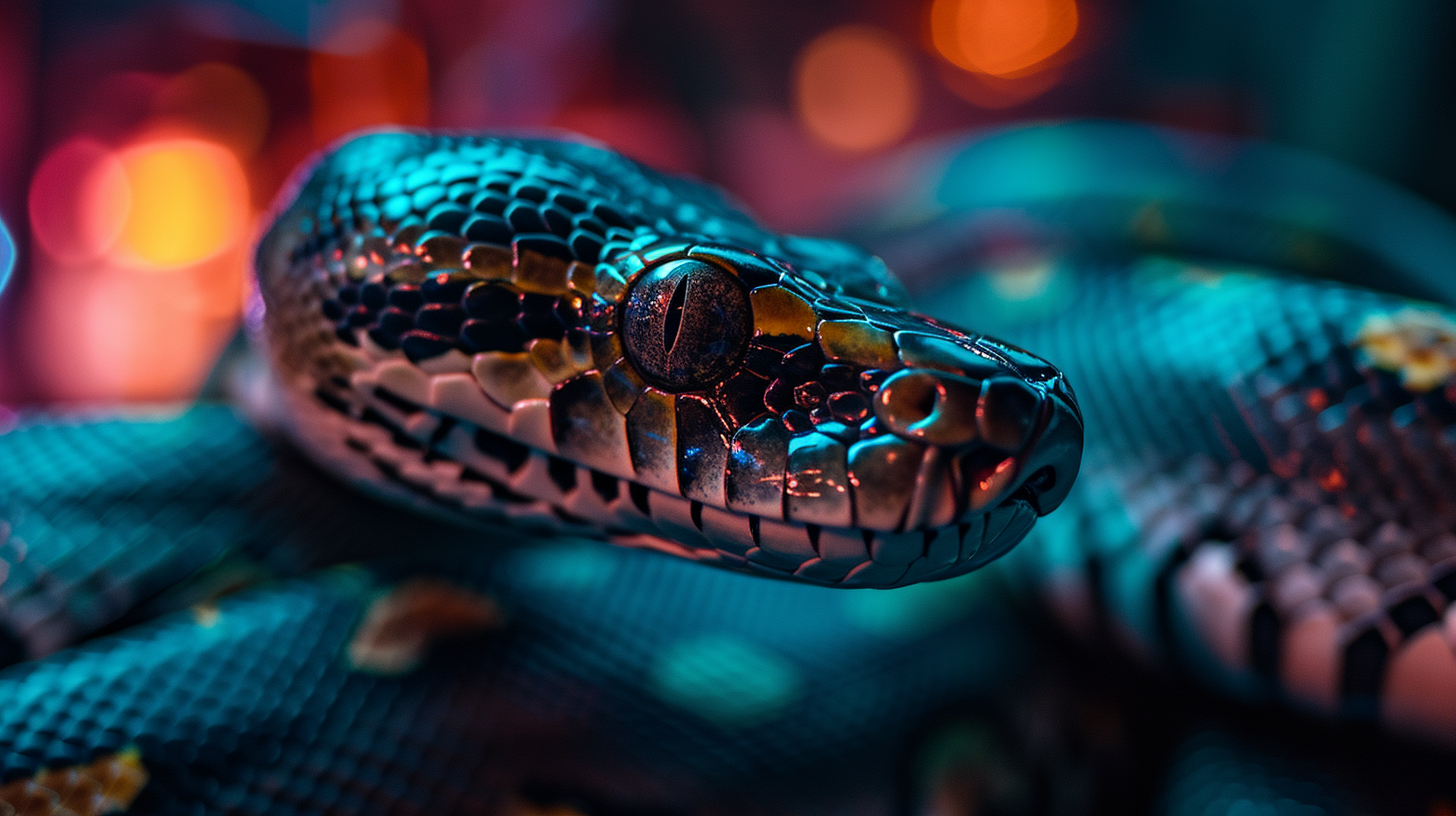
When working with sockets in Python, it’s essential to properly close and release the resources once your work is done. Sockets are a limited system resource, and leaving them open can lead to resource leaks, which could eventually exhaust the system’s available sockets and cause your program or even the entire system to become unstable.
Closing a socket is a two-step process. The first step is to shut down the connection, and the second step is to release the socket resources. It is important to understand that just shutting down the connection does not immediately release the resources. The socket remains in a TIMED_WAIT state for a certain period, ensuring that all the data is sent and acknowledged. Only after this state can the resources be fully released.
The socket.close()
method in Python is used to close the socket and release the resources. However, simply calling socket.close()
does not guarantee that all the data has been sent or that the resources are released immediately. There are best practices and error handling techniques that need to be applied when using socket.close()
to ensure that resources are properly managed.
Here is a basic example of how to close a socket in Python:
import socket # Create a socket object s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Establish a connection s.connect(('hostname', port)) # Perform socket operations # ... # Close the socket s.close()
This simple example demonstrates the use of socket.close()
to close the connection. However, there are additional considerations and steps you should take to ensure that all resources are properly released, which we will explore in the following sections.
Closing a Socket Connection
Before invoking socket.close()
, it’s a good practice to shut down the socket connection for both reading and writing using socket.shutdown()
. This helps to make sure that all data is flushed and no more I/O operations are allowed on the socket. Here is an example:
# Shutting down the socket s.shutdown(socket.SHUT_RDWR)
After shutting down the connection, you can then proceed to close the socket. The socket.close()
method frees up the resources associated with the socket immediately. Here is the updated code:
# Shutting down and closing the socket s.shutdown(socket.SHUT_RDWR) s.close()
It is important to note that if you’re working with a non-blocking socket, you may encounter a socket.error
exception when you perform a shutdown. This could happen if there are no more data on the socket to be read or written. You should be prepared to catch this exception to prevent your program from crashing. Here’s how you could modify the code to handle this:
try: s.shutdown(socket.SHUT_RDWR) except socket.error as e: print('Socket shutdown error:', e) finally: s.close()
By using the try...except...finally
block, you ensure that the s.close()
is always executed, even if there’s an error during the shutdown process.
When the socket is closed using socket.close()
, it cannot be reused. If you try to use the socket after calling close()
, you will get a socket.error
exception. This is why it’s crucial to make sure that you are completely done with the socket operations before closing it.
Lastly, it is worth mentioning that if you are using a context manager with your socket, the socket.close()
method will be called automatically when the block is exited, thus simplifying resource management:
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s: s.connect(('hostname', port)) # Perform socket operations # ... # No need to manually close the socket
Using a context manager is a recommended way to handle sockets as it ensures that the socket is properly closed regardless of whether an exception occurs during socket operations.
Releasing Socket Resources
In addition to the socket.close() method, it very important to understand that the underlying operating system may still hold onto the socket resources for a short period after the close() method has been invoked. That’s due to the TCP protocol’s design, which requires a waiting period to ensure all data has been transmitted and acknowledged. This period is known as TIME_WAIT.
During TIME_WAIT, the socket is still consuming system resources, which can be problematic if your application frequently opens and closes sockets. To mitigate this, you can set the SO_REUSEADDR socket option before binding the socket. This allows the socket to be bound to an address that’s in the TIME_WAIT state, which can be useful in situations where you need to restart a service that binds to a specific port.
# Enabling SO_REUSEADDR option s.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
However, use the SO_REUSEADDR option with caution, as it can lead to security risks and unexpected behavior if not used correctly, especially in a production environment.
Another aspect of releasing socket resources is ensuring that no references to the socket object remain in your code after calling socket.close(). In Python, objects are garbage collected when there are no more references to them. If you inadvertently hold a reference to a socket object, it may not be collected immediately, and the resources will not be released.
To avoid this, make sure to delete any references to the socket object or set them to None
after closing the socket:
s.close() s = None
By following these additional considerations for releasing socket resources, you can ensure that your Python applications manage sockets efficiently and avoid potential resource leaks.
Best Practices for Using socket.close
It is also a best practice to handle exceptions that may occur when closing a socket. Exceptions can be raised for various reasons, such as trying to close a socket that is already closed or facing network issues. Here’s an example of how you could handle such exceptions:
try: s.close() except socket.error as e: print('Socket close error:', e)
By handling exceptions, you can prevent your program from crashing and possibly log the error for further investigation. That’s especially important in a production environment where stability and robustness are crucial.
Moreover, when working with threads or asynchronous operations, it’s important to ensure that the socket is not closed while another thread or operation is still using it. This could cause data loss or corruption. To handle this, you could use synchronization mechanisms such as threading locks to coordinate the closing of the socket:
import threading # Assuming 's' is a shared socket object lock = threading.Lock() # When closing the socket with lock: s.shutdown(socket.SHUT_RDWR) s.close()
To wrap it up, closing and releasing socket resources properly requires careful consideration of the socket state, exception handling, and synchronization in multi-threaded environments. By following these best practices and using the socket.close()
method correctly, you can ensure that your Python programs manage network resources effectively and maintain system stability.
Handling Errors and Exceptions with socket.close
When dealing with errors and exceptions during the use of socket.close()
, it’s important to have a robust error handling strategy in place. This becomes even more critical in network programming, where unexpected events such as network failures, timeouts, or even improper use of the socket API can occur. Python’s socket module provides a way to handle these exceptions to ensure that your program remains stable and reliable.
One common issue that may arise when calling socket.close()
is trying to close a socket that has already been closed. This will raise a socket.error
exception. To handle this, you can check the socket’s state before attempting to close it:
if not s._closed: s.close()
Another potential error is when there are still data being sent over the socket when you attempt to close it. This can be mitigated by ensuring that all data has been sent and acknowledged before calling socket.close()
. You can use the socket.settimeout()
method to specify a timeout period for socket operations to prevent the program from hanging indefinitely:
s.settimeout(5.0) # Set timeout to 5 seconds try: # Perform socket operations # ... finally: s.close()
If a timeout occurs or any other exception is raised, you can catch it and handle it appropriately:
try: # Perform socket operations # ... except socket.timeout as e: print('Socket operation timed out:', e) except socket.error as e: print('Socket error:', e) finally: s.close()
It’s also possible for a socket.error
to be raised if there is an issue with the network connection, such as a reset connection. In such cases, it is important to log the error and perform any necessary cleanup:
try: # Perform socket operations # ... except socket.error as e: print('Network error occurred:', e) # Additional cleanup if necessary finally: s.close()
In summary, handling errors and exceptions with socket.close()
is about anticipating what can go wrong and being prepared to handle such situations gracefully. Proper error handling ensures that your program does not crash unexpectedly and maintains the integrity of the resources it uses. By including these error handling techniques in your socket programming, you can build more robust and reliable network applications in Python.
Source: https://www.pythonlore.com/closing-and-releasing-resources-with-socket-close/