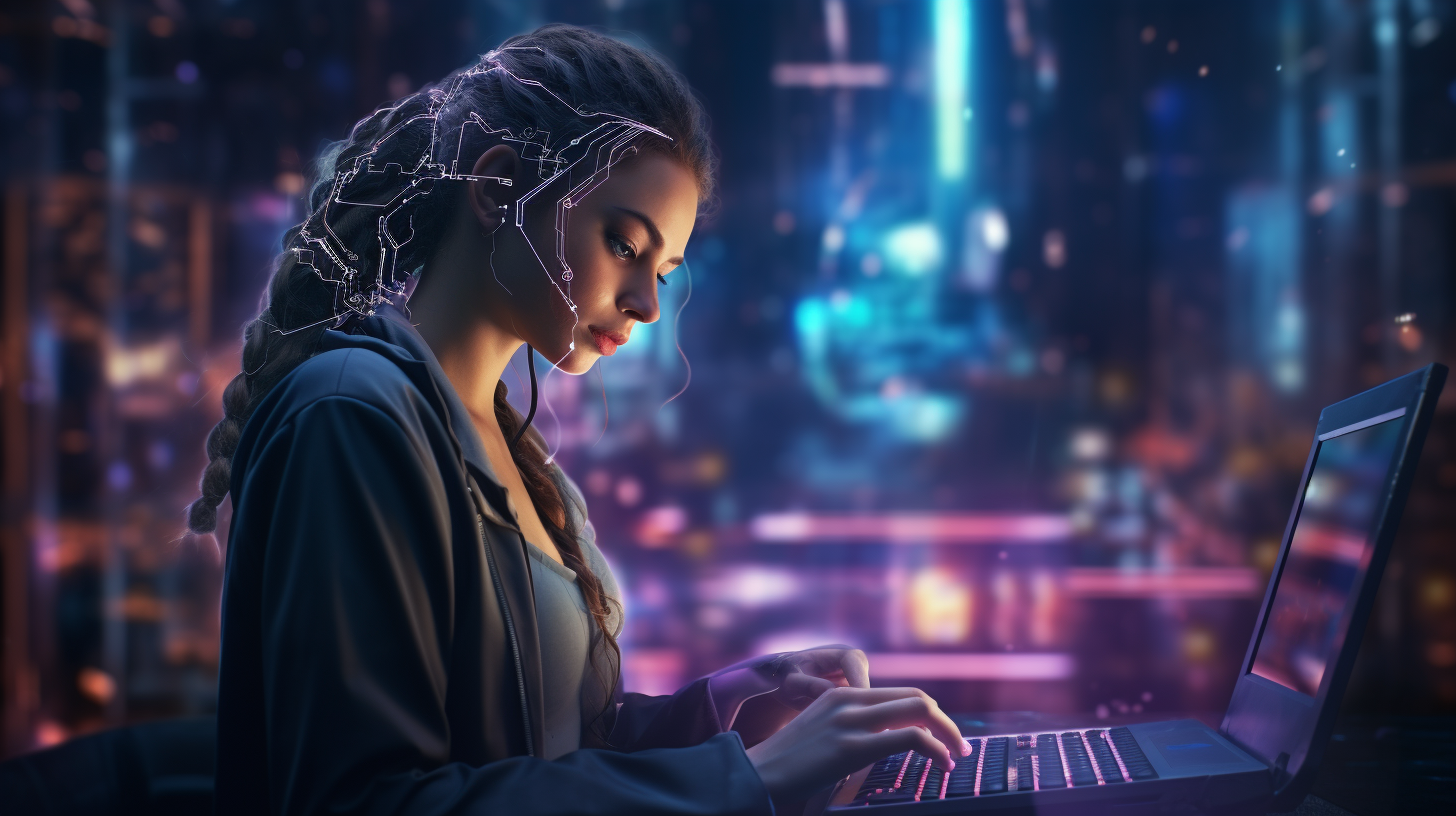
In the field of computer graphics, 3D modeling is a technique used to create and manipulate three-dimensional representations of objects. Python, a versatile programming language, can be utilized to assist in various aspects of 3D modeling. In this article, we will explore the different techniques and tools available for working with 3D modeling using Python.
Blender
Blender is a popular open-source 3D computer graphics software tool that offers a comprehensive set of features for modeling, animation, rendering, and more. Blender provides a Python API (Application Programming Interface) that allows developers to interact with and extend its functionalities programmatically.
To work with Blender using Python, you need to have both Blender and Python installed on your system. Once you have them set up, you can start exploring the world of 3D modeling with Python!
Working with 3D Models in Blender using Python
Let’s dive into some of the fundamental tasks you can accomplish with Python in Blender.
Importing 3D Models
Blender supports various file formats for importing 3D models, such as .obj
, .fbx
, .stl
, and more. Using Python, you can automate the process of importing 3D models into Blender. Here’s an example:
import bpy path_to_model = "path/to/model.obj" bpy.ops.import_scene.obj(filepath=path_to_model)
Modifying 3D Models
Python allows you to manipulate 3D models within Blender programmatically. You can access various properties of objects, such as vertices, edges, and faces, to modify them. Here’s an example where we translate an object along the X-axis:
import bpy obj = bpy.context.object obj.location.x += 1.0
Creating 3D Models
You can also create 3D models from scratch using Python in Blender. For example, here’s how you can create a simple cube:
import bpy bpy.ops.mesh.primitive_cube_add(size=2.0, location=(0, 0, 0))
Rendering 3D Models
Blender excels at rendering realistic images and animations of 3D models. Using Python, you can automate the rendering process and adjust various rendering settings. Here’s an example:
import bpy scene = bpy.context.scene # Set rendering engine to Cycles scene.render.engine = 'CYCLES' # Set number of samples scene.cycles.samples = 100 # Render the scene bpy.ops.render.render(write_still=True)
Python Libraries for 3D Modeling
In addition to Blender’s Python API, there are other Python libraries available that can assist in 3D modeling. Let’s explore two popular ones:
Open3D
Open3D is a state-of-the-art library for 3D data processing. It provides functionality for working with point clouds, meshes, and other 3D representations. Open3D is designed to be easy to use, efficient, and portable. Here’s an example of how to visualize a point cloud using Open3D:
import open3d as o3d # Load point cloud from file point_cloud = o3d.io.read_point_cloud("path/to/point_cloud.ply") # Visualize the point cloud o3d.visualization.draw_geometries([point_cloud])
PyVista
PyVista is another powerful library for 3D data processing and visualization. It provides a Pythonic interface to various 3D file formats and features advanced functionalities for working with meshes, grids, and point clouds. Here’s an example of creating a structured grid using PyVista:
import pyvista as pv # Create a structured grid grid = pv.UniformGrid() grid.dimensions = (10, 10, 10) grid.origin = (0, 0, 0) grid.spacing = (1, 1, 1) # Plot the grid grid.plot(show_edges=True)
Python provides powerful tools and libraries for working with 3D modeling. Whether you’re using Blender’s Python API or using external libraries like Open3D and PyVista, Python allows you to automate tasks, manipulate models, create new ones, and render stunning visuals. With Python as your ally, the possibilities in the realm of 3D modeling are endless!
References:
- //www.blender.org/”>Blender
- //open3d.org/”>Open3D
- //docs.pyvista.org/”>PyVista
Code snippets are from official documentation and examples.
Source: https://www.plcourses.com/python-and-3d-modeling-techniques-and-tools/