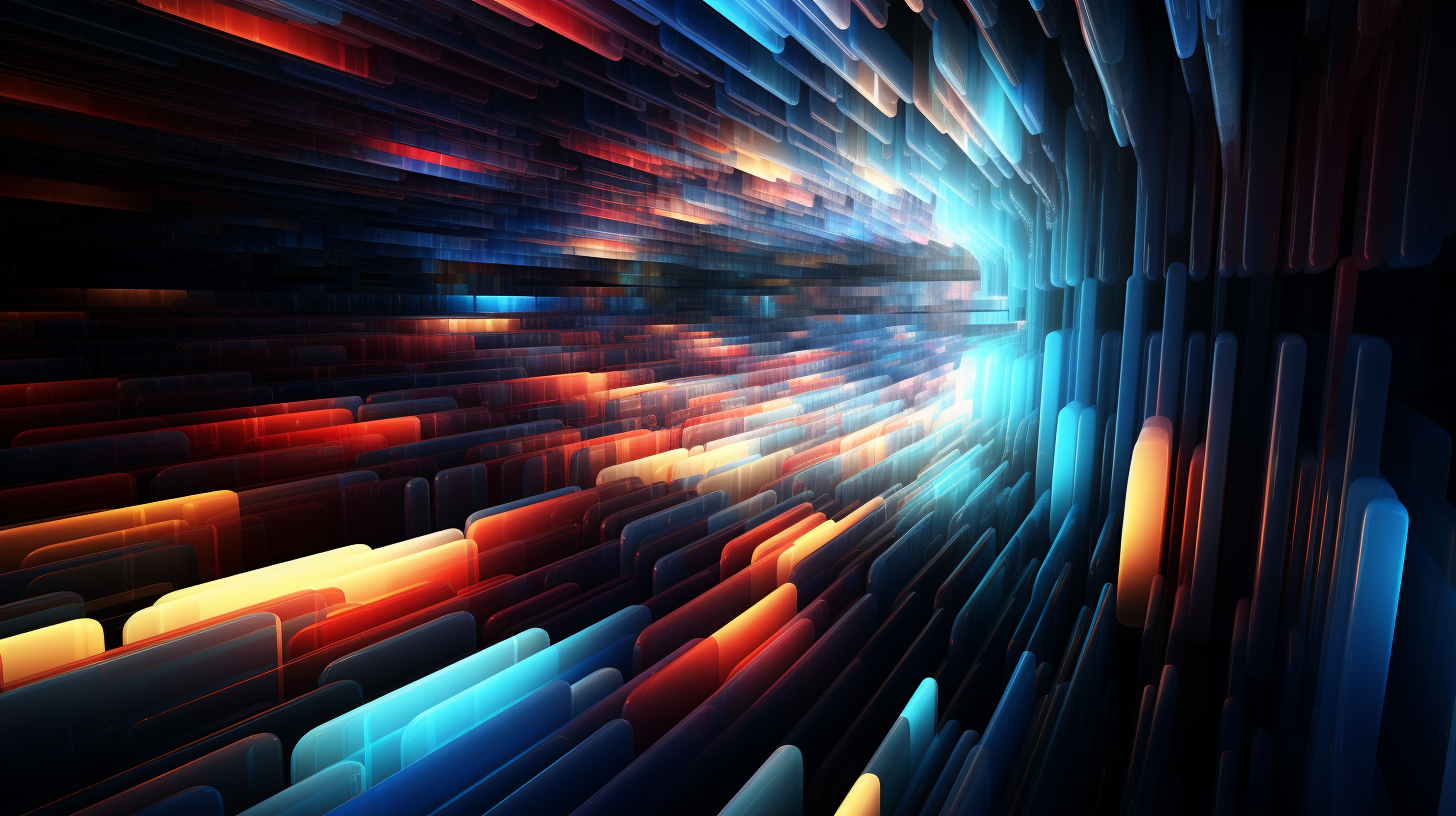
Introduction to Interactive Maps
Interactive maps have transformed the way we visualize and interact with geographical data. They allow users to zoom in and out, pan across different regions, and click on specific areas to get more information. This level of interactivity is made possible through JavaScript, which is a powerful programming language that enables dynamic content on web pages.
At its core, an interactive map is simply a web-based map that responds to user actions. This could be as simple as changing the color of a country when it is clicked, or as complex as displaying real-time data overlays based on user inputs. JavaScript, together with HTML and CSS, makes these dynamic features possible.
One of the most popular libraries for creating interactive maps in JavaScript is Leaflet. It’s an open-source library that provides a wide range of features for building rich, interactive maps. Here’s an example of how to create a basic interactive map using Leaflet:
// Include the Leaflet library // Initialize the map and set its view to our chosen geographical coordinates and a zoom level var mymap = L.map('mapid').setView([51.505, -0.09], 13); // Add a tile layer to add to our map, in this case, it's a Mapbox Streets tile layer L.tileLayer('https://api.mapbox.com/styles/v1/{id}/tiles/{z}/{x}/{y}?access_token={your.mapbox.access.token}', { maxZoom: 18, id: 'mapbox/streets-v11', tileSize: 512, zoomOffset: -1, accessToken: 'your.mapbox.access.token' }).addTo(mymap);
This code snippet creates a basic map centered on London, with zoom controls and a street view provided by Mapbox. However, the true power of interactive maps lies in their ability to add layers and controls that respond to user actions.
For example, you can add a marker to the map that, when clicked, displays a popup with more information:
var marker = L.marker([51.5, -0.09]).addTo(mymap); marker.bindPopup("Hello world!
I am a popup.").openPopup();
Or you can add a layer that shows the user’s current location:
mymap.locate({setView: true, maxZoom: 16}); mymap.on('locationfound', function(e){ var marker = L.marker([e.latitude, e.longitude]).addTo(mymap); marker.bindPopup("You are here!").openPopup(); });
These are just a few examples of the interactive features that can be implemented using JavaScript. In the following sections, we will delve deeper into how to implement these features and the best practices to keep in mind when creating dynamic maps.
Implementing JavaScript for Interactive Features
Implementing JavaScript for interactive features on maps requires a good understanding of event handling and the DOM (Document Object Model). JavaScript provides a variety of methods for interacting with HTML elements and responding to user actions such as clicks, mouse movements, and keyboard input. Here are some key concepts to ponder when adding interactive elements to your maps:
- JavaScript allows you to add event listeners to HTML elements. You can use these to trigger functions when a user interacts with your map. For example, you can use the
addEventListener()
method to listen for a click event on a map marker and display information related to that marker.
marker.addEventListener('click', function() { alert('Marker clicked!'); });
function updatePopupContent(content) { var popup = document.getElementById('info-popup'); popup.innerHTML = content; }
var baseLayers = { "Street View": streets, "Satellite View": satellite }; var overlays = { "Points of Interest": poiLayer, "Traffic": trafficLayer }; L.control.layers(baseLayers, overlays).addTo(mymap);
Implementing these interactive features may require some additional libraries or plugins, depending on the complexity of the map and the specific functionality you want to achieve. For example, you might use the leaflet-draw
plugin to allow users to draw shapes on the map, or the leaflet-geocoder
plugin to add a search bar for address lookups.
Remember, the key to a successful interactive map is not just the technology behind it, but the user experience it provides. Always consider the end-user when implementing interactive features, and strive to make the map as intuitive and simple to operate as possible.
Best Practices for Creating Dynamic Maps
When creating dynamic maps, it’s essential to follow best practices that ensure your maps are not only interactive but also performant, accessible, and simple to operate. Here are some crucial best practices to keep in mind:
- Interactive maps can quickly become resource-intensive, especially when dealing with large datasets or multiple layers. To optimize performance, think using techniques such as lazy loading, where map assets are only loaded when needed, and reducing the number of active layers at any given time.
// Example: Lazy loading a map layer var lazyLayer = L.tileLayer.lazy('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png'); mymap.addLayer(lazyLayer);
// Example: Adding alternative text to a map marker var marker = L.marker([51.5, -0.09], { alt: "Marker representing a location on the map" }).addTo(mymap);
By following these best practices, you’ll create dynamic maps that are not only interactive but also reliable and simple to operate. Always keep in mind the end goal of providing a seamless experience for your users, and continually test and refine your maps to meet their needs.
Source: https://www.plcourses.com/javascript-and-interactive-maps/