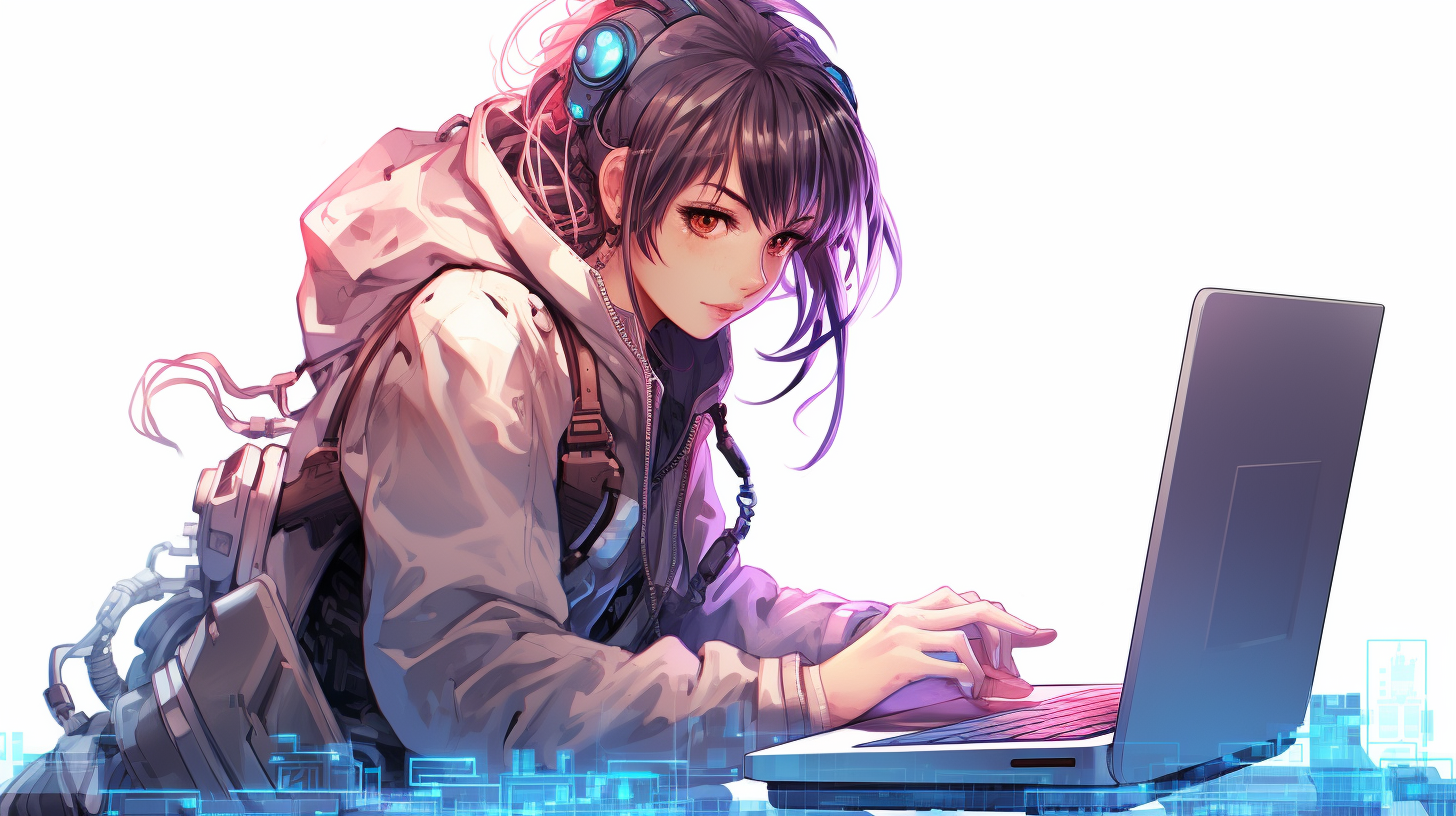
If you’re an app developer, you know that testing your mobile applications is essential before releasing them to the public. One of the challenges in mobile app development is automating tests to ensure that your app functions properly across different devices and platforms.
One tool that can greatly assist in mobile app testing is ADB (Android Debug Bridge). ADB is a powerful command-line tool that allows you to communicate with an Android device or emulator from your development machine.
In this tutorial, we will explore how you can leverage Bash scripting to automate mobile app testing using ADB. Bash is the default shell for most Unix-like operating systems and provides a rich set of features for scripting.
Installing ADB
Before we dive into the Bash scripting part, let’s make sure ADB is installed on your development machine. ADB comes bundled with the Android SDK platform tools, so you’ll need to have the Android SDK installed first.
You can check if ADB is already installed by opening a terminal and running the following command:
adb version
If you see the version information, ADB is already installed. If not, you can follow the official Android documentation to install the Android SDK and set up ADB on your machine.
Writing a Basic Bash Script
Let’s start by writing a basic Bash script that connects to an Android device using ADB and retrieves the list of installed applications on the device. Create a new file called list_apps.sh
and open it in a text editor.
#!/bin/bash # Connect to device using ADB adb connect # Get list of installed applications adb shell pm list packages
In the above script, we start by adding a shebang
directive (#!/bin/bash
) at the beginning of the file. This informs the system that the script should be executed using the Bash shell.
Next, we use the adb connect
command to establish a connection to the Android device. Replace with the IP address of your device. Ensure that your Android device is connected to the same network as your development machine.
Finally, we use the adb shell pm list packages
command to retrieve the list of installed applications on the device. This command lists all the package names of installed apps.
Executing the Bash Script
Now that we’ve written the Bash script, let’s execute it and see the results. Open a terminal, navigate to the directory where you saved the list_apps.sh
file, and run the following command:
bash list_apps.sh
If everything is set up correctly, you should see the list of installed application package names printed in the terminal.
Passing Arguments to the Bash Script
Often, you’ll need to pass arguments to your Bash script to customize its behavior. For example, you might want to specify the device IP address as a command-line argument instead of hardcoding it in the script.
Here’s an updated version of the list_apps.sh
script that accepts the device IP address as an argument:
#!/bin/bash # Connect to device using ADB adb connect # Get list of installed applications adb shell pm list packages
In the updated script, represents the first command-line argument passed to the script. When executing the script, you can now provide the device IP address as an argument:
bash list_apps.sh
Iterating Over a List of Devices
When testing on multiple devices, you might want to iterate over a list of device IP addresses and perform the same set of operations on each device. This can be achieved using a Bash for
loop.
Here’s an example script that connects to multiple devices using ADB and retrieves the list of installed applications on each device:
#!/bin/bash # List of device IP addresses devices=("192.168.1.101" "192.168.1.102" "192.168.1.103") # Iterate over devices for device in ${devices[@]}; do # Connect to device using ADB adb connect $device # Get list of installed applications adb shell pm list packages echo "---------------------------------------" done
In the above script, we define an array called devices
that contains the IP addresses of the devices we want to connect to. Then, we use a for
loop to iterate over each device in the array.
Inside the loop, we connect to each device using ADB, retrieve the list of installed applications, and print a separator line before moving on to the next device.
To run the script, simply execute it in the terminal:
bash list_apps_multi_devices.sh
You should see the list of installed application package names for each device, separated by separator lines.
Bash scripting can greatly simplify and automate various tasks in mobile app development, especially when it comes to testing and interacting with Android devices using ADB. In this tutorial, we explored how to write basic Bash scripts to connect to an Android device and retrieve the list of installed applications.
With this foundation, you can now expand your Bash scripts to perform more complex operations, such as installing/uninstalling apps, capturing screenshots, running tests, and much more. The possibilities are endless!
Remember to refer to the official Android documentation and ADB documentation for more details on the available ADB commands and options.
Happy scripting!
Source: https://www.plcourses.com/bash-scripting-for-mobile-app-development/