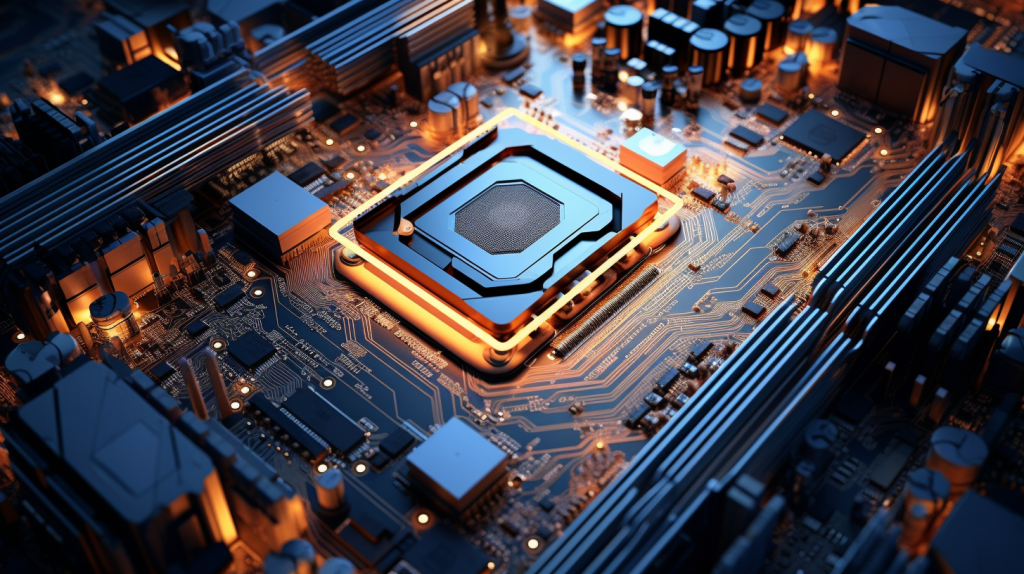
Java methods are an essential part of Java programming, as they help to organize and structure the code in a logical and manageable way. A method in Java is a block of code that performs a specific task and can be executed when called upon. It allows for code reuse, which means that the same method can be used in different parts of the program without the need to rewrite the same code again.
Methods in Java can be classified into two categories: predefined methods and user-defined methods. Predefined methods are those that are provided by Java libraries, such as System.out.println()
which is used to print output to the console. User-defined methods, on the other hand, are created by the programmer to perform specific tasks.
Each method in Java has a method signature which includes the method name, the return type, and the parameter list. The return type specifies the data type of the value that the method returns to the caller. If a method does not return any value, its return type is void
. The parameter list contains the variables that are passed to the method as input.
Here is an example of a simple user-defined method in Java:
public int add(int a, int b) { return a + b; }
In this example, add
is the method name, int
is the return type, and int a, int b
are the parameters. When this method is called, it will return the sum of the two input integers.
Methods in Java can also be overloaded, which means that multiple methods can have the same name but different parameter lists. This allows for methods to be called with different types of inputs. For example:
public int add(int a, int b) { return a + b; } public double add(double a, double b) { return a + b; }
In this case, there are two add
methods: one that takes integer parameters and another that takes double parameters. The Java compiler determines which method to call based on the types of the arguments passed to it.
Methods can also have variable arguments, which allows for a variable number of arguments to be passed. That’s denoted by the ...
syntax. For example:
public int add(int... numbers) { int sum = 0; for (int number : numbers) { sum += number; } return sum; }
In this example, the add
method can take any number of integer arguments, and it will return the sum of all the numbers passed to it.
Methods in Java are a powerful feature that helps to create modular, reusable, and maintainable code. They allow for tasks to be encapsulated in self-contained blocks of code that can be called upon when needed, making the programming process more efficient and organized.
Definition of Java Methods
A Java method is defined as a collection of statements that are grouped together to perform an operation. When you call the method, the statements that are grouped together under the method name are executed. The basic syntax for defining a Java method is as follows:
access_modifier return_type method_name(parameter_list) { // method body }
The access_modifier determines the visibility of the method and can be public
, private
, protected
, or package-private (no explicit modifier). The return_type is the data type of the value that the method returns. If the method does not return a value, the return type is void
. The method_name is the name of the method, which is used to call it. The parameter_list is a comma-separated list of parameters that are passed to the method when it is called.
Here is an example of a Java method that calculates the area of a rectangle:
public double calculateArea(double length, double width) { return length * width; }
In this example, public
is the access modifier, double
is the return type, calculateArea
is the method name, and double length, double width
is the parameter list. When this method is called with length and width values, it will return the area of the rectangle.
Methods can also include various types of parameters, such as:
- Parameters that are declared in the method signature and used within the method body.
- Parameters that are passed to the method when it is called.
For example:
public void greet(String name) { System.out.println("Hello, " + name + "!"); } // Calling the method with an actual parameter greet("Alice");
In this example, String name
is the formal parameter and "Alice"
is the actual parameter.
Methods can also be defined with no parameters:
public void sayHello() { System.out.println("Hello, world!"); }
In this case, the sayHello
method can be called without passing any arguments, and it will print “Hello, world!” to the console.
Defining methods properly very important for creating organized and maintainable code in Java. It allows you to encapsulate functionality and reuse code throughout your program, making it easier to read and debug.
Usage of Java Methods
Java methods are commonly used to perform operations on data, manipulate objects, or handle repetitive tasks. Usage of Java methods can greatly simplify the code and improve its readability. Here are some ways in which methods are used in Java:
- Methods allow you to break down complex problems into smaller, more manageable pieces. This modularity makes it easier to develop, test, and maintain your code.
- Once a method is written, it can be called multiple times from different parts of the program. This reduces duplication and errors.
- Methods provide a layer of abstraction. You can use a method without knowing its internal implementation, as long as you understand its inputs and outputs.
- Methods can be organized into classes based on functionality, which helps in organizing the overall code structure.
Let’s consider an example where we want to calculate the average of an array of numbers. Instead of writing the calculation logic every time we need it, we can create a method:
public double calculateAverage(int[] numbers) { int sum = 0; for (int number : numbers) { sum += number; } return (double) sum / numbers.length; }
Now, we can simply call the calculateAverage
method whenever we need to find the average of an array of integers:
int[] scores = {85, 90, 78, 100, 65}; double averageScore = calculateAverage(scores); System.out.println("The average score is: " + averageScore);
Another usage of methods is to validate user input. For example, we can create a method to check if a user’s input is a valid integer:
public boolean isValidInteger(String input) { try { Integer.parseInt(input); return true; } catch (NumberFormatException e) { return false; } }
Then, we can use this method to ensure that we only process valid integers:
String userInput = "123"; if (isValidInteger(userInput)) { int validNumber = Integer.parseInt(userInput); // Continue processing with the valid number } else { System.out.println("Invalid input. Please enter a valid integer."); }
Methods are a fundamental aspect of Java programming that help in creating organized, reusable, and maintainable code. Understanding how to define and use methods efficiently very important for any Java developer.
Source: https://www.plcourses.com/java-methods-definition-and-usage/