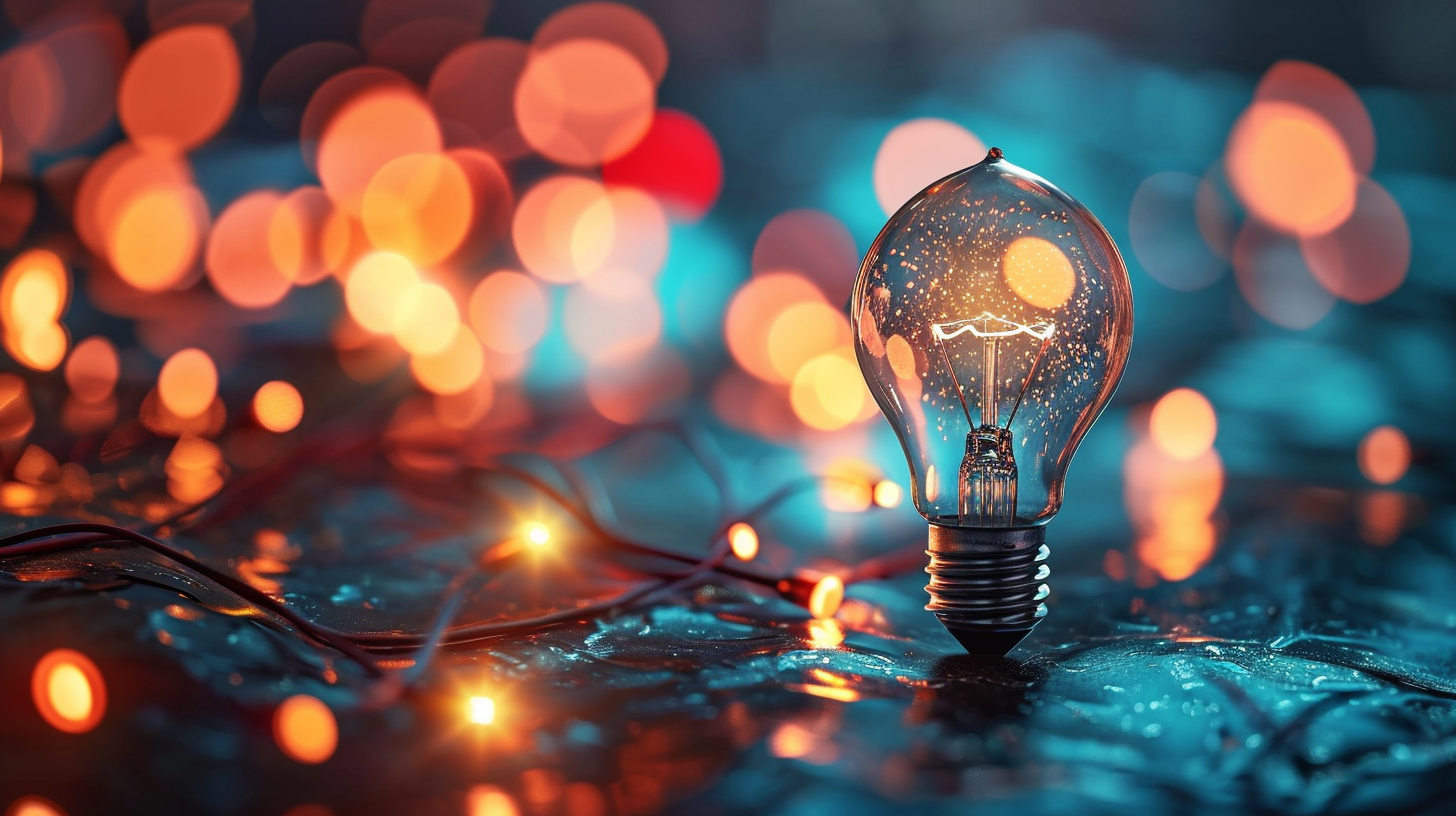
Keras callbacks are functions that are executed at certain points during the training process of a neural network. They provide a way to automate certain tasks that can occur during training, such as saving the model at specific intervals, adjusting the learning rate, or even stopping the training early if the model is not improving. Callbacks are an essential tool in Keras, as they can help you monitor the training process, debug issues, and improve the performance of your model.
There are several built-in callbacks available in Keras, which can be used by simply importing them and adding them to the list of callbacks when fitting the model. Some of the commonly used built-in callbacks include:
- Saves the model after every epoch.
- Stops training when a monitored metric has stopped improving.
- Reduces learning rate when a metric has stopped improving.
- Streams epoch results to a CSV file.
- Enables visualizations for TensorBoard.
These callbacks are used by passing them to the callbacks
parameter in the fit
method of a Keras model. Here is an example of how to use a built-in callback:
from keras.callbacks import EarlyStopping # Define early stopping callback early_stopping = EarlyStopping(monitor='val_loss', patience=3) # Train the model with the callback model.fit(x_train, y_train, validation_data=(x_val, y_val), callbacks=[early_stopping])
While built-in callbacks cover many common scenarios, there are times when you need more control and customization over the training process. That is where custom callbacks come into play. Custom callbacks allow you to define your own logic for what should happen at different stages of the training process.
Building Custom Callbacks in Keras
Building custom callbacks in Keras involves creating a subclass of the Callback
class and then implementing any number of methods that represent the different stages of the training process. These methods include on_train_begin
, on_train_end
, on_epoch_begin
, on_epoch_end
, on_batch_begin
, and on_batch_end
. Each of these methods gets called at their respective points in the training process and can be used to execute custom code.
Let’s build a simple custom callback that prints a message at the start and end of every training epoch:
from keras.callbacks import Callback class CustomCallback(Callback): def on_epoch_begin(self, epoch, logs=None): print(f"Starting epoch {epoch}") def on_epoch_end(self, epoch, logs=None): print(f"Finished epoch {epoch}") # Now we can use our custom callback model.fit(x_train, y_train, validation_data=(x_val, y_val), callbacks=[CustomCallback()])
You can also use the logs dictionary this is passed to some of these methods to access metrics such as loss and accuracy. For example, you might want to implement a custom callback that stops training once the loss goes below a certain threshold:
class CustomEarlyStopping(Callback): def __init__(self, threshold): super(CustomEarlyStopping, self).__init__() self.threshold = threshold def on_epoch_end(self, epoch, logs=None): loss = logs.get('loss') if loss is not None and loss < self.threshold: print(f"Stopping training as loss reached {loss} which is below the threshold") self.model.stop_training = True # Use the custom early stopping callback with a threshold of 0.1 model.fit(x_train, y_train, validation_data=(x_val, y_val), callbacks=[CustomEarlyStopping(threshold=0.1)])
The self.model
attribute within a callback refers to the model this is being trained, and you can use it to modify the behavior of the training process. Setting self.model.stop_training
to True
will stop the training at the end of the current epoch.
These are just simple examples to demonstrate how custom callbacks work. Depending on your specific needs, you can build complex callbacks that monitor and adjust any aspect of the training process in Keras.
Implementing Advanced Monitoring Techniques
Implementing advanced monitoring techniques with custom callbacks in Keras allows for more granular control over the training process. For instance, you can create a callback that dynamically adjusts the learning rate based on certain conditions or one that logs additional information during training for further analysis.
Here is an example of a custom callback that reduces the learning rate when the validation loss plateaus:
from keras.callbacks import Callback import numpy as np class ReduceLROnPlateauCustom(Callback): def __init__(self, monitor='val_loss', factor=0.1, patience=10, min_lr=0.00001): super(ReduceLROnPlateauCustom, self).__init__() self.monitor = monitor self.factor = factor self.patience = patience self.min_lr = min_lr self.wait = 0 self.best = np.Inf def on_epoch_end(self, epoch, logs=None): current = logs.get(self.monitor) if current is None: return if np.less(current, self.best): self.best = current self.wait = 0 else: self.wait += 1 if self.wait >= self.patience: lr = self.model.optimizer.lr.numpy() new_lr = max(lr * self.factor, self.min_lr) self.model.optimizer.lr.assign(new_lr) print(f"Reducing learning rate to {new_lr}.") self.wait = 0 # Use the custom ReduceLROnPlateau callback model.fit(x_train, y_train, validation_data=(x_val, y_val), callbacks=[ReduceLROnPlateauCustom()])
This custom callback monitors the validation loss and reduces the learning rate by a factor of 0.1 if the loss does not improve for 10 consecutive epochs, with a minimum learning rate set to 0.00001.
Another advanced technique is to implement a callback that saves model weights with the best performance based on a custom metric, which is not available in the built-in ModelCheckpoint callback. Here’s how you might implement it:
class CustomModelCheckpoint(Callback): def __init__(self, filepath, monitor='val_custom_metric', mode='max'): super(CustomModelCheckpoint, self).__init__() self.filepath = filepath self.monitor = monitor self.mode = mode if mode == 'min': self.best = np.Inf else: self.best = -np.Inf def on_epoch_end(self, epoch, logs=None): metric = logs.get(self.monitor) if metric is None: return if (self.mode == 'min' and np.less(metric, self.best)) or (self.mode == 'max' and np.greater(metric, self.best)): print(f"Saving model weights as {self.monitor} improved from {self.best} to {metric}.") self.best = metric self.model.save_weights(self.filepath) # Use the custom ModelCheckpoint callback model.fit(x_train, y_train, validation_data=(x_val, y_val), callbacks=[CustomModelCheckpoint(filepath='best_weights.h5')])
With this custom ModelCheckpoint callback, you can save the model weights based on any custom metric that you have implemented, ensuring that the model captures the best possible performance during training.
These are just a few examples of how you can implement advanced monitoring techniques using custom callbacks in Keras. The possibilities are endless, and by using the power of custom callbacks, you can gain deeper insights into your model’s training process and optimize its performance to a greater extent.
Case Study: Using Custom Callbacks for Model Training
Now let’s look at a practical case study where custom callbacks can be particularly useful in the training of a Keras model. Imagine we are working on a complex image classification problem, and we want to monitor not only the loss and accuracy but also the precision and recall for each class. Keras does not provide built-in callbacks that support this level of detailed monitoring, so we will create a custom callback to handle this task.
from keras.callbacks import Callback from sklearn.metrics import classification_report import numpy as np class DetailedMetrics(Callback): def __init__(self, validation_data): super(DetailedMetrics, self).__init__() self.validation_data = validation_data def on_epoch_end(self, epoch, logs=None): val_predict = np.argmax(self.model.predict(self.validation_data[0]), axis=1) val_targ = self.validation_data[1] _val_report = classification_report(val_targ, val_predict) print(f"Classification Report for epoch {epoch}:n{_val_report}")
In this example, we’ve created a DetailedMetrics custom callback that calculates precision, recall, and F1-score for each class at the end of every epoch using the classification_report
function from sklearn.metrics
. The callback requires the validation data to be passed as an argument to its constructor. The precision, recall, and F1-score for each class are printed out at the end of every epoch, providing a detailed view of the model’s performance across all classes.
# Using the custom DetailedMetrics callback during model training model.fit(x_train, y_train, validation_data=(x_val, y_val), callbacks=[DetailedMetrics(validation_data=(x_val, y_val))])
By implementing and using our custom DetailedMetrics callback, we can ensure that our model is not only optimizing for loss and accuracy but also improving its precision and recall for each class, which is critical in a multi-class classification problem.
This case study demonstrates how custom callbacks in Keras can be employed to monitor advanced metrics that are not available in the default callbacks. By creating callbacks tailored to our specific needs, we can enhance our model’s training process and ensure that our neural network performs optimally on the task at hand.
Source: https://www.pythonlore.com/custom-callbacks-in-keras-for-advanced-monitoring/