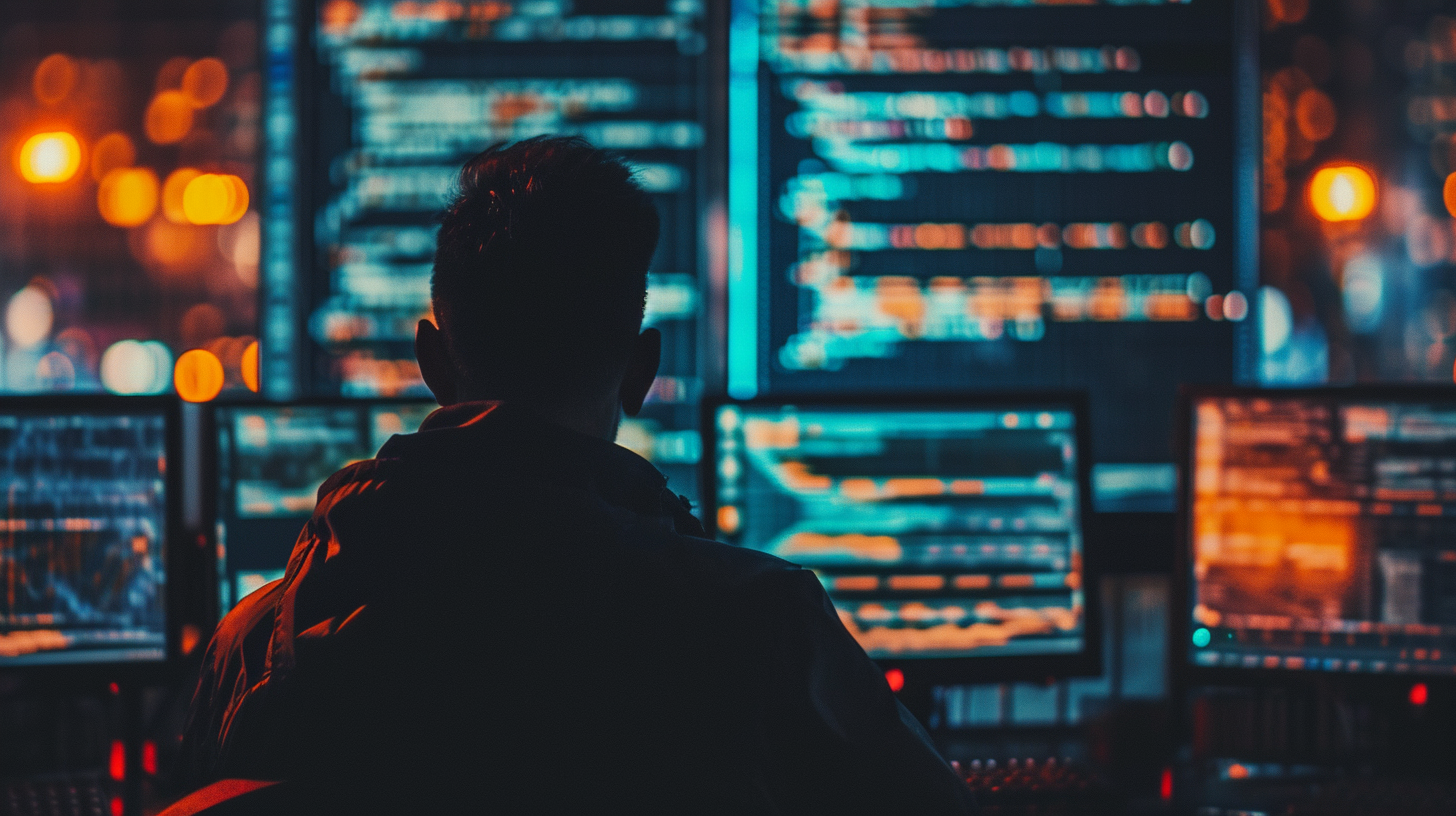
The sys.version
attribute in Python is an essential tool for developers to understand the version of Python they are working with. This attribute is a string that contains the version number of the currently executing Python interpreter, along with additional information such as the build number and date, and the compiler used to build the interpreter.
Accessing this information is straightforward, requiring only a simple import statement for the sys
module and then referencing the version
attribute. Here’s an example:
import sys print(sys.version)
The output from this code snippet will typically look something like this:
3.8.5 (default, Jul 28 2020, 12:59:40)
[GCC 9.3.0]
As we can see, the version string provides a wealth of information. It tells us the major version (3), the minor version (8), and the micro version (5) of Python that’s running, along with the date it was released, and the compiler used to build it. This information can be critical when writing code that needs to be compatible with specific Python versions or when debugging issues related to the Python interpreter.
Understanding and using the sys.version
attribute is an important part of Python development, as it helps ensure that your code runs as expected across different Python environments.
Parsing sys.version for Python version number
To extract the Python version number from the sys.version
string, we can use the split()
method to separate the string into individual components. This will allow us to isolate the version number and utilize it for our programming needs. Consider the following code example:
import sys version_info = sys.version.split() version_number = version_info[0] print("Python version number:", version_number)
The above code splits the sys.version
string by spaces, which separates the version number from the rest of the string. The version number is then the first element in the resulting list, which we can access and print out. This will give us an output similar to:
Python version number: 3.8.5
However, to get more granular control over the version number, we can further split the version number string by the dot character to get the major, minor, and micro versions as separate values:
major, minor, micro = version_number.split('.') print("Major version:", major) print("Minor version:", minor) print("Micro version:", micro)
The output will then display each version component individually:
Major version: 3 Minor version: 8 Micro version: 5
For situations where we need to compare version numbers or check if we are running a specific version, it’s helpful to convert these string values to integers. This allows us to perform numerical comparisons:
major = int(major) minor = int(minor) micro = int(micro) # Example: Check if the Python version is at least 3.7 if major > 3 or (major == 3 and minor >= 7): print("This Python version is 3.7 or higher") else: print("This Python version is lower than 3.7")
By parsing the sys.version
attribute, developers can write code that adapts to different Python versions, ensuring compatibility and optimal performance across various environments.
Understanding the components of sys.version
The sys.version
attribute is not only about the version number; it also includes other components that can be useful for developers. Let’s dive deeper into the sys.version
string to understand all the components it provides.
The sys.version
string contains the following components:
- The Python version number (major.minor.micro)
- The level of the release (alpha, beta, candidate, final)
- The serial number of the release (used for alpha, beta, candidate versions)
- The date the release was created
- The compiler used to build the interpreter
To get a comprehensive view of this information, let’s print out the sys.version
string and break down each part:
import sys version_components = sys.version.split() version_number = version_components[0] release_level = version_components[1].strip('()') release_serial = version_components[2].strip(',') build_date = version_components[3] + ' ' + version_components[4] compiler = ' '.join(version_components[5:]) print("Version Number:", version_number) print("Release Level:", release_level) print("Release Serial:", release_serial) print("Build Date:", build_date) print("Compiler:", compiler)
This code will extract each component from the sys.version
string and print it out neatly. The output could look something like this:
- 3.8.5
- default
- Jul 28 2020
- 12:59:40
- GCC 9.3.0
Understanding these components is beneficial when dealing with cross-compatibility or specific build requirements. For instance, if your code relies on a feature introduced in a particular Python version, knowing the exact version and build date can help you quickly determine if your code will run without issues.
In addition to the sys.version
string, Python provides a named tuple called sys.version_info
that contains the version components as accessible attributes:
import sys print(sys.version_info) # Output: sys.version_info(major=3, minor=8, micro=5, releaselevel='final', serial=0)
With sys.version_info
, you can access the version components directly without needing to parse the string:
major = sys.version_info.major minor = sys.version_info.minor micro = sys.version_info.micro releaselevel = sys.version_info.releaselevel serial = sys.version_info.serial print("Major version:", major) print("Minor version:", minor) print("Micro version:", micro) print("Release level:", releaselevel) print("Serial:", serial)
The named tuple approach provides a more Pythonic and convenient way to work with Python version information. It allows for clearer and more maintainable code, especially when dealing with version checks and comparisons.
By understanding the components of sys.version
and knowing how to access and use them, Python developers can write more robust and adaptable code that can gracefully handle different Python environments and versions.
Practical applications of sys.version in Python programming
As we’ve seen, the sys.version
attribute and sys.version_info
named tuple are powerful tools that have practical applications in Python programming. One common use case is ensuring that certain features or libraries are only used when the correct version of Python is running. For example, you may have a script that utilizes syntax or functions introduced in Python 3.6, and you want to prevent the script from running on earlier versions of Python. You could use sys.version_info
to check the Python version before executing the code, as shown in the following example:
import sys if sys.version_info < (3, 6): print("This script requires Python 3.6 or higher!") sys.exit(1) # rest of your code goes here
This simple check ensures that your code doesn’t run in an incompatible environment, potentially preventing runtime errors and other issues.
Another practical application is in the development of cross-version libraries. When creating a library that needs to support multiple Python versions, developers can use sys.version_info
to apply version-specific logic. For instance, a function may have a more efficient implementation in Python 3.8 due to a new language feature. You could write code that checks the version and selects the appropriate implementation like this:
import sys def my_function(): if sys.version_info >= (3, 8): # Use Python 3.8 specific feature pass else: # Use alternative method for older versions pass
This ensures that your library is backward compatible while still taking advantage of newer Python features when available.
Additionally, sys.version
can be used in logging and error reporting. Including the Python version information in logs can be invaluable for debugging issues, especially when working with deployments across multiple environments. An error log entry might include the Python version like this:
import sys import logging logging.error("An error occurred on Python version %s", sys.version)
This helps developers quickly identify if a problem is related to a specific Python version or a particular build of the interpreter.
Lastly, version information can be used in conditional imports. For example, a module may have been renamed or its API changed in a newer version of Python. You can use sys.version_info
to import the correct module or use the correct API, as demonstrated here:
import sys if sys.version_info >= (3, 3): import collections.abc as collections_abc else: import collections as collections_abc # Use collections_abc in your code
In this example, the collections.abc
was introduced in Python 3.3 to store abstract base classes of collections
. The code checks the Python version and imports the correct module accordingly.
Overall, sys.version
and sys.version_info
are essential for writing Python code that is robust, maintainable, and compatible across different Python versions and environments. By using these attributes, developers can build applications and libraries that adapt seamlessly to the Python ecosystem’s evolving landscape.
Source: https://www.pythonlore.com/examining-sys-version-for-python-version-information/