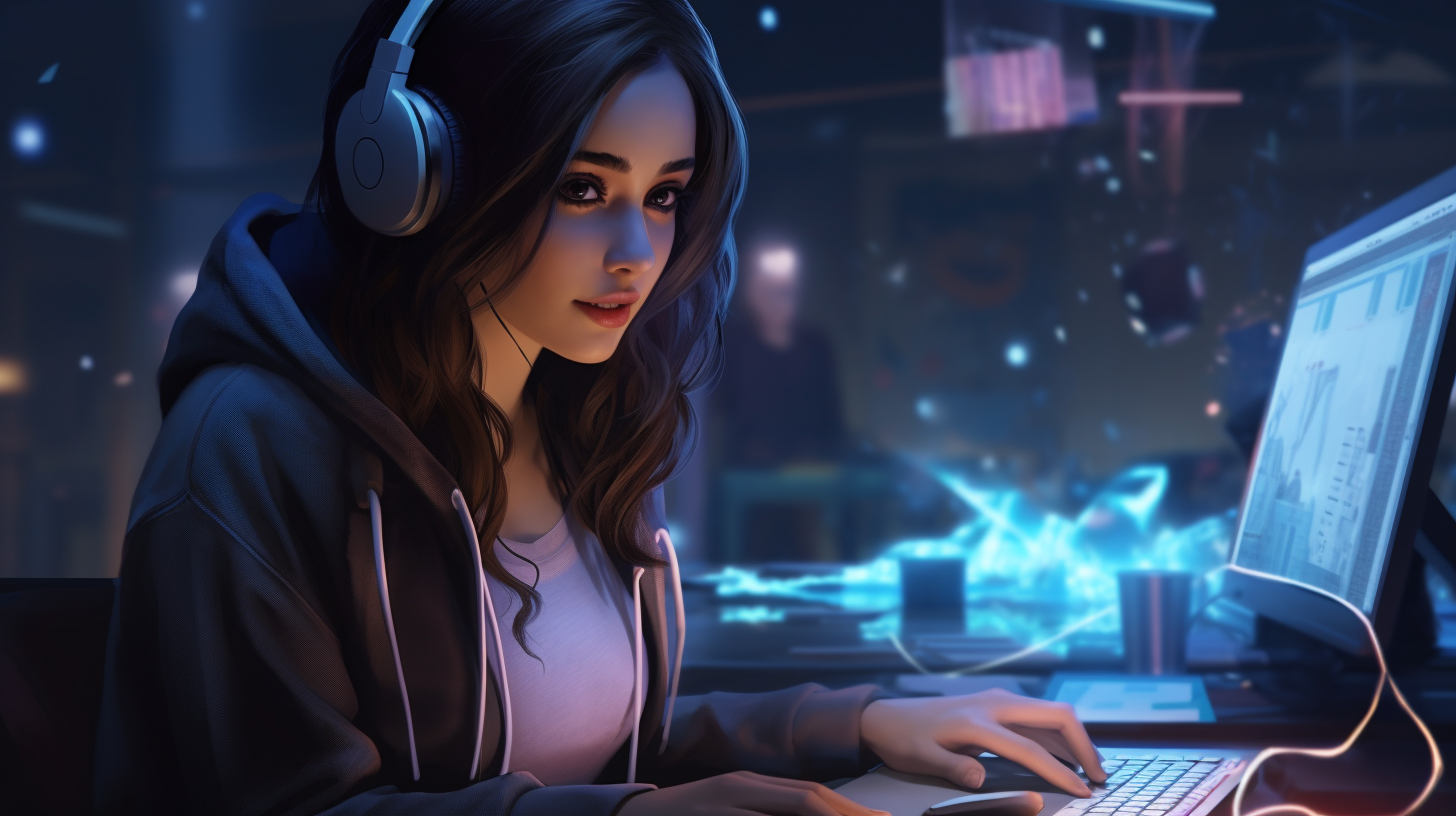
To get started with text search in MongoDB using Pymongo, you will first need to have MongoDB installed and running on your machine. If you haven’t already, you can download MongoDB from https://www.mongodb.com/try/download/community and follow the installation instructions for your operating system.
Once MongoDB is installed and the MongoDB server is running, the next step is to set up Pymongo, which is the Python driver for MongoDB. To install Pymongo, you can simply use pip:
pip install pymongo
After installing Pymongo, you can connect to your MongoDB server using the MongoClient
class:
from pymongo import MongoClient client = MongoClient('localhost', 27017)
Replace ‘localhost’ with the address of your MongoDB server if it’s not running on your local machine, and ‘27017’ with the port number if you are using a non-default port.
With the client object, you can access databases and collections. For example, to access a database named ‘mydatabase’ and a collection named ‘mycollection’, you would do the following:
db = client.mydatabase collection = db.mycollection
Now that you have set up MongoDB and Pymongo, you are ready to start implementing text search in your collections.
Indexing Text Fields for Efficient Search
Before performing any text search queries, it’s important to create an index on the fields you want to search. Indexing these fields can significantly improve the performance of your text search queries. In MongoDB, you can create a text index on a field that holds string content. Here’s how you can create a text index on a field named “content” in your collection:
collection.create_index([("content", "text")])
Once the index is created, MongoDB will use it to perform full-text search operations more efficiently. You can also create a compound text index if you want to perform text searches on multiple fields. Here’s an example of creating a compound text index on the fields “title” and “description”:
collection.create_index([("title", "text"), ("description", "text")])
If you have a large collection, creating an index may take some time. You can check the status of index creation by using the list_indexes()
method:
for index in collection.list_indexes(): print(index)
This will print out a list of all indexes on the collection, including the newly created text index(es).
It’s also worth noting that you can set additional options on your text index, such as specifying the language for the text index or setting weights on fields for relevance scoring. For instance, if you want to create a text index that considers “title” to be more important than “description”, you can set weights like so:
collection.create_index([("title", "text"), ("description", "text")], weights={'title': 10, 'description': 5})
By setting up indexes on text fields, you lay the groundwork for efficient and effective text search capabilities within your MongoDB collections.
Performing Text Search Queries
Performing text search queries in MongoDB using Pymongo is straightforward once you have your text indexes set up. You can use the $text
operator in your queries to search for specific words or phrases within the indexed fields. The basic syntax for a text search query is:
results = collection.find({"$text": {"$search": "search term"}})
For example, if you want to search for documents that contain the word “Python” in the indexed fields, you would write:
results = collection.find({"$text": {"$search": "Python"}})
By default, text search queries are case-insensitive and will match on any words in the search string. If you want to search for an exact phrase, you can enclose the phrase in double quotes:
results = collection.find({"$text": {"$search": ""exact phrase""}})
Additionally, you can exclude words from the search by prefixing them with a minus sign. For instance, to search for documents that contain “Python” but not “Java”, you would write:
results = collection.find({"$text": {"$search": "Python -Java"}})
It’s also possible to sort the search results by relevance score, which is a measure of how well each document matches the search terms. You can access the relevance score using the $meta
operator and sort the results like this:
results = collection.find( {"$text": {"$search": "search term"}}, {"score": {"$meta": "textScore"}} ).sort([("score", {"$meta": "textScore"})])
Here’s an example of how to perform a search and display the results along with their relevance scores:
results = collection.find( {"$text": {"$search": "Python"}}, {"score": {"$meta": "textScore"}} ).sort([("score", {"$meta": "textScore"})]) for result in results: print(result["_id"], result["score"])
By using text search queries, you can quickly and efficiently search through large amounts of text data in your MongoDB collections. Whether you are looking for exact matches, excluding certain words, or sorting by relevance, Pymongo provides the tools you need to implement powerful text search functionality in your applications.
Advanced Text Search Techniques
Now that we have covered the basics of text search in MongoDB with Pymongo, let’s dive into some of the advanced techniques that can further refine your text search capabilities. One such technique is using the $regex operator for pattern matching. This operator allows you to perform complex searches using regular expressions. For example, you can search for documents that have a field containing a specific pattern:
results = collection.find({"content": {"$regex": "pattern"}})
Here, “pattern” is the regular expression you want to match against the “content” field. You can also specify options for the regular expression, such as case-insensitivity:
results = collection.find({"content": {"$regex": "pattern", "$options": "i"}})
Another advanced technique is using the $where operator to execute JavaScript expressions in your query. This can be useful when you have more complex conditions that cannot be easily expressed with the standard query operators. However, be cautious with the use of $where, as it can lead to performance issues due to its nature of evaluating JavaScript code:
results = collection.find({"$where": "this.content.match(/pattern/i)"})
Additionally, you can combine text search with other query operators to create more targeted searches. For example, you can search for documents that match a text search query and also meet certain conditions:
results = collection.find({ "$text": {"$search": "search term"}, "author": "Frank McKinnon", "published": {"$gte": datetime(2020, 1, 1)} })
This query will find documents that contain the “search term” in the text-indexed fields, were authored by “Nick Johnson”, and were published on or after January 1, 2020.
Lastly, you can take advantage of MongoDB’s aggregation framework to perform more complex text searches. The aggregation framework allows you to pipeline multiple stages of data processing to transform and analyze your data. Here’s an example of using text search within an aggregation pipeline:
pipeline = [ {"$match": {"$text": {"$search": "search term"}}}, {"$sort": {"score": {"$meta": "textScore"}}}, {"$limit": 10}, {"$project": {"title": 1, "score": {"$meta": "textScore"}}} ] results = collection.aggregate(pipeline) for result in results: print(result["title"], result["score"])
This aggregation pipeline performs a text search, sorts the results by relevance score, limits the results to the top 10 matches, and projects only the “title” field and relevance score for each document. Aggregation provides a powerful way to build complex queries and analyze your text data in MongoDB.
With these advanced text search techniques, you can create more sophisticated search functionalities in your MongoDB collections, providing a richer experience for your users when they interact with your data.
Source: https://www.pythonlore.com/implementing-text-search-in-mongodb-collections-with-pymongo/