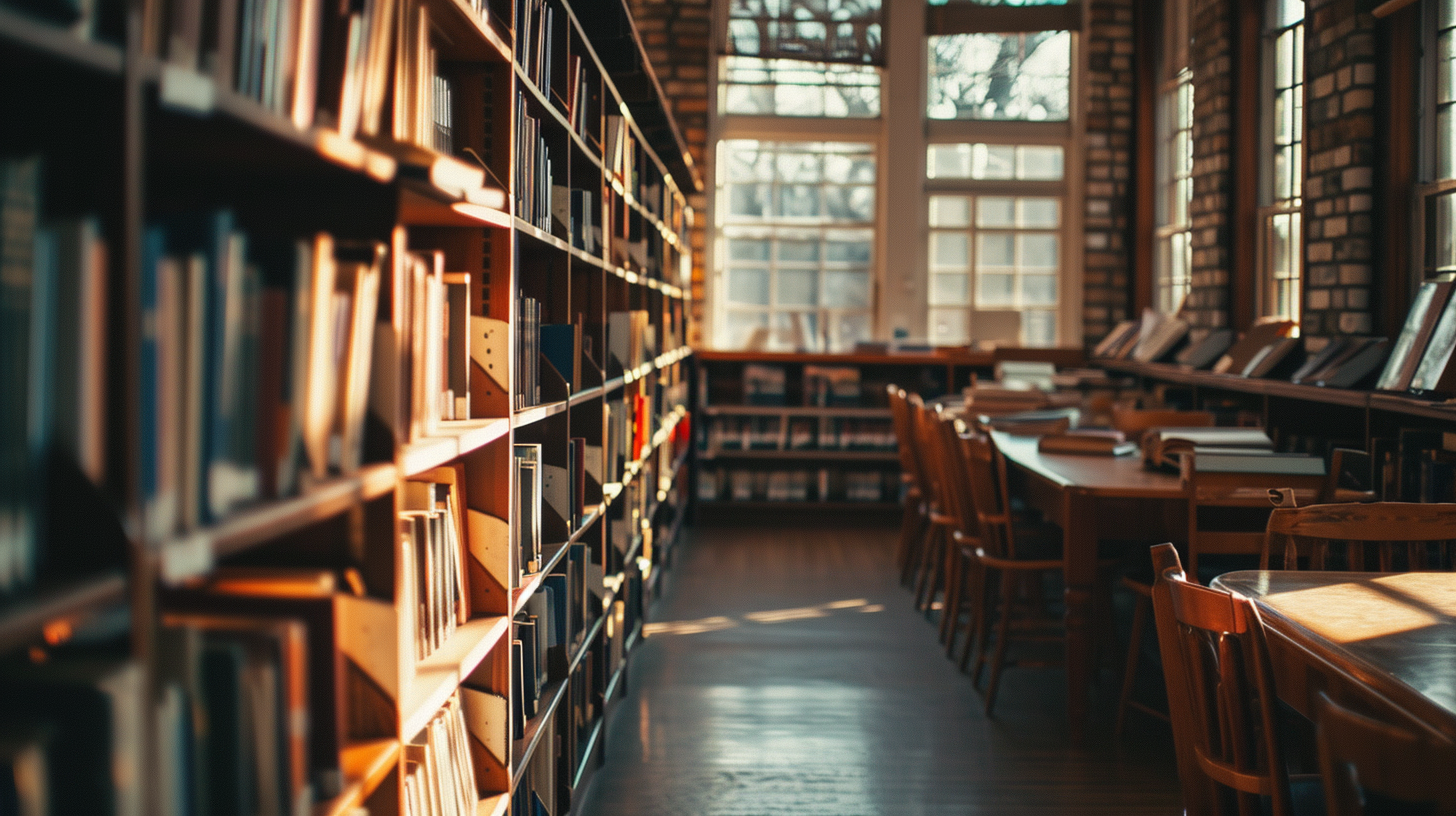
The re.subn()
function in Python is a powerful tool for performing search and replace operations on strings. This function is part of the re
module, which provides support for regular expressions. The re.subn()
function is similar to re.sub()
, but it returns a tuple containing the new string and the number of substitutions made.
Here is the basic syntax for re.subn()
:
import re new_string, number_of_subs = re.subn(pattern, repl, string, count=0, flags=0)
The pattern
parameter is the regular expression pattern to search for within the string
. The repl
parameter is the replacement string that will replace every match. The count
parameter is optional and specifies the maximum number of pattern occurrences to be replaced; the default value of 0 means that all occurrences will be replaced. The flags
parameter is also optional and can be used to modify how the regular expression is interpreted (more on that in a later subsection).
One of the key features of re.subn()
is that it helps keep track of the number of substitutions made, which can be valuable for debugging or for further processing in your code.
Let’s look at a simple example:
import re text = "The rain in Spain" pattern = r"ain" replacement = "ow" result = re.subn(pattern, replacement, text) print(result) # Output: ('The row in Spow', 2)
In this example, re.subn()
replaced all occurrences of “ain” with “ow”, resulting in the new string “The row in Spow” and a substitution count of 2.
As you can see, re.subn()
is quite straightforward to use. However, its real power comes into play when dealing with more complex patterns and replacement scenarios, which we will explore in the following subsections.
Using re.subn with Regular Expressions
Regular expressions are patterns used to match character combinations in strings. In Python, regular expressions are supported by the re
module. Some of the most common regular expression symbols include:
- . (dot) – Matches any character except a newline.
- ^ – Matches the start of a string.
- $ – Matches the end of a string.
- * – Matches 0 or more repetitions of the preceding regex.
- + – Matches 1 or more repetitions of the preceding regex.
- ? – Matches 0 or 1 repetition of the preceding regex.
- {n} – Matches exactly n copies of the previous regex.
- {n,} – Matches n or more copies of the previous regex.
- {n,m} – Matches between n and m copies of the previous regex.
- […] – Matches any single character in brackets.
- [^…] – Matches any single character not in brackets.
When using re.subn()
, you can leverage the power of regular expressions to perform complex pattern matching and substitution. For example, suppose you want to replace all sequences of multiple spaces with a single space. You could use the following regular expression pattern: r's+'
, which matches one or more whitespace characters. Here’s how you would do it:
import re text = "The quick brown fox" pattern = r"s+" replacement = " " result = re.subn(pattern, replacement, text) print(result) # Output: ('The quick brown fox', 3)
As shown in the example, re.subn()
replaced all sequences of multiple spaces with a single space, and it also returned the count of substitutions made, which is 3 in this case.
You can also use regular expressions to match and replace patterns in more complex scenarios, such as email address obfuscation. Suppose you want to replace the domain part of email addresses with the string “[at]domain.com”. Here is an example of how you might write the pattern and use re.subn()
:
import re text = "Contact us at [email protected] for more info." pattern = r"(@)([a-zA-Z0-9_.]+)" replacement = "[at]domain.com" result = re.subn(pattern, replacement, text) print(result) # Output: ('Contact us at info[at]domain.com for more info.', 1)
In the above example, the pattern r"(@)([a-zA-Z0-9_.]+)"
is used to match the “@” symbol followed by the domain name, which consists of alphanumeric characters, underscores, or dots. The replacement string “[at]domain.com
” is then used to obfuscate the domain part of the email address.
Using re.subn()
with regular expressions allows you to handle various text processing tasks efficiently and with minimal code. In the following subsections, we will delve into other features and advanced options available with re.subn()
.
Handling Substitution Count with re.subn
Another powerful capability of re.subn() is controlling the number of substitutions it performs using the count
parameter. This can be particularly useful when you only want to replace a certain number of matches within a string, rather than all possible matches.
For instance, ponder the scenario where you have a string with several instances of a specific word, but you only want to replace the first two occurrences. Here’s how you would do it:
import re text = "cat, bat, rat, cat, cat" pattern = r"cat" replacement = "dog" max_replacements = 2 result = re.subn(pattern, replacement, text, count=max_replacements) print(result) # Output: ('dog, bat, rat, dog, cat', 2)
In the example above, by setting the count
parameter to 2, we limit the re.subn()
function to only replace the first two occurrences of “cat” with “dog”. As a result, the third “cat” in the string remains unchanged, and the result
outputs the new string along with the number of substitutions made, which is 2.
Sometimes, you may want to replace all occurrences except for the last one, or you might want to skip the first few matches. You can achieve this by combining a counter with a function as the repl
parameter. Here’s an example:
import re text = "cat, bat, rat, cat, cat" pattern = r"cat" replacement = "dog" skip_first = 1 replacement_count = 0 def conditional_replacement(match): global replacement_count if replacement_count >= skip_first: return replacement replacement_count += 1 return match.group(0) result = re.subn(pattern, conditional_replacement, text) print(result) # Output: ('cat, bat, rat, dog, dog', 2)
In the code above, the conditional_replacement
function is used as the replacement parameter. It checks a counter to determine whether to perform the substitution. In this case, it skips the first match, replacing the subsequent “cat” strings with “dog”. The re.subn()
function then returns the new string and the number of actual replacements that took place, which is 2.
The ability to handle substitution count with re.subn() adds another layer of flexibility to your text processing, allowing for more precise and targeted string manipulation. As we continue, we will explore additional advanced options and flags that can be used with re.subn() to further enhance its capabilities.
Advanced Options and Flags with re.subn
In addition to the basic usage of re.subn()
, there are several advanced options and flags that can be used to modify the behavior of the function. These flags can be passed as the flags
parameter and can alter the way the regular expression pattern is interpreted or how the substitution is performed.
One of the most commonly used flags is re.IGNORECASE
(or re.I
for short), which makes the pattern matching case-insensitive. This means that uppercase and lowercase characters will be treated as equal when matching. Here’s an example:
import re text = "The Rain in Spain" pattern = r"ain" replacement = "ow" flags = re.IGNORECASE result = re.subn(pattern, replacement, text, flags=flags) print(result) # Output: ('The Row in Spow', 2)
In this example, even though the original text has “Rain” with an uppercase “R”, the pattern still matches it because of the re.IGNORECASE
flag, resulting in two substitutions.
Another useful flag is re.MULTILINE
(or re.M
for short), which changes the behavior of the ^
and $
metacharacters. When this flag is set, ^
matches the start of each line within the string, and $
matches the end of each line, rather than the start and end of the entire string. This is particularly useful when working with multi-line strings. Here’s an example:
import re text = "Start of line onenStart of line twonEnd of line two" pattern = r"^Start" replacement = "Beginning" flags = re.MULTILINE result = re.subn(pattern, replacement, text, flags=flags) print(result) # Output: ('Beginning of line onenBeginning of line twonEnd of line two', 2)
In this example, the re.MULTILINE
flag allows the pattern to match “Start” at the beginning of each line, resulting in two substitutions instead of just one without the flag.
Other flags include re.DOTALL
(or re.S
), which makes the .
metacharacter match any character including a newline, and re.ASCII
(or re.A
), which makes the pattern matching based on ASCII character set instead of Unicode. These flags can be combined by bitwise OR-ing them (using the |
operator), enabling multiple behaviors simultaneously.
Let’s look at an example that uses both re.DOTALL
and re.IGNORECASE
flags:
import re text = "First line.nSecond line." pattern = r".*" replacement = "---" flags = re.DOTALL | re.IGNORECASE result = re.subn(pattern, replacement, text, flags=flags) print(result) # Output: ('---n---', 2)
In the above example, the pattern r".*"
matches everything including the newline character because of the re.DOTALL
flag. The re.IGNORECASE
flag is also used here, though it doesn’t impact the outcome since there are no alphabetic characters in the pattern. The result shows that the entire content was replaced with “—” on each line, with two substitutions.
Using these advanced options and flags with re.subn()
provides you with more control and flexibility in your search and replace operations, allowing you to handle more complex text processing tasks with ease.
In the next subsection, we will look at some practical examples and best practices for advanced substitution using re.subn()
.
Examples and Best Practices for Advanced Substitution
When working with text substitutions in Python, using the re.subn()
function can significantly enhance your ability to handle complex patterns and replacement scenarios. In this section, we will explore some practical examples and best practices for advanced substitution using re.subn()
.
Ponder a scenario where you want to replace dates in a string with a uniform format. Suppose you have dates in different formats like “12/31/2020”, “31-12-2020”, and “2020.12.31”, and you want to replace them all with the format “YYYY-MM-DD”. Here’s how you can achieve this:
import re text = "Event dates: 12/31/2020, 31-12-2020, and 2020.12.31." pattern = r"(d{2})[/-](d{2})[/-](d{4})|(d{4})[.](d{2})[.](d{2})" replacement = lambda m: f"{m.group(3) or m.group(4)}-{m.group(1) or m.group(5)}-{m.group(2) or m.group(6)}" result = re.subn(pattern, replacement, text) print(result) # Output: ('Event dates: 2020-12-31, 2020-12-31, and 2020-12-31.', 3)
In this example, we use a regular expression pattern that matches different date formats and a lambda function as the replacement parameter to rearrange the date components into the desired format. The re.subn()
function then returns the updated string with uniform date formats and the number of substitutions made.
Another best practice is using named groups in your regular expressions, which can make your patterns more readable and easier to maintain. Here’s an example that demonstrates this approach:
import re text = "Username: johndoe, email: [email protected]" pattern = r"Username: (?Pw+), email: (?P[w.]+@[w.]+)" replacement = "User email: g, User name: g" result = re.subn(pattern, replacement, text) print(result) # Output: ('User email: [email protected], User name: johndoe', 1)
In the above code, we use named groups username
and email
within the pattern, making it clear what each part of the pattern is capturing. The replacement string then uses the g
syntax to reference the named groups.
Lastly, it especially important to handle edge cases and special characters carefully. For instance, if you need to replace a dollar sign ($) with another currency symbol, you should escape the dollar sign in the pattern to avoid it being interpreted as the end-of-string metacharacter:
import re text = "The price is $100." pattern = r"$" replacement = "€" result = re.subn(pattern, replacement, text) print(result) # Output: ('The price is €100.', 1)
In this example, we escape the dollar sign with a backslash in the pattern to match it literally in the text and replace it with the euro symbol.
By following these examples and best practices, you can leverage re.subn()
to perform advanced text substitutions with confidence and precision. Whether you’re formatting data, obfuscating sensitive information, or handling special characters, re.subn()
is a versatile tool that can simplify complex string manipulation tasks in Python.
Source: https://www.pythonlore.com/advanced-substitution-with-re-subn/