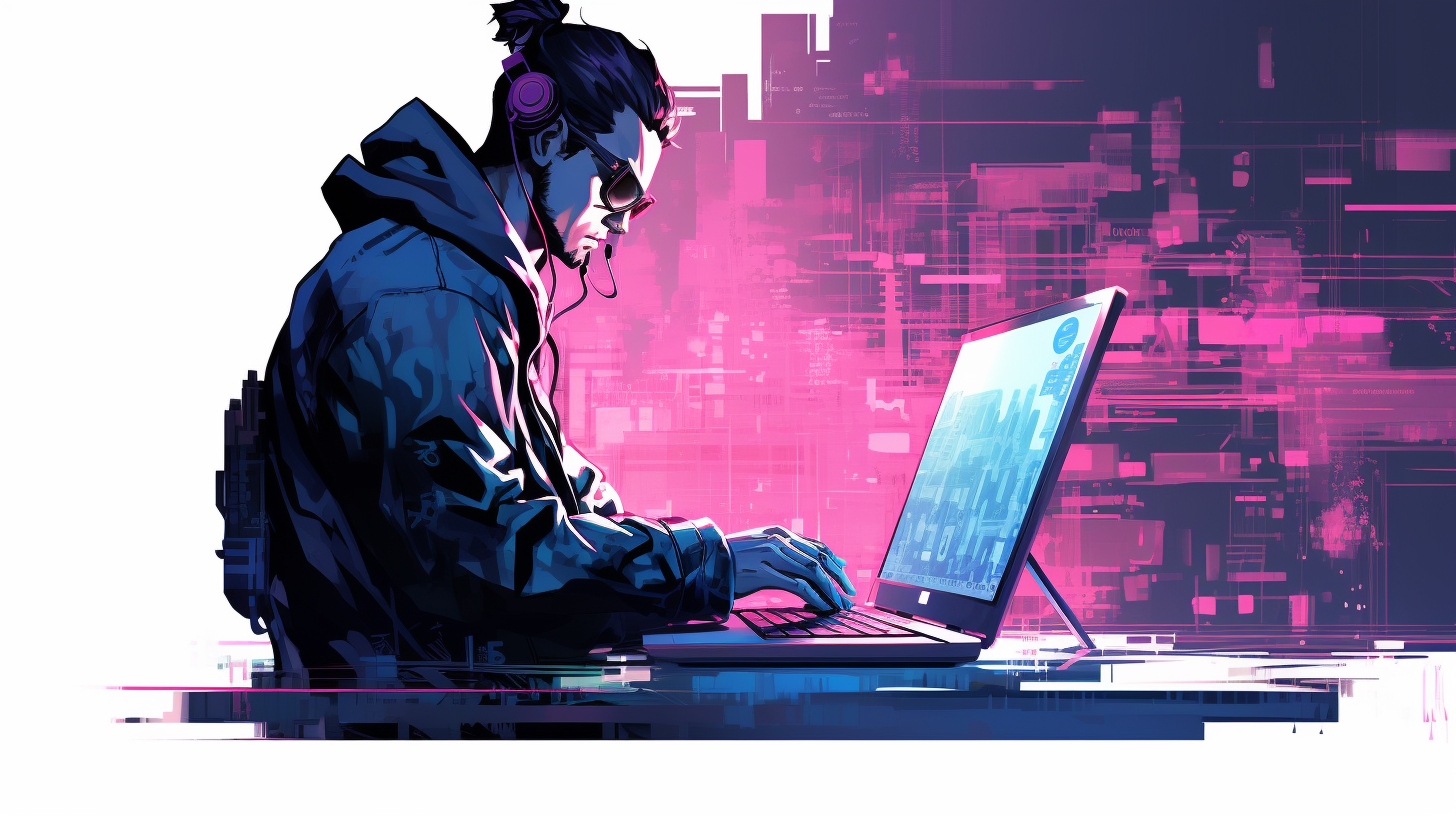
In numpy
, an ndarray object is a multidimensional container of items of the same type and size. Indexing in numpy
works in a similar way to indexing in standard Python sequences, like lists, but with some added capabilities.
Basic indexing in a numpy.ndarray
is done by using square brackets, with the indices separated by commas. For a one-dimensional array, indexing works just like a list:
import numpy as np # Create a one-dimensional array arr = np.array([1, 2, 3, 4, 5]) # Access the first element print(arr[0]) # Output: 1 # Access the last element print(arr[-1]) # Output: 5
For a two-dimensional array, you can access elements using a pair of indices – the first one denotes the row, and the second one denotes the column:
# Create a two-dimensional array arr_2d = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) # Access the element on the first row and second column print(arr_2d[0, 1]) # Output: 2 # Access the last element in the last row print(arr_2d[-1, -1]) # Output: 9
It is important to note that indexing in numpy
starts at 0, just like in standard Python. Negative indices can be used to access elements from the end of the array, with -1
being the last element.
Complex indexing operations can be performed by combining indexing with :
, which is used to select a range of elements. For example:
# Select all elements from the second row print(arr_2d[1, :]) # Output: [4 5 6] # Select all elements from the second column print(arr_2d[:, 1]) # Output: [2 5 8]
Using a single colon :
without specifying start or end, selects all elements in that dimension, allowing for easy selection of rows, columns, or even higher dimensions in a multidimensional array.
Important to remember: Basic indexing in numpy
returns views, not copies of the array data. This means that modifications to the view will affect the original array, and vice versa.
Basic indexing is the foundation for more advanced array manipulations in numpy
. Understanding how to access and modify elements of an ndarray
is important for efficient data manipulation and analysis.
Advanced Slicing Techniques in numpy.ndarray
Now let’s delve into more advanced slicing techniques in numpy.ndarray which allow for more sophisticated data manipulation. In numpy, you can use slices to select a subsequence of an array, or a “slice” of it. That is done by using the colon operator :
within the square brackets, along with optional start, stop, and step parameters. The general syntax for slicing is array[start:stop:step]
. Let’s look at some examples to understand this better.
# Create a one-dimensional array arr = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10]) # Select elements from index 2 to 5 print(arr[2:6]) # Output: [3 4 5 6] # Select elements from the beginning to index 5 print(arr[:6]) # Output: [1 2 3 4 5 6] # Select elements from index 5 to the end of the array print(arr[5:]) # Output: [6 7 8 9 10] # Select every other element from the entire array print(arr[::2]) # Output: [1 3 5 7 9] # Select elements in reverse order print(arr[::-1]) # Output: [10 9 8 7 6 5 4 3 2 1]
Advanced slicing can also be applied to multidimensional arrays. In a two-dimensional array, for example, you can use slicing to select entire rows or columns, or sub-matrices. Let’s see some examples:
# Create a two-dimensional array arr_2d = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) # Select the first two rows and the first two columns print(arr_2d[:2, :2]) # Output: # [[1 2] # [4 5]] # Select all rows and every other column print(arr_2d[:, ::2]) # Output: # [[1 3] # [4 6] # [7 9]] # Select a sub-matrix from the center of the array print(arr_2d[1:3, 1:3]) # Output: # [[5 6] # [8 9]]
Advanced slicing is especially powerful because it allows you to manipulate the shape and size of the resulting sub-arrays. By carefully selecting the start, stop, and step values, you can extract just about any subset of an array that you need for your data analysis tasks.
Note: Just like with basic indexing, slicing in numpy returns views of the original array, not copies. This is an important consideration when modifying the data in the sub-arrays, as changes will propagate back to the original array.
By mastering advanced slicing techniques, you can leverage numpy’s powerful data manipulation capabilities to preprocess and analyze your datasets with ease.
Working with Multidimensional Arrays
Working with multidimensional arrays in numpy is made simple with the indexing and slicing techniques we have discussed so far. For larger arrays with more than two dimensions, the same principles apply. We use a comma to separate each dimension’s index or slice, with each dimension’s indexing starting at 0.
Let’s think a three-dimensional array as an example:
# Create a three-dimensional array arr_3d = np.array([[[1, 2], [3, 4]], [[5, 6], [7, 8]], [[9, 10], [11, 12]]]) # Access the element on the first matrix, first row, and second column print(arr_3d[0, 0, 1]) # Output: 2 # Access the second matrix print(arr_3d[1]) # Output: # [[5 6] # [7 8]]
With multidimensional arrays, you can also combine slicing with indexing. For instance, to select the first column from each matrix, you can use:
# Select the first column from each matrix print(arr_3d[:, :, 0]) # Output: # [[ 1 3] # [ 5 7] # [ 9 11]]
To further illustrate the power of slicing in multidimensional arrays, let’s select a 2×2 sub-matrix from the second and third matrices:
# Select a 2x2 sub-matrix from the second and third matrices print(arr_3d[1:, 0:2, 0:2]) # Output: # [[[ 5 6] # [ 7 8]] # [[ 9 10] # [11 12]]]
As you can see, working with multidimensional arrays in numpy is a matter of understanding the dimensionality and applying the appropriate indexing and slicing operations. Remember, the result of indexing or slicing multidimensional arrays is always a view, not a copy, which can be both powerful and efficient, but also something to keep in mind when modifying the array.
Combining Indexing and Slicing for Complex Operations
When working with multidimensional arrays, you might often need to perform complex operations that involve both indexing and slicing. This combination allows you to access specific elements, rows, columns, or sub-arrays within a larger array. By understanding how to combine indexing and slicing, you can perform more intricate array manipulations.
For example, if you want to access specific rows from a two-dimensional array and select certain columns from those rows, you can do so by combining slicing and indexing:
# Access specific rows and select certain columns print(arr_2d[[0, 2], 1:]) # Output: # [[2 3] # [8 9]]
Another common operation is to use boolean indexing along with slicing to filter arrays based on some condition. For instance, you can select all rows where the first element is greater than a certain value:
# Select rows where the first element is greater than 4 print(arr_2d[arr_2d[:, 0] > 4]) # Output: # [[7 8 9]]
By combining slicing, indexing, and boolean operations, you can create highly flexible and powerful expressions to manipulate and analyze your data in numpy. This level of control is one of the reasons why numpy is such a valuable tool for data scientists and researchers working with large and complex datasets.
Combining Indexing and Slicing for Complex Operations
Let’s take a more complex example involving a three-dimensional array. Suppose you want to select all elements from the second and third matrices where the last element of each row is greater than 6. You can accomplish this by combining slicing and boolean indexing:
# Select elements from the second and third matrices where the last element of each row is greater than 6 mask = arr_3d[:, :, -1] > 6 result = arr_3d[1:][mask[1:]] print(result) # Output: # [ 7 8 9 10 11 12]
Notice how the mask is created by applying a boolean condition to a slice of the array. The mask is then used to index the array and select the desired elements. This technique can be extremely useful when dealing with large arrays and complex conditions.
Another powerful way to combine indexing and slicing is to use the np.ix_
function, which allows you to construct an open mesh from multiple sequences. This is particularly useful when you want to select a specific rectangle of elements from a two-dimensional array:
# Use np.ix_ to select a rectangle of elements rows_to_select = [0, 2] cols_to_select = [1, 2] selected_rectangle = arr_2d[np.ix_(rows_to_select, cols_to_select)] print(selected_rectangle) # Output: # [[2 3] # [8 9]]
The np.ix_
function is creating a mesh that specifies the cross-product of the desired rows and columns. This results in a two-dimensional array this is a subset of the original array, defined by the selected rows and columns.
Combining indexing and slicing is an essential skill for any numpy user. It allows you to write concise and efficient code that can perform complex array manipulations. Whether you’re filtering data, selecting specific elements, or reshaping arrays, understanding how to combine these operations will enable you to work more effectively with numpy arrays.
Source: https://www.pythonlore.com/advanced-slicing-and-indexing-with-numpy-ndarray/