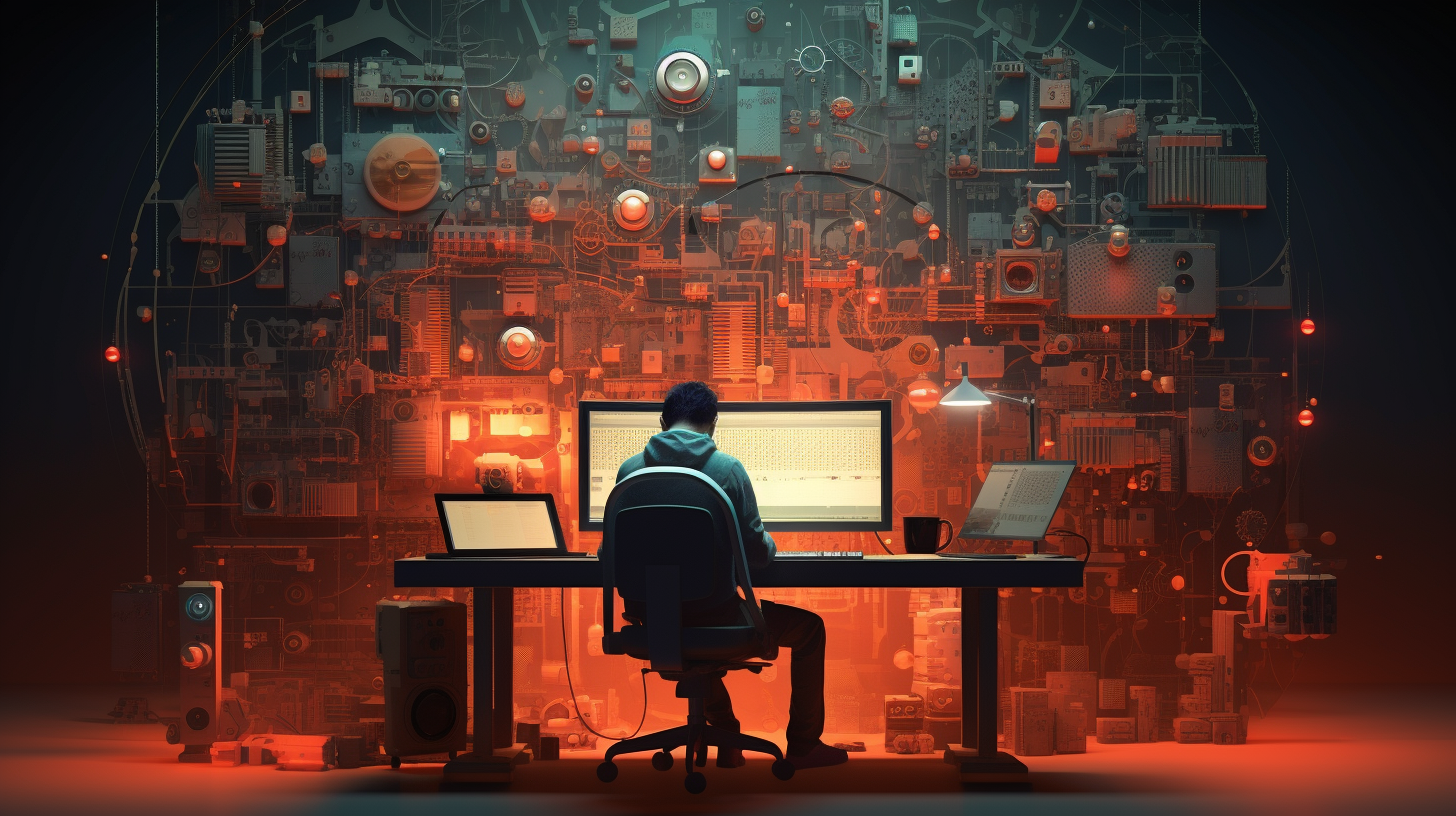
Python is a powerful programming language that can be used in various domains including cybersecurity. In this tutorial, we will explore the basic principles of Python in the context of cybersecurity. We will cover concepts such as encryption, hashing, and more, and provide code examples to illustrate how Python can be used effectively in cybersecurity.
Encryption:
Encryption is the process of encoding information in a way that only authorized parties can access it. It’s commonly used to protect sensitive data from unauthorized access. Python provides several libraries that can be used for encryption, such as cryptography
and pycryptodome
.
Here’s an example of how to use the cryptography
library to encrypt and decrypt a message:
from cryptography.fernet import Fernet # Generate a key key = Fernet.generate_key() # Create a cipher object cipher = Fernet(key) # Encrypt a message message = b"Hello, Python!" encrypted_message = cipher.encrypt(message) # Decrypt the message decrypted_message = cipher.decrypt(encrypted_message) print("Original message:", message) print("Encrypted message:", encrypted_message) print("Decrypted message:", decrypted_message)
Hashing:
Hashing is a process of converting data of any size into a fixed-size value. It’s commonly used to verify data integrity and password storage. Python provides a built-in hashlib
library that supports various hashing algorithms.
Here’s an example of how to use the hashlib
library to compute hash values:
import hashlib # Create a hash object hash_object = hashlib.sha256() # Update the hash object with input data data = b"Hello, Python!" hash_object.update(data) # Get the hash value hash_value = hash_object.hexdigest() print("Input data:", data) print("Hash value:", hash_value)
Secure Socket Layer (SSL):
Secure Socket Layer (SSL) is a cryptographic protocol that provides secure communication over the internet. Python provides the ssl
module to enable SSL/TLS support in your applications.
Here’s an example of using the ssl
module to create a secure socket connection:
import ssl import socket # Create a secure socket connection context = ssl.create_default_context() with socket.create_connection(('www.google.com', 443)) as sock: with context.wrap_socket(sock, server_hostname='www.google.com') as ssock: # Send and receive data ssock.sendall(b'GET / HTTP/1.1rnHost: www.google.comrnrn') response = ssock.recv(4096) print("Response:", response.decode())
Python provides a wide range of capabilities for cybersecurity tasks, including encryption, hashing, and SSL/TLS support. These examples demonstrate just a small portion of what can be accomplished with Python in the field of cybersecurity. As you continue to explore and learn more about Python, you’ll discover even more possibilities to imropve your cybersecurity efforts.
Remember, when working with sensitive data, it is important to follow best practices and ensure that your code is secure. Stay updated with the latest cybersecurity trends and continuously improve your knowledge and skills to protect against modern threats.
Source: https://www.plcourses.com/python-and-cybersecurity-basic-principles/