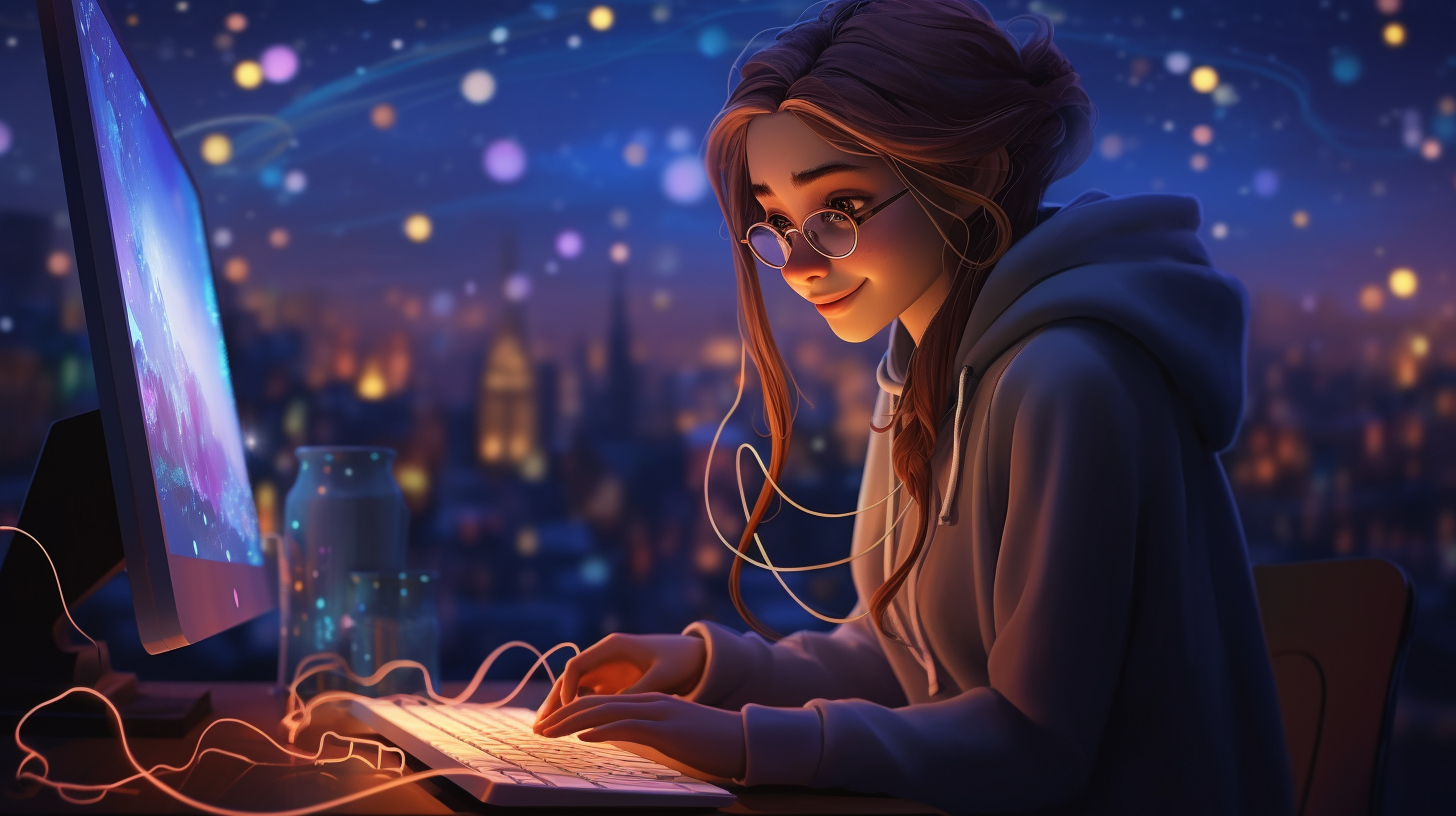
Advanced Bash Variables
Bash variables are powerful tools that allow you to store and manipulate data within your shell scripts. In this article, we will explore advanced techniques for working with Bash variables, including arrays and strings.
Arrays
An array is a variable that can store multiple values. You can think of it as a collection of variables, where each element has a unique index. Bash arrays are zero-based, meaning the first element has an index of 0.
# Creating an array my_array=("apple" "banana" "cherry") # Accessing array elements echo "The first element is ${my_array[0]}" echo "All elements: ${my_array[@]}"
The above code creates an array called my_array
with three elements. We can access individual elements using their index within curly braces (${my_array[index]}
). To access all elements of an array, we use the @
symbol inside curly braces (${my_array[@]}
).
Arrays can be useful for storing lists of values or iterating over a set of data. Let’s see an example:
# Iterating over array elements for fruit in "${my_array[@]}"; do echo "I like $fruit" done
The above code uses a for
loop to iterate over each element in the my_array
array and prints a message for each fruit.
Strings
In Bash, strings are sequences of characters enclosed in single or double quotes. You can manipulate strings using various operators and functions.
Concatenation:
# Concatenating strings name="John" greeting="Hello, $name!" echo $greeting
In the above example, we concatenate the value of the name
variable with a greeting message and store it in the greeting
variable. Then we print the value of the greeting
variable.
Substring Extraction:
# Extracting substrings fruit="apple" echo ${fruit:1:3}
This code snippet extracts a substring from the fruit
variable. It starts at index 1 and includes the next 3 characters. The output will be ppl
.
String Length:
# Getting string length fruit="banana" echo ${#fruit}
The above example calculates the length of the fruit
string using the #
operator. The output will be 6
.
These are just a few examples of what you can do with Bash variables, arrays, and strings. Armed with these tools, you can write more powerful and flexible shell scripts.
In this article, we covered advanced techniques for working with Bash variables. We explored arrays and how to manipulate them. We also looked at various string operations like concatenation, substring extraction, and getting string length. With these skills, you can take your Bash scripting to the next level.
Source: https://www.plcourses.com/advanced-bash-variables/