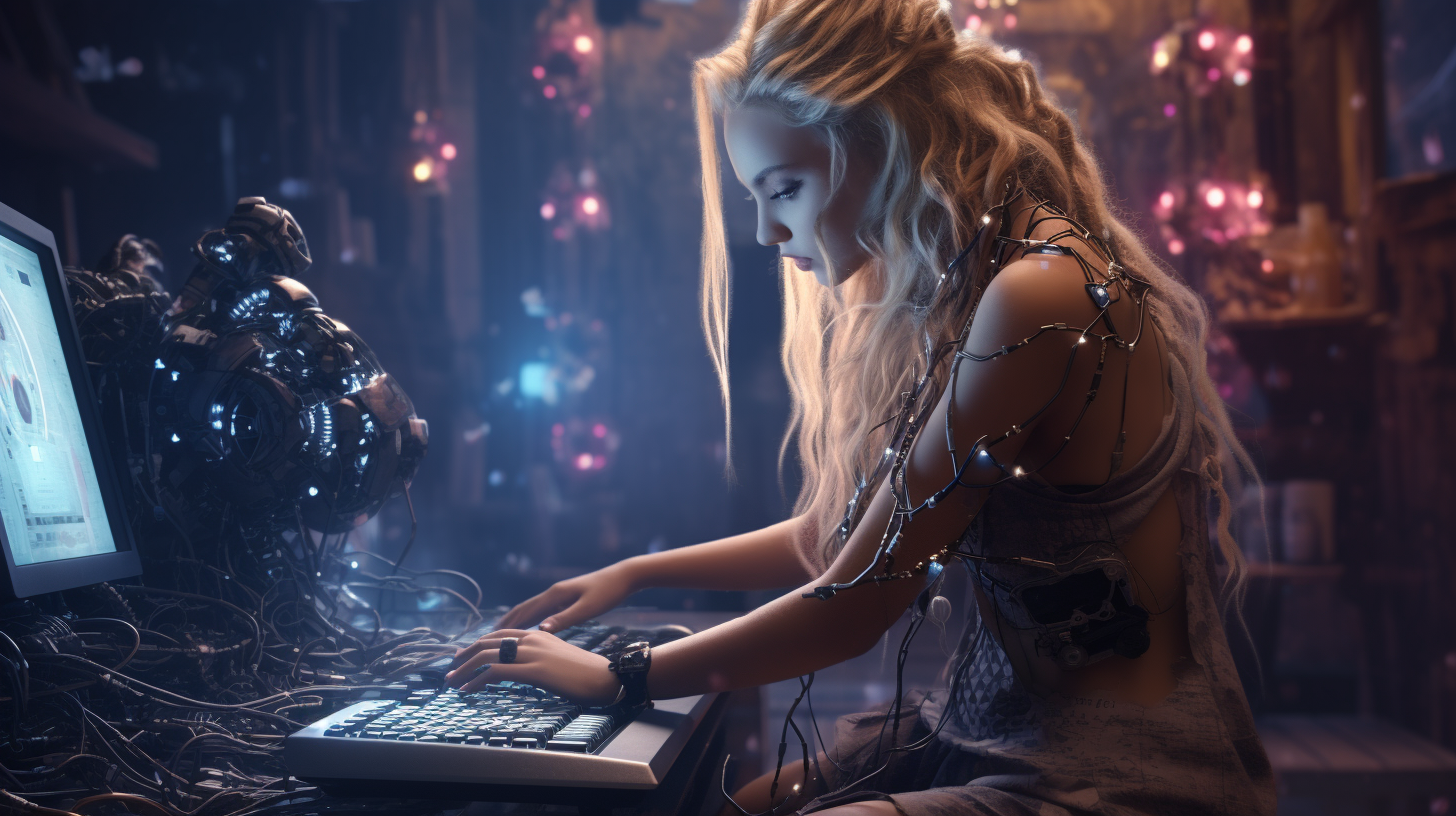
Introduction to Swift Programming Language
Swift is a powerful and intuitive programming language created by Apple for building apps for iOS, Mac, Apple TV, and Apple Watch. It’s designed to give developers more freedom than ever before. Swift is effortless to handle and open source, so anyone with an idea can create something incredible.
Developers often favor Swift due to its state-of-the-art features that make code easier to read and write, while also providing the performance and safety needed for app development. Some of the key features of Swift include:
- Optionals which handle the absence of a value simply by declaring whether there is a value or not.
- Type Inference which makes the compiler able to deduce types from the code, making code cleaner and less prone to mistakes.
- Memory Management with Automatic Reference Counting (ARC) handling the memory usage of apps.
- Functional Programming Patterns, such as map and filter, which are first-class functions in Swift.
- Protocol-Oriented Programming, which is a more powerful form of object-oriented programming.
Here is a simple example of Swift code:
// Define a constant with an explicit type. let explicitDouble: Double = 70 // Define a variable with an inferred type. var myVariable = 42 myVariable = 50 // Use a tuple to return multiple values from a function. func calculateStatistics(scores: [Int]) -> (min: Int, max: Int, sum: Int) { var min = scores[0] var max = scores[0] var sum = 0 for score in scores { if score > max { max = score } else if score < min { min = score } sum += score } return (min, max, sum) } let statistics = calculateStatistics(scores: [5, 3, 100, 3, 9]) print(statistics.sum) // Prints "120" print(statistics.2) // Also prints "120"
Swift’s syntax encourages you to write clean and consistent code which may feel strict at times, but it helps to prevent errors and improve readability. For instance, Swift requires you to write out the types of variables if there’s no initial value because that helps catch errors as early as possible in the coding process.
Note: Swift is constantly evolving with new features and capabilities being added regularly. It’s important for developers to stay updated with the latest versions.
Swift’s integration with existing Objective-C codebases allows for seamless transition and coexistence within the same project. This makes it an attractive choice for teams looking to modernize their app development without having to start from scratch.
Swift is not just another programming language; it’s the result of the latest research on programming languages, combined with decades of experience building Apple platforms. It provides safe programming patterns and adds modern features to make programming easier, more flexible, and more fun.
Overview of SpriteKit Framework
SpriteKit is Apple’s 2D game development framework that is part of the iOS and macOS SDKs. It’s designed to be easy to use and incredibly efficient, which makes it an perfect choice for swift developers looking to create interactive games. SpriteKit simplifies the process of displaying graphics and animations, handling user input, and implementing game logic.
One of the core components of SpriteKit is the SKScene, which represents a single view of the game. Ponder of it as a stage where all the action happens. Each SKScene contains a tree of SKNode objects, which can be anything from sprites (SKSpriteNode), text labels (SKLabelNode), or even custom drawing (SKShapeNode).
// Create a new scene let scene = SKScene(size: CGSize(width: 1024, height: 768)) // Create a new sprite node let sprite = SKSpriteNode(imageNamed: "Spaceship") // Add the sprite node to the scene scene.addChild(sprite)
SpriteKit also provides a physics engine that allows for realistic movement and collisions without the need for extensive coding. You can easily add physics properties to your SKNode objects, such as mass, velocity, and friction, using SKPhysicsBody.
// Add a physics body to the sprite node for collision detection sprite.physicsBody = SKPhysicsBody(texture: sprite.texture!, size: sprite.size)
Another powerful feature of SpriteKit is its actions system. Actions are used to change the properties of nodes over time, such as moving, scaling, rotating, or fading. They can be sequenced, grouped, or even repeated indefinitely.
// Move the sprite node across the screen let moveAction = SKAction.moveBy(x: 100, y: 0, duration: 1) // Rotate the sprite node let rotateAction = SKAction.rotate(byAngle: CGFloat.pi, duration: 1) // Run the actions sequentially let sequence = SKAction.sequence([moveAction, rotateAction]) sprite.run(sequence)
SpriteKit also includes support for particle systems (SKParticleEmitterNode), which can be used to create effects like fire, smoke, or rain. The framework also provides an easy way to handle user input through touch events or mouse clicks depending on the platform.
SpriteKit’s integration with Swift provides a powerful platform for creating high-performance 2D games with minimal effort. Its robust set of features allows developers to focus on creating a great gaming experience rather than dealing with low-level details.
Overall, SpriteKit provides a comprehensive set of tools that make 2D game development accessible and enjoyable. With Swift’s syntax and SpriteKit’s capabilities combined, developers have an unparalleled opportunity to create amazing games for Apple platforms.
Integrating Swift with SpriteKit for Game Development
Integrating Swift with SpriteKit for game development is a seamless process that leverages the strengths of both technologies. To start building a game using Swift and SpriteKit, you need to set up your project correctly in Xcode, Apple’s integrated development environment (IDE). Here’s how you can do it:
- Create a new Xcode project and select the ‘Game’ template.
- Choose Swift as the programming language and SpriteKit as the game technology.
- Set up your target platforms, such as iOS or macOS.
Once your project is set up, you can begin by creating your game scenes. Each scene represents a different level or screen in your game, such as the main menu, game levels, or the game-over screen.
// Define a new class for your game scene class GameScene: SKScene { override func didMove(to view: SKView) { // Set up your scene here } }
The didMove(to view: SKView)
method is called once the scene is presented by a view. That’s where you initialize your game elements and set up the scene.
One of the key aspects of game development with SpriteKit is handling user input. Swift makes it easy to respond to touch events or mouse clicks:
override func touchesBegan(_ touches: Set, with event: UIEvent?) { for touch in touches { let location = touch.location(in: self) // Respond to the touch, such as moving a character or firing a weapon } }
To animate sprites and create gameplay mechanics, you can use SpriteKit’s action system. Actions can be combined in sequences, groups, or even repeated forever. Here’s an example of how to make a sprite jump using an action:
let jumpUpAction = SKAction.moveBy(x: 0, y: 50, duration: 0.2) let jumpDownAction = SKAction.moveBy(x: 0, y: -50, duration: 0.2) let jumpSequence = SKAction.sequence([jumpUpAction, jumpDownAction]) // Run the jump action on a sprite sprite.run(jumpSequence)
For more complex games, you might need to implement a game loop for continuous updates. SpriteKit provides the update(_:)
method which is called before each frame is rendered:
override func update(_ currentTime: TimeInterval) { // Called before each frame is rendered // Update game state, such as checking for collisions or updating scores }
Remember: Performance is key in game development. SpriteKit and Swift are designed to work efficiently together, but it’s important to optimize your code and assets for the best gaming experience.
Integrating Swift with SpriteKit provides a robust environment for developing 2D games. With Swift’s clean syntax and SpriteKit’s powerful tools, you can focus on bringing your creative vision to life.
Source: https://www.plcourses.com/swift-and-spritekit/