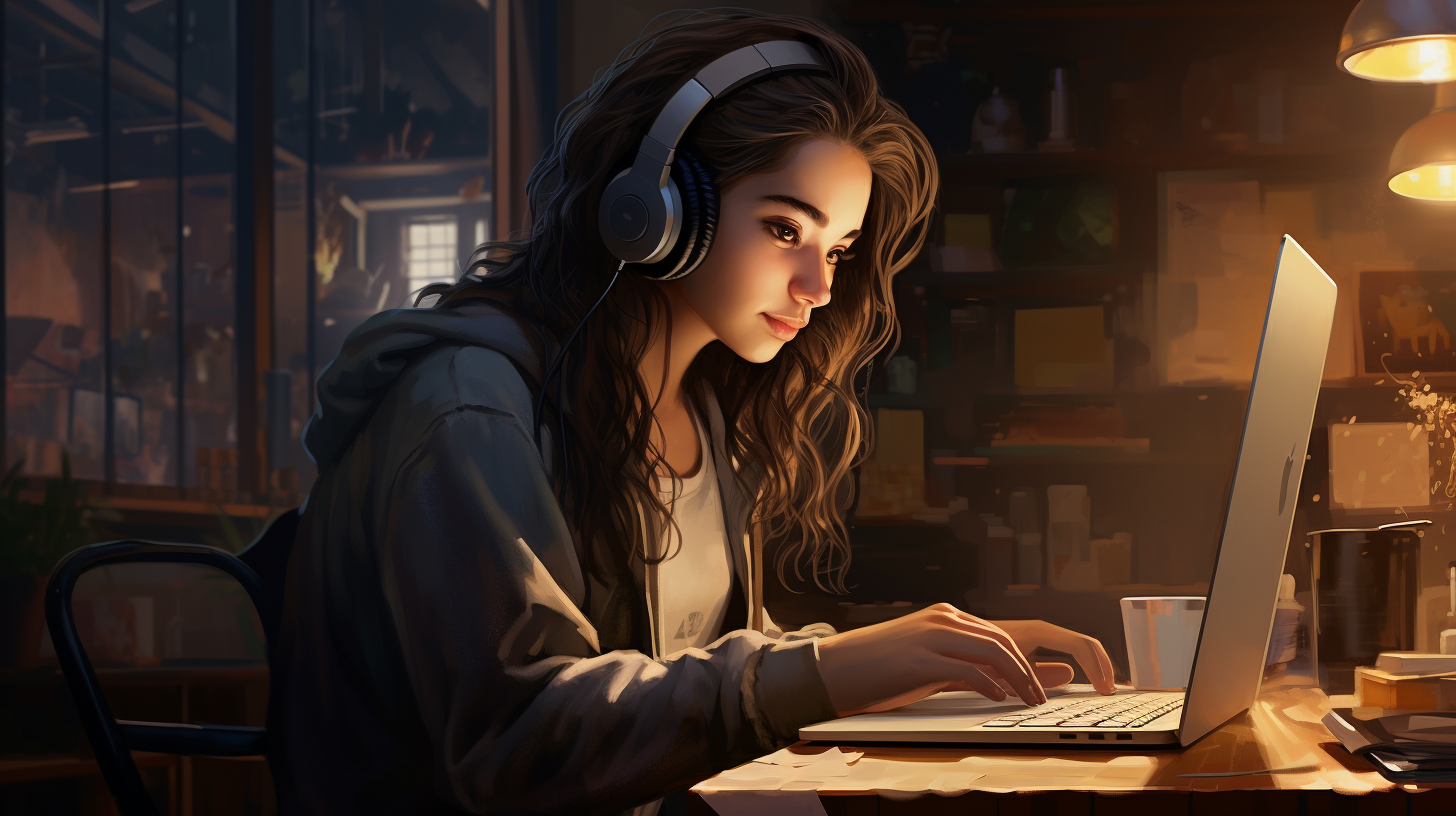
Definition and Syntax of the “this” Keyword
The this keyword in JavaScript is a special identifier that’s automatically defined in the scope of every function. It’s a context-sensitive reference that can change depending on the way a function is called, and it generally refers to the object that a function is a method of.
When it comes to the syntax, this is used just like any other variable. However, its value is determined by the execution context and cannot be set by assignment. Here’s a simple example:
function showContext() { console.log(this); } showContext(); // logs the global object (Window in browsers)
In the example above, this
inside showContext()
function refers to the global object because the function is called in the global scope.
When this is used inside an object’s method, it refers to the object itself:
const myObject = { property: 'Value', myMethod: function() { console.log(this.property); } }; myObject.myMethod(); // logs 'Value'
In this case, this
inside myMethod
refers to myObject
, so this.property
is equivalent to myObject.property
.
It’s important to understand that the value of this is not static. It depends on how the function is invoked, not how it’s defined. For instance, if you take a method out of an object, this will no longer refer to that object:
const method = myObject.myMethod; method(); // logs undefined or throws a TypeError in strict mode
This happens because method
is now being called in the global context, so this refers to the global object, which doesn’t have a property
property.
In summary, the value of this in JavaScript is determined by how a function is called. It’s important to keep track of the context in which your functions are executed to understand what this will refer to.
Binding and Execution Context in JavaScript
In JavaScript, the idea of binding and execution context plays a important role in determining the value of this
. Binding is the process of associating an identifier with a value, while execution context refers to the environment in which a piece of code is executed. The execution context determines what this
refers to, and it can change each time a function is invoked.
There are four primary ways that functions can be called in JavaScript, and each has its own rules for this
binding:
- Function Invocation: When a function is called as a standalone function,
this
refers to the global object. In strict mode,this
will beundefined
. - Method Invocation: When a function is called as a method of an object,
this
refers to the object itself. - Constructor Invocation: When a function is used as a constructor with the
new
keyword,this
refers to the newly created object. - Indirect Invocation: Functions can also be invoked indirectly using
call()
,apply()
, orbind()
, which allows for explicit setting ofthis
.
The execution context is created when a function is invoked. This context includes information about where the function was called from, how it was called, the parameters passed to it, and the value of this
. The execution stack, which is a stack of all execution contexts, keeps track of all the executing and waiting functions. The currently executing function runs in its execution context at the top of this stack.
Here’s an example illustrating different execution contexts and bindings:
function globalFunction() { console.log(this); } const myObj = { method: function() { console.log(this); } }; globalFunction(); // logs global object or undefined in strict mode myObj.method(); // logs myObj // Using call() to set this explicitly globalFunction.call(myObj); // logs myObj // Constructor invocation function MyConstructor() { console.log(this); } new MyConstructor(); // logs instance of MyConstructor
In the above code snippet, we see how this
changes based on the invocation method. Understanding this behavior and the rules governing execution contexts is vital for working effectively with JavaScript’s dynamic nature.
An important aspect of execution context is that it can create closures. A closure is a function that remembers its outer variables even after the outer function has finished executing. That’s possible because the inner function retains a reference to its execution context:
function outerFunction() { let outerVariable = 'I am outside!'; function innerFunction() { console.log(outerVariable); } return innerFunction; } const myClosure = outerFunction(); myClosure(); // logs 'I am outside!'
In this example, innerFunction()
forms a closure. It remembers the variable outerVariable
from the outer scope, even after outerFunction()
has executed and its execution context is no longer on the stack.
To sum up, understanding binding and execution context in JavaScript is essential for mastering the behavior of this
. It enables developers to predict and control how functions will behave in different scenarios.
Common Use Cases and Pitfalls with the “this” Keyword
One common use case for this in JavaScript is within event handlers. When an event is triggered, the this keyword inside the event handler function will refer to the element that received the event:
button.addEventListener('click', function() { console.log(this); // refers to the button element });
However, a common pitfall occurs when using this within a callback function that’s not an object method. In such cases, this may not refer to the object you expect:
const myObject = { myMethod: function() { setTimeout(function() { console.log(this); // does not refer to myObject, but to the global object or undefined in strict mode }, 1000); } }; myObject.myMethod();
To remedy this, one can use ES6 arrow functions, which do not have their own this and inherit it from the enclosing execution context:
const myObject = { myMethod: function() { setTimeout(() => { console.log(this); // correctly refers to myObject }, 1000); } }; myObject.myMethod();
Another common scenario is in constructor functions. When a function is used as a constructor with the new
keyword, this refers to the newly created instance:
function Person(name) { this.name = name; } const person1 = new Person('John'); console.log(person1.name); // logs 'John'
However, if you forget to use the new
keyword, this will refer to the global object, leading to unintended consequences:
function Person(name) { this.name = name; } const person1 = Person('John'); // missing 'new' keyword console.log(name); // 'John' is added to the global object
In conclusion, while this is an incredibly powerful feature of JavaScript, it can easily lead to bugs if not used carefully. Understanding its behavior in different contexts and remembering to use arrow functions or binding methods such as bind()
, call()
, or apply()
can help avoid common pitfalls associated with this.
Alternative Approaches and Best Practices for Managing “this” in JavaScript
As you become more experienced with JavaScript, you may find that managing the this
keyword can become quite complex, especially in larger applications. However, there are alternative approaches and best practices that can help simplify this process. Below are some strategies that can be applied:
- Arrow Functions: As mentioned earlier, arrow functions do not have their own
this
context and inherit it from the enclosing scope. This feature can be particularly useful when dealing with asynchronous code, such as callbacks and promises.
const myObject = { myMethod: function() { setTimeout(() => { console.log(this); // correctly refers to myObject }, 1000); } }; myObject.myMethod();
bind()
method which creates a new function with this
bound to a specific object. This can be helpful when passing functions as arguments or setting them as event listeners.const myObject = { myMethod: function() { console.log(this); } }; const boundMethod = myObject.myMethod.bind(myObject); setTimeout(boundMethod, 1000); // logs myObject
call()
and apply()
: These methods are similar to bind()
, but they immediately invoke the function with a specified this
value and arguments. This can be useful for borrowing methods from other objects.function greet() { console.log(`Hello, my name is ${this.name}`); } const person = { name: 'Alice' }; greet.call(person); // logs "Hello, my name is Alice"
this
Altogether: Sometimes, the best approach is to avoid using this
entirely. You can do this by using closures or by passing objects explicitly as arguments to functions.function greet(person) { console.log(`Hello, my name is ${person.name}`); } const person = { name: 'Alice' }; greet(person); // logs "Hello, my name is Alice"
In addition to these strategies, it’s also important to follow some general best practices:
- Always use strict mode (
'use strict';
) to avoid accidentally creating global variables. - Be mindful of the context in which your functions are called, especially when using third-party libraries.
- If you’re working with classes, ponder using class fields to bind methods within the constructor.
Remember, managing
this
effectively is key to writing clean and maintainable JavaScript code. Don’t hesitate to use the tools and techniques available to you to make your life easier.
Source: https://www.plcourses.com/understanding-this-keyword-in-javascript/