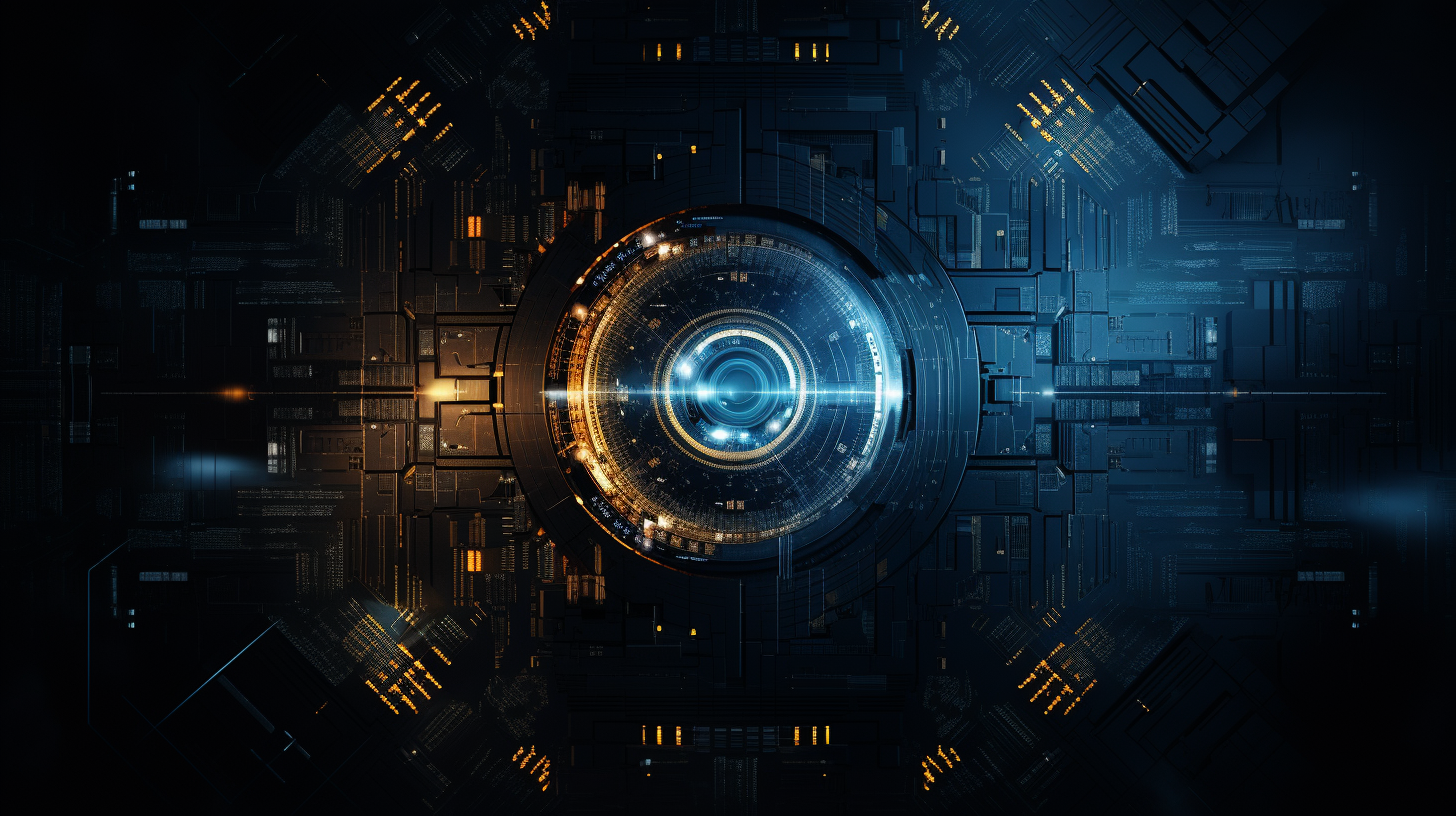
Introduction to PHP Loops
Loops are one of the most fundamental and powerful concepts in programming. They allow you to execute a block of code repeatedly, which can be incredibly useful for tasks that involve repetitive actions. In PHP, there are three main types of loops: for, while, and foreach.
The for loop is used when you know in advance how many times you want to execute a statement or a block of statements. It’s defined by three expressions: the initialization, the condition, and the increment.
for ($i = 0; $i < 10; $i++) { echo $i; }
The while loop is used when you want to execute a block of code as long as a certain condition is true. The condition is evaluated before the execution of the loop’s body.
$counter = 0; while ($counter < 10) { echo $counter; $counter++; }
The foreach loop is used for iterating over arrays and objects. It provides an easy way to access each key/value pair within an array.
$colors = array("red", "green", "blue", "yellow"); foreach ($colors as $color) { echo $color; }
Each type of loop has its own use cases and understanding when to use which loop is essential for writing efficient and readable code. In the following sections, we will explore each of these loops in more detail and provide examples of how to use them effectively.
Exploring the For Loop in PHP
When it comes to the for loop in PHP, it’s one of the most commonly used loop structures. As mentioned earlier, it consists of three main parts: the initialization, the condition, and the increment or decrement operation. Let’s explore each of these components in more detail.
The initialization is executed only once at the beginning of the loop. It usually initializes one or more loop counters, but it can also include other code that you want to run just once before the loop starts. For example:
for ($i = 0; ...; ...) { // Initialization code }
The condition is evaluated at the beginning of each iteration. If it evaluates to true, the loop body is executed. If it evaluates to false, the loop ends. Here’s an example:
for (...; $i < 10; ...) { // Loop body }
The increment/decrement operation is executed at the end of each iteration. It’s typically used to update the loop counter, but it can also include other code that you want to run at the end of each loop iteration. For instance:
for (...; ...; $i++) { // Increment operation }
Now let’s put all these components together in a simple for loop
example:
for ($i = 0; $i < 10; $i++) { echo "The number is: $in"; }
This loop will print out the numbers 0 through 9. The $i
variable starts at 0, and as long as $i
is less than 10, the loop will continue. After each iteration, $i
is incremented by 1.
You can also use a for loop
to iterate over arrays, although the foreach loop
is often a simpler option for that purpose. Here’s how you might do it with a for loop
:
$fruits = array("apple", "banana", "cherry"); for ($i = 0; $i < count($fruits); $i++) { echo "Fruit: " . $fruits[$i] . "n"; }
In this example, we’re using count($fruits)
to determine the number of elements in the array, and then we’re using that number to control how many times the loop runs.
The for loop
is very versatile and can be used in a variety of different ways. However, it’s important to ensure that your condition will eventually evaluate to false, otherwise you may end up with an infinite loop!
Here’s an example of a common mistake that can lead to an infinite loop:
for ($i = 10; $i >= 0; $i++) { // This will run forever because $i will never be less than 0 }
To avoid this, make sure that your increment/decrement operation will eventually cause the condition to become false.
To wrap it up, the for loop
is a powerful tool in PHP that can help you execute code a specific number of times. By understanding and manipulating its three main components – initialization, condition, and increment/decrement – you can control exactly how many times your loop runs and what it does each time.
Understanding the While and Foreach Loops in PHP
The while loop is quite similar to the for loop in that it continues to execute a block of code as long as a certain condition is true. However, the while loop is often used in situations where the number of iterations is not known beforehand. The basic syntax of a while loop is as follows:
$counter = 0; while ($counter < 10) { echo "Counter value: $countern"; $counter++; }
In this example, the loop will continue to run as long as $counter
is less than 10. Inside the loop, we print out the current value of $counter
and then increment it by 1. If we forget to increment $counter
, the condition will always be true, and we would end up with an infinite loop.
Another common use of the while loop is to read data from a resource until there is no more data to read. For example, reading lines from a file until the end of the file is reached:
$handle = fopen("file.txt", "r"); if ($handle) { while (($line = fgets($handle)) !== false) { echo $line; } fclose($handle); }
In this code snippet, we open a file for reading and then use a while loop to read each line until fgets()
returns false, which indicates the end of the file.
The foreach loop, on the other hand, is specifically designed for iterating over arrays and objects. It’s the most straightforward way to access each element in an array without worrying about the keys or keeping track of the number of elements. Here’s a basic syntax of a foreach loop:
$colors = array("red", "green", "blue", "yellow"); foreach ($colors as $color) { echo "Color: $colorn"; }
This loop will output each color in the array. The $color
variable takes on the value of each array element in turn.
You can also use foreach to get both keys and values from an associative array:
$person = array("name" => "John", "age" => 30, "job" => "developer"); foreach ($person as $key => $value) { echo "$key: $valuen"; }
In this example, $key
will be the name of the current element’s key, and $value
will be the value of that element.
Understanding when to use a while or foreach loop comes with practice and depends on what you’re trying to accomplish. While loops are great when you’re working with conditions that need to be checked before each iteration, and foreach loops are ideal for working with arrays and objects where you need to access each element.
Source: https://www.plcourses.com/php-loops-for-while-foreach/