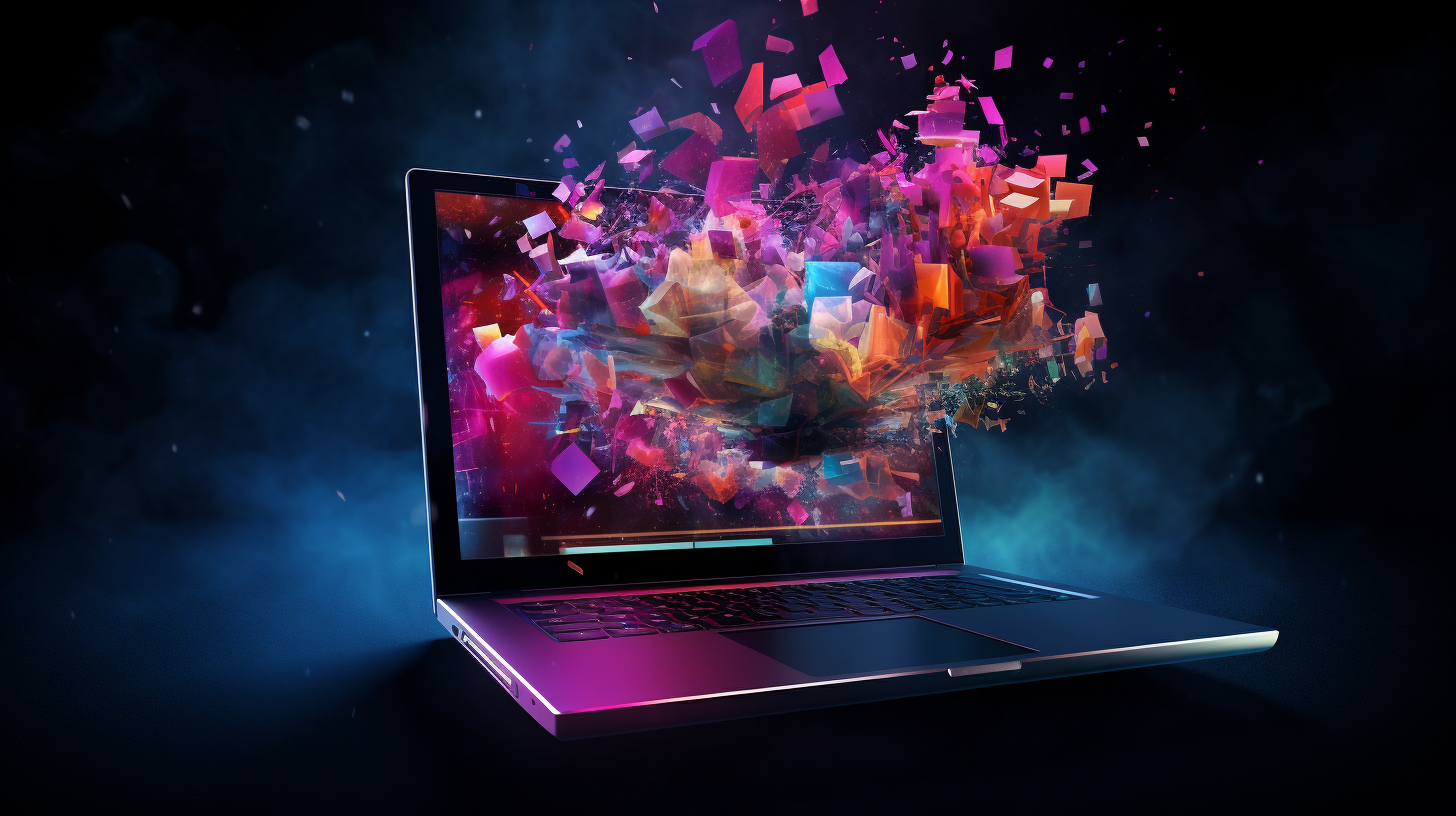
MongoDB is a NoSQL database that offers high performance, high availability, and easy scalability. It is an open-source document database, which means it stores data in JSON-like documents. This provides a flexible schema that can easily adapt to changes in your application’s data structure. MongoDB is widely used for big data and high volume data storage.
When working with MongoDB in Python, the library of choice is PyMongo. PyMongo is a Python distribution containing tools for working with MongoDB, and is the recommended way to work with MongoDB from Python. It provides a rich set of features for interacting with the database, such as querying, inserting, updating, and deleting documents, as well as managing database users and roles.
Here’s a simple example to connect to a MongoDB using PyMongo:
from pymongo import MongoClient # create a MongoClient to the running mongod instance client = MongoClient('localhost', 27017) # getting a database db = client['example-database'] # getting a collection collection = db['example-collection']
Once you have obtained a collection, you can start performing various operations on it, such as inserting documents or querying for data. The flexibility of MongoDB combined with the power of PyMongo makes it an excellent choice for Python developers who need to work with large or variable data sets.
User Authentication in MongoDB
Authentication is a critical aspect of managing users in MongoDB. It involves verifying the identity of a user before they are allowed access to the database. MongoDB supports various authentication mechanisms, such as SCRAM, x.509 certificates, LDAP, and Kerberos. The most common and default authentication method used is SCRAM (Salted Challenge Response Authentication Mechanism).
To authenticate a user using PyMongo, you must first ensure that the MongoDB instance is running with access control enabled. This can be done by starting mongod with the --auth
option. Once access control is enabled, you can create users with various roles and permissions.
Here is an example of how to create a new user in MongoDB using PyMongo:
from pymongo import MongoClient from pymongo.errors import OperationFailure # connect to the MongoDB server client = MongoClient('localhost', 27017) # get the admin database admin_db = client['admin'] # authenticate as the admin user admin_db.authenticate('admin_username', 'admin_password') # create a new user with read and write access to the 'example-database' try: admin_db.command("createUser", "new_username", pwd="new_password", roles=[{"role": "readWrite", "db": "example-database"}]) print("User created successfully.") except OperationFailure as e: print(f"Error creating user: {e}")
After creating a user, you can authenticate as that user when connecting to the database. Here is how you can authenticate using PyMongo:
from pymongo import MongoClient # create a MongoClient object with the necessary URI client = MongoClient('mongodb://new_username:new_password@localhost:27017/example-database') # get the database db = client['example-database'] # verify if authentication was successful try: # perform a database operation to trigger the authentication db.command('ping') print("Authenticated successfully.") except Exception as e: print(f"Authentication failed: {e}")
It’s important to handle exceptions when performing authentication-related operations since they can fail due to incorrect credentials or network issues.
Once authenticated, you can use the database client to perform any further operations that the user has permission for. Managing user authentication properly ensures that only authorized users have access to sensitive data within your MongoDB databases.
Managing User Roles and Permissions
Managing user roles and permissions is essential for maintaining security and ensuring that users have the appropriate level of access to the database. MongoDB provides a flexible role-based access control system that allows you to define custom roles with granular permissions.
To create a new role, you can use the createRole
command. Here’s an example:
from pymongo import MongoClient # connect to the MongoDB server client = MongoClient('localhost', 27017) # get the admin database admin_db = client['admin'] # authenticate as the admin user admin_db.authenticate('admin_username', 'admin_password') # create a new role with specific privileges try: admin_db.command("createRole", "customRole", privileges=[ {"resource": {"db": "example-database", "collection": ""}, "actions": ["find", "insert", "update"]} ], roles=[]) print("Role created successfully.") except Exception as e: print(f"Error creating role: {e}")
Once you have created a custom role, you can assign it to a user using the grantRolesToUser
command:
# grant the custom role to an existing user try: admin_db.command("grantRolesToUser", "existing_username", roles=["customRole"]) print("Role granted successfully.") except Exception as e: print(f"Error granting role: {e}")
It’s also possible to revoke roles from a user using the revokeRolesFromUser
command:
# revoke the custom role from an existing user try: admin_db.command("revokeRolesFromUser", "existing_username", roles=["customRole"]) print("Role revoked successfully.") except Exception as e: print(f"Error revoking role: {e}")
When managing user roles and permissions, it’s important to regularly review and update the roles as necessary to adapt to changes in your application’s requirements and security policies. This will help ensure that users only have access to the data and operations they need to perform their job functions, and no more.
Proper role management, combined with careful user authentication, forms the cornerstone of a secure MongoDB deployment. By using PyMongo, you can programmatically manage roles and permissions, making it easier to integrate these tasks into your application’s administrative interface or deployment pipeline.
Implementing User Registration and Login
Implementing user registration and login functionality is a key aspect of user management in MongoDB using PyMongo. It allows users to create accounts, securely authenticate their identity, and access personalized content or features within your application.
To begin with, let’s implement a user registration system. You’ll need to collect user details such as username, password, and possibly email or other personal information. It’s important to hash passwords before storing them in the database to enhance security. Here’s a Python function to register a new user:
from pymongo import MongoClient from werkzeug.security import generate_password_hash def register_user(username, password, email): client = MongoClient('localhost', 27017) db = client['user_database'] users_collection = db['users'] # Check if the username already exists if users_collection.find_one({'username': username}): return "Username already exists" # Hash the password hashed_password = generate_password_hash(password) # Insert the new user into the database users_collection.insert_one({ 'username': username, 'password': hashed_password, 'email': email }) return "User registered successfully"
For user login, you’ll need to verify the username and password provided against those stored in the database. Here’s how you can implement a login function:
from werkzeug.security import check_password_hash def login_user(username, password): client = MongoClient('localhost', 27017) db = client['user_database'] users_collection = db['users'] # Find the user by username user = users_collection.find_one({'username': username}) # Check if user exists and verify password if user and check_password_hash(user['password'], password): return "Logged in successfully" else: return "Invalid credentials"
In both functions, we use the werkzeug.security
library to hash passwords and verify hashed passwords, which is a common practice in web development for security reasons.
By implementing these registration and login systems, you can create a secure way for users to authenticate with your MongoDB database. It’s important to note that you should always use HTTPS to encrypt data transmitted between the client and server during registration and login processes to prevent potential eavesdropping.
Additionally, it’s a good practice to implement measures such as account lockouts after multiple failed login attempts, password complexity requirements, and multi-factor authentication to further enhance security.
When designing your user management system, always consider the principles of least privilege and defense in depth to minimize the risk of unauthorized access to sensitive data.
Best Practices for User Management in MongoDB
When managing users in MongoDB, it’s essential to follow best practices to ensure the security and integrity of your data. Here are some best practices for user management in MongoDB:
- Use Strong, Unique Passwords: Always encourage or enforce the use of strong, unique passwords for MongoDB user accounts. This can be done by implementing password policies that require a mix of uppercase and lowercase letters, numbers, and special characters.
- Regularly Rotate Passwords: Implement a system for regular password rotation to reduce the risk of compromised credentials. Notify users to update their passwords at set intervals, such as every 90 days.
- Limit Access with Roles: Assign users only the roles they need to perform their tasks. Avoid giving unnecessary permissions that can lead to security vulnerabilities.
- Audit User Activities: Keep track of user activities within the database. You can use MongoDB’s auditing feature to log operations performed by users, which can help identify unauthorized actions or breaches.
- Implement Account Lockout Mechanisms: To prevent brute force attacks, implement account lockout mechanisms after a certain number of failed login attempts.
- Use TLS/SSL Connections: Always use TLS/SSL connections for data in transit between your application and MongoDB to prevent eavesdropping and man-in-the-middle attacks.
Here’s an example of how to enforce password policies by checking the password complexity before creating a new user account:
import re from pymongo import MongoClient def create_user(username, password): if not re.match("^(?=.*[a-z])(?=.*[A-Z])(?=.*d)(?=.*[@$!%*?&])[A-Za-zd@$!%*?&]{8,}$", password): return "Password does not meet complexity requirements" client = MongoClient('localhost', 27017) db = client['user_database'] users_collection = db['users'] # Rest of the user creation logic...
In addition to these best practices, it’s also important to keep your MongoDB server and PyMongo library up to date with the latest security patches. Regularly review your security settings and update them as needed to stay ahead of potential threats.
By adhering to these best practices for user management in MongoDB, you can create a more secure environment for your data and protect your application from unauthorized access.
Source: https://www.pythonlore.com/authenticating-and-managing-users-in-mongodb-with-pymongo/