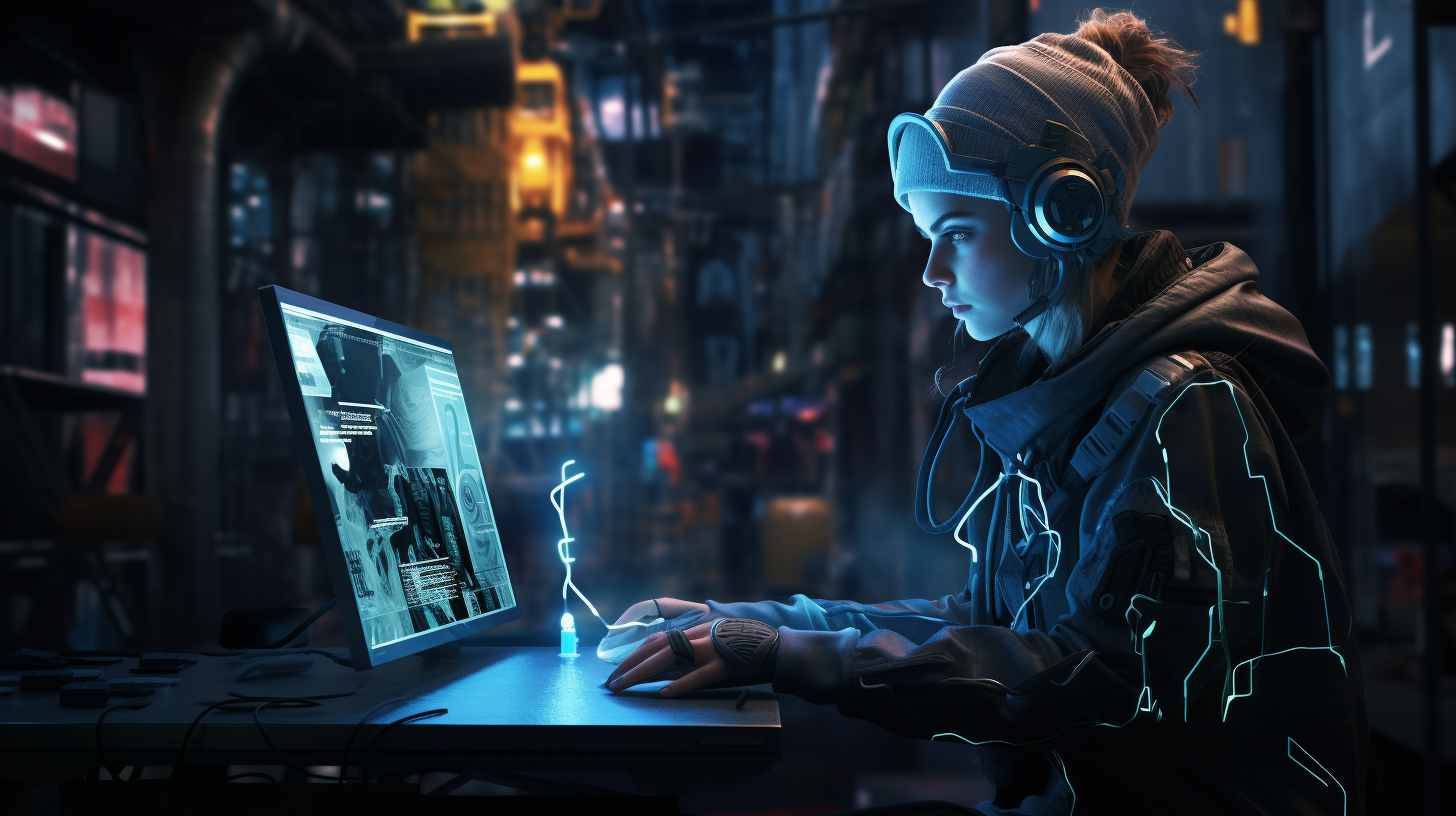
Python is a powerful programming language that can be applied in various domains, including marine biology. In this tutorial, we will explore how Python can be used for data analysis in the field of marine biology. We will dive into concepts and provide code examples to illustrate each step of the analysis process.
Data Collection:
Before we begin our data analysis, it’s important to understand how data is collected in marine biology. Marine biologists use a variety of methods to gather data, ranging from manually recording observations to using specialized instruments. Once the data has been collected, it’s often stored in spreadsheets or databases for further analysis.
Data Cleaning and Preparation:
Typically, marine biology datasets contain errors, missing values, and inconsistencies. Therefore, the first step in any data analysis is to clean and prepare the data. Python provides powerful libraries such as Pandas for data manipulation. Let’s ponder an example where we have a dataset of ocean temperatures:
import pandas as pd # Load the dataset data = pd.read_csv('ocean_temperatures.csv') # Check the dimensions of the dataset print("Number of rows:", data.shape[0]) print("Number of columns:", data.shape[1]) # Remove duplicate rows data.drop_duplicates(inplace=True) # Handle missing values data.dropna(inplace=True) # Replace outliers with median values median = data['temperature'].median() data.loc[data['temperature'] > 50, 'temperature'] = median # Normalize the temperature values data['temperature'] = (data['temperature'] - data['temperature'].min()) / (data['temperature'].max() - data['temperature'].min()) # Save the cleaned dataset data.to_csv('cleaned_ocean_temperatures.csv', index=False)
In the above code, we use Pandas to load the dataset and perform various data cleaning operations. We remove duplicate rows, handle missing values by dropping them, replace outliers with the median temperature value, and normalize the temperature values. Finally, we save the cleaned dataset to a new CSV file.
Data Analysis and Visualization:
Once the data has been cleaned and prepared, we can move on to the analysis phase. Python offers several libraries that are widely used for data analysis and visualization, including NumPy and Matplotlib. Let’s continue with our example and analyze the relationship between ocean temperature and marine life:
import numpy as np import matplotlib.pyplot as plt # Load the cleaned dataset cleaned_data = pd.read_csv('cleaned_ocean_temperatures.csv') # Calculate the mean temperature mean_temperature = cleaned_data['temperature'].mean() # Calculate the correlation between temperature and marine life correlation = np.corrcoef(cleaned_data['temperature'], cleaned_data['marine_life'])[0, 1] # Create a scatter plot of temperature vs. marine life plt.scatter(cleaned_data['temperature'], cleaned_data['marine_life']) plt.xlabel('Temperature') plt.ylabel('Marine Life') plt.title('Temperature vs. Marine Life') plt.axhline(y=mean_temperature, color='r', linestyle='--', label='Mean Temperature') plt.legend() plt.show()
In this code snippet, we load the cleaned dataset and calculate the mean temperature and the correlation between temperature and marine life. We then create a scatter plot using Matplotlib to visualize the relationship between temperature and marine life. Additionally, we add a horizontal line representing the mean temperature and a legend to the plot.
Statistical Analysis:
Python provides various statistical analysis libraries that can be utilized in marine biology research. One popular library is SciPy, which offers a wide range of statistical functions. Let’s perform a hypothesis test to compare the mean temperature of two different ocean regions:
from scipy.stats import ttest_ind # Load the temperature data for two different regions region1_data = pd.read_csv('temperature_region1.csv') region2_data = pd.read_csv('temperature_region2.csv') # Perform an independent t-test statistic, p_value = ttest_ind(region1_data['temperature'], region2_data['temperature']) # Print the test statistic and p-value print("Test Statistic:", statistic) print("p-value:", p_value)
In the above code, we use SciPy’s ttest_ind function to perform an independent t-test on the temperature data for two different regions. The function returns the test statistic and the p-value, which can be used to make statistical conclusions.
Machine Learning:
Python’s machine learning libraries are also valuable tools in marine biology research. They can be used to build predictive models and classify marine species based on various features. Let’s consider an example where we use scikit-learn to classify fish species based on their physical characteristics:
from sklearn.model_selection import train_test_split from sklearn.neighbors import KNeighborsClassifier from sklearn.metrics import accuracy_score # Load the fish species dataset fish_species_data = pd.read_csv('fish_species.csv') # Split the dataset into training and testing sets X = fish_species_data.drop('species', axis=1) y = fish_species_data['species'] X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Build and train the KNN classifier knn_classifier = KNeighborsClassifier(n_neighbors=3) knn_classifier.fit(X_train, y_train) # Make predictions on the test set y_pred = knn_classifier.predict(X_test) # Calculate the accuracy of the classifier accuracy = accuracy_score(y_test, y_pred) print("Accuracy:", accuracy)
In this code, we load a dataset containing fish species information and split it into training and testing sets using the train_test_split function from scikit-learn. We then build a K-nearest neighbors (KNN) classifier and train it on the training set. Finally, we make predictions on the test set and calculate the accuracy of the classifier using accuracy_score.
Python is a versatile language that can be effectively applied in marine biology for data analysis. It provides powerful libraries for data manipulation, analysis, visualization, statistical analysis, and machine learning. By leveraging Python’s capabilities, marine biologists can gain valuable insights from their data and make informed decisions in their research.
In this tutorial, we explored the use of Python for data analysis in marine biology step by step. Starting from data cleaning and preparation using Pandas, we moved on to data analysis and visualization with NumPy and Matplotlib. We then delved into statistical analysis using SciPy and finally demonstrated how scikit-learn can be used for machine learning tasks in marine biology. Python’s vast ecosystem of libraries makes it a valuable tool for marine biologists working with large and complex datasets.
Keep exploring and analyzing!
Source: https://www.plcourses.com/python-and-marine-biology-data-analysis/