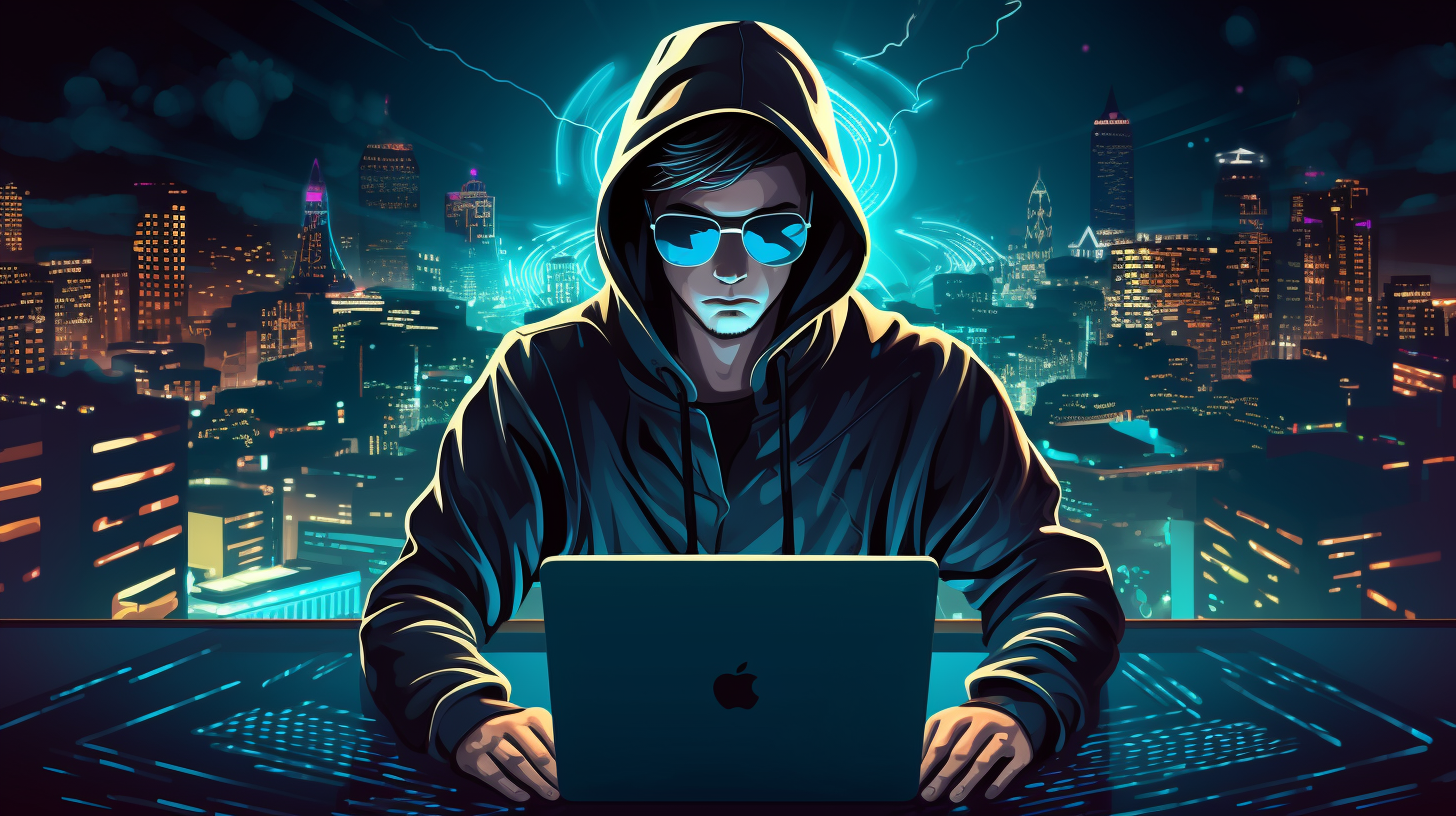
Data cleanup is an essential aspect of data management and analysis that involves removing or correcting inaccurate, incomplete, or irrelevant data from a database. SQL (Structured Query Language) provides a variety of techniques that can help in cleansing the data effectively. Here we will discuss some key SQL techniques for data cleanup with detailed explanations and code examples.
1. Removing Duplicate Rows
Duplicate rows can lead to erroneous data analysis and reports. It’s important to identify and remove any duplicates. Here’s how you can do it in SQL:
DELETE FROM table_name WHERE id NOT IN ( SELECT MIN(id) FROM table_name GROUP BY column1, column2, ..., columnN);
In the above code, replace table_name
with your table name and column1, column2, ..., columnN
with the columns you want to check for duplicates. This will keep one instance of the duplicate row and remove the rest.
2. Correcting Data Inconsistencies
Data inconsistencies occur when the data is not aligned with the expected format or values. To correct this, you can use the UPDATE
statement:
UPDATE table_name SET column_name = 'Correct Value' WHERE column_name = 'Incorrect Value';
Replace table_name
, column_name
, and 'Correct Value'
, and 'Incorrect Value'
with your specifics.
3. Dealing With Missing Values
Missing values in your dataset can also cause issues. You may want to either remove these or replace them with a placeholder value using IS NULL
condition:
-- To delete rows with missing values DELETE FROM table_name WHERE column_name IS NULL; -- To replace missing values UPDATE table_name SET column_name = 'Default Value' WHERE column_name IS NULL;
Replace table_name
, column_name
, and 'Default Value'
as needed.
4. Trimming Whitespaces
Unwanted whitespaces can cause unexpected results in queries. Use the TRIM()
function to remove them:
UPDATE table_name SET column_name = TRIM(column_name);
This will remove both leading and trailing spaces from the specified column.
5. Standardizing Date Formats
If your dates are not standardized, this might affect sorting and other date operations. Use the STR_TO_DATE()
function in MySQL, for example, to standardize date formats:
UPDATE table_name SET column_name = STR_TO_DATE(column_name, '%m/%d/%Y') WHERE column_name REGEXP '^[0-9]{2}/[0-9]{2}/[0-9]{4}$';
This converts dates in the format mm/dd/yyyy to a standard MySQL date format.
6. Converting Data Types
Sometimes, it might be necessary to change the data type of a column, such as converting a VARCHAR to an INT. Use the CAST()
or CONVERT()
functions:
ALTER TABLE table_name MODIFY column_name INT; UPDATE table_name SET column_name = CAST(column_name AS UNSIGNED);
This will change the datatype and update the existing values to integers.
7. Normalization with Look-Up Tables
If there are repeating values in a column that can be normalized, ponder creating a lookup table and replacing those values with foreign keys:
-- Create lookup table CREATE TABLE lookup_table ( lookup_id INT AUTO_INCREMENT, value VARCHAR(255), PRIMARY KEY (lookup_id) ); -- Insert unique values into lookup table and update original table INSERT INTO lookup_table (value) SELECT DISTINCT column_name FROM table_name; UPDATE table_name t1 INNER JOIN lookup_table t2 ON t1.column_name = t2.value SET t1.column_name = t2.lookup_id;
This normalizes the data and reduces redundancy.
In conclusion, SQL provides powerful tools and functions for cleaning up data efficiently. By employing techniques like removing duplicates, correcting inconsistencies, dealing with missing values, trimming whitespaces, standardizing date formats, converting data types, and normalization with look-up tables, you can ensure that your dataset is clean and reliable for analysis.
Source: https://www.plcourses.com/sql-techniques-for-data-cleanup/