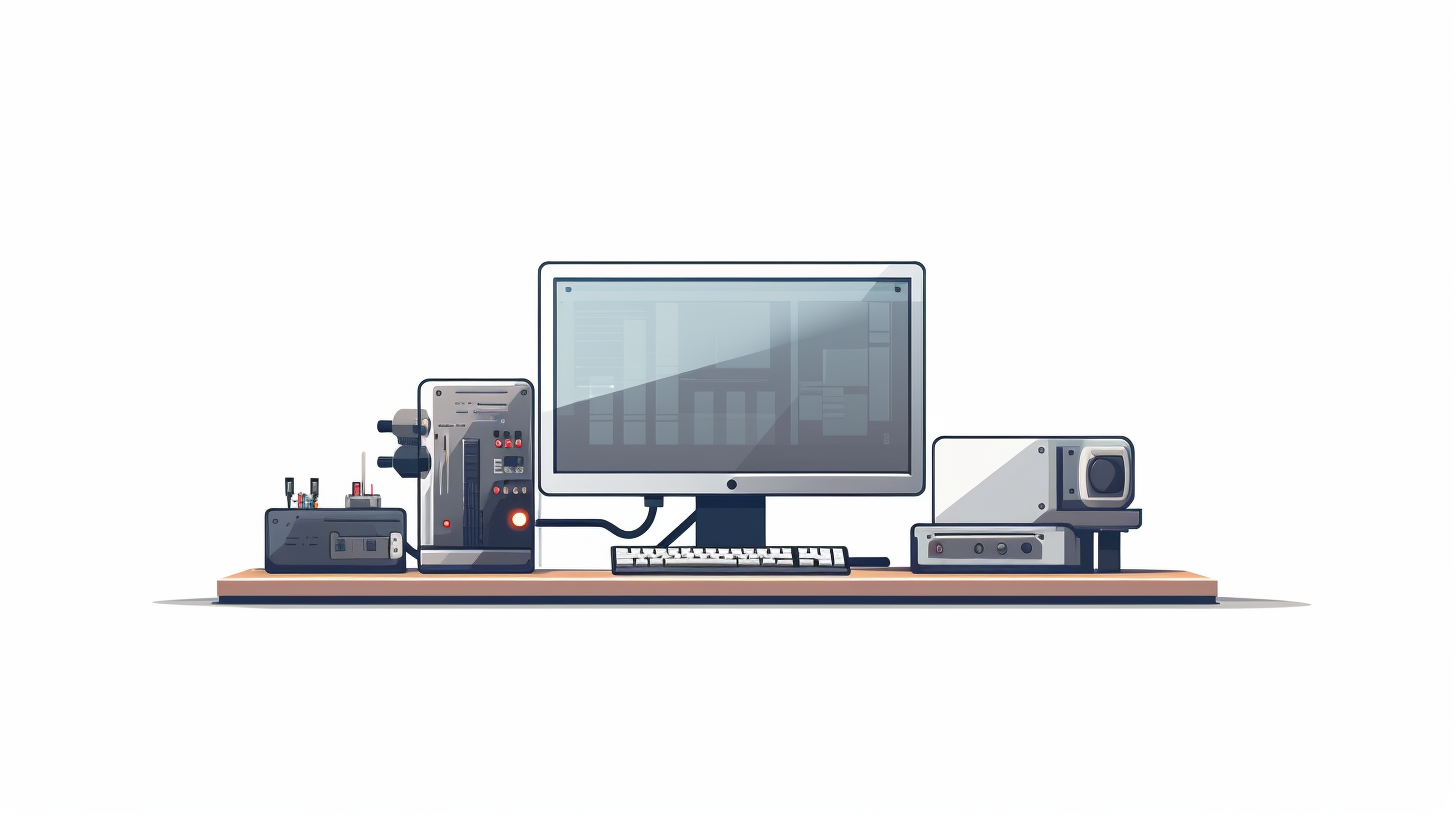
Introduction to JavaScript Data Structures
Data structures are a important aspect of programming, as they determine how data is organized, managed, and stored. In JavaScript, there are several built-in data structures that developers should be familiar with. Understanding these data structures is essential for writing efficient and effective code.
The most basic data structure in JavaScript is the Array. Arrays are used to store lists of values and can be manipulated using various array methods. Here’s an example of creating and manipulating an array in JavaScript:
let fruits = ['apple', 'banana', 'cherry']; console.log(fruits.length); // Output: 3 fruits.push('date'); console.log(fruits); // Output: ['apple', 'banana', 'cherry', 'date']
Another fundamental data structure is the Object. Objects are used to store key-value pairs and can represent more complex data. Here’s an example of creating and using an object in JavaScript:
let person = { firstName: 'John', lastName: 'Doe', age: 30 }; console.log(person.firstName); // Output: John person.job = 'Developer'; console.log(person); // Output: {firstName: 'John', lastName: 'Doe', age: 30, job: 'Developer'}
JavaScript also supports Sets and Maps. A Set is a collection of unique values, while a Map is a collection of key-value pairs where keys are unique. Here’s how you can use a Set and a Map:
let mySet = new Set([1, 2, 3, 4, 5]); console.log(mySet.has(3)); // Output: true mySet.add(6); console.log(mySet); // Output: Set(6) {1, 2, 3, 4, 5, 6} let myMap = new Map([ ['key1', 'value1'], ['key2', 'value2'] ]); console.log(myMap.get('key1')); // Output: value1 myMap.set('key3', 'value3'); console.log(myMap); // Output: Map(3) {'key1' => 'value1', 'key2' => 'value2', 'key3' => 'value3'}
These are just a few examples of the data structures available in JavaScript. Each has its use cases and can be combined in various ways to solve complex problems. As we delve deeper into JavaScript algorithms, we’ll see how these data structures are put to use.
Common JavaScript Algorithms
Algorithms are a set of instructions or steps to solve a problem or perform a computation. In JavaScript, common algorithms include sorting, searching, and traversing data structures. Let’s take a closer look at some of these algorithms and how they can be implemented in JavaScript.
Sorting Algorithms:
Sorting is a common operation in many applications. JavaScript has a built-in .sort()
method for arrays, but understanding how sorting algorithms work is important for any developer. Here are two popular sorting algorithms:
- Bubble Sort: A simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. The pass through the list is repeated until the list is sorted.
function bubbleSort(arr) { let len = arr.length; for (let i = 0; i len; i++) { for (let j = 0; j len - i - 1; j++) { if (arr[j] > arr[j + 1]) { let temp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = temp; } } } return arr; }
function quickSort(arr) { if (arr.length = 1) { return arr; } let pivot = arr[0]; let left = []; let right = []; for (let i = 1; i arr.length; i++) { arr[i] pivot ? left.push(arr[i]) : right.push(arr[i]); } return quickSort(left).concat(pivot, quickSort(right)); }
Searching Algorithms:
Searching algorithms are used to retrieve information stored within some data structure. Here’s an example of a linear search and a binary search algorithm:
- Linear Search: A simple search algorithm that checks every element in the list until the desired element is found or the list ends.
function linearSearch(arr, target) { for (let i = 0; i arr.length; i++) { if (arr[i] === target) { return i; } } return -1; }
function binarySearch(arr, target) { let left = 0; let right = arr.length - 1; while (left = right) { const mid = Math.floor((left + right) / 2); if (arr[mid] === target) { return mid; } else if (arr[mid] target) { left = mid + 1; } else { right = mid - 1; } } return -1; }
Implementing these algorithms can improve your understanding of data structures and their complexities. These examples represent just a few of the foundational algorithms that can be used to manipulate and traverse JavaScript data structures effectively.
Implementing Data Structures in JavaScript
<= 1) { return arr; } const pivot = arr[arr.length - 1]; const leftArr = []; const rightArr = []; for (const el of arr.slice(0, arr.length - 1)) { el pivot ? leftArr.push(el) : rightArr.push(el); } return [...quickSort(leftArr), pivot, ...quickSort(rightArr)]; }
Searching Algorithms:
-
Linear Search: This is the simplest searching algorithm where each element of the array is checked sequentially until the desired element is found or the end of the array is reached.
function linearSearch(arr, target) { for (let i = 0; i arr.length; i++) { if (arr[i] === target) { return i; } } return -1; }
-
Binary Search: A more efficient algorithm used for sorted arrays. It works by repeatedly dividing the search interval in half. If the value of the search key is less than the item in the middle of the interval, narrow the interval to the lower half. Otherwise, narrow it to the upper half.
function binarySearch(sortedArr, target) { let start = 0; let end = sortedArr.length - 1; while (start <= end) { const mid = Math.floor((start + end) / 2); if (sortedArr[mid] === target) { return mid; } else if (sortedArr[mid] target) { start = mid + 1; } else { end = mid - 1; } } return -1; }
Traversing Data Structures:
- Tree Traversal: In a tree data structure, traversal refers to the process of visiting each node in the tree exactly once. There are several methods to traverse a tree, such as pre-order, in-order, and post-order traversal.
- Graph Traversal: Graphs can be traversed using algorithms like Depth-First Search (DFS) and Breadth-First Search (BFS).
These algorithms are fundamental for manipulating data structures and are often used as building blocks for more complex operations.
Now that we’ve covered some common algorithms let’s move on to how we can implement data structures in JavaScript.
Arrays and Objects
As we’ve seen earlier, Arrays and Objects are native JavaScript data structures that can be used right out of the box. However, sometimes we need more control over our data structures, or we need to implement custom behavior that isn’t provided by the default implementations.
For example, let’s say we want to implement a stack using an array. A stack is a Last-In-First-Out (LIFO) data structure where elements are added and removed from the same end. Here’s how we can create a simple stack:
class Stack { constructor() { this.items = []; } push(item) { this.items.push(item); } pop() { if (this.items.length === 0) { throw new Error("Stack is empty"); } return this.items.pop(); } peek() { return this.items[this.items.length - 1]; } isEmpty() { return this.items.length === 0; } } const stack = new Stack(); stack.push("apple"); stack.push("banana"); console.log(stack.peek()); // Output: banana console.log(stack.pop()); // Output: banana console.log(stack.isEmpty()); // Output: false
In this example, we’ve defined a Stack
class with methods for adding and removing elements, as well as checking the top element and whether the stack is empty. This gives us more control over how our stack operates compared to using an array directly.
Linked Lists
A linked list is another common data structure that consists of a sequence of nodes, each containing data and a reference to the next node in the sequence. Here’s an example of how to implement a simple linked list in JavaScript:
class LinkedListNode { constructor(data) { this.data = data; this.next = null; } } class LinkedList { constructor() { this.head = null; } insertFirst(data) { const newNode = new LinkedListNode(data); newNode.next = this.head; this.head = newNode; } getFirst() { return this.head; } removeFirst() { if (!this.head) { return; } this.head = this.head.next; } // Additional methods can be added here to extend functionality } const list = new LinkedList(); list.insertFirst("apple"); list.insertFirst("banana"); console.log(list.getFirst().data); // Output: banana list.removeFirst(); console.log(list.getFirst().data); // Output: apple
In this example, we’ve created classes for both the individual nodes (LinkedListNode
) and the linked list itself (LinkedList
). We’ve implemented methods to insert and remove nodes at the beginning of the list, as well as to retrieve the first node.
Sets and Maps
Sets and Maps are also built-in JavaScript data structures, but like Arrays and Objects, you may need to extend their functionality or implement your own version for specific use cases. For example, you may want to implement a set that allows for more complex operations such as union, intersection, or difference between sets.
Implementing data structures in JavaScript allows developers to have greater control over their functionality and performance. It also provides an opportunity to practice algorithmic thinking and problem-solving skills. As you become more comfortable with these concepts, you can move on to more advanced algorithms and optimization techniques.
Advanced Algorithms and Optimization Techniques
When it comes to advanced algorithms and optimization techniques in JavaScript, there are several strategies that can significantly improve the performance and efficiency of your code. These techniques are important for tackling complex problems and handling large datasets. Here, we’ll discuss some of the advanced algorithms and optimization methods that every JavaScript developer should know.
Dynamic Programming: Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It is applicable where the problem can be divided into overlapping subproblems that can be solved independently. A classic example of dynamic programming is the Fibonacci sequence, where each number is the sum of the two preceding ones. Here’s how you can implement it in JavaScript:
function fibonacci(n, memo = {}) { if (n in memo) return memo[n]; if (n = 2) return 1; memo[n] = fibonacci(n - 1, memo) + fibonacci(n - 2, memo); return memo[n]; }
Greedy Algorithms: Greedy algorithms are used for optimization problems where the best solution is built up piece by piece. The concept is to choose the ‘locally optimal solution’ at each step with the hope of finding the global optimum. An example of a greedy algorithm is the coin change problem, where you want to make change for a certain amount of money with the fewest coins possible.
function coinChange(coins, amount) { let result = 0; coins.sort((a, b) => b - a); for (let i = 0; i coins.length; i++) { while (amount >= coins[i]) { amount -= coins[i]; result++; } if (amount === 0) break; } return result; }
Backtracking: Backtracking is an algorithmic technique for solving recursive problems by trying to build a solution incrementally. When a part of the solution doesn’t work, backtracking allows you to go back and try another path. A common example of backtracking is the N-Queens problem, where you need to place N queens on an NxN chessboard so that no two queens threaten each other.
Divide and Conquer: This technique involves dividing a problem into smaller subproblems, solving each subproblem independently, and then combining their results to solve the original problem. Merge Sort is an example of a divide and conquer algorithm, where the array is recursively split in half until it can no longer be divided, then merged back together in sorted order.
function mergeSort(arr) { if (arr.length = 1) return arr; const mid = Math.floor(arr.length / 2); const left = mergeSort(arr.slice(0, mid)); const right = mergeSort(arr.slice(mid)); return merge(left, right); } function merge(left, right) { let result = [], leftIndex = 0, rightIndex = 0; while (leftIndex left.length && rightIndex right.length) { if (left[leftIndex] right[rightIndex]) { result.push(left[leftIndex]); leftIndex++; } else { result.push(right[rightIndex]); rightIndex++; } } return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex)); }
These are just a few examples of advanced algorithms and optimization techniques in JavaScript. By understanding and applying these concepts, you can write code that not only solves complex problems but does so efficiently and effectively.
Source: https://www.plcourses.com/javascript-data-structures-and-algorithms/