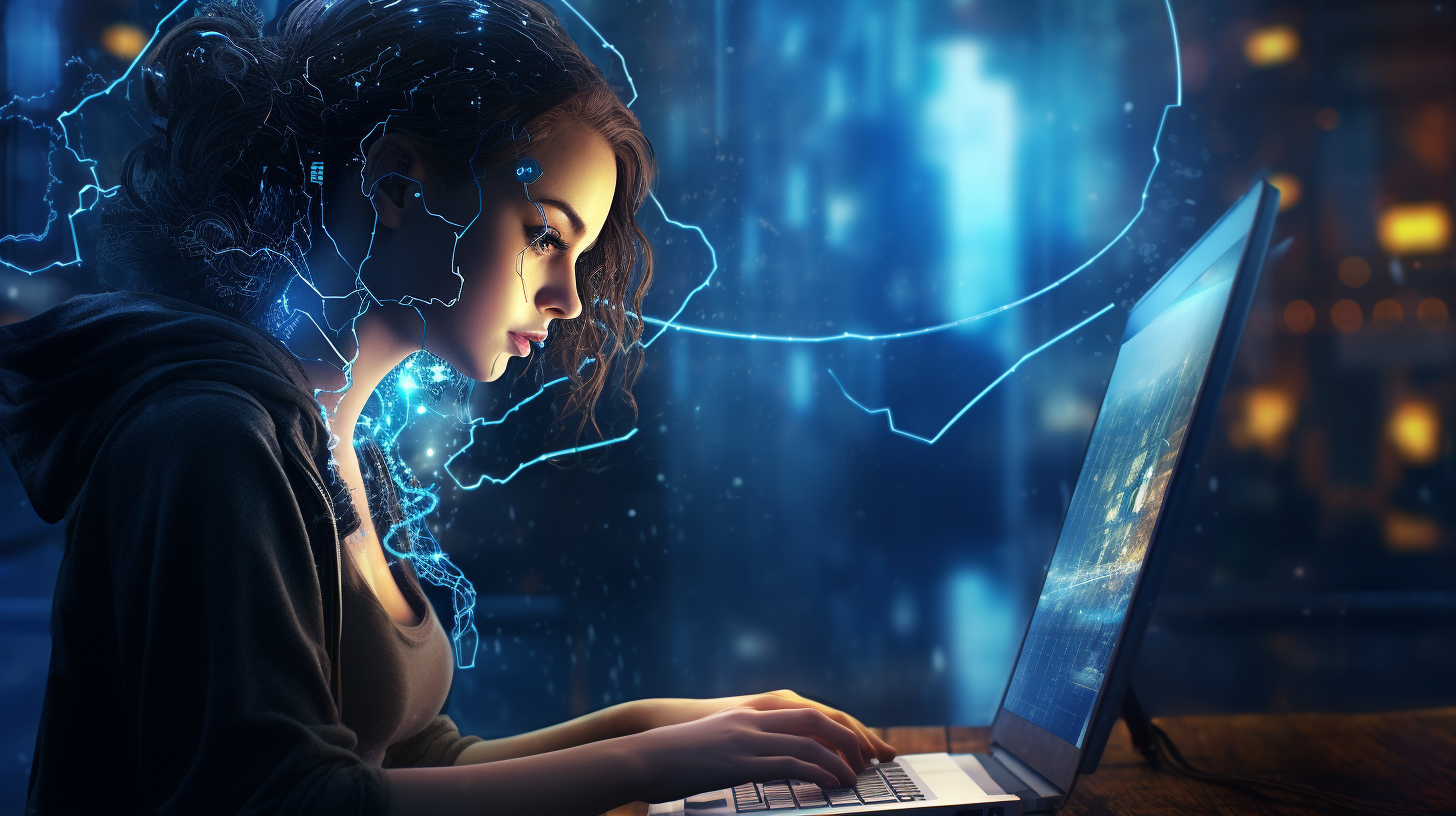
When it comes to network operations, Bash is a powerful tool that allows you to automate various tasks and interact with network resources. In this tutorial, we will explore how to perform network operations using Bash, including making HTTP requests, downloading files, and interacting with web APIs.
Making HTTP Requests
To make HTTP requests in Bash, you can use either the curl or wget command-line tools. Both tools are widely used and offer different features and capabilities.
Using curl
curl
is a versatile command-line tool for making HTTP requests. It supports a wide range of protocols and provides various options for customizing the request.
Here’s an example of making a GET request using curl
:
curl https://api.example.com/posts
This command sends a GET request to the specified URL and returns the response body. You can also specify additional options, such as headers, authentication, and request types.
Using wget
wget
is another popular command-line tool for downloading files from the web. It supports HTTP, HTTPS, and FTP protocols and provides options for customizing the download process.
Here’s an example of downloading a file using wget
:
wget https://example.com/file.txt
This command downloads the specified file and saves it in the current directory. You can also specify options like output file name, download location, and authentication credentials.
Interacting with Web APIs
Bash can be used to interact with web APIs and retrieve data in JSON or XML formats. This allows you to automate data retrieval and integration with other systems.
Processing JSON Responses
Bash doesn’t have built-in support for JSON parsing, but it can leverage external tools like jq to parse and manipulate JSON responses.
Here’s an example of using curl
and jq
to fetch and extract data from a web API:
json_response=$(curl -s https://api.example.com/posts) title=$(echo $json_response | jq -r '.[0].title')
This code retrieves the response from the API, extracts the title of the first post using jq
, and stores it in the title
variable.
Sending POST Requests
In addition to GET requests, you can also send POST requests to web APIs using Bash. That’s useful when you need to send data to a server or create new resources.
Here’s an example of sending a POST request using curl
:
curl -X POST -H "Content-Type: application/json" -d '{"name": "John", "age": 30}' https://api.example.com/users
This command sends a POST request with a JSON payload to create a new user on the server. You can customize the payload and headers based on your API requirements.
Bash provides powerful capabilities for performing various network operations, such as making HTTP requests, downloading files, and interacting with web APIs. The curl
and wget
command-line tools, along with external tools like jq
, can be used to automate and streamline network-related tasks. By leveraging Bash, you can enhance your automation workflows and efficiently manage network resources.
Source: https://www.plcourses.com/network-operations-using-bash/