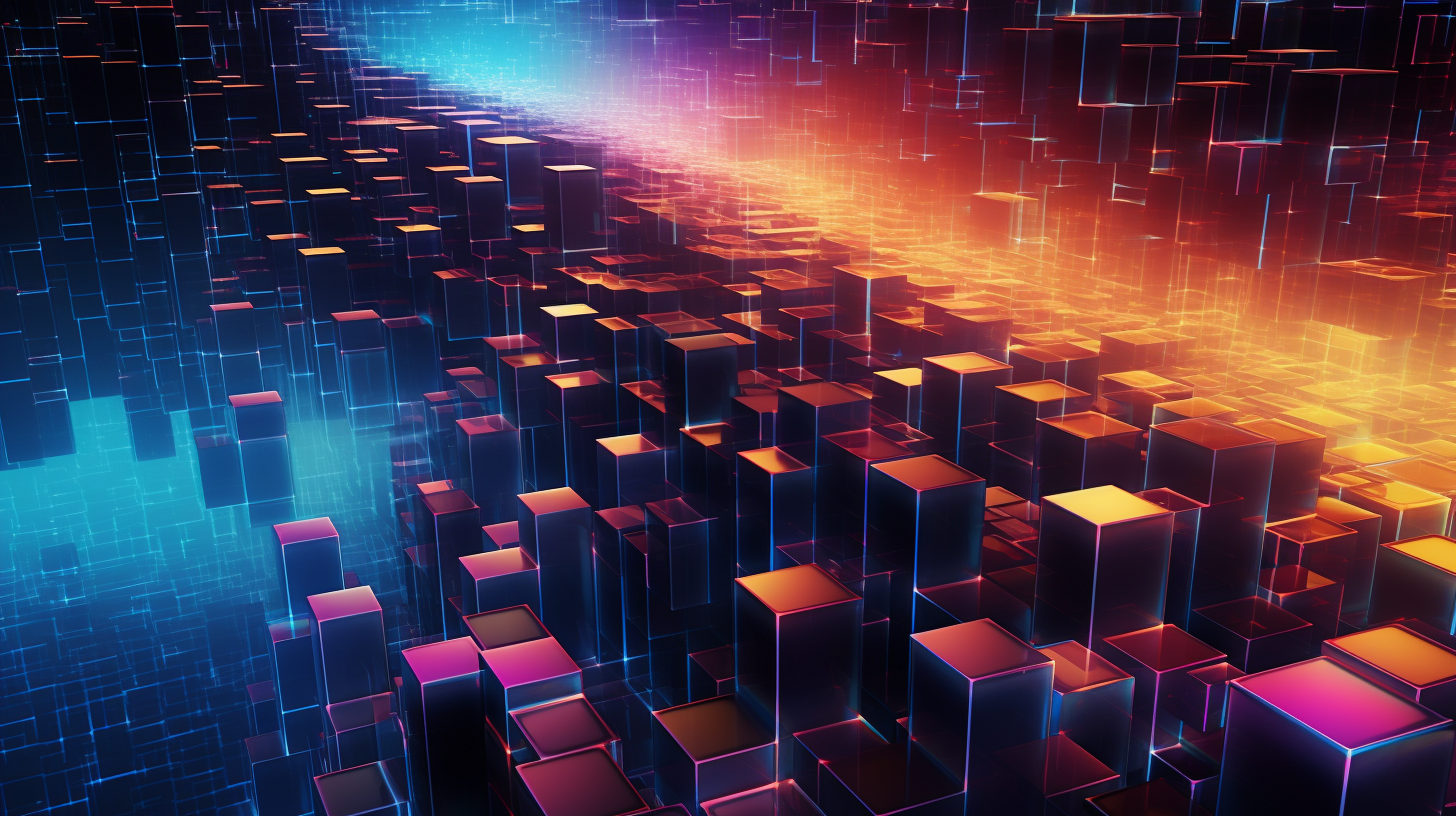
SQL and XML are two powerful technologies for managing and retrieving data. SQL is the standard language for managing relational databases, while XML is a flexible markup language used to structure, store, and transport data. When combined, SQL and XML provide a robust solution for working with complex and hierarchical data structures.
XML Data Management in SQL
Most contemporary databases support the storage of XML data. This allows for the creation of hybrid databases that can store both structured (relational) and semi-structured (XML) data. To manage XML data in SQL, you can use the XML
data type, which is available in many RDBMS like SQL Server, Oracle, PostgreSQL and more.
To create a table with an XML column in SQL Server, you can use the following code:
CREATE TABLE xml_table ( id INT PRIMARY KEY, xdata XML );
Once you have an XML column, you can insert XML data either as a plain string or using specific XML methods provided by the database system. For example:
INSERT INTO xml_table (id, xdata) VALUES (1, ''); value
Retrieving XML Data
Retrieving XML data from the database can be done with standard SQL queries. However, to work with the contents of the XML data, you’ll need to use functions and methods that are specific to XML processing. Here are some examples:
- Extracting Element Values: Use the
.value()
method in SQL Server orXMLTABLE
in Oracle to retrieve specific element values from XML.
-- SQL Server SELECT xdata.value('(/root/child)[1]', 'VARCHAR(50)') as child_value FROM xml_table WHERE id=1; -- Oracle SELECT xt.child_value FROM xml_table, XMLTABLE('/root/child' PASSING xdata COLUMNS child_value VARCHAR2(50) PATH '.') as xt WHERE id = 1;
.query()
method to retrieve specific elements within the XML data that match certain criteria.SELECT xdata.query('/root/child[text()="value"]') as result FROM xml_table WHERE id=1;
.modify()
method allows you to insert, update, or delete parts of the XML data.UPDATE xml_table SET xdata.modify('replace value of (/root/child/text())[1] with "new value"') WHERE id=1;
OPENXML
in SQL Server or XMLTABLE
in Oracle, you can transform XML data into relational rows and columns for easier querying and integration with traditional SQL queries.-- SQL Server SELECT * FROM OPENXML (@idoc,/root/child) WITH (child_value VARCHAR(50) 'text()'); -- Oracle SELECT xt.child_value FROM xml_table, XMLTABLE('/root/child' PASSING xdata COLUMNS child_value VARCHAR2(50) PATH '.') as xt;
In summary, combining SQL and XML offers numerous advantages for managing and accessing complex and hierarchical data in a structured manner. Whether it’s through creating tables with XML columns, extracting element values, or shredding XML into relational format, there are various tools within SQL that make it possible to effectively handle XML data.
By understanding these concepts and applying the appropriate code examples, developers can leverage the full power of both SQL and XML to meet their data management and retrieval needs.
Source: https://www.plcourses.com/sql-and-xml-data-management-and-retrieval/