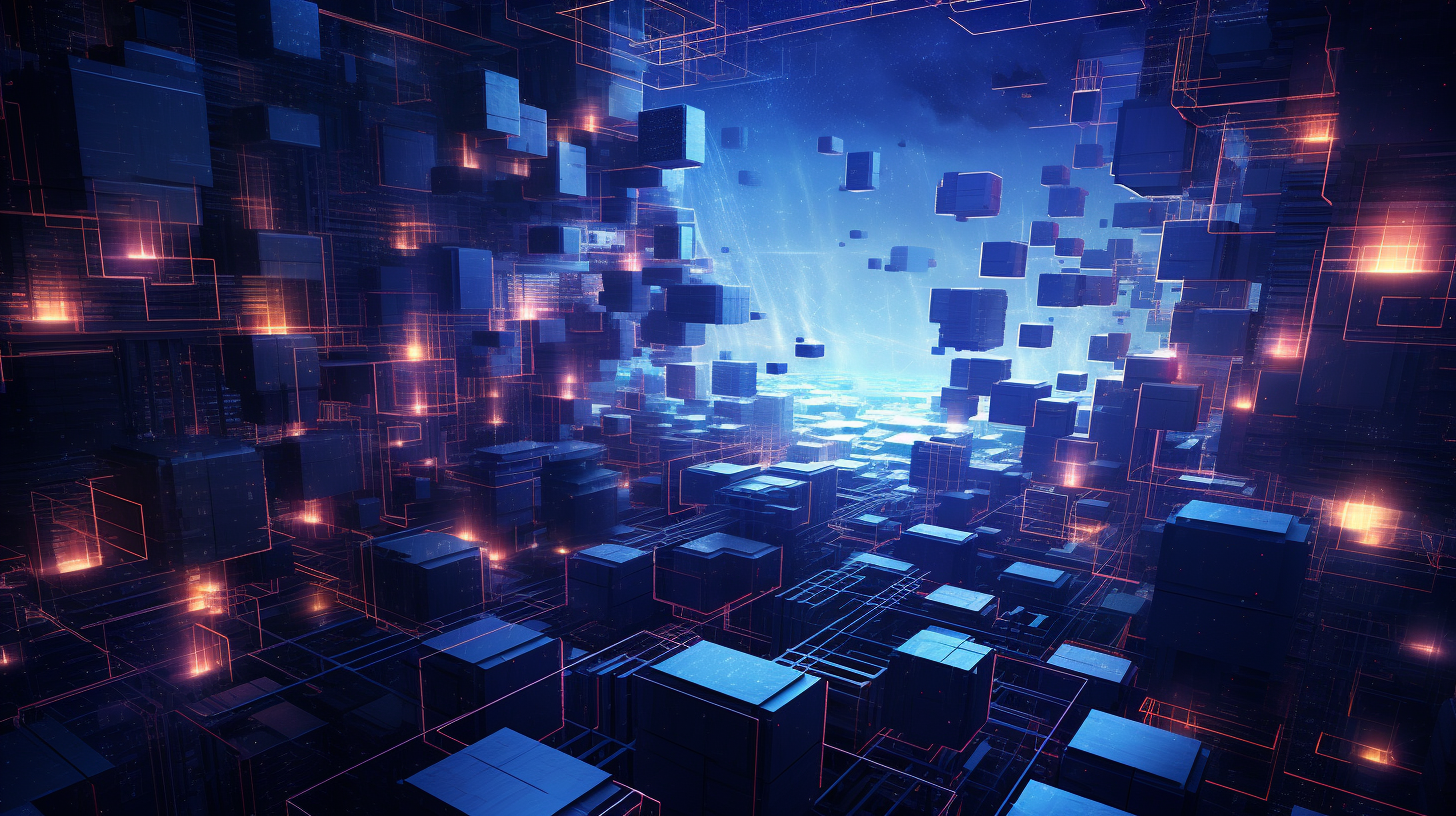
Introduction to JavaScript Programming
JavaScript is a powerful programming language that’s commonly used to create interactive effects within web browsers. It’s a versatile language that can be used for a wide range of tasks, from simple form validation to complex web applications.
One of the key features of JavaScript is its ability to manipulate the Document Object Model (DOM), which is the structure of an HTML document. With JavaScript, you can dynamically change the content, structure, and style of a webpage.
document.getElementById("demo").innerHTML = "Hello, World!";
This line of code selects an element with the id of “demo” and changes its inner HTML to “Hello, World!”.
JavaScript also has the ability to respond to user events, such as clicks, mouse movements, and keyboard input. This allows developers to create interactive and responsive user interfaces.
document.getElementById("myButton").addEventListener("click", function() { alert("Button clicked!"); });
This code adds an event listener to a button with the id of “myButton” and displays an alert when the button is clicked.
Another important aspect of JavaScript is its use of functions. Functions are blocks of code that can be defined once and called multiple times. They allow for code reuse and can make your code more organized and easier to read.
function sayHello(name) { alert("Hello, " + name + "!"); } sayHello("Alice"); sayHello("Bob");
This code defines a function called “sayHello” that takes a parameter called “name” and displays an alert with a greeting. The function is then called twice with different arguments.
JavaScript also has a variety of built-in objects and methods that can be used to perform common tasks, such as working with dates, math calculations, and text manipulation.
- Date Object: Used to work with dates and times.
- Math Object: Provides mathematical functions and constants.
- String Methods: Used to manipulate and work with strings of text.
var today = new Date(); console.log(today); var pi = Math.PI; console.log(pi); var greeting = "Hello, World!"; console.log(greeting.toUpperCase());
In this example, we create a new date object, log the value of pi from the Math object, and use the toUpperCase method to convert a string to uppercase letters.
Overall, JavaScript is a powerful and flexible language that is essential for contemporary web development. By understanding its core features and concepts, you can create dynamic and interactive web experiences for users.
Common Mistakes in JavaScript Coding
When learning JavaScript, it’s common for beginners to make mistakes that can lead to errors or unexpected behavior in their code. Understanding these mistakes can help you avoid them and write better, more efficient code. Here are some of the most common mistakes made by JavaScript developers:
-
Not Declaring Variables Properly
One of the most common mistakes is not declaring variables properly using
var
,let
, orconst
. This can lead to unexpected results due to variable hoisting.x = 5; // This is an implicit global variable because it's not declared. console.log(x); // Outputs: 5
Always declare your variables to avoid scope issues:
let x = 5; // This variable is properly declared and scoped. console.log(x); // Outputs: 5
-
Confusing Equality Operators
JavaScript has both abstract equality (==) and strict equality (===) operators. Using the wrong one can cause type coercion and lead to bugs in your code.
if (0 == '0') { console.log('0 is equal to "0"'); // This will be logged because == performs type coercion. } if (0 === '0') { console.log('0 is strictly equal to "0"'); // This will not be logged because === checks for both value and type. }
-
Ignoring Semicolons
Although JavaScript has automatic semicolon insertion, relying on it can cause issues. Omitting semicolons can lead to unexpected results, especially when minifying code.
let a = 3 let b = 4 (a + b).toString() // This might be interpreted as let a = 3; let b = 4(a + b).toString(); which will throw an error.
Always use semicolons to end your statements:
let a = 3; let b = 4; (a + b).toString(); // Correct
-
Misunderstanding Scope
Another mistake is misunderstanding the scope of variables, especially when it comes to the difference between global and local scope.
let globalVar = 'I am global'; function testScope() { let localVar = 'I am local'; console.log(globalVar); // Outputs: I am global } testScope(); console.log(localVar); // Throws an error: localVar is not defined
Remember that variables declared within a function are local to that function and not accessible outside of it.
-
Forgetting to Handle Errors
Not properly handling errors can lead to code that fails silently, which makes debugging much more difficult.
try { nonExistentFunction(); } catch(error) { console.error('An error occurred: ' + error.message); } // Outputs: An error occurred: nonExistentFunction is not defined
Always use try-catch blocks to handle errors gracefully.
-
Misusing the
this
KeywordThe
this
keyword in JavaScript can be confusing, especially when it comes to its value in different contexts such as event handlers or callbacks.const obj = { name: 'Alice', greet: function() { console.log('Hello, ' + this.name); } }; setTimeout(obj.greet, 1000); // Outputs: Hello, undefined // The value of 'this' inside setTimeout is the global object (window), not 'obj'.
To fix this, bind the correct context using
.bind()
:setTimeout(obj.greet.bind(obj), 1000); // Outputs: Hello, Alice
Making mistakes is a natural part of the learning process, but by being aware of these common errors, you can become a more proficient JavaScript developer. Remember to always test your code thoroughly and don’t hesitate to consult documentation or seek help from the community if you’re unsure about something.
Tips for Writing Efficient JavaScript Code
Writing efficient JavaScript code is not just about avoiding common mistakes; it’s also about adopting practices that improve the performance and readability of your code. Here are some tips to help you write more efficient JavaScript:
- Use Functions Wisely: Functions are the building blocks of JavaScript, but they can also be costly in terms of performance if not used properly. Avoid creating unnecessary functions, and ponder using arrow functions for cleaner and more concise code.
const add = (a, b) => a + b; console.log(add(2, 3)); // Outputs: 5
- Minimize DOM Manipulations: Interacting with the DOM can be expensive. Minimize the number of DOM updates by batching changes together, and use document fragments to insert multiple elements at once.
const fragment = document.createDocumentFragment(); const newDiv = document.createElement('div'); const newText = document.createTextNode('Hello, World!'); newDiv.appendChild(newText); fragment.appendChild(newDiv); document.body.appendChild(fragment);
- Optimize Loops: Loops can slow down your code if not optimized correctly. Use the most efficient loop for the task, and think breaking out of loops early if a condition is met.
for (let i = 0; i array.length; i++) { if (array[i] === targetValue) { console.log('Found at index:', i); break; // Exit the loop once the target value is found } }
- Avoid Global Variables: Global variables can lead to conflicts and make your code harder to maintain. Use local variables whenever possible and ponder using modules or closures to encapsulate your code.
(function() { let localVar = 'I am local'; console.log(localVar); // Outputs: I am local })(); // localVar is not accessible outside of the immediately invoked function expression (IIFE)
- Use Caching: Repeatedly accessing a property or performing a calculation can be inefficient. Cache results in variables when possible to avoid unnecessary work.
let element = document.getElementById('myElement'); for (let i = 0; i 1000; i++) { element.style.left = i + 'px'; // Accessing element.style once instead of in each iteration }
- Debounce and Throttle Events: Events like resizing or scrolling can fire many times in a short period, leading to performance issues. Use debouncing or throttling to limit the rate at which event handlers are called.
function debounce(func, wait) { let timeout; return function() { const later = function() { timeout = null; func.apply(this, arguments); }; clearTimeout(timeout); timeout = setTimeout(later, wait); }; } window.addEventListener('resize', debounce(function() { console.log('Resize event debounced!'); }, 250));
By following these tips, you’ll be on your way to writing more efficient and maintainable JavaScript code. Remember, efficient code is not only about performance but also about writing code that’s easy to understand and modify. Happy coding!
Debugging Techniques for JavaScript Beginners
Best Practices for Learning and Improving JavaScript Skills
Source: https://www.plcourses.com/javascript-for-beginners-common-mistakes-and-tips/